Using the Shopify API to Get Products (with Javascript examples)
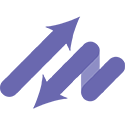
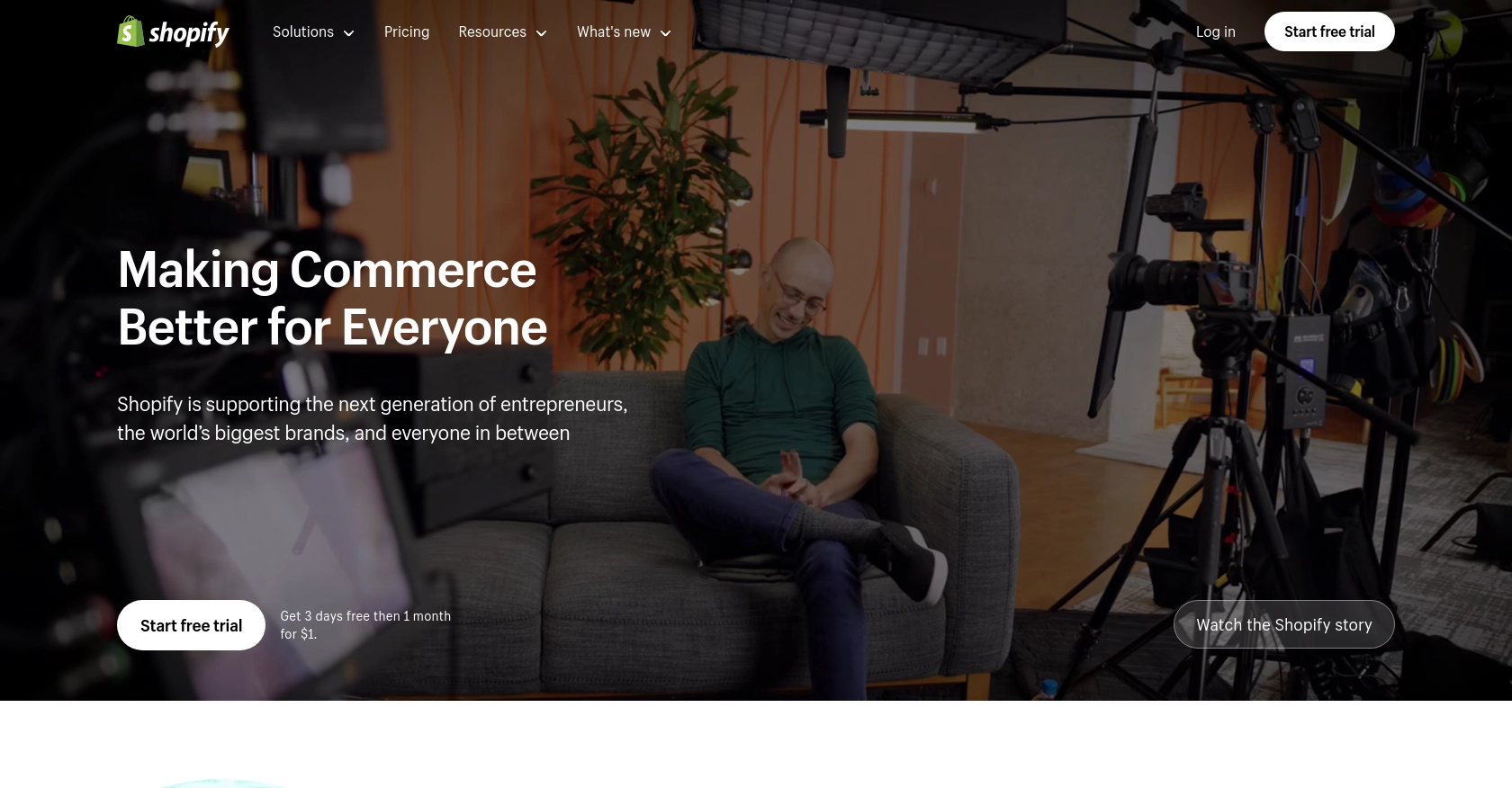
Introduction to Shopify API Integration
Shopify is a leading e-commerce platform that empowers businesses to create and manage online stores with ease. It offers a robust suite of tools and resources to help merchants sell products, manage inventory, and engage with customers effectively.
Integrating with Shopify's API allows developers to access and manipulate store data programmatically, enabling seamless automation and customization. For example, a developer might use the Shopify API to retrieve product information and display it on a custom storefront or synchronize inventory data with external systems.
This article will guide you through using JavaScript to interact with the Shopify API, specifically focusing on retrieving product data. By the end of this tutorial, you'll be equipped to efficiently access and manage product information on the Shopify platform using JavaScript.
Setting Up Your Shopify Test Account for API Integration
Before diving into the Shopify API, it's essential to set up a test environment. This allows you to experiment with API calls without affecting a live store. Shopify provides a development store option for this purpose, which is ideal for testing and building apps.
Creating a Shopify Development Store
- Visit the Shopify Partners Dashboard and sign up for a free account if you haven't already.
- Once logged in, navigate to the "Stores" section and click on "Add store."
- Select "Development store" as the store type. This option is specifically designed for testing and development purposes.
- Fill in the required details, such as store name and password, and click "Create store."
Your development store is now ready for testing API integrations.
Setting Up OAuth for Shopify API Access
Shopify uses OAuth 2.0 for authentication, allowing secure access to store data. Follow these steps to set up OAuth:
- In the Shopify Partners Dashboard, go to "Apps" and click "Create app."
- Enter your app name and select the development store you created earlier.
- Under "App setup," note the API key and API secret key. These credentials are essential for authenticating API requests.
- Set the "App URL" and "Redirect URL" to your app's URL. These are required for the OAuth flow.
- Under "Scopes," request the necessary permissions for your app. For accessing products, ensure you have the "read_products" scope.
- Save your changes.
With OAuth configured, you can now authenticate and make API requests to your Shopify development store.
Generating Access Tokens for API Requests
To interact with the Shopify API, you'll need an access token. Here's how to generate one:
- Direct the user to the following URL, replacing
{shop}
with your store's name and{api_key}
with your app's API key: - Upon authorization, Shopify will redirect to your specified redirect URI with a code parameter.
- Exchange this code for an access token by making a POST request to:
- Shopify will respond with an access token, which you can use to authenticate API requests.
https://{shop}.myshopify.com/admin/oauth/authorize?client_id={api_key}&scope=read_products&redirect_uri={redirect_uri}
https://{shop}.myshopify.com/admin/oauth/access_token
Include the following in the request body:
{
"client_id": "{api_key}",
"client_secret": "{api_secret}",
"code": "{code}"
}
With the access token, you're ready to start making authenticated requests to the Shopify API.
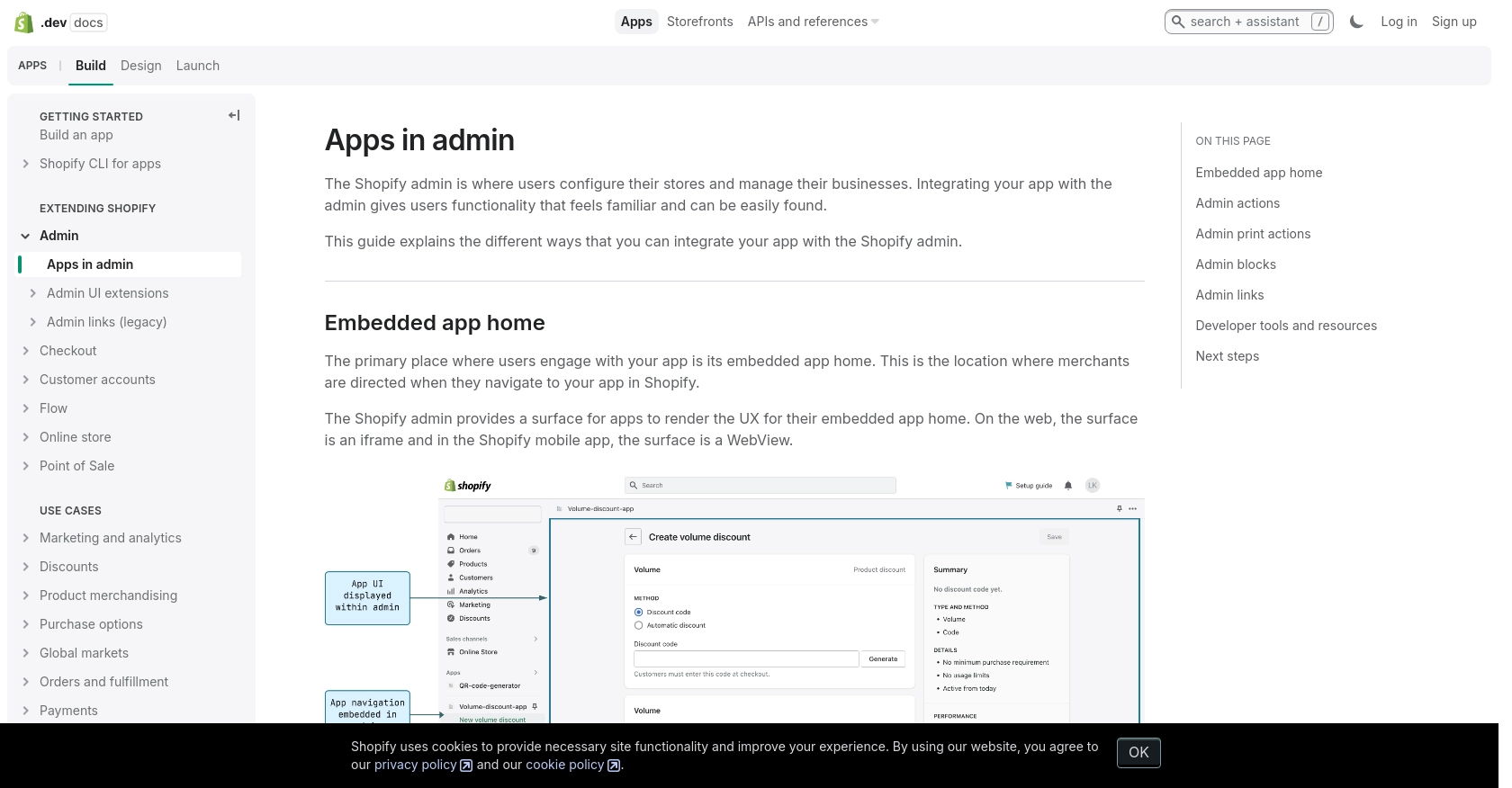
sbb-itb-96038d7
Making API Calls to Retrieve Shopify Products Using JavaScript
To interact with the Shopify API and retrieve product data, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling responses effectively.
Setting Up Your JavaScript Environment for Shopify API
Before making API calls, ensure you have a suitable JavaScript environment. You can use Node.js for server-side scripting or a browser-based environment for client-side operations. For this tutorial, we'll focus on using Node.js.
- Ensure you have Node.js installed on your machine. You can download it from the official Node.js website.
- Initialize a new Node.js project by running
npm init -y
in your terminal. - Install the
axios
library to handle HTTP requests by runningnpm install axios
.
Writing JavaScript Code to Fetch Products from Shopify
With your environment set up, you can now write the JavaScript code to fetch products from Shopify. Here's a step-by-step guide:
const axios = require('axios');
// Define the Shopify store and API version
const storeName = 'your-development-store';
const apiVersion = '2024-07';
// Set the API endpoint and headers
const endpoint = `https://${storeName}.myshopify.com/admin/api/${apiVersion}/products.json`;
const headers = {
'Content-Type': 'application/json',
'X-Shopify-Access-Token': 'your_access_token'
};
// Function to fetch products
async function fetchProducts() {
try {
const response = await axios.get(endpoint, { headers });
const products = response.data.products;
console.log('Products retrieved:', products);
} catch (error) {
console.error('Error fetching products:', error.response ? error.response.data : error.message);
}
}
// Call the function to fetch products
fetchProducts();
Replace your-development-store
with your store's name and your_access_token
with the access token you generated earlier.
Understanding and Handling Shopify API Responses
When you run the above code, the response will include a list of products from your Shopify store. You can verify the request's success by checking the console output for product details.
If the request fails, the error handling block will log the error message, helping you diagnose issues such as incorrect authentication or exceeding rate limits.
Verifying API Call Success in Shopify Dashboard
To ensure the API call was successful, you can cross-check the retrieved product data with the products listed in your Shopify development store's dashboard. This verification step is crucial for confirming that your integration is working as expected.
Handling Shopify API Errors and Rate Limits
Shopify's API has a rate limit of 40 requests per app per store per minute. If you exceed this limit, you'll receive a 429 Too Many Requests error. To handle this, implement a retry mechanism with exponential backoff.
Additionally, familiarize yourself with common error codes such as 401 Unauthorized and 403 Forbidden, which indicate authentication issues or insufficient permissions. For a complete list of error codes, refer to the Shopify API documentation.
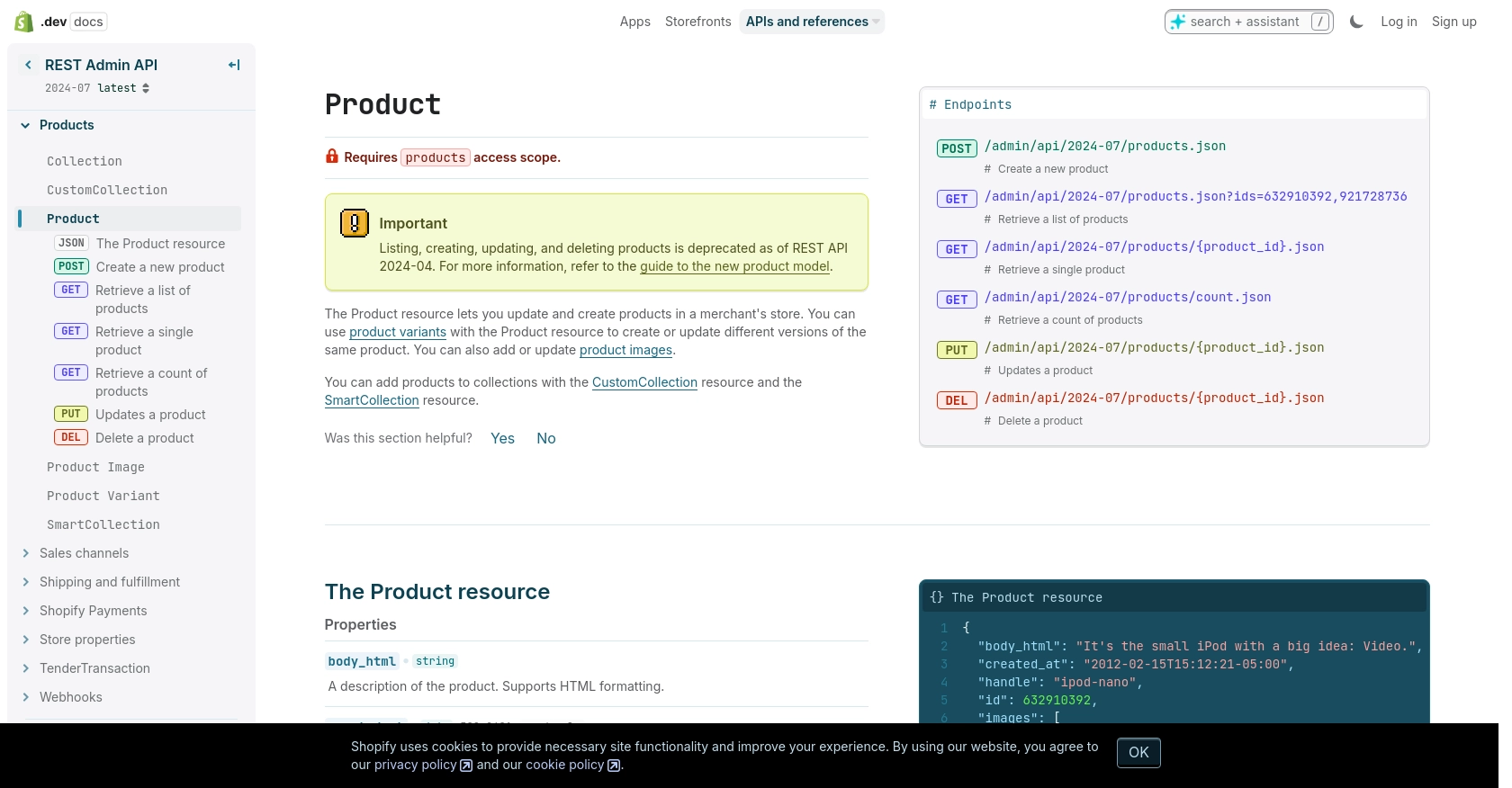
Conclusion and Best Practices for Shopify API Integration
Integrating with the Shopify API using JavaScript offers a powerful way to manage and automate your e-commerce operations. By following the steps outlined in this guide, you can efficiently retrieve product data and enhance your store's functionality.
Here are some best practices to consider when working with the Shopify API:
- Securely Store Credentials: Always store your API keys and access tokens securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limits: Shopify imposes a rate limit of 40 requests per app per store per minute. Implement a retry mechanism with exponential backoff to handle 429 Too Many Requests errors gracefully.
- Optimize Data Handling: When retrieving large datasets, use pagination to manage data efficiently and reduce the load on your application.
- Monitor API Usage: Regularly monitor your API usage to ensure compliance with Shopify's terms and to optimize your application's performance.
By adhering to these best practices, you can ensure a robust and efficient integration with Shopify's API, enhancing your store's capabilities and providing a seamless experience for your users.
Streamline Your Integrations with Endgrate
If you're looking to simplify your integration process, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?