How to Get Orders with the Younium API in PHP
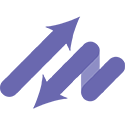
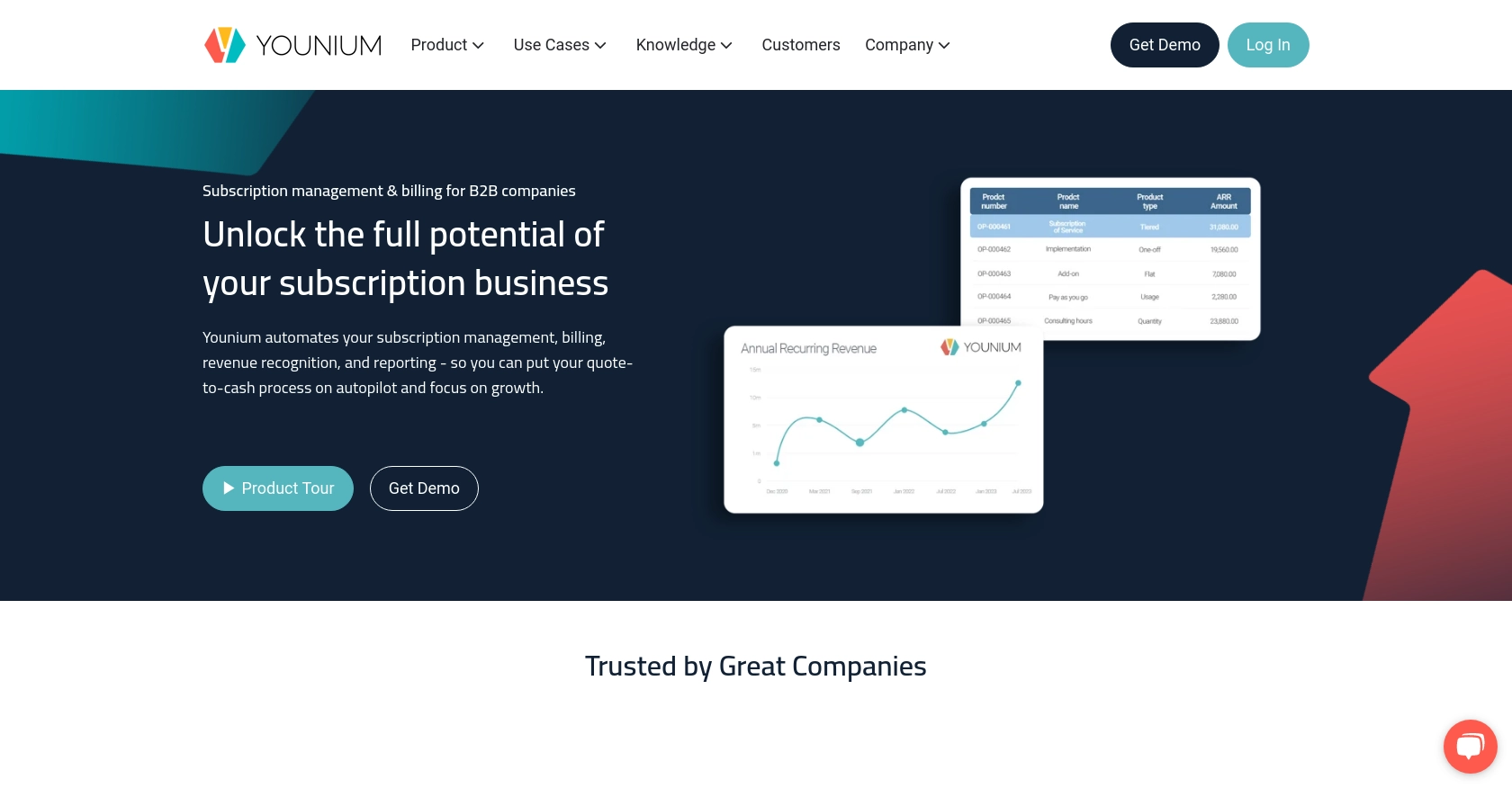
Introduction to Younium API Integration
Younium is a comprehensive subscription management platform designed to streamline billing and revenue operations for B2B SaaS companies. It offers robust features for managing subscriptions, invoicing, and financial reporting, making it an essential tool for businesses looking to optimize their financial processes.
Integrating with the Younium API allows developers to automate and enhance their subscription management workflows. For example, by accessing order data through the Younium API, a developer can automatically synchronize sales orders with their internal systems, ensuring accurate and up-to-date financial records.
Setting Up Your Younium Test/Sandbox Account
Before you can start interacting with the Younium API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting your production data.
Creating a Younium Sandbox Account
To begin, visit the Younium developer portal and sign up for a sandbox account. This account will provide you with the necessary environment to test your API integrations.
- Navigate to the Younium developer portal: Younium Developer Portal.
- Follow the instructions to create a sandbox account. If you already have an account, simply log in.
Generating API Token and Client Credentials
Once your sandbox account is set up, you'll need to generate an API token and client credentials to authenticate your API requests.
- Open the user profile menu by clicking your name in the top right corner and select “Privacy & Security”.
- In the left panel, click on “Personal Tokens” and then “Generate Token”.
- Provide a relevant description and click “Create”.
- Copy the generated Client ID and Secret Key. These credentials are crucial for generating the JWT access token and will not be visible again.
Acquiring a JWT Access Token
With your client credentials ready, you can now generate a JWT access token, which is required for authenticating API requests.
// Set the API endpoint for token generation
$url = 'https://api.sandbox.younium.com/auth/token';
// Prepare the request headers and body
$headers = [
'Content-Type: application/json'
];
$body = json_encode([
'clientId' => 'Your_Client_ID',
'secret' => 'Your_Secret_Key'
]);
// Use cURL to make the POST request
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $body);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
// Decode the response to get the access token
$data = json_decode($response, true);
$accessToken = $data['accessToken'];
Replace Your_Client_ID
and Your_Secret_Key
with the credentials you copied earlier. If successful, this code will return a JWT access token valid for 24 hours.
For more details on authentication, refer to the official documentation: Younium Authentication Guide.
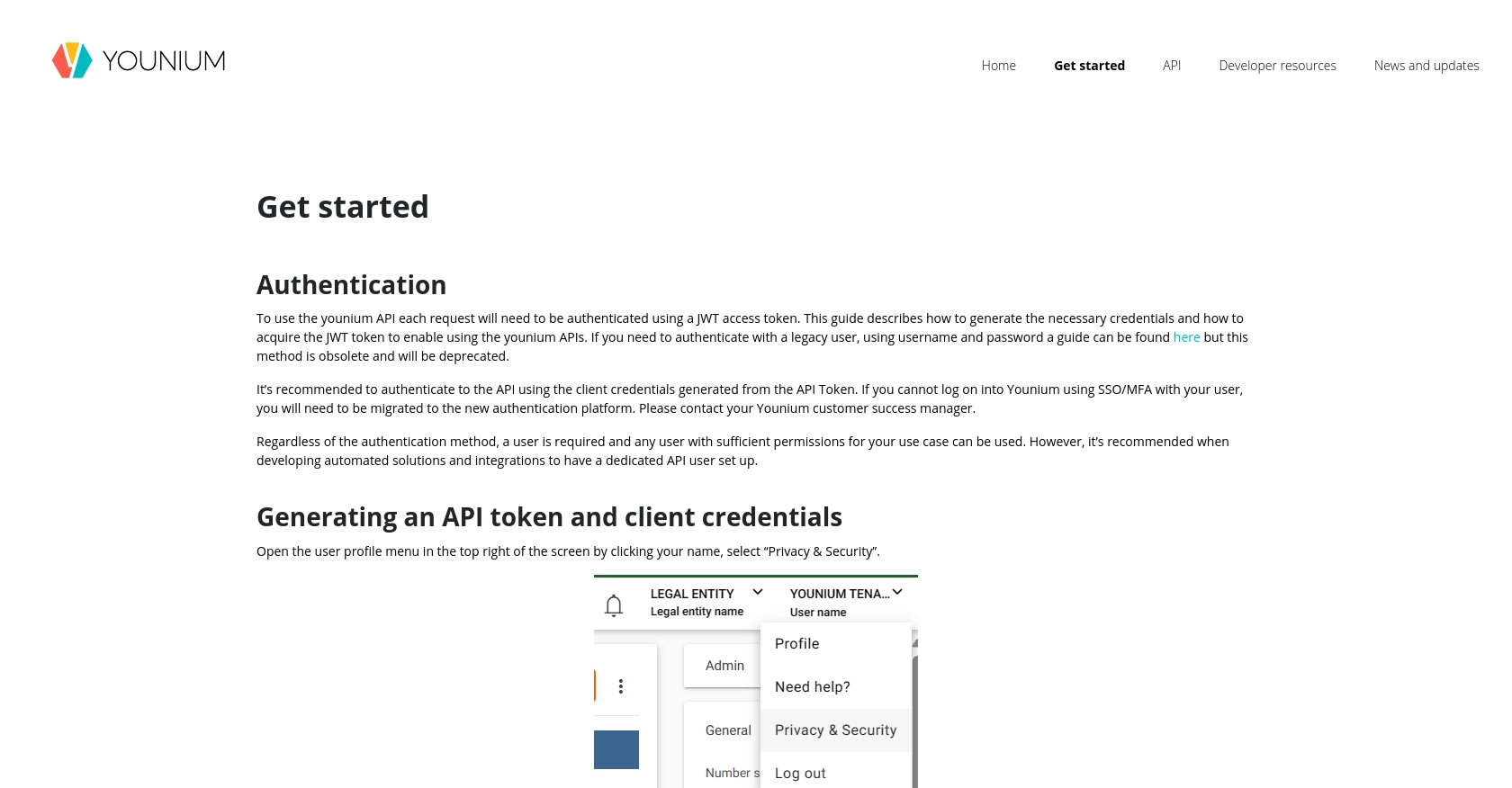
sbb-itb-96038d7
Making API Calls to Retrieve Orders with Younium API in PHP
Now that you have your JWT access token, you can proceed to make authenticated API calls to the Younium API to retrieve order data. This section will guide you through the process of setting up your PHP environment and executing the necessary API calls.
Setting Up Your PHP Environment for Younium API Integration
Before making API calls, ensure your PHP environment is properly configured. You will need:
- PHP 7.4 or higher
- cURL extension enabled
Verify your PHP version and ensure cURL is enabled by running the following command in your terminal:
php -v
php -m | grep curl
Executing the API Call to Get Sales Orders from Younium
With your environment set up, you can now write the PHP code to fetch sales orders from the Younium API.
// Set the API endpoint for retrieving sales orders
$url = 'https://api.sandbox.younium.com/salesorders';
// Prepare the request headers
$headers = [
'Authorization: Bearer ' . $accessToken,
'Content-Type: application/json',
'api-version: 2.1',
'legal-entity: Your_Legal_Entity_ID'
];
// Initialize cURL session
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error:' . curl_error($ch);
} else {
// Decode and process the response
$data = json_decode($response, true);
foreach ($data['data'] as $order) {
echo 'Order ID: ' . $order['id'] . "\n";
echo 'Order Number: ' . $order['orderNumber'] . "\n";
// Add more fields as needed
}
}
// Close cURL session
curl_close($ch);
Replace Your_Legal_Entity_ID
with your actual legal entity ID. This code will fetch and display sales orders from your Younium sandbox account.
Verifying Successful API Requests and Handling Errors
After executing the API call, verify the request's success by checking the response data. If the request is successful, you should see the sales orders listed in your sandbox account.
Handle potential errors by checking the HTTP status codes:
- 200 OK: The request was successful.
- 400 Bad Request: The request was malformed. Check your parameters.
- 401 Unauthorized: The access token is missing or invalid. Ensure your token is correct and not expired.
- 403 Forbidden: The legal entity is incorrect or permissions are insufficient. Verify your headers and permissions.
For more information on error handling, refer to the official API documentation: Younium API Call Documentation.
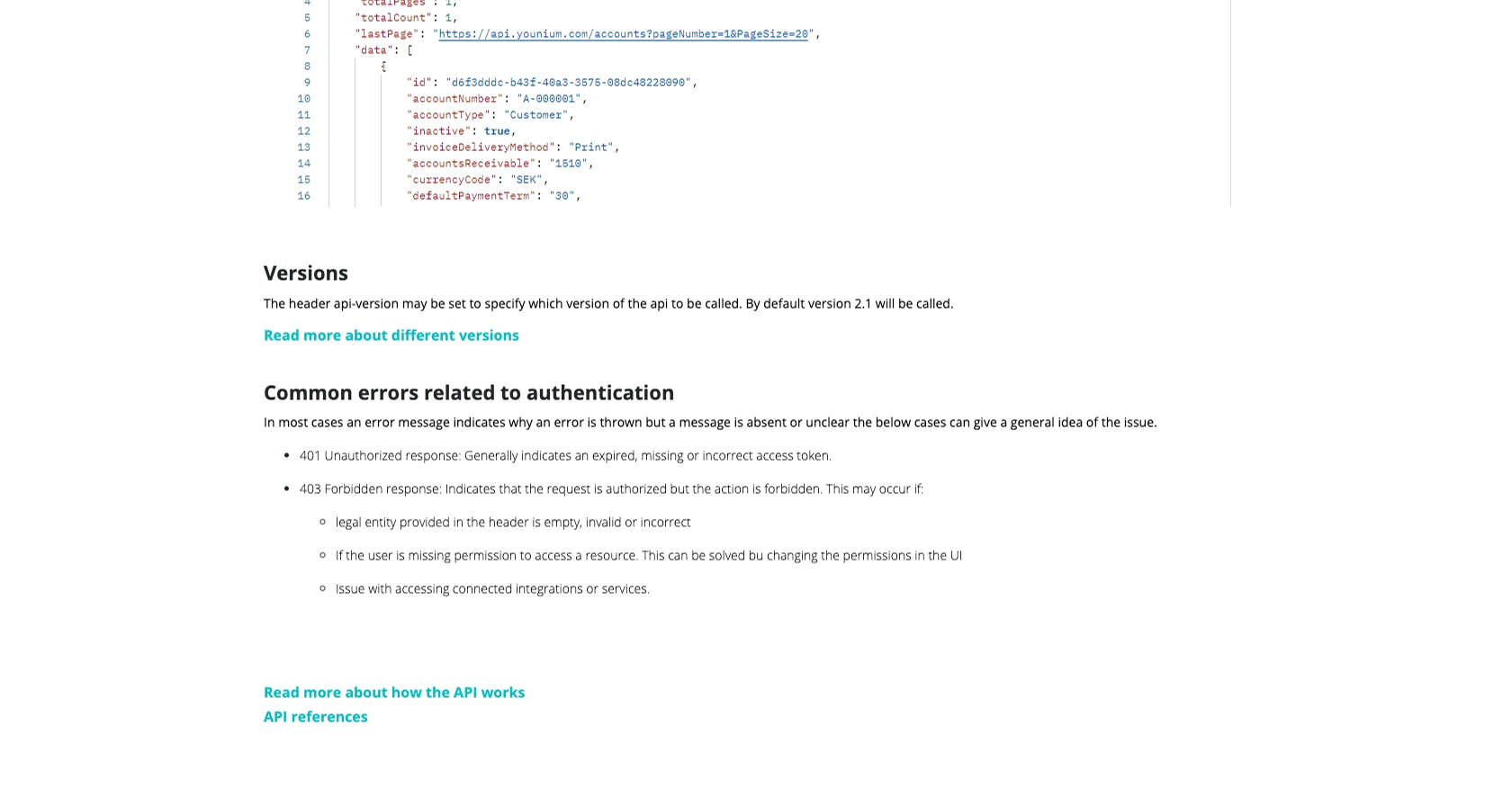
Conclusion and Best Practices for Younium API Integration in PHP
Integrating with the Younium API using PHP provides a powerful way to automate and streamline your subscription management processes. By following the steps outlined in this guide, you can efficiently retrieve sales orders and synchronize them with your internal systems, ensuring accurate and up-to-date financial records.
Best Practices for Secure and Efficient Younium API Usage
- Secure Storage of Credentials: Always store your client credentials and JWT access tokens securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Data Transformation: Standardize and transform data fields as needed to ensure compatibility with your internal systems. This will help maintain data integrity and consistency.
- Regular Token Refresh: Since the JWT access token is valid for 24 hours, implement a mechanism to refresh the token automatically to maintain uninterrupted API access.
Enhance Your Integration Strategy with Endgrate
While integrating with the Younium API can greatly enhance your subscription management capabilities, managing multiple integrations can be complex and time-consuming. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage a single API endpoint to connect with multiple platforms, including Younium. This not only saves time and resources but also provides an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration strategy by visiting Endgrate today.
Read More
Ready to get started?