How to Get Product Catalog with the Chargebee API in PHP
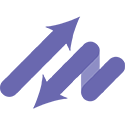
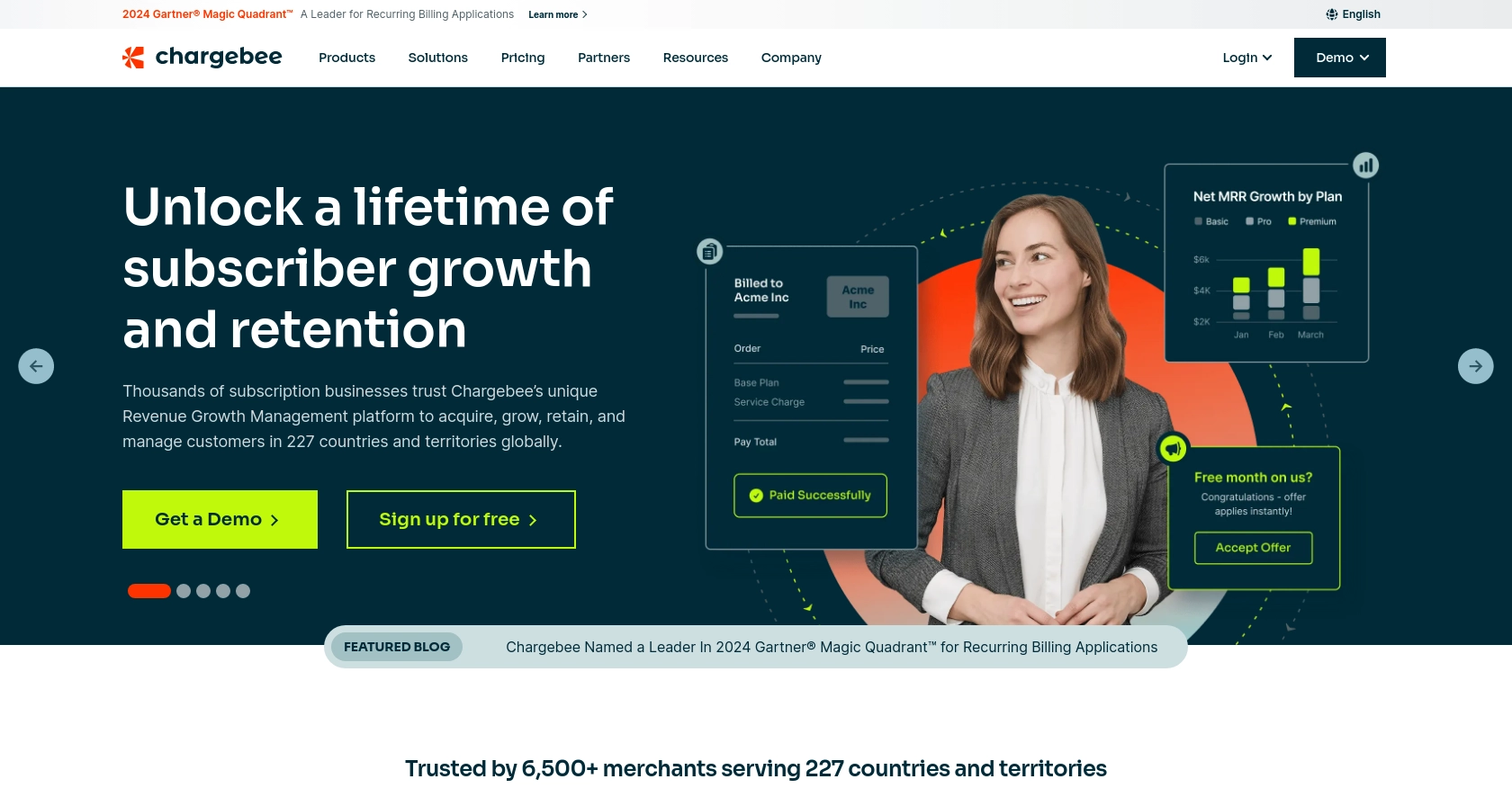
Introduction to Chargebee API for Product Catalog Management
Chargebee is a robust subscription billing and revenue management platform that empowers businesses to streamline their billing processes and manage subscriptions efficiently. With its comprehensive suite of tools, Chargebee is a preferred choice for SaaS companies looking to automate billing workflows and enhance customer experience.
Integrating with Chargebee's API allows developers to access and manage the product catalog, enabling seamless synchronization of product data across platforms. For example, a developer might use the Chargebee API to retrieve a list of available products and their pricing details, facilitating the creation of a dynamic pricing page on a website.
This article will guide you through the process of using PHP to interact with Chargebee's API, focusing on retrieving the product catalog. You'll learn how to set up your environment, authenticate API requests, and handle the data effectively.
Setting Up Your Chargebee Test Account for API Integration
Before you can start interacting with the Chargebee API, you'll need to set up a test account. Chargebee provides a sandbox environment that allows developers to experiment with API calls without affecting live data. This is an essential step for testing and development purposes.
Creating a Chargebee Sandbox Account
To begin, you'll need to create a Chargebee sandbox account. Follow these steps:
- Visit the Chargebee signup page and register for a free account.
- Once registered, log in to your Chargebee dashboard.
- Navigate to the 'Sites' section and create a new test site. This will serve as your sandbox environment.
Generating API Keys for Chargebee
Chargebee uses HTTP Basic authentication for API requests. You'll need an API key to authenticate your requests. Here's how to generate one:
- In your Chargebee dashboard, go to 'Settings' and select 'API Keys'.
- Click on 'Create a Key' and provide a name for your API key. This helps in identifying the key later.
- Choose the appropriate permissions for your API key. For accessing the product catalog, ensure you have read permissions.
- Once created, copy the API key. This will be used as the username in your API requests, with the password field left empty.
Remember, the API keys for your test site and live site are different. Ensure you are using the correct key for your sandbox environment.
Configuring OAuth for Chargebee API Access
While Chargebee primarily uses API keys for authentication, you can also configure OAuth for more secure access. Follow these steps if you prefer OAuth:
- In the Chargebee dashboard, navigate to 'Settings' and select 'OAuth Apps'.
- Click 'Create App' and fill in the required details, such as the app name and redirect URL.
- Once the app is created, note down the client ID and client secret. These will be used for OAuth authentication.
With your sandbox account and API keys set up, you're ready to start making API calls to Chargebee. In the next section, we'll explore how to retrieve the product catalog using PHP.
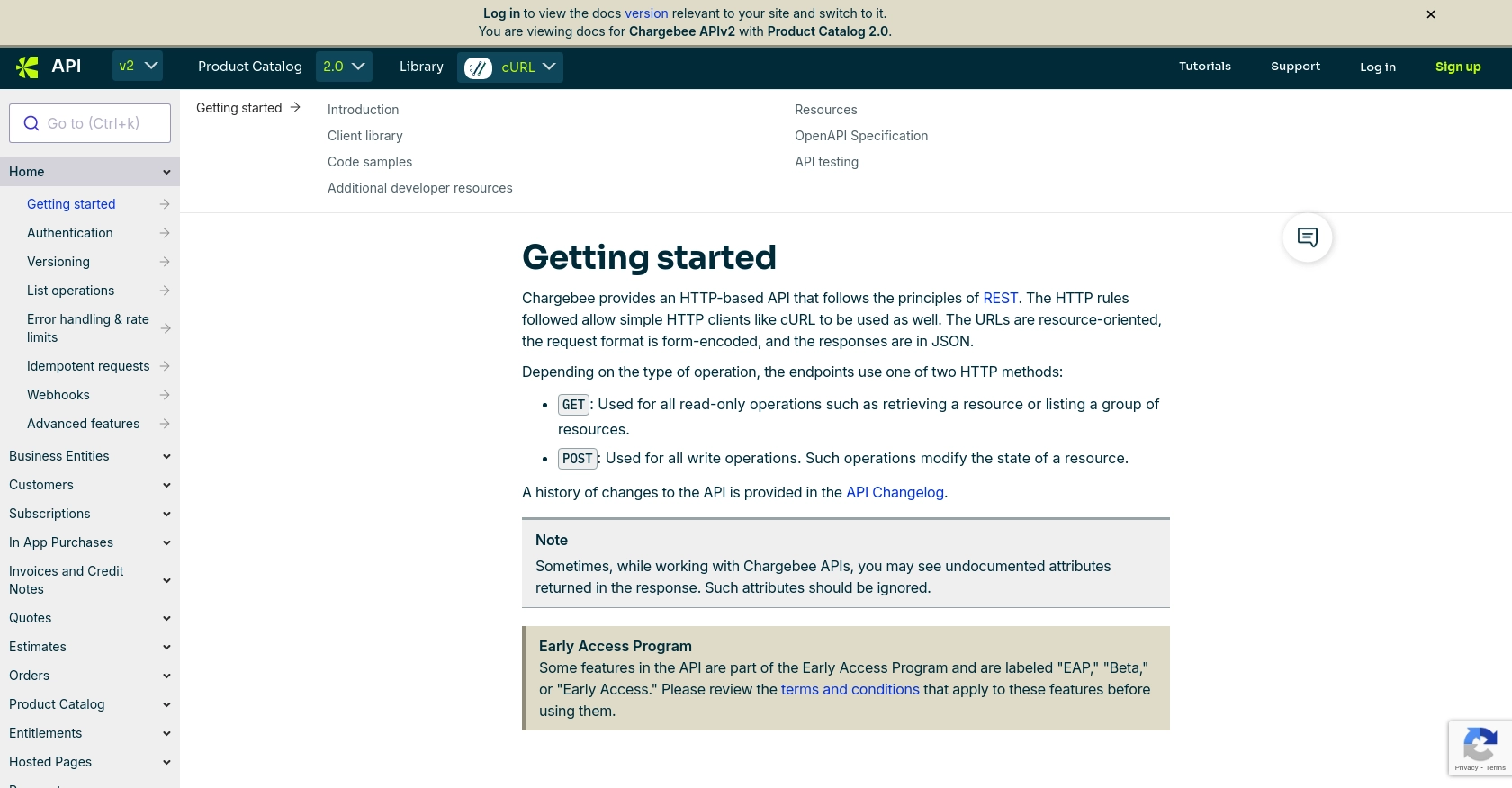
sbb-itb-96038d7
Making API Calls to Retrieve Product Catalog with Chargebee API in PHP
To interact with Chargebee's API using PHP, you'll need to ensure your environment is properly set up. This includes having the correct PHP version and necessary dependencies installed. In this section, we'll guide you through making an API call to retrieve the product catalog from Chargebee.
Setting Up Your PHP Environment for Chargebee API Integration
Before making API calls, ensure your PHP environment is ready:
- PHP version 7.4 or higher is recommended.
- Install the
cURL
extension for PHP, which is essential for making HTTP requests.
To install the cURL extension, you can use the following command:
sudo apt-get install php-curl
Writing PHP Code to Retrieve Product Catalog from Chargebee
With your environment set up, you can now write the PHP code to make an API call to Chargebee. Follow these steps:
- Create a new PHP file, e.g.,
get_product_catalog.php
. - Add the following code to make a GET request to Chargebee's API:
<?php
// Set your Chargebee site and API key
$site = 'your-site';
$apiKey = 'your-api-key';
// Initialize cURL session
$ch = curl_init();
// Set the API endpoint
$url = "https://{$site}.chargebee.com/api/v2/items";
// Set cURL options
curl_setopt($ch, CURLOPT_URL, $url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_USERPWD, $apiKey . ":");
curl_setopt($ch, CURLOPT_HTTPAUTH, CURLAUTH_BASIC);
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error:' . curl_error($ch);
} else {
// Decode the JSON response
$data = json_decode($response, true);
// Output the product catalog
foreach ($data['list'] as $item) {
echo "Item ID: " . $item['item']['id'] . "\n";
echo "Name: " . $item['item']['name'] . "\n";
echo "Type: " . $item['item']['type'] . "\n\n";
}
}
// Close cURL session
curl_close($ch);
?>
Replace your-site
and your-api-key
with your Chargebee site name and API key respectively.
Verifying API Call Success and Handling Errors
After running the script, you should see a list of items from your Chargebee product catalog. If the request fails, ensure:
- Your API key is correct and has the necessary permissions.
- The Chargebee site URL is accurate.
Chargebee uses standard HTTP status codes for error handling. For example, a 401 error indicates authentication failure. Refer to the Chargebee error handling documentation for more details.
By following these steps, you can successfully retrieve and manage your product catalog using Chargebee's API in PHP. This integration allows for seamless synchronization of product data across your platforms.
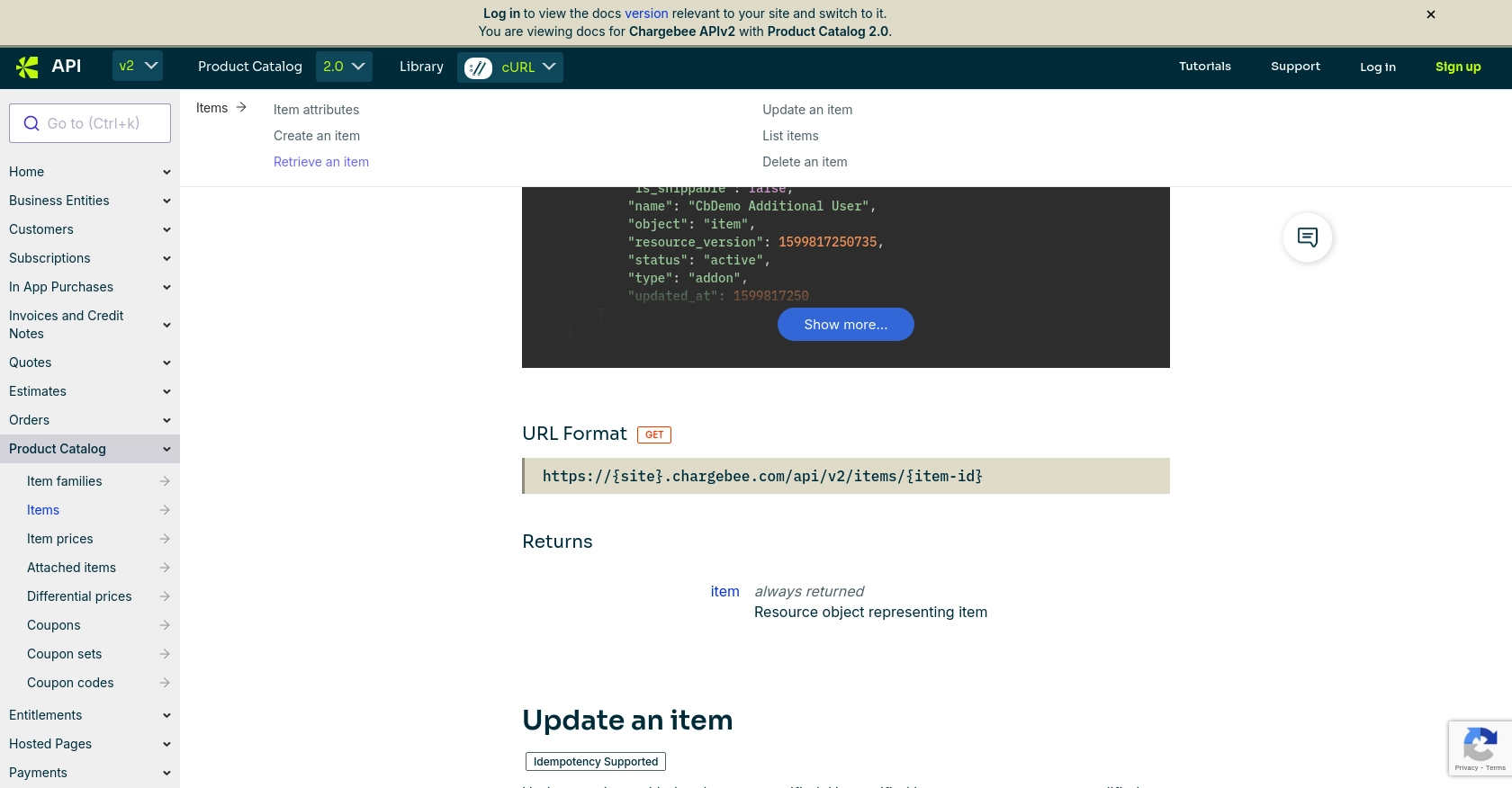
Best Practices for Chargebee API Integration in PHP
Successfully integrating with the Chargebee API in PHP requires adherence to best practices to ensure security, efficiency, and maintainability. Here are some recommendations:
- Secure API Keys: Store API keys securely and avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Chargebee imposes rate limits on API requests. Implement exponential backoff strategies and monitor the
Retry-After
header to manage request throttling effectively. For more details, refer to the Chargebee API rate limits documentation. - Error Handling: Implement robust error handling by checking HTTP status codes and parsing error messages. This ensures that your application can gracefully handle failures and provide meaningful feedback to users.
- Data Transformation: Standardize and transform data fields as needed to ensure consistency across your application and Chargebee's platform.
Leveraging Endgrate for Efficient Chargebee API Integrations
While integrating with Chargebee's API can be straightforward, managing multiple integrations across different platforms can become complex and time-consuming. This is where Endgrate can be a valuable asset.
Endgrate simplifies the integration process by providing a unified API endpoint that connects to multiple platforms, including Chargebee. By using Endgrate, you can:
- Save Time and Resources: Focus on your core product development while Endgrate handles the complexities of integration.
- Build Once, Use Everywhere: Develop a single integration for each use case and apply it across various platforms without redundant efforts.
- Enhance Customer Experience: Offer an intuitive and seamless integration experience to your customers, reducing friction and improving satisfaction.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate's website and discover the benefits of a unified integration approach.
Read More
Ready to get started?