Using the Constant Contact API to Create or Update Contacts in PHP
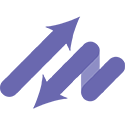
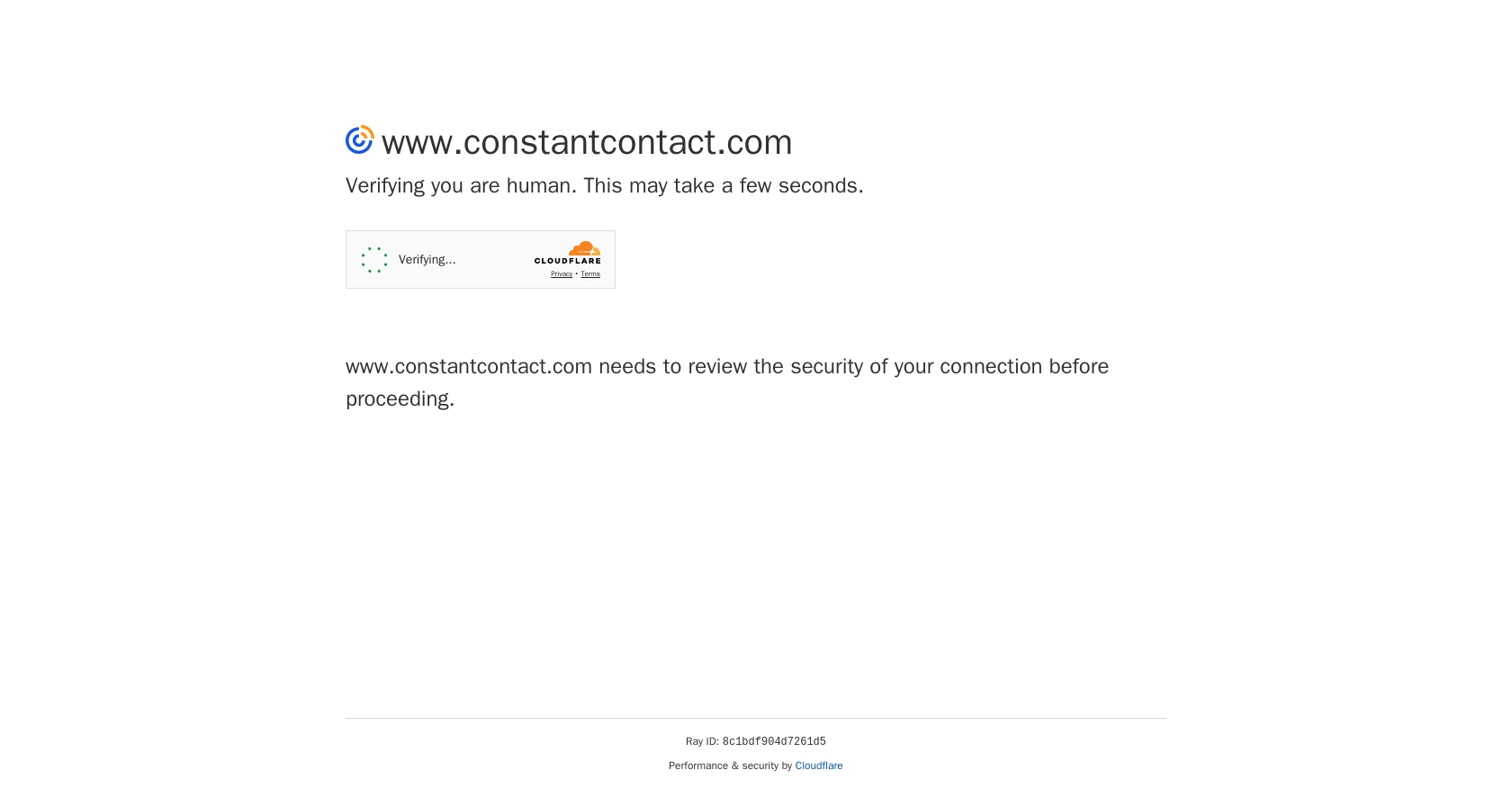
Introduction to Constant Contact API
Constant Contact is a powerful email marketing platform that enables businesses to create and manage effective email campaigns. With its robust set of tools, Constant Contact helps businesses engage with their audience, track campaign performance, and grow their customer base.
For developers, integrating with the Constant Contact API offers the opportunity to automate and enhance marketing efforts. By using the API, developers can programmatically create or update contact information, ensuring that customer data is always up-to-date and campaigns are targeted effectively.
For example, a developer might use the Constant Contact API to automatically update contact details from an external CRM system, ensuring seamless data synchronization and more personalized marketing communications.
Setting Up Your Constant Contact Developer Account
Before you can start integrating with the Constant Contact API, you'll need to set up a developer account. This will allow you to create an application and obtain the necessary credentials for OAuth2 authentication.
Creating a Constant Contact Developer Account
If you don't have a Constant Contact developer account, follow these steps to create one:
- Visit the Constant Contact Developer Portal.
- Click on "Sign Up" and fill out the required information to create your account.
- Once your account is created, log in to access the developer dashboard.
Creating an Application for OAuth2 Authentication
To interact with the Constant Contact API, you need to create an application that will provide you with a client ID and client secret. Follow these steps:
- Navigate to the "My Applications" tab in the developer dashboard.
- Click on "Create Application" and fill in the required details, such as the application name and description.
- Specify the redirect URI, which is where users will be redirected after they authorize your application.
- Save your application to generate the client ID and client secret.
Configuring OAuth2 Scopes
When creating your application, you'll need to specify the scopes that define the level of access your application requires. For creating or updating contacts, ensure you include the contact_data
scope.
Obtaining the Access Token
With your client ID and client secret, you can now obtain an access token to authenticate API requests:
- Direct users to the Constant Contact authorization endpoint to grant access to your application.
- Once authorized, exchange the authorization code for an access token by making a POST request to the token endpoint.
- Include the access token in the Authorization header of your API requests as
Bearer {your_access_token}
.
For more detailed information on setting up OAuth2 authentication, refer to the Constant Contact OAuth2 Documentation.
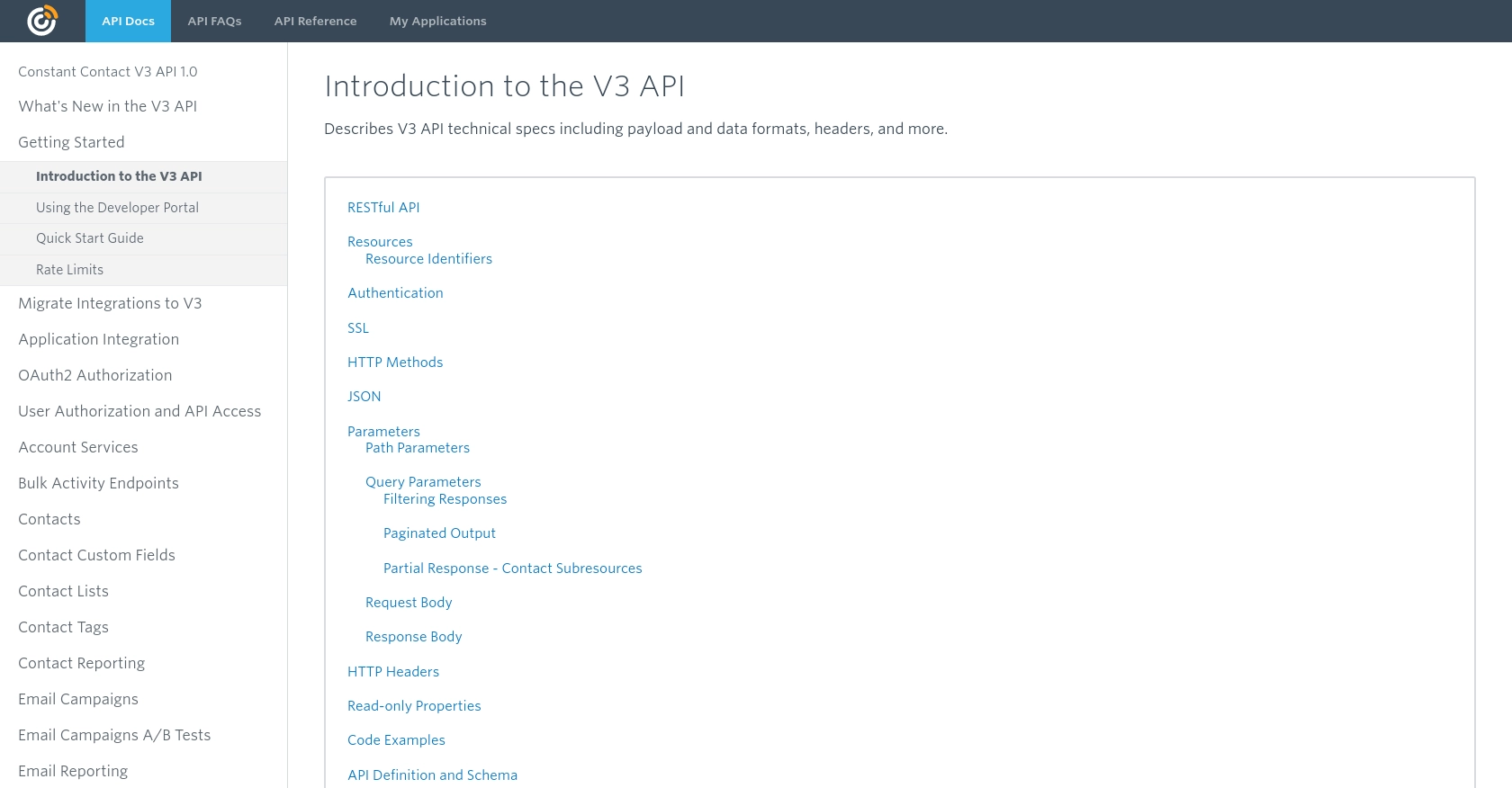
sbb-itb-96038d7
Making API Calls to Constant Contact Using PHP
To interact with the Constant Contact API using PHP, you'll need to ensure your environment is set up correctly. This involves using the right PHP version and installing necessary dependencies. Follow these steps to get started:
Setting Up Your PHP Environment
Before making API calls, ensure you have the following:
- PHP 7.4 or higher installed on your machine.
- Composer, the PHP package manager, to manage dependencies.
Install the Guzzle HTTP client, which simplifies making HTTP requests in PHP:
composer require guzzlehttp/guzzle
Creating or Updating Contacts with Constant Contact API
With your environment ready, you can now create or update contacts using the Constant Contact API. Here's a step-by-step guide:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token'; // Replace with your access token
// Define the API endpoint
$url = 'https://api.cc.email/v3/contacts';
// Set the contact data
$contactData = [
'email_address' => 'example@example.com',
'first_name' => 'John',
'last_name' => 'Doe'
];
// Make the API request
$response = $client->request('PUT', $url, [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Content-Type' => 'application/json'
],
'json' => $contactData
]);
// Check the response
if ($response->getStatusCode() == 200) {
echo "Contact created or updated successfully.";
} else {
echo "Failed to create or update contact.";
}
Replace Your_Access_Token
with the access token you obtained during the OAuth2 authentication process.
Verifying API Call Success in Constant Contact
After executing the script, verify the success of your API call by checking the Constant Contact dashboard. The contact should appear in your contact list with the updated information.
Handling Errors and Response Codes
It's crucial to handle potential errors when making API calls. The Constant Contact API uses standard HTTP response codes to indicate success or failure:
- 200 OK: The request was successful.
- 400 Bad Request: The request was malformed or invalid.
- 401 Unauthorized: The access token is invalid or expired.
- 403 Forbidden: Insufficient permissions or scopes.
- 429 Too Many Requests: Rate limit exceeded. Wait before retrying.
For more details on error handling, refer to the Constant Contact Response Codes Documentation.
Best Practices for Using Constant Contact API in PHP
When integrating with the Constant Contact API, it's essential to follow best practices to ensure efficient and secure operations. Here are some recommendations:
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Constant Contact imposes a rate limit of 10,000 requests per day and 4 requests per second. Implement logic to handle
429 Too Many Requests
responses by retrying after a delay. - Data Standardization: Ensure that contact data is standardized before sending it to the API. This includes validating email formats and ensuring consistency in name fields.
- Error Handling: Implement comprehensive error handling to manage different HTTP response codes. Log errors for debugging and provide user-friendly messages where applicable.
Conclusion and Call to Action for Using Endgrate
Integrating with the Constant Contact API can significantly enhance your marketing automation efforts by keeping contact data synchronized and up-to-date. However, managing multiple integrations can be time-consuming and complex.
Endgrate offers a streamlined solution for handling integrations, allowing you to focus on your core product. With Endgrate, you can build once for each use case and leverage a unified API endpoint for multiple platforms, including Constant Contact.
Visit Endgrate to learn how you can save time and resources by outsourcing your integration needs, providing an intuitive experience for your customers, and scaling your integrations efficiently.
Read More
- https://endgrate.com/provider/constantcontact
- https://developer.constantcontact.com/api_guide/v3_technical_overview.html
- https://developer.constantcontact.com/api_guide/auth_overview.html
- https://developer.constantcontact.com/api_guide/scopes.html
- https://developer.constantcontact.com/api_guide/glossary_responses.html
Ready to get started?