Using the Front API to Create Or Update Accounts (with PHP examples)
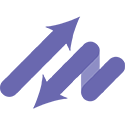
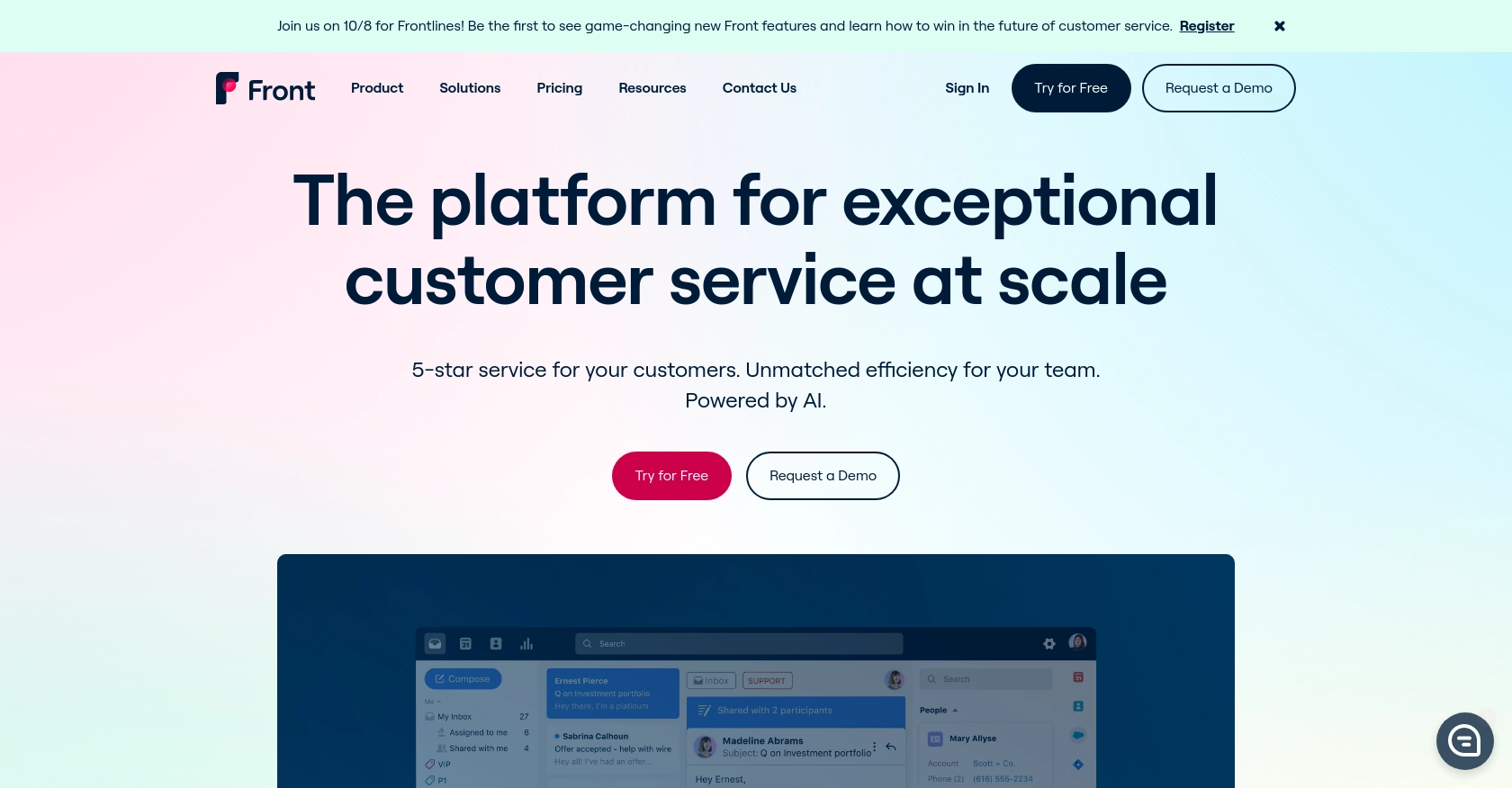
Introduction to Front API for Account Management
Front is a powerful communication platform that centralizes emails, messages, and other customer interactions into a single collaborative interface. It is widely used by businesses to enhance team collaboration and streamline customer communication processes.
Developers might want to integrate with Front's API to automate account management tasks, such as creating or updating customer accounts. For example, a developer could use the Front API to automatically update account information from an external CRM, ensuring that all customer data remains consistent and up-to-date across platforms.
Setting Up Your Front Developer Account for API Integration
Before you can start integrating with the Front API, you'll need to set up a developer account. This account will allow you to test your integration in a controlled environment, ensuring that your application interacts seamlessly with Front's platform.
Creating a Front Developer Account
If you don't already have a Front account, you can create a developer account by signing up on the Front website. This free developer environment provides all the necessary tools to begin building with Front's APIs.
- Visit the Front Developer Portal.
- Click on the sign-up link and follow the instructions to create your account.
- Once your account is created, you'll have access to a sandbox environment where you can test your API calls without affecting production data.
Generating an API Token for Authentication
Front uses API tokens for authentication, allowing secure access to its services. Follow these steps to generate an API token:
- Log in to your Front developer account.
- Navigate to the API tokens section in your account settings.
- Click on "Create API Token" and select the appropriate scopes for your integration. For account management, ensure you have access to the necessary resources.
- Copy the generated API token and store it securely, as it will be used to authenticate your API requests.
For more details on authentication, refer to the Front API Authentication Documentation.
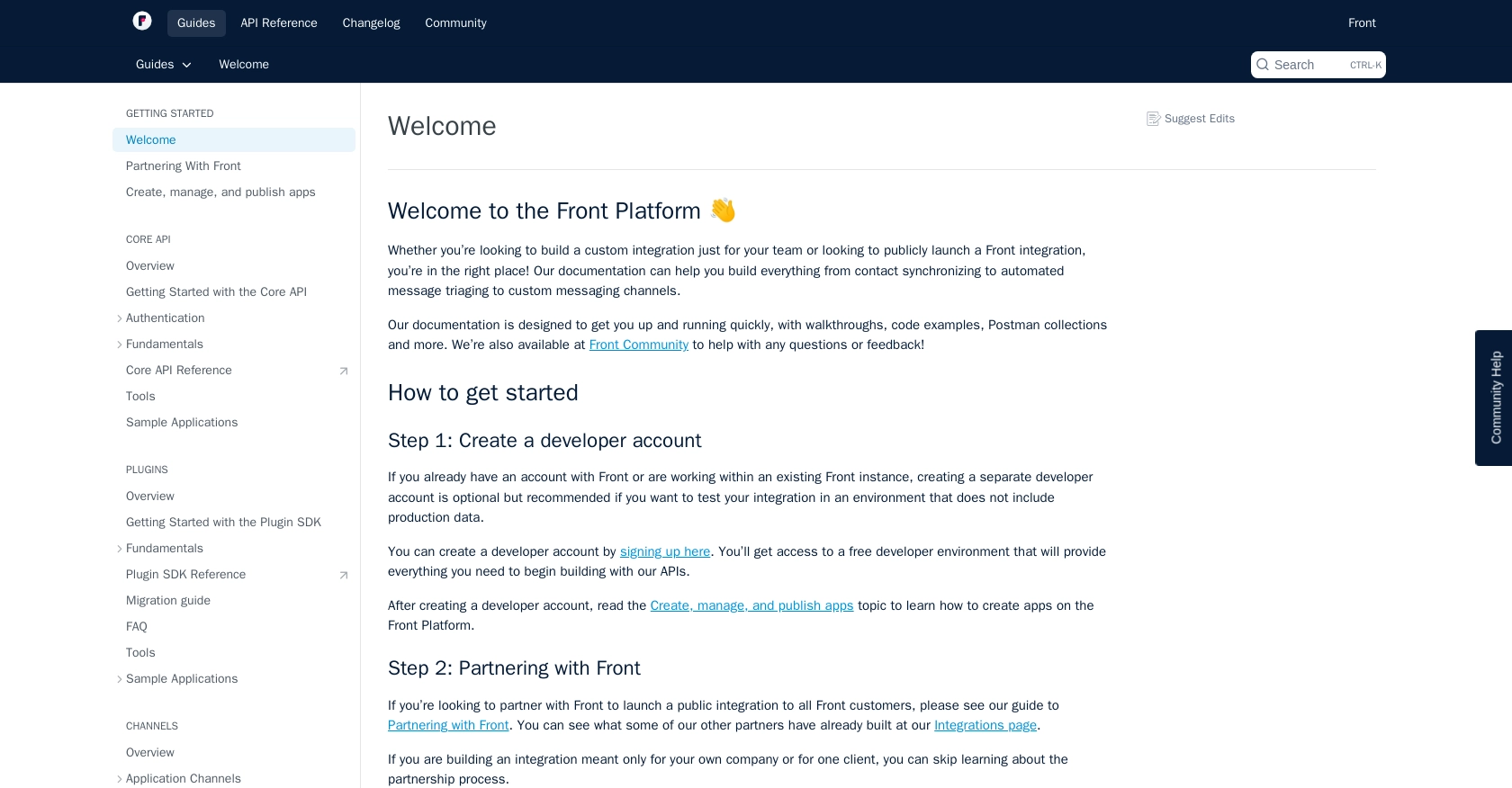
sbb-itb-96038d7
Making API Calls to Create or Update Accounts Using Front API with PHP
To interact with the Front API for creating or updating accounts, you'll need to use PHP to make HTTP requests. This section will guide you through the process of setting up your PHP environment, writing the necessary code, and handling responses from the API.
Setting Up Your PHP Environment for Front API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- PHP 7.4 or higher
- Composer (for managing dependencies)
Next, install the Guzzle HTTP client, which simplifies making HTTP requests in PHP:
composer require guzzlehttp/guzzle
Creating an Account with Front API Using PHP
To create an account, you'll need to send a POST request to the Front API's account creation endpoint. Here's a sample PHP script to achieve this:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiToken = 'YOUR_API_TOKEN';
$response = $client->post('https://api2.frontapp.com/accounts', [
'headers' => [
'Authorization' => 'Bearer ' . $apiToken,
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'json' => [
'name' => 'New Account Name',
'description' => 'Description of the account',
],
]);
echo $response->getBody();
Replace YOUR_API_TOKEN
with the API token you generated earlier. This script sends a POST request to create a new account with the specified name and description.
Updating an Account with Front API Using PHP
To update an existing account, you'll need to use the PATCH method. Here's how you can update an account's details:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiToken = 'YOUR_API_TOKEN';
$accountId = 'ACCOUNT_ID_TO_UPDATE';
$response = $client->patch("https://api2.frontapp.com/accounts/{$accountId}", [
'headers' => [
'Authorization' => 'Bearer ' . $apiToken,
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'json' => [
'name' => 'Updated Account Name',
'description' => 'Updated description of the account',
],
]);
echo $response->getStatusCode();
Replace ACCOUNT_ID_TO_UPDATE
with the ID of the account you wish to update. This script sends a PATCH request to modify the account's name and description.
Handling API Responses and Errors
After making an API call, it's crucial to handle the response correctly. Check the status code to verify if the request was successful:
- A status code of
201
indicates successful account creation. - A status code of
204
indicates successful account update.
In case of errors, the API might return status codes like 400
for bad requests or 401
for unauthorized access. Handle these errors gracefully in your application.
For more details on error handling, refer to the Front API Documentation.
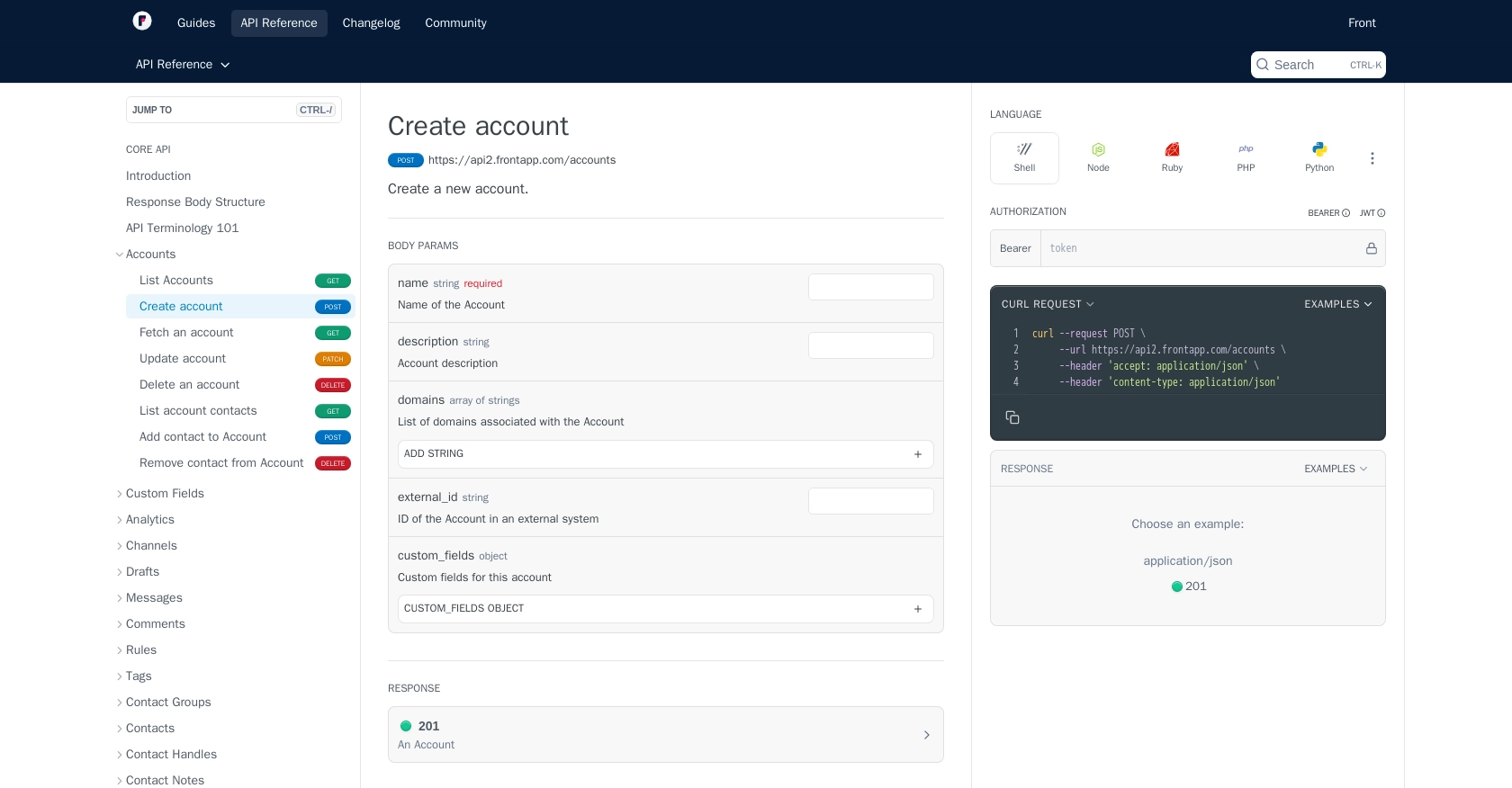
Conclusion and Best Practices for Using Front API with PHP
Integrating with the Front API to manage accounts can significantly enhance your application's ability to automate and streamline customer interactions. By following the steps outlined in this guide, you can efficiently create and update accounts using PHP, ensuring that your data remains consistent across platforms.
Best Practices for Secure and Efficient API Integration
- Securely Store API Tokens: Always store your API tokens securely and avoid hardcoding them in your application. Consider using environment variables or secure vaults.
- Handle Rate Limits: Front's API enforces rate limits to ensure platform stability. Monitor the
X-RateLimit-Remaining
header in API responses and implement retry logic with exponential backoff if you encounter a429 Too Many Requests
error. For more details, refer to the Front API Rate Limiting Documentation. - Implement Error Handling: Gracefully handle errors by checking response status codes and implementing appropriate fallback mechanisms. This ensures a robust integration that can handle unexpected issues.
- Data Transformation and Standardization: Ensure that data fields are transformed and standardized before making API calls to maintain data integrity across systems.
Enhance Your Integration Strategy with Endgrate
While integrating with Front API can be straightforward, managing multiple integrations can become complex. Endgrate offers a unified API solution that simplifies integration management, allowing you to focus on your core product development. By leveraging Endgrate, you can:
- Save time and resources by outsourcing integration complexities.
- Build once for each use case, reducing redundancy across different integrations.
- Provide an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration processes by visiting Endgrate.
Read More
- https://endgrate.com/provider/front
- https://dev.frontapp.com/docs/welcome
- https://dev.frontapp.com/docs/core-api-getting-started
- https://dev.frontapp.com/docs/oauth
- https://dev.frontapp.com/docs/rate-limiting
- https://dev.frontapp.com/docs/pagination
- https://dev.frontapp.com/reference/create-account
- https://dev.frontapp.com/reference/update-account
Ready to get started?