Using the Google Ads API to Get Leads in Python
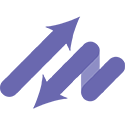
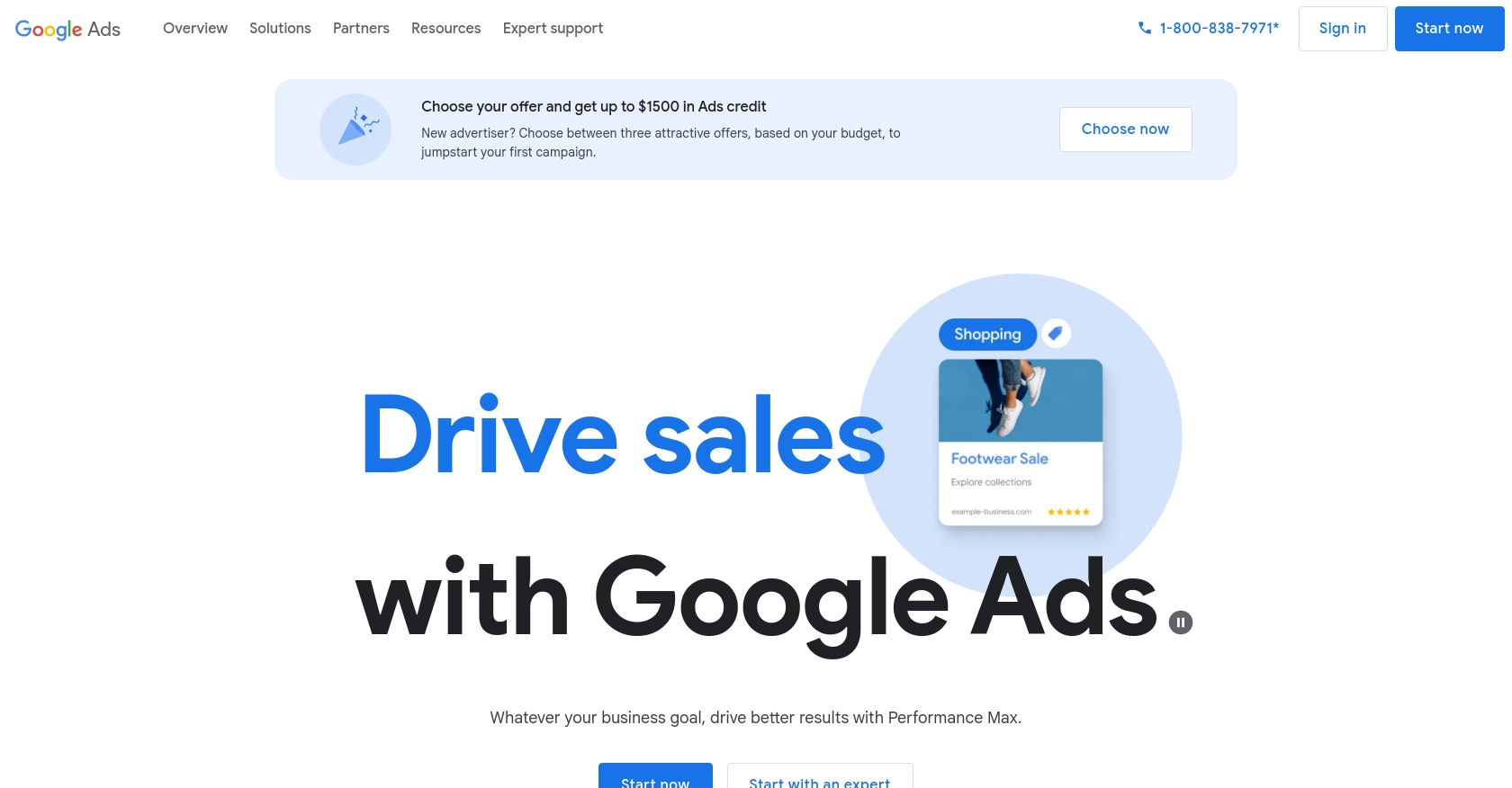
Introduction to Google Ads API for Lead Generation
The Google Ads API is a powerful tool that enables developers to manage large or complex Google Ads accounts and campaigns programmatically. It offers the flexibility to automate account management, create custom reports, and manage smart bidding strategies, making it an essential resource for businesses looking to optimize their advertising efforts.
Integrating with the Google Ads API allows developers to access and manage lead data efficiently. For example, you can retrieve lead form submissions directly into your CRM system, enabling real-time lead management and enhancing your marketing strategies.
In this article, we will explore how to use Python to interact with the Google Ads API, specifically focusing on retrieving leads. This integration can streamline your lead management process, ensuring that you capture and respond to potential customers promptly.
Setting Up Your Google Ads Sandbox Account for API Integration
Before diving into the Google Ads API, it's crucial to set up a sandbox account to test and experiment with API calls without affecting live data. This section will guide you through the process of creating a sandbox account and obtaining the necessary credentials for OAuth-based authentication.
Create a Google Ads Manager Account
To begin, you'll need a Google Ads manager account. This account acts as a central hub for managing multiple Google Ads accounts, making it ideal for testing and development purposes.
- Visit the Google Ads Manager Accounts page.
- Sign up for a manager account if you don't already have one.
- Follow the on-screen instructions to complete the setup.
Obtain a Developer Token
A developer token is required to access the Google Ads API. This token is linked to your manager account and controls the number of API calls you can make.
- Log in to your Google Ads manager account.
- Navigate to the Tools & Settings menu and select API Center.
- Apply for a developer token by following the instructions provided.
Set Up a Google API Console Project
Next, you'll need to create a project in the Google API Console to generate OAuth 2.0 credentials.
- Go to the Google API Console.
- Create a new project and name it appropriately.
- Enable the Google Ads API for your project by navigating to the Library and searching for "Google Ads API".
Generate OAuth 2.0 Credentials
OAuth 2.0 credentials are necessary for authorizing API requests. Follow these steps to generate them:
- In the Google API Console, navigate to Credentials.
- Click on Create Credentials and select OAuth client ID.
- Configure the consent screen by providing the necessary information.
- Select Web application as the application type and configure the authorized redirect URIs.
- Once created, note down the client ID and client secret for use in your API calls.
Prepare Your Google Ads Client Account
Finally, ensure you have a Google Ads client account to make API calls against. You'll need the 10-digit account number, which can be found in the Google Ads interface.
- Log in to your Google Ads account.
- Locate your account number in the format 123-456-7890 and remove the hyphens for API usage.
With these steps completed, you are now ready to authenticate and interact with the Google Ads API using Python. For more detailed information, refer to the Google Ads API documentation.
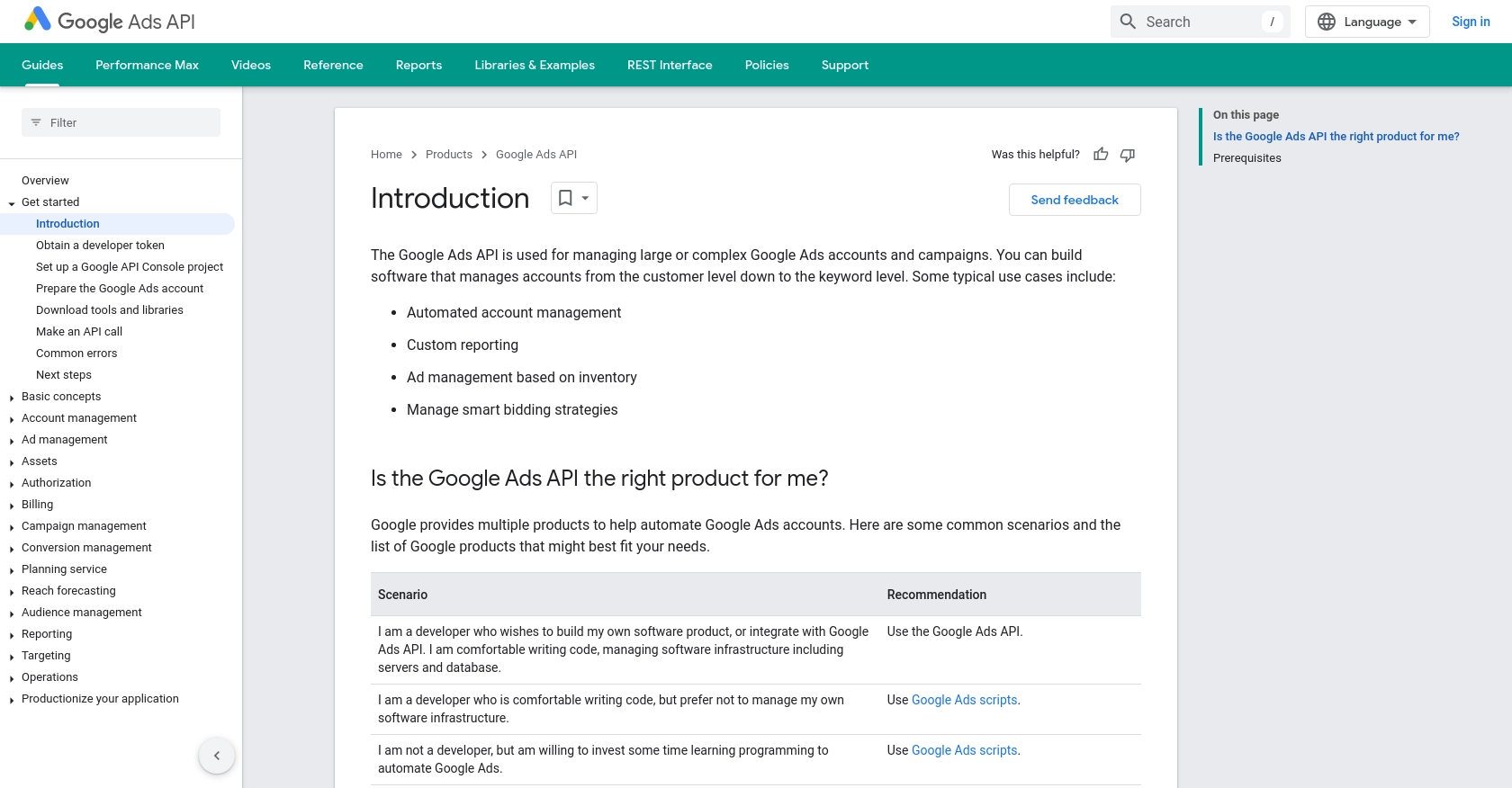
sbb-itb-96038d7
Making API Calls to Retrieve Leads from Google Ads Using Python
In this section, we will guide you through the process of making API calls to retrieve leads from Google Ads using Python. This involves setting up your Python environment, writing the necessary code to interact with the Google Ads API, and handling potential errors.
Setting Up Your Python Environment for Google Ads API
Before you start coding, ensure that you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer
pip
Once you have these installed, open your terminal or command prompt and install the Google Ads API client library using the following command:
pip install google-ads
Writing Python Code to Retrieve Leads from Google Ads
Now that your environment is set up, let's write the Python code to retrieve leads from Google Ads. Create a file named get_google_ads_leads.py
and add the following code:
import google.ads.google_ads.client
# Initialize the Google Ads client
client = google.ads.google_ads.client.GoogleAdsClient.load_from_storage()
# Define the query to retrieve lead form submissions
query = """
SELECT
lead_form_submission_data.resource_name,
lead_form_submission_data.id,
lead_form_submission_data.asset,
lead_form_submission_data.campaign,
lead_form_submission_data.submission_date_time
FROM
lead_form_submission_data
WHERE
lead_form_submission_data.submission_date_time DURING LAST_30_DAYS
"""
# Set the customer ID
customer_id = "INSERT_CUSTOMER_ID"
# Execute the query
response = client.service.google_ads.search(customer_id=customer_id, query=query)
# Process and print the results
for row in response:
lead = row.lead_form_submission_data
print(f"Lead ID: {lead.id}, Campaign: {lead.campaign}, Submitted At: {lead.submission_date_time}")
Replace INSERT_CUSTOMER_ID
with your actual Google Ads customer ID. This script initializes the Google Ads client, defines a query to retrieve lead form submissions from the last 30 days, and executes the query to fetch the leads.
Running the Python Script and Verifying Results
Run the script from your terminal or command prompt using the following command:
python get_google_ads_leads.py
You should see the lead details printed in the console. Verify the results by checking the lead form submissions in your Google Ads account.
Handling Errors and Troubleshooting Google Ads API Calls
When making API calls, you may encounter errors. Here are some common error codes and their meanings:
- AuthenticationError: Check your OAuth credentials and ensure they are correct.
- AuthorizationError: Verify that your developer token and account permissions are properly set.
- QuotaError: Ensure you are within your API call limits.
For more detailed error handling, refer to the Google Ads API error documentation.
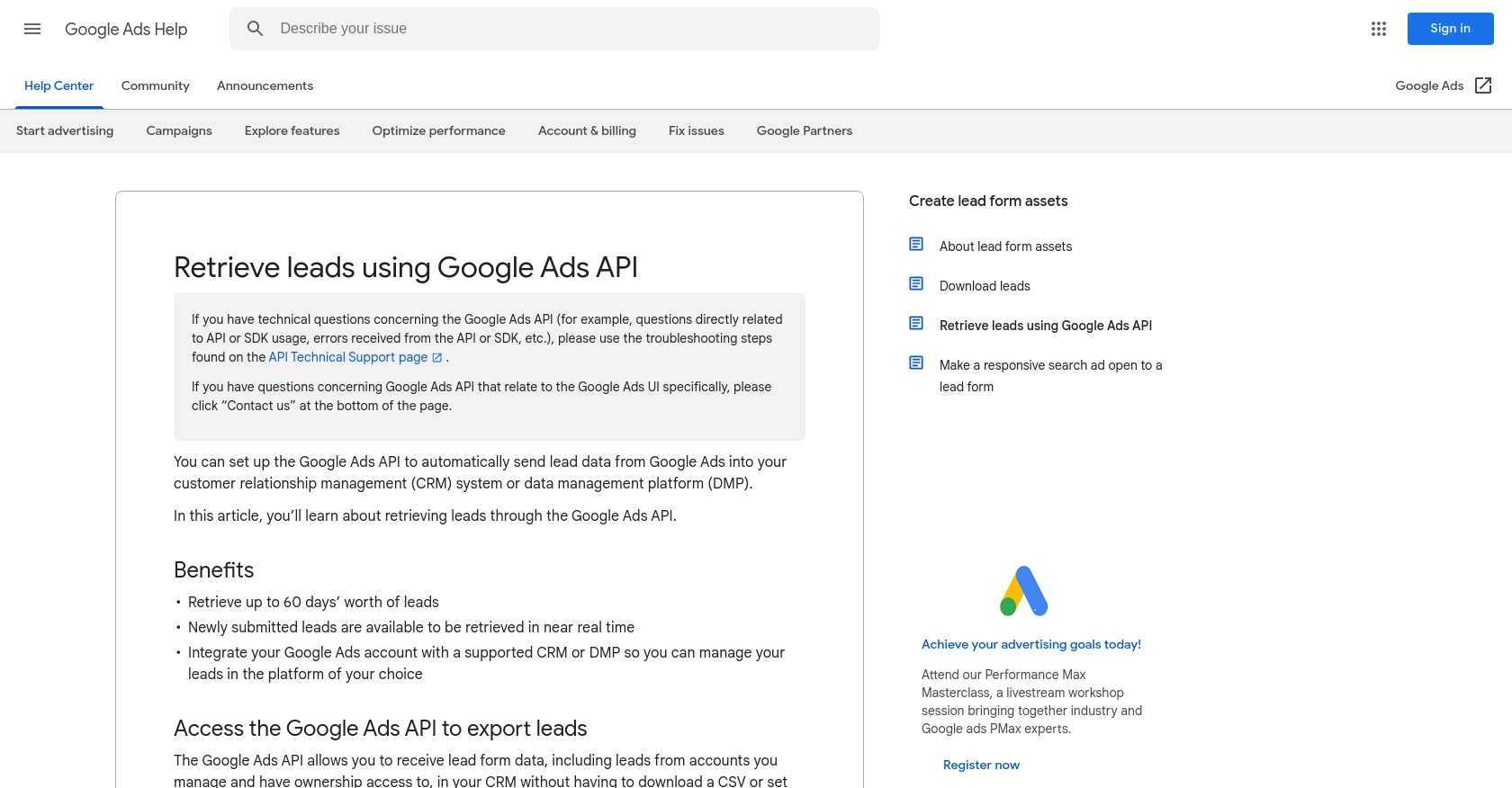
Conclusion and Best Practices for Using Google Ads API in Python
Integrating with the Google Ads API using Python offers a powerful way to manage and optimize your advertising efforts by automating lead retrieval and enhancing your marketing strategies. By following the steps outlined in this guide, you can efficiently access and manage lead data, ensuring timely responses to potential customers.
Best Practices for Google Ads API Integration
- Securely Store Credentials: Always store your OAuth credentials and developer tokens securely. Consider using environment variables or secure vaults to manage sensitive information.
- Handle Rate Limits: Be mindful of the API rate limits to avoid disruptions. Implement retry logic and exponential backoff strategies to handle quota errors gracefully.
- Standardize Data: Ensure that the lead data retrieved from Google Ads is standardized and transformed as needed for seamless integration with your CRM or data management systems.
- Monitor API Usage: Regularly monitor your API usage and performance to identify any potential issues or areas for optimization.
Streamline Your Integrations with Endgrate
While integrating with the Google Ads API can significantly enhance your lead management process, managing multiple integrations can be complex and time-consuming. Endgrate simplifies this by providing a unified API endpoint that connects to various platforms, including Google Ads.
With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers. Learn more about how Endgrate can streamline your integration processes by visiting Endgrate.
Read More
Ready to get started?