How to Create or Update Invoices with the Xero API in Python
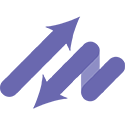
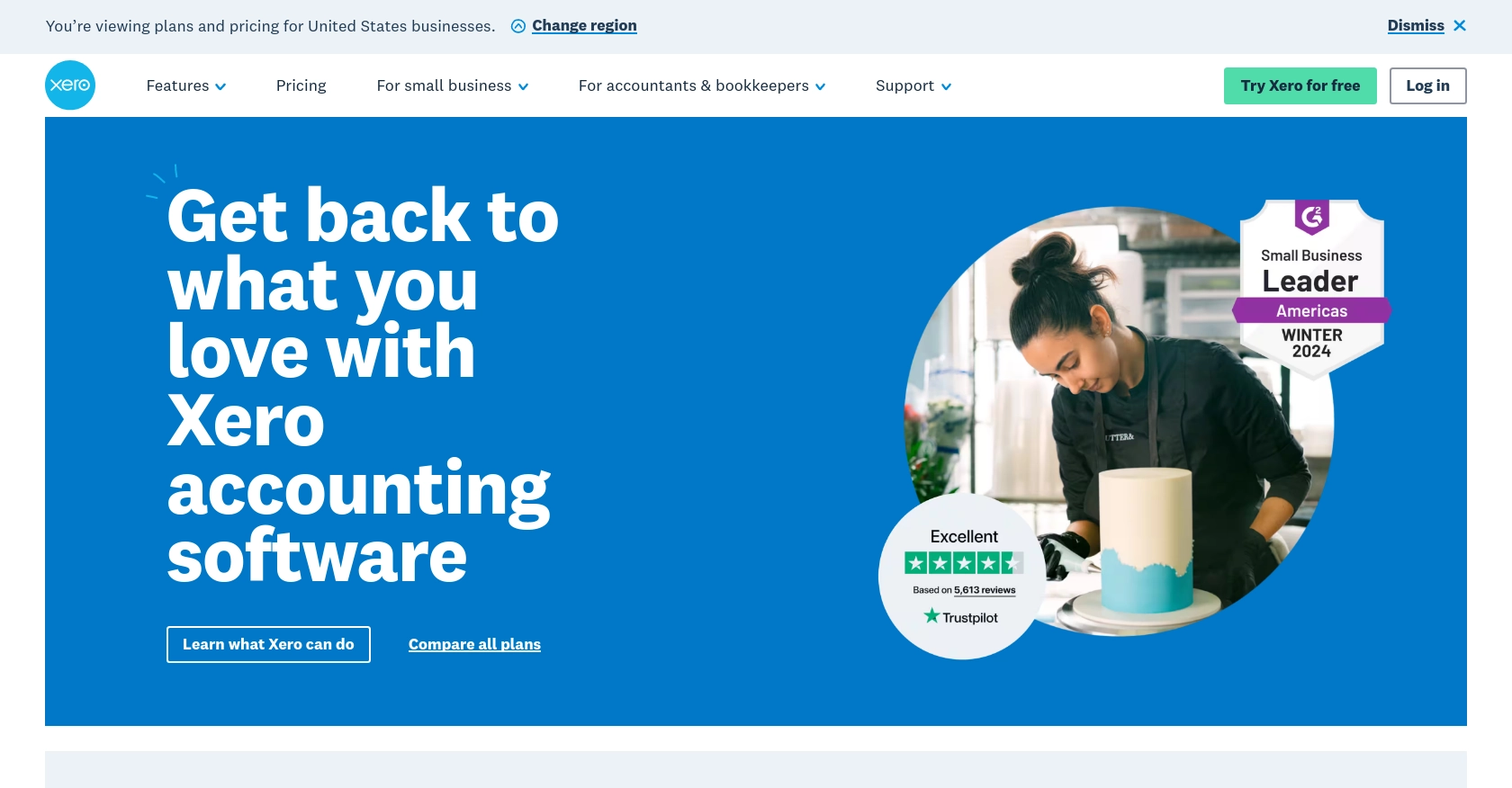
Introduction to Xero API for Invoice Management
Xero is a powerful cloud-based accounting software platform designed to help small and medium-sized businesses manage their finances efficiently. With features like invoicing, payroll, and expense tracking, Xero provides a comprehensive solution for businesses looking to streamline their financial operations.
Integrating with Xero's API allows developers to automate and enhance financial processes, such as creating or updating invoices. For example, a developer might use the Xero API to automatically generate invoices from a sales platform, ensuring that billing is accurate and up-to-date without manual intervention.
Setting Up a Xero Test or Sandbox Account for API Integration
Before diving into creating or updating invoices with the Xero API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data, making it an essential step for developers looking to integrate with Xero.
Creating a Xero Developer Account
To begin, you'll need a Xero developer account. Follow these steps to create one:
- Visit the Xero Developer Portal and sign up for a free account.
- Once registered, log in to your developer account to access the dashboard.
Setting Up a Xero Sandbox Organization
Next, create a sandbox organization to test your API interactions:
- In the Xero Developer Portal, navigate to the "My Apps" section.
- Select "Add a new app" and fill in the required details, such as app name and company URL.
- Choose the "Web app" option for the app type, as this supports OAuth 2.0 authentication.
- Once your app is created, you'll receive a client ID and client secret, which are crucial for authentication.
Configuring OAuth 2.0 Authentication
Xero uses OAuth 2.0 for secure API access. Here's how to set it up:
- In your app settings, specify the redirect URI, which is the URL Xero will redirect to after authentication.
- Ensure that your app has the necessary scopes for invoice management. You can configure these in the "Scopes" section of your app settings.
- Use the client ID and client secret to initiate the OAuth 2.0 flow, obtaining an access token for API requests.
For detailed guidance on OAuth 2.0, refer to the Xero OAuth 2.0 documentation.
Generating API Keys and Access Tokens
With OAuth 2.0 configured, you can generate access tokens:
- Use the client ID and client secret to request an authorization code from Xero.
- Exchange the authorization code for an access token, which will allow you to make API calls.
- Access tokens are temporary, so ensure you handle token refreshes as needed.
With your sandbox account and OAuth 2.0 authentication set up, you're ready to start integrating with the Xero API to manage invoices programmatically.
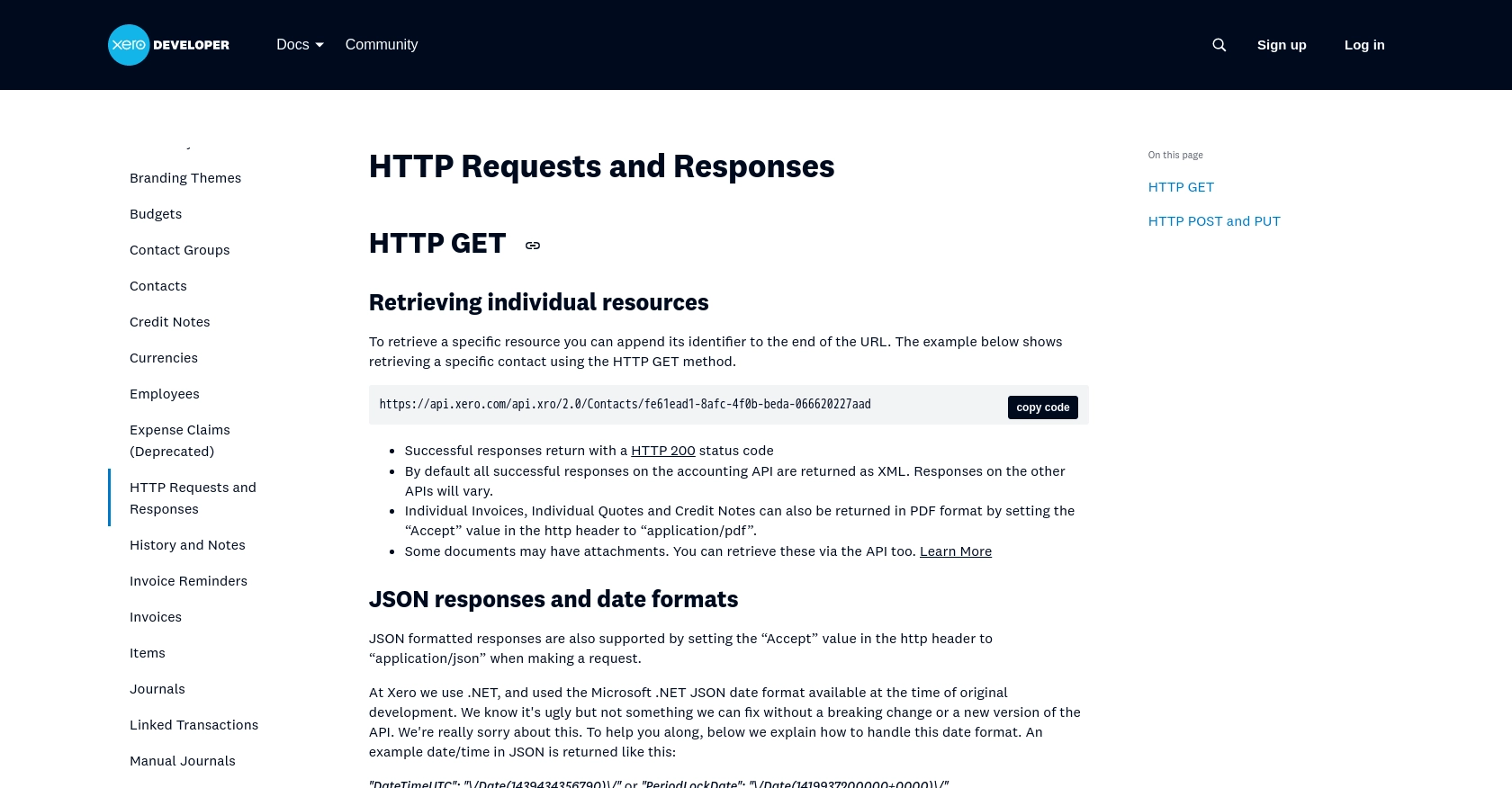
sbb-itb-96038d7
Making API Calls to Create or Update Invoices with Xero API in Python
To interact with the Xero API for creating or updating invoices, you'll need to use Python, a versatile programming language known for its simplicity and readability. This section will guide you through the necessary steps to set up your environment, make API calls, and handle responses effectively.
Setting Up Your Python Environment for Xero API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1
- The Python package installer, pip
Once these are installed, open your terminal or command prompt and install the requests
library, which will be used to make HTTP requests:
pip install requests
Creating or Updating Invoices Using Xero API
Now that your environment is set up, you can proceed to create or update invoices using the Xero API. Below is a sample code snippet to help you get started:
import requests
import json
# Set the API endpoint for creating or updating invoices
url = "https://api.xero.com/api.xro/2.0/Invoices"
# Set the request headers, including the authorization token
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Define the invoice data
invoice_data = {
"Type": "ACCREC",
"Contact": {
"Name": "John Doe"
},
"LineItems": [
{
"Description": "Consulting services",
"Quantity": 1.0,
"UnitAmount": 100.0,
"AccountCode": "200"
}
],
"Date": "2023-10-01",
"DueDate": "2023-10-15",
"Status": "AUTHORISED"
}
# Make a POST request to create or update the invoice
response = requests.post(url, headers=headers, data=json.dumps(invoice_data))
# Check the response status
if response.status_code == 200:
print("Invoice created or updated successfully.")
print(response.json())
else:
print("Failed to create or update invoice.")
print(f"Error: {response.status_code} - {response.text}")
Replace Your_Access_Token
with the access token obtained during the OAuth 2.0 authentication process.
Verifying API Call Success and Handling Errors
After running the code, you should verify the success of your API call by checking the response. A successful request will return a status code of 200, and you should see the invoice details in the response JSON.
If the request fails, the response will include an error code and message. Common error codes include:
- 400 Bad Request: The request was invalid. Check your data formatting.
- 401 Unauthorized: Authentication failed. Verify your access token.
- 403 Forbidden: You do not have permission to perform this action.
For more detailed error handling, refer to the Xero API documentation.
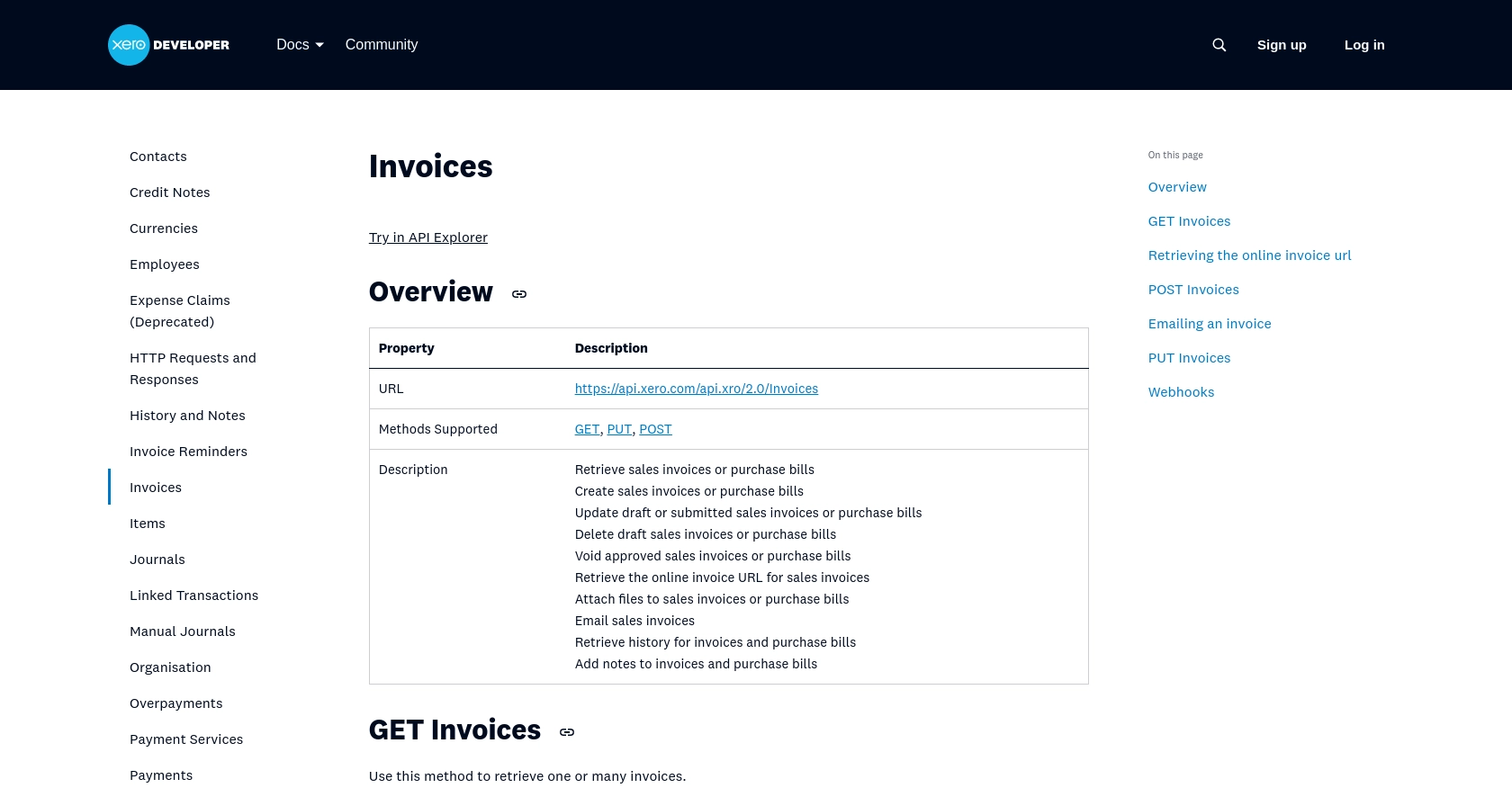
Conclusion and Best Practices for Xero API Integration in Python
Integrating with the Xero API to create or update invoices using Python can significantly streamline your financial processes, allowing for automation and improved accuracy. By following the steps outlined in this guide, you can efficiently manage invoices and ensure seamless integration with Xero's robust accounting platform.
Best Practices for Secure and Efficient Xero API Usage
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handle Rate Limiting: Xero's API has rate limits to ensure fair usage. Be mindful of these limits and implement retry logic to handle rate limit errors gracefully. For more details, refer to the Xero API rate limits documentation.
- Data Transformation and Standardization: Ensure that the data you send to Xero is correctly formatted and standardized. This will help prevent errors and ensure consistency across your financial records.
- Regularly Refresh Access Tokens: Access tokens are temporary. Implement a mechanism to refresh tokens before they expire to maintain uninterrupted access to the API.
Leverage Endgrate for Simplified Integration Management
While integrating with Xero's API can be powerful, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies integration management, allowing you to focus on your core product development. By using Endgrate, you can build once for each use case and enjoy an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover how you can save time and resources while delivering seamless integrations.
Read More
- https://endgrate.com/provider/xero
- https://developer.xero.com/documentation/api/accounting/requests-and-responses
- https://developer.xero.com/documentation/guides/oauth2/limits/
- https://developer.xero.com/documentation/guides/oauth2/overview
- https://developer.xero.com/documentation/guides/oauth2/scopes
- https://developer.xero.com/documentation/guides/oauth2/auth-flow
- https://developer.xero.com/documentation/guides/oauth2/tenants
- https://developer.xero.com/documentation/api/accounting/invoices
Ready to get started?