How to Get Tickets with the Zendesk Support API in PHP
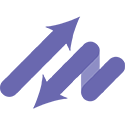
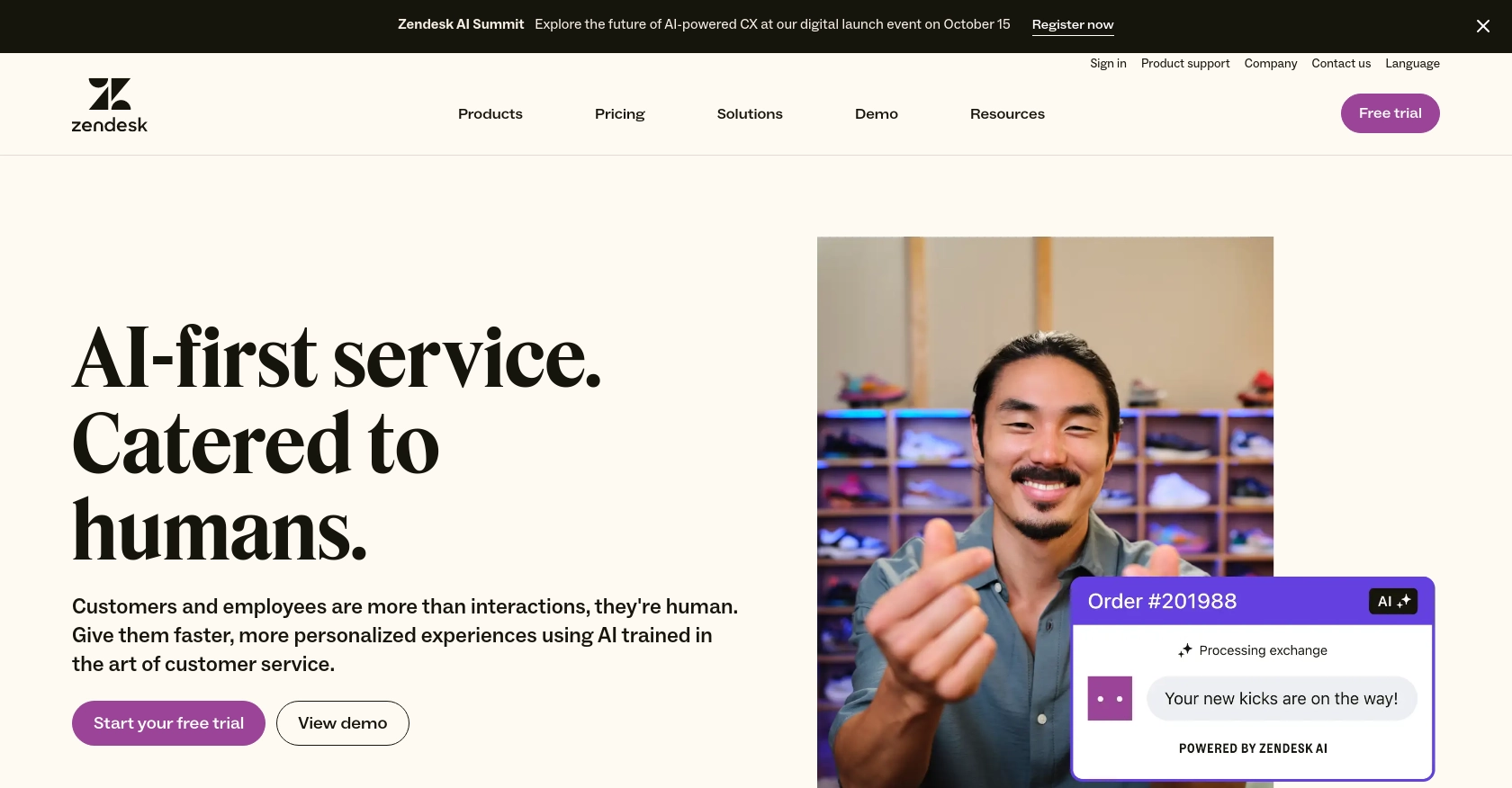
Introduction to Zendesk Support
Zendesk Support is a powerful customer service platform that enables businesses to manage and streamline their customer interactions across multiple channels. It offers a comprehensive suite of tools for ticketing, reporting, and customer engagement, making it a popular choice for companies looking to enhance their support operations.
Developers often integrate with the Zendesk Support API to automate and optimize customer service processes. For example, by using the API, developers can retrieve and manage support tickets programmatically, allowing for seamless integration with other business systems and enhancing the efficiency of customer support teams.
In this article, we will explore how to interact with the Zendesk Support API using PHP to retrieve tickets. This integration can be particularly useful for developers looking to build custom reporting tools or automate ticket management workflows.
Setting Up Your Zendesk Support Test or Sandbox Account
Before diving into the integration with the Zendesk Support API using PHP, it's essential to set up a test or sandbox account. This environment allows developers to experiment and test API interactions without affecting live data.
Creating a Zendesk Support Sandbox Account
If you don't already have a Zendesk account, you can sign up for a free trial or a sandbox account. Follow these steps to get started:
- Visit the Zendesk registration page and fill out the required information to create a new account.
- Once registered, log in to your Zendesk account.
- Navigate to the Admin Center and select Sandbox under the Manage section to create a sandbox environment.
Setting Up OAuth Authentication for Zendesk Support API
Zendesk Support API uses OAuth 2.0 for authentication, providing a secure way to access data without storing user passwords. Follow these steps to set up OAuth authentication:
- In the Zendesk Admin Center, go to Apps and integrations and select APIs.
- Click on the OAuth Clients tab and then Add OAuth client.
- Fill in the required fields:
- Client Name: Enter a name for your application.
- Description: Optionally, provide a brief description.
- Redirect URLs: Enter the URL where Zendesk should redirect after authorization.
- Click Save to generate your Client ID and Client Secret. Make sure to store these credentials securely.
For more detailed instructions, refer to the Zendesk OAuth documentation.
Generating an Access Token
To interact with the Zendesk Support API, you'll need to generate an access token using the OAuth credentials:
- Direct users to the Zendesk authorization page using the following URL format:
https://{subdomain}.zendesk.com/oauth/authorizations/new?response_type=code&client_id={your_client_id}&redirect_uri={your_redirect_url}&scope=read
- After authorization, Zendesk will redirect to your specified URL with an authorization code.
- Exchange this code for an access token by making a POST request to:
https://{subdomain}.zendesk.com/oauth/tokens
Include the following parameters in your request body:
{ "grant_type": "authorization_code", "code": "{authorization_code}", "client_id": "{your_client_id}", "client_secret": "{your_client_secret}", "redirect_uri": "{your_redirect_url}" }
Once you have the access token, you can use it to authenticate API requests by including it in the Authorization header as follows:
Authorization: Bearer {access_token}
For more information on OAuth authentication, visit the Zendesk Developer Docs.
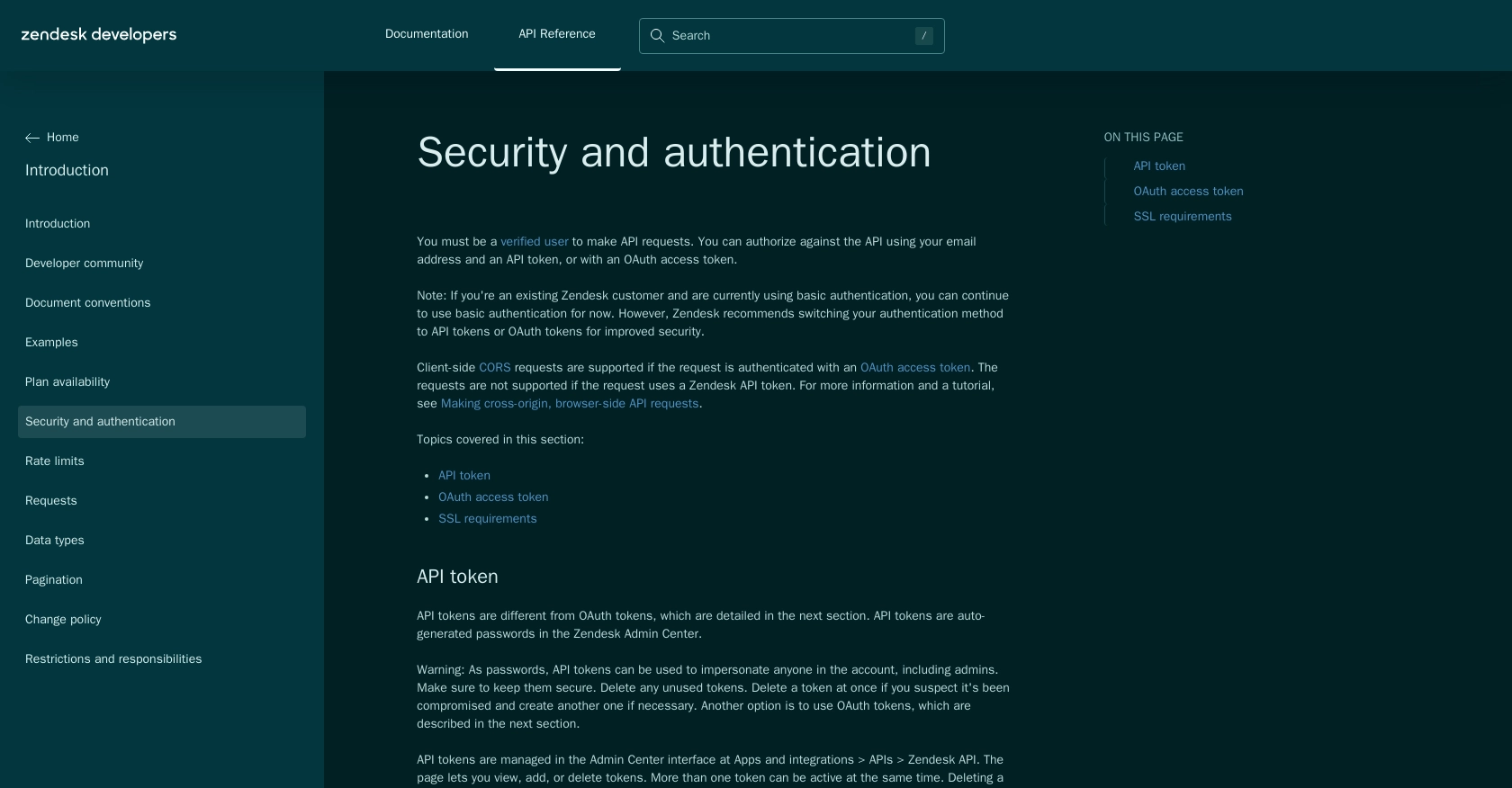
sbb-itb-96038d7
Making API Calls to Retrieve Zendesk Support Tickets Using PHP
To interact with the Zendesk Support API and retrieve tickets using PHP, you'll need to ensure your development environment is properly set up. This section will guide you through the necessary steps, including setting up PHP, installing required dependencies, and crafting the API request.
Setting Up PHP Environment for Zendesk Support API Integration
Before making API calls, verify that your PHP environment is correctly configured. Ensure you have the following:
- PHP 7.4 or higher installed on your machine.
- Composer, the PHP package manager, for managing dependencies.
To install Composer, follow the instructions on the Composer download page.
Installing Required PHP Dependencies
To make HTTP requests in PHP, you'll need the Guzzle HTTP client. Install it using Composer with the following command:
composer require guzzlehttp/guzzle
Crafting the Zendesk Support API Request in PHP
With your environment ready, you can now create a PHP script to retrieve tickets from Zendesk Support. Below is an example of how to make a GET request to the Zendesk Support API:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$subdomain = 'your_subdomain';
$accessToken = 'your_access_token';
$response = $client->request('GET', "https://{$subdomain}.zendesk.com/api/v2/tickets.json", [
'headers' => [
'Authorization' => "Bearer {$accessToken}",
'Content-Type' => 'application/json',
]
]);
if ($response->getStatusCode() === 200) {
$tickets = json_decode($response->getBody(), true);
foreach ($tickets['tickets'] as $ticket) {
echo "Ticket ID: " . $ticket['id'] . " - Subject: " . $ticket['subject'] . "\n";
}
} else {
echo "Failed to retrieve tickets. Status Code: " . $response->getStatusCode();
}
Replace your_subdomain
and your_access_token
with your actual Zendesk subdomain and access token.
Verifying Successful API Requests and Handling Errors
After running the script, you should see a list of ticket IDs and subjects printed in your terminal. If the request fails, ensure you handle errors gracefully by checking the status code and printing an appropriate message.
Common error codes include:
- 401 Unauthorized: Check your access token and ensure it is valid.
- 429 Too Many Requests: You have exceeded the rate limit. Refer to the Zendesk rate limits documentation for more information.
For further details on handling errors, consult the Zendesk Developer Docs.
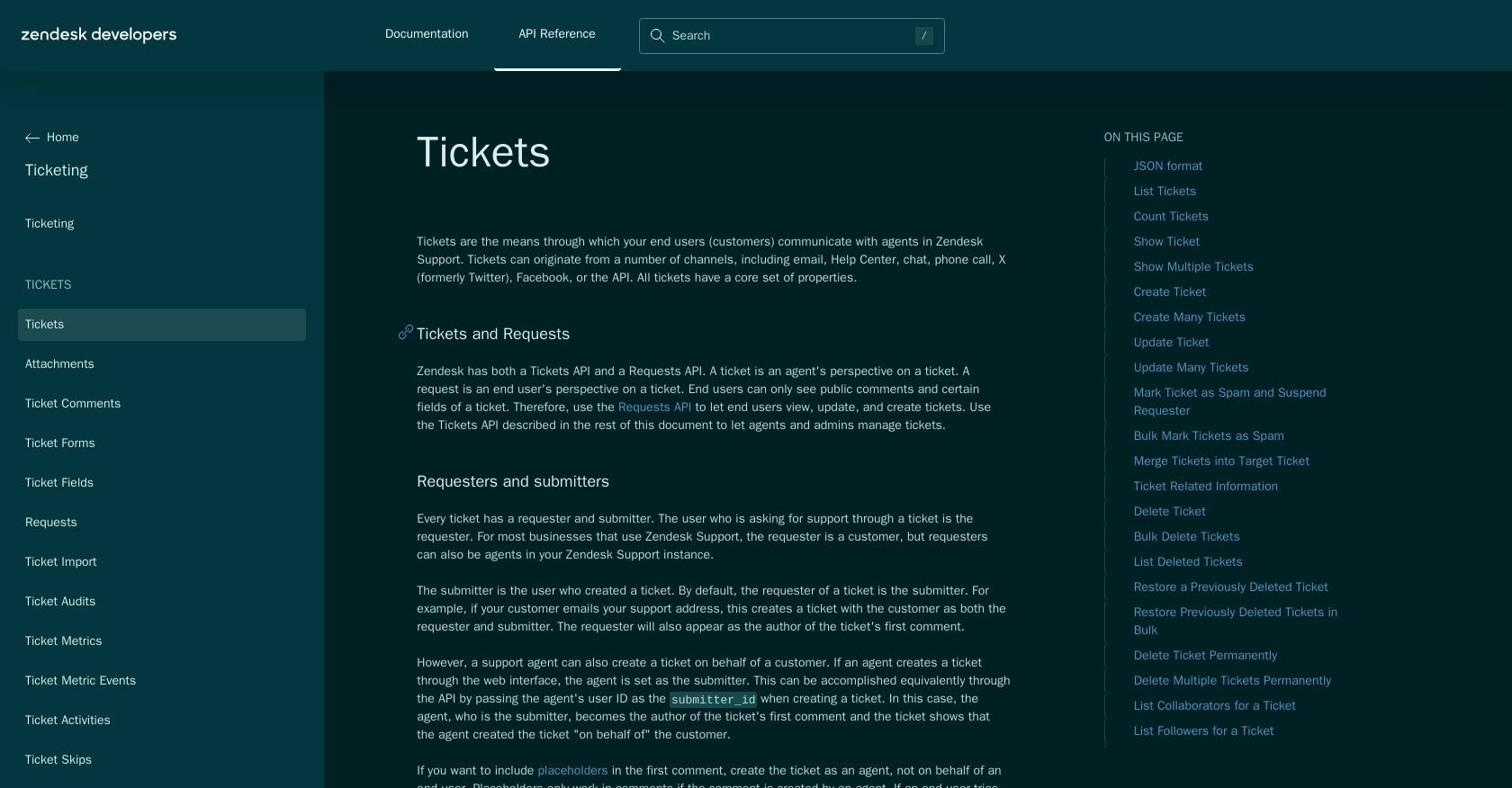
Best Practices for Zendesk Support API Integration
When integrating with the Zendesk Support API, it's crucial to follow best practices to ensure security, efficiency, and maintainability. Here are some recommendations:
- Secure Storage of Credentials: Always store your OAuth credentials, such as the Client ID and Client Secret, securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Zendesk imposes rate limits on API requests. Monitor the
X-Rate-Limit-Remaining
andRetry-After
headers in API responses to handle rate limiting gracefully. Implement exponential backoff strategies to retry requests when limits are exceeded. For more details, refer to the Zendesk rate limits documentation. - Data Transformation and Standardization: When retrieving data from Zendesk, ensure that you transform and standardize it according to your application's needs. This can involve mapping fields, converting data types, or normalizing values.
Enhancing Integration Efficiency with Endgrate
Building and maintaining integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including Zendesk Support. With Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the intricacies of integration.
- Build Once, Use Everywhere: Develop a single integration that works across various platforms, reducing redundancy and effort.
- Offer a Seamless Experience: Provide your customers with an intuitive and efficient integration experience.
Explore how Endgrate can streamline your integration processes by visiting Endgrate.
Conclusion
Integrating with the Zendesk Support API using PHP allows developers to automate and enhance customer support processes. By following best practices and leveraging tools like Endgrate, you can create robust and efficient integrations that improve your support operations. Start exploring the possibilities with Zendesk Support and take your customer service to the next level.
Read More
- https://endgrate.com/provider/zendesksupport
- https://developer.zendesk.com/api-reference/introduction/security-and-auth/
- https://support.zendesk.com/hc/en-us/articles/4408845965210-Using-OAuth-authentication-with-your-application
- https://developer.zendesk.com/api-reference/introduction/rate-limits/
- https://developer.zendesk.com/api-reference/introduction/pagination/
- https://developer.zendesk.com/api-reference/ticketing/tickets/tickets/
Ready to get started?