How to Get People with the Pipedrive API in Javascript
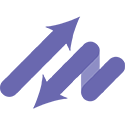
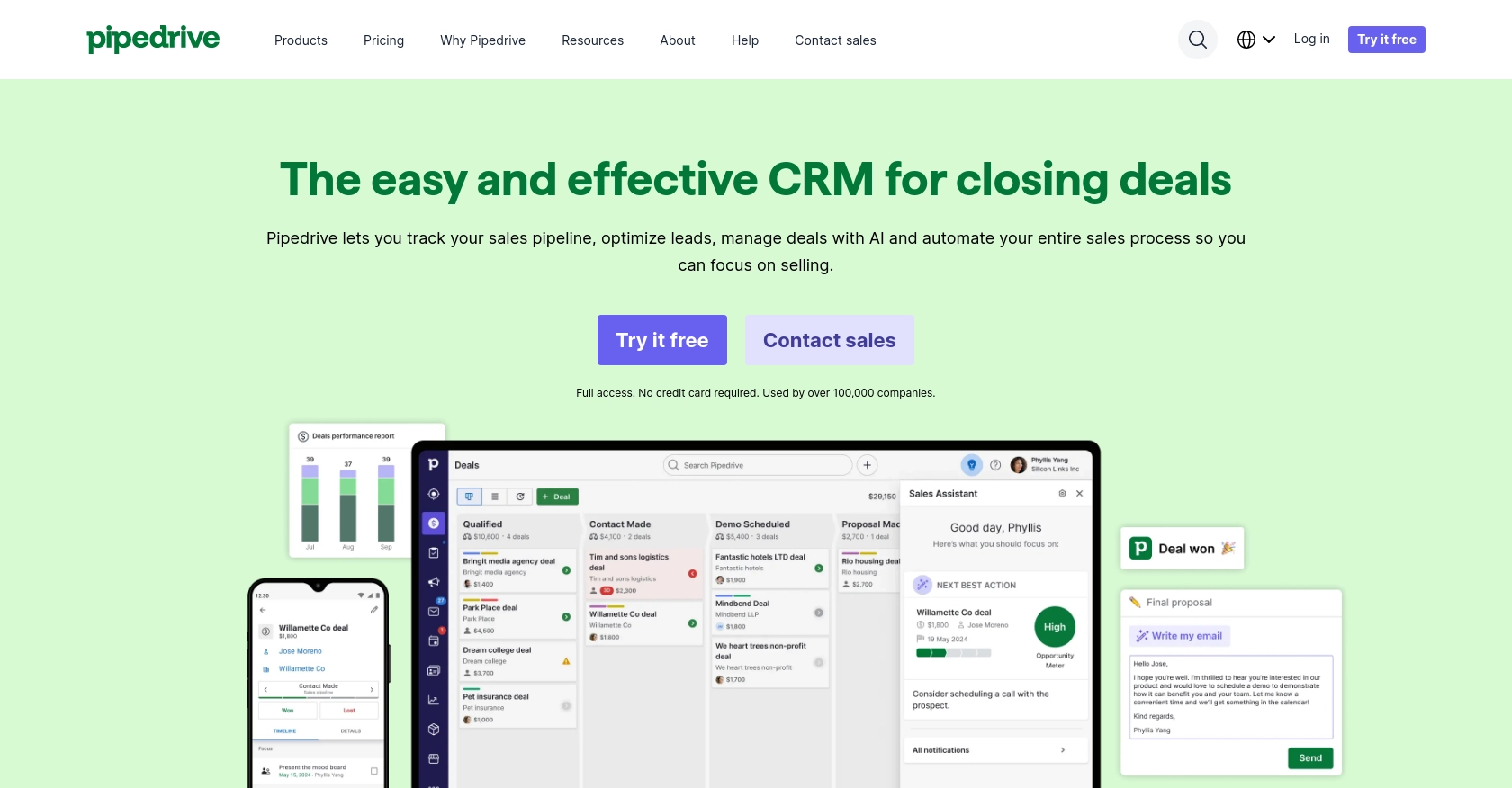
Introduction to Pipedrive CRM
Pipedrive is a powerful sales CRM platform designed to help businesses manage their sales processes more effectively. With its intuitive interface and robust features, Pipedrive enables sales teams to track leads, manage customer relationships, and close deals efficiently.
Developers often integrate with Pipedrive's API to automate and enhance sales workflows. For example, accessing the Pipedrive API allows developers to retrieve and manage contact information, enabling seamless synchronization of customer data across different platforms. This can be particularly useful for businesses looking to streamline their sales operations and improve customer engagement.
In this article, we will explore how to use JavaScript to interact with the Pipedrive API, specifically focusing on retrieving people (contacts) data. This integration can help developers build applications that leverage Pipedrive's CRM capabilities to enhance sales productivity.
Setting Up Your Pipedrive Developer Sandbox Account
Before diving into the Pipedrive API integration, it's essential to set up a developer sandbox account. This account provides a risk-free environment to test and develop your applications without affecting live data. Follow these steps to get started:
Create a Pipedrive Developer Sandbox Account
- Visit the Pipedrive Developer Sandbox page.
- Fill out the necessary form to request access to a sandbox account. This account will mimic a regular Pipedrive company account, allowing you to perform testing and development.
- Once your sandbox account is set up, you can import sample data to familiarize yourself with Pipedrive's interface. Navigate to “...” (More) > Import data > From a spreadsheet in the Pipedrive web app to import template spreadsheets.
Register Your App in Pipedrive Developer Hub
To interact with the Pipedrive API, you need to create an app in the Developer Hub. This process will provide you with the necessary credentials for OAuth 2.0 authentication:
- Log in to your Pipedrive sandbox account and access the Developer Hub.
- Register your app by providing the required details. This includes the app name, description, and redirect URL.
- After registration, you will receive a client ID and client secret. These credentials are crucial for implementing OAuth 2.0 authentication in your application.
Implement OAuth 2.0 Authentication
With your app registered, you can now set up OAuth 2.0 authentication to securely access Pipedrive's API:
- Use the client ID and client secret obtained from the Developer Hub to initiate the OAuth flow.
- Direct users to Pipedrive's authorization URL, where they can grant your app access to their data.
- Once authorized, Pipedrive will redirect users back to your specified redirect URL with an authorization code.
- Exchange this authorization code for an access token, which will be used to authenticate API requests.
By following these steps, you will have a fully functional sandbox environment and the necessary authentication setup to begin integrating with the Pipedrive API using JavaScript.
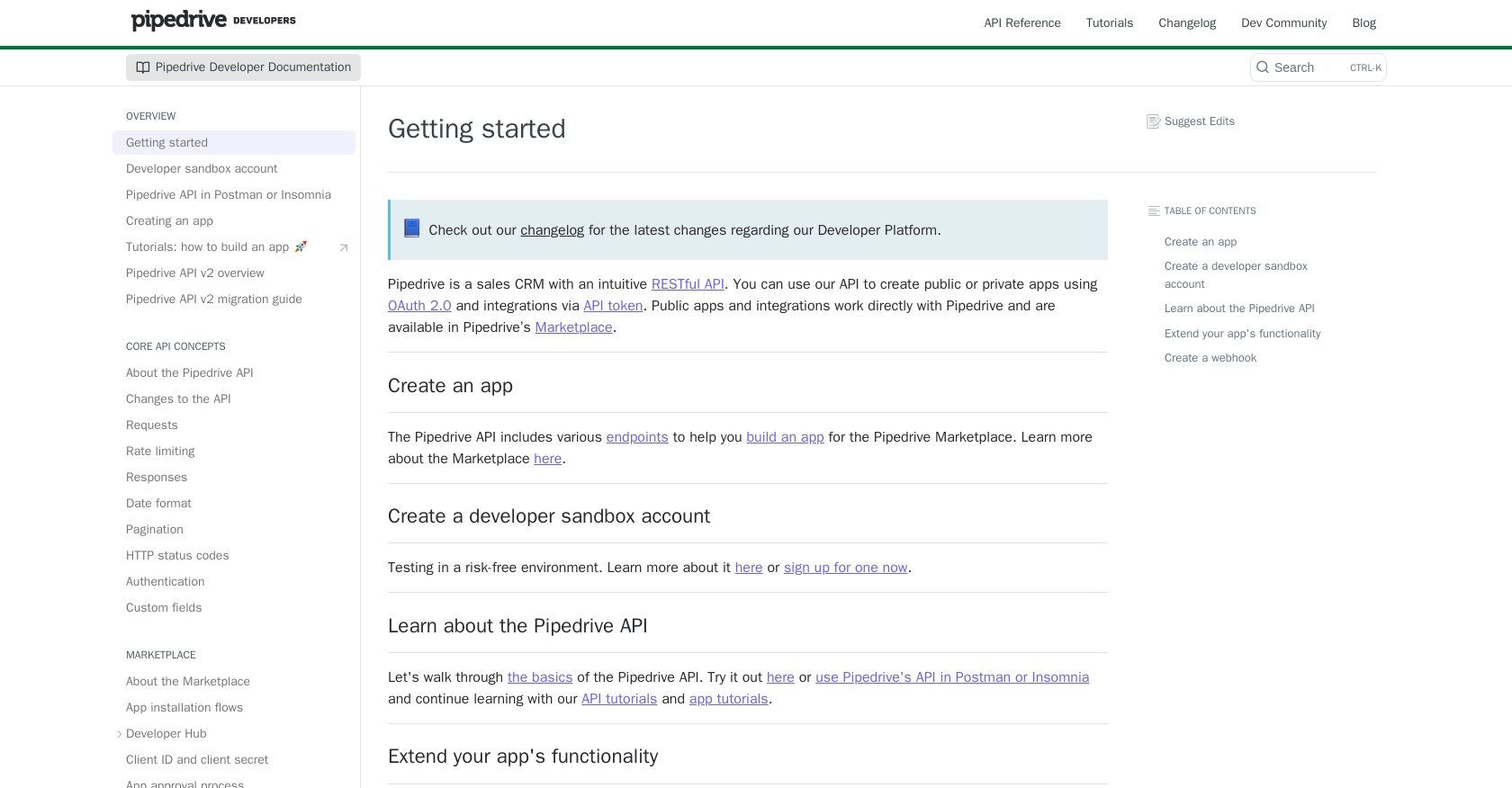
sbb-itb-96038d7
Making API Calls to Retrieve People Data from Pipedrive Using JavaScript
To interact with the Pipedrive API and retrieve people data, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling responses effectively.
Setting Up Your JavaScript Environment for Pipedrive API Integration
Before making API calls, ensure you have the necessary tools and libraries installed:
- Node.js: Ensure you have Node.js installed on your machine. You can download it from the official Node.js website.
- Axios: This is a popular HTTP client for making requests. Install it using npm:
npm install axios
Writing JavaScript Code to Fetch People Data from Pipedrive
With your environment set up, you can now write the JavaScript code to interact with the Pipedrive API. Create a file named getPipedrivePeople.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.pipedrive.com/v1/persons';
const accessToken = 'Your_Access_Token'; // Replace with your access token
const headers = {
'Authorization': `Bearer ${accessToken}`
};
// Function to get people data
async function getPeople() {
try {
const response = await axios.get(endpoint, { headers });
const people = response.data.data;
// Output the people data
people.forEach(person => {
console.log(`Name: ${person.name}, Email: ${person.email}`);
});
} catch (error) {
console.error('Error fetching people:', error.response ? error.response.data : error.message);
}
}
// Execute the function
getPeople();
Replace Your_Access_Token
with the access token obtained from the OAuth 2.0 authentication process.
Running Your JavaScript Code and Verifying Pipedrive API Responses
To execute the code and retrieve people data, run the following command in your terminal:
node getPipedrivePeople.js
If successful, the console will display the names and emails of the people retrieved from your Pipedrive account.
Handling Errors and Understanding Pipedrive API Response Codes
When making API calls, it's crucial to handle potential errors gracefully. The Pipedrive API may return various HTTP status codes, such as:
- 200 OK: The request was successful.
- 401 Unauthorized: Invalid access token. Ensure your token is correct.
- 429 Too Many Requests: Rate limit exceeded. Refer to the rate limiting documentation for more details.
For a complete list of status codes, visit the Pipedrive HTTP status codes documentation.
By following these steps, you can effectively retrieve and manage people data from Pipedrive using JavaScript, enhancing your application's capabilities and improving sales productivity.
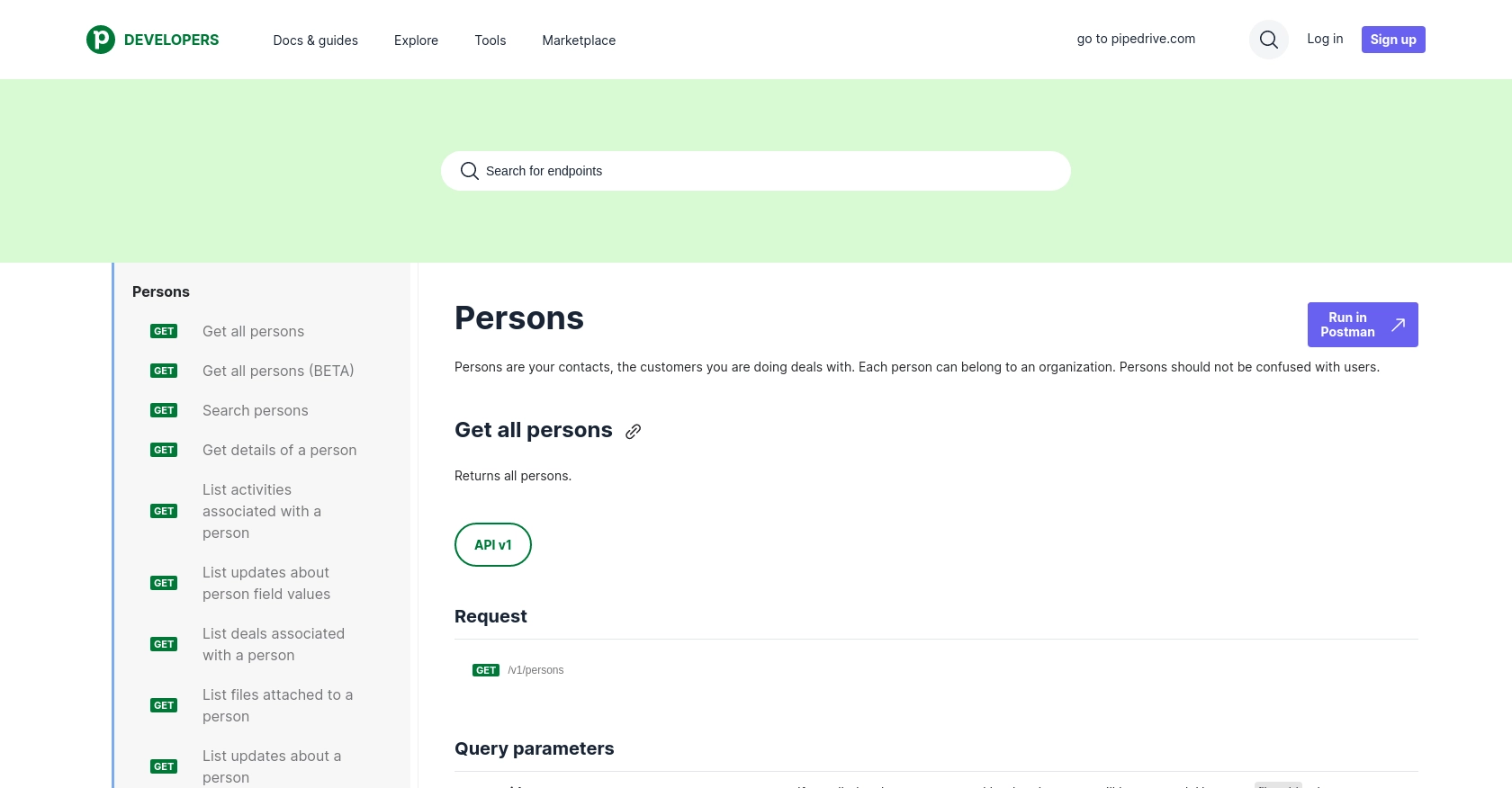
Conclusion and Best Practices for Integrating with Pipedrive API Using JavaScript
Integrating with the Pipedrive API using JavaScript offers a powerful way to enhance your sales processes by automating data retrieval and management. By following the steps outlined in this article, you can efficiently access and manipulate people data within Pipedrive, streamlining your sales operations and improving customer engagement.
Best Practices for Secure and Efficient Pipedrive API Integration
- Secure Storage of Credentials: Always store your OAuth tokens securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of Pipedrive's rate limits. Implement logic to handle HTTP 429 responses gracefully and consider using webhooks to reduce the need for frequent polling. For more details, refer to the rate limiting documentation.
- Data Transformation and Standardization: Ensure that the data retrieved from Pipedrive is transformed and standardized to fit your application's requirements, maintaining consistency across platforms.
Enhance Your Integration Strategy with Endgrate
While building integrations with Pipedrive can significantly boost your sales productivity, managing multiple integrations can become complex and time-consuming. This is where Endgrate can make a difference.
Endgrate offers a unified API solution that simplifies the integration process, allowing you to connect with multiple platforms, including Pipedrive, through a single API endpoint. By leveraging Endgrate, you can save time and resources, focusing on your core product while providing an intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration strategy by visiting Endgrate's website and discover the benefits of outsourcing your integration needs.
Read More
- https://endgrate.com/provider/pipedrive
- https://pipedrive.readme.io/docs/getting-started
- https://pipedrive.readme.io/docs/developer-sandbox-account
- https://pipedrive.readme.io/docs/marketplace-creating-a-proper-app
- https://pipedrive.readme.io/docs/core-api-concepts-rate-limiting
- https://pipedrive.readme.io/docs/core-api-concepts-pagination
- https://pipedrive.readme.io/docs/core-api-concepts-http-status-codes
- https://pipedrive.readme.io/docs/core-api-concepts-custom-fields
- https://developers.pipedrive.com/docs/api/v1/Persons
- https://developers.pipedrive.com/docs/api/v1/PersonFields
Ready to get started?