How to Get Companies with the PipelineCRM API in PHP
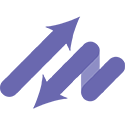
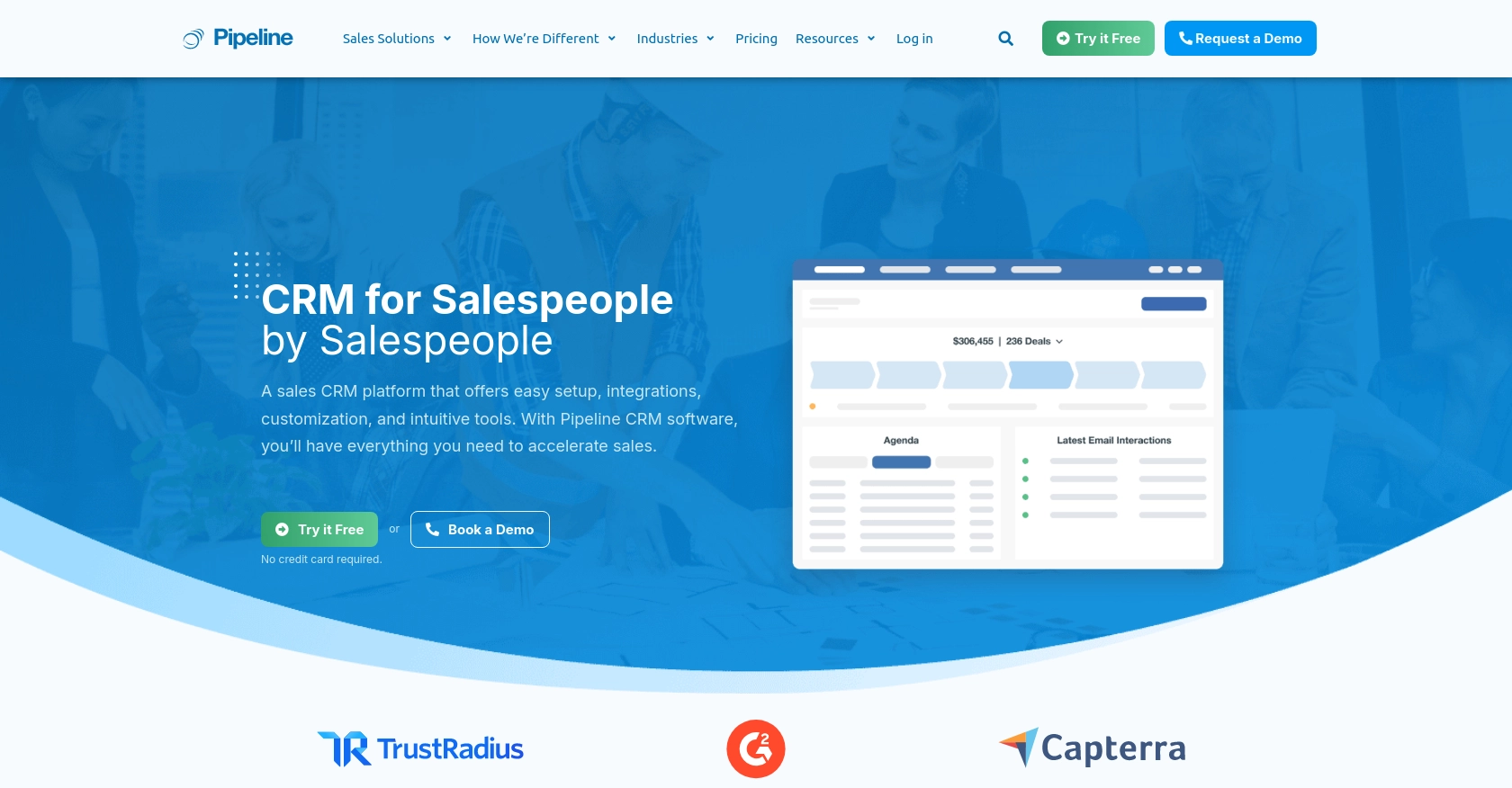
Introduction to PipelineCRM
PipelineCRM is a robust customer relationship management platform designed to help businesses manage their sales processes efficiently. With features such as contact management, sales tracking, and reporting, PipelineCRM offers a comprehensive solution for sales teams looking to streamline their operations.
Developers may want to integrate with PipelineCRM's API to access and manage company data, enabling seamless synchronization between their applications and the CRM platform. For example, a developer might use the PipelineCRM API to retrieve a list of companies, allowing for enhanced data analysis and reporting within their own software solutions.
Setting Up Your PipelineCRM Test Account
Before you can start using the PipelineCRM API to retrieve company data, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting live data.
Creating a PipelineCRM Account
If you don't already have a PipelineCRM account, you can sign up for a free trial on their website. This trial will give you access to the necessary features to test API interactions.
- Visit the PipelineCRM website and click on the "Sign Up" button.
- Fill out the registration form with your details and submit it to create your account.
- Once your account is created, log in to access the dashboard.
Generating Your API Key for Authentication
PipelineCRM uses API key-based authentication, which means you'll need to generate an API key to authorize your requests.
- Log in to your PipelineCRM account and navigate to the "Settings" section.
- Look for the "API Keys" option and click on it.
- Click on "Generate New API Key" and provide a name for your key to easily identify it later.
- Copy the generated API key and store it securely, as you'll need it for making API calls.
With your API key ready, you can now proceed to interact with the PipelineCRM API using PHP to retrieve company data.
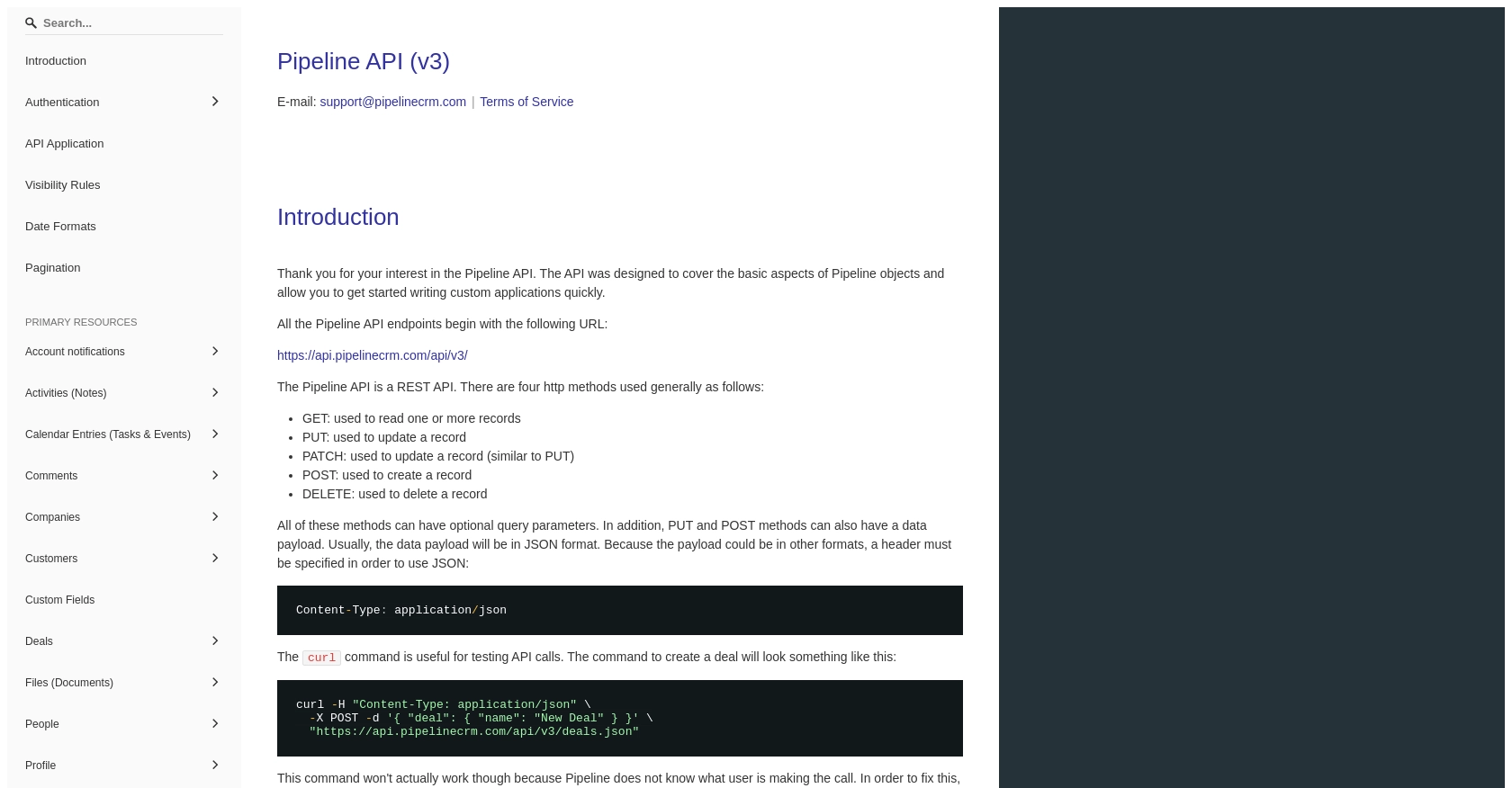
sbb-itb-96038d7
Making API Calls to Retrieve Companies with PipelineCRM in PHP
To interact with the PipelineCRM API and retrieve company data, you'll need to use PHP to make HTTP requests. This section will guide you through the process of setting up your PHP environment, writing the code to make the API call, and handling the response.
Setting Up Your PHP Environment for PipelineCRM API Integration
Before you start coding, ensure that you have PHP installed on your machine. You can download it from the official PHP website. Additionally, you'll need the cURL
extension enabled to make HTTP requests.
- Verify your PHP installation by running
php -v
in your terminal. - Ensure the
cURL
extension is enabled by checking yourphp.ini
file or runningphp -m | grep curl
.
Writing PHP Code to Make the PipelineCRM API Call
With your environment ready, you can now write the PHP code to make the API call to PipelineCRM. The following example demonstrates how to retrieve a list of companies using your API key for authentication.
<?php
// Set the API endpoint URL
$endpoint = "https://api.pipelinecrm.com/api/v3/companies";
// Your API key
$apiKey = "Your_API_Key";
// Initialize cURL session
$ch = curl_init();
// Set cURL options
curl_setopt($ch, CURLOPT_URL, $endpoint);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Authorization: Bearer $apiKey",
"Content-Type: application/json"
]);
// Execute the cURL request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Decode the JSON response
$data = json_decode($response, true);
// Output the company data
foreach ($data['companies'] as $company) {
echo "Company Name: " . $company['name'] . "\n";
}
}
// Close the cURL session
curl_close($ch);
?>
Replace Your_API_Key
with the API key you generated earlier. This script initializes a cURL session, sets the necessary headers for authentication, and makes a GET request to the PipelineCRM API endpoint to fetch company data.
Verifying the API Request and Handling Errors
After running the script, you should see a list of company names printed in your terminal. If the request is successful, the data returned will match the companies available in your PipelineCRM test account.
In case of errors, the script will output the error message. Common issues might include incorrect API keys or network problems. Ensure your API key is correct and that your network connection is stable.
For more information on error codes and handling, refer to the PipelineCRM API documentation.
Best Practices for Using the PipelineCRM API in PHP
When working with the PipelineCRM API, it's essential to follow best practices to ensure secure and efficient integration. Here are some recommendations:
- Securely Store API Keys: Always store your API keys securely. Avoid hardcoding them directly into your scripts. Instead, use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be mindful of any rate limits imposed by the PipelineCRM API. Implement logic to handle rate limit responses gracefully, such as retrying requests after a specified delay. Check the PipelineCRM API documentation for specific rate limit details.
- Implement Error Handling: Ensure your application can handle various error responses from the API. This includes checking for HTTP status codes and parsing error messages to provide meaningful feedback to users.
- Data Transformation and Standardization: When integrating data from PipelineCRM into your application, consider transforming and standardizing data fields to match your application's data model.
Streamlining Integrations with Endgrate
Integrating multiple platforms can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including PipelineCRM. By using Endgrate, you can:
- Save Time and Resources: Focus on your core product development while Endgrate handles the complexities of integration.
- Build Once, Use Everywhere: Develop a single integration for each use case, reducing the need for multiple implementations.
- Enhance User Experience: Offer your customers a seamless and intuitive integration experience with minimal effort.
Explore how Endgrate can help you streamline your integration processes by visiting Endgrate today.
Read More
Ready to get started?