Using the Zoho Books API to Create or Update Sales Orders in Python
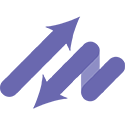
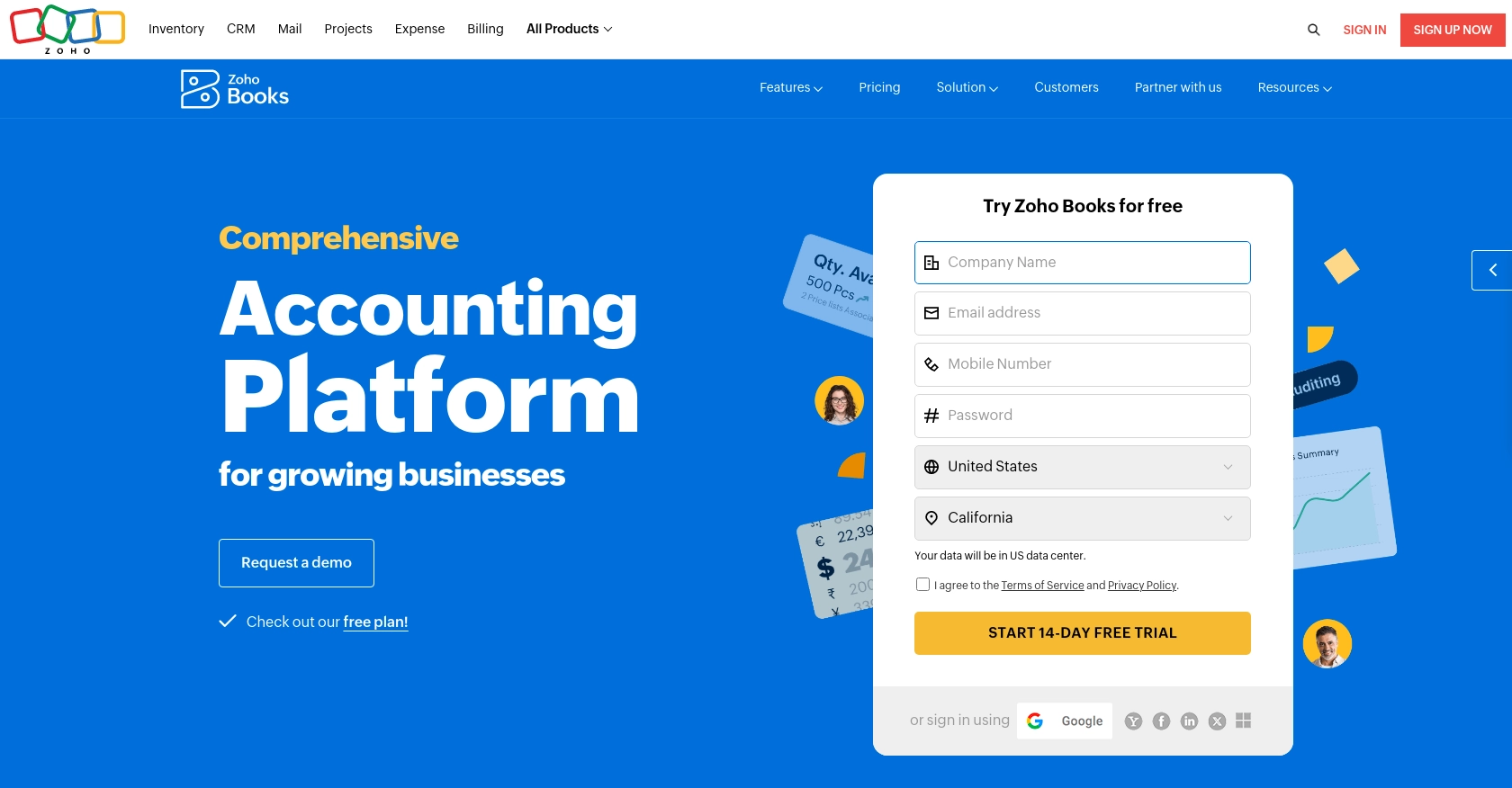
Introduction to Zoho Books API for Sales Order Management
Zoho Books is a comprehensive cloud-based accounting software designed to streamline financial operations for businesses of all sizes. It offers a suite of features including invoicing, expense tracking, and inventory management, making it a popular choice for businesses looking to automate their accounting processes.
Integrating with the Zoho Books API allows developers to efficiently manage sales orders, a crucial component in the sales process. By automating the creation and updating of sales orders, businesses can ensure accuracy and save time. For example, a developer might use the Zoho Books API to automatically generate sales orders from an e-commerce platform, ensuring that inventory levels are updated in real-time and reducing manual data entry errors.
Setting Up Your Zoho Books Test/Sandbox Account for API Integration
Before you can start creating or updating sales orders using the Zoho Books API, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data.
Step-by-Step Guide to Creating a Zoho Books Sandbox Account
-
Sign Up for Zoho Books:
Visit the Zoho Books website and sign up for a free trial or demo account. This will give you access to the features needed for testing API integrations.
-
Access the Developer Console:
Navigate to the Zoho Developer Console. Here, you can register your application to obtain the necessary OAuth credentials.
-
Register Your Application:
Click on "Add Client ID" and fill in the required details. Upon successful registration, you will receive a Client ID and Client Secret. Keep these credentials secure as they are essential for authenticating API requests.
Configuring OAuth Authentication for Zoho Books API
Zoho Books uses OAuth 2.0 for secure API access. Follow these steps to generate the necessary tokens:
-
Generate a Grant Token:
Redirect users to the following URL to obtain a grant token:
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.salesorders.CREATE,ZohoBooks.salesorders.UPDATE&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI&access_type=offline
Replace
YOUR_CLIENT_ID
andYOUR_REDIRECT_URI
with your actual client ID and redirect URI. -
Exchange Grant Token for Access and Refresh Tokens:
Make a POST request to the following URL to exchange the grant token for access and refresh tokens:
https://accounts.zoho.com/oauth/v2/token?code=GRANT_TOKEN&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code
Replace
GRANT_TOKEN
,YOUR_CLIENT_ID
,YOUR_CLIENT_SECRET
, andYOUR_REDIRECT_URI
with the appropriate values. -
Store Tokens Securely:
Ensure that the access and refresh tokens are stored securely in your application. The access token will expire after a set period, but the refresh token can be used to generate a new access token.
For more detailed information, refer to the Zoho Books OAuth documentation.
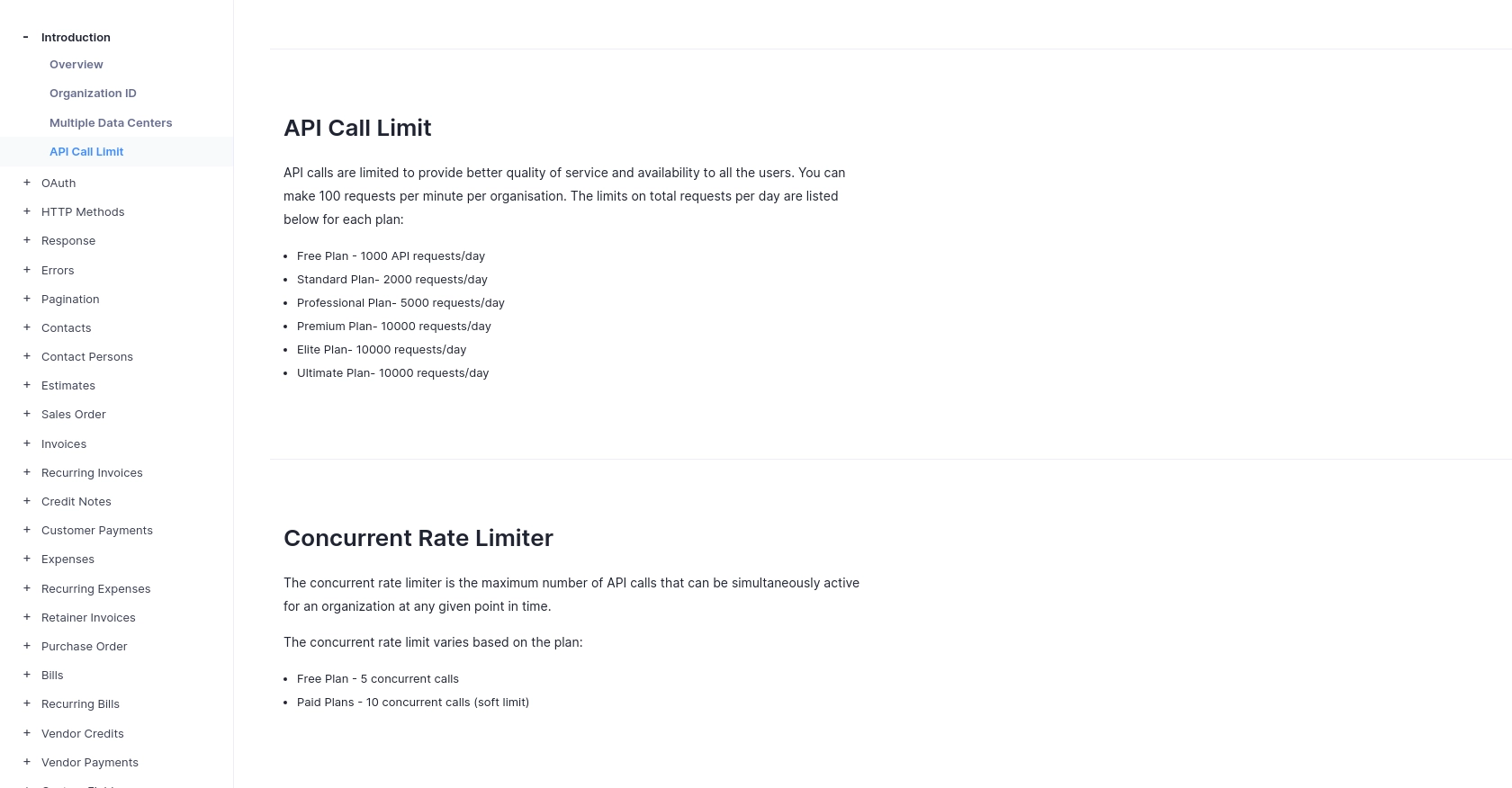
sbb-itb-96038d7
Making API Calls to Create or Update Sales Orders in Zoho Books Using Python
Once you have set up your Zoho Books account and configured OAuth authentication, you can proceed to make API calls to create or update sales orders. This section will guide you through the process using Python, ensuring you have the necessary tools and code snippets to interact with the Zoho Books API effectively.
Prerequisites for Zoho Books API Integration with Python
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer
pip
Install the requests
library, which is essential for making HTTP requests:
pip install requests
Creating a Sales Order in Zoho Books Using Python
To create a sales order, you will need to make a POST request to the Zoho Books API. Below is an example of how to do this using Python:
import requests
# Define the API endpoint and headers
url = "https://www.zohoapis.com/books/v3/salesorders?organization_id=YOUR_ORG_ID"
headers = {
"Authorization": "Zoho-oauthtoken YOUR_ACCESS_TOKEN",
"Content-Type": "application/json"
}
# Define the sales order data
sales_order_data = {
"customer_id": "460000000017138",
"line_items": [
{
"item_id": "460000000017088",
"quantity": 10,
"rate": 100
}
],
"date": "2023-10-01"
}
# Make the POST request
response = requests.post(url, headers=headers, json=sales_order_data)
# Check if the request was successful
if response.status_code == 201:
print("Sales Order Created Successfully")
else:
print("Failed to Create Sales Order:", response.json())
Replace YOUR_ORG_ID
and YOUR_ACCESS_TOKEN
with your actual organization ID and access token. The sales_order_data
dictionary contains the necessary fields for creating a sales order.
Updating a Sales Order in Zoho Books Using Python
To update an existing sales order, use a PUT request. Here's how you can update a sales order:
import requests
# Define the API endpoint and headers
url = "https://www.zohoapis.com/books/v3/salesorders/SALES_ORDER_ID?organization_id=YOUR_ORG_ID"
headers = {
"Authorization": "Zoho-oauthtoken YOUR_ACCESS_TOKEN",
"Content-Type": "application/json"
}
# Define the updated sales order data
updated_sales_order_data = {
"line_items": [
{
"line_item_id": "460000000039131",
"quantity": 15
}
]
}
# Make the PUT request
response = requests.put(url, headers=headers, json=updated_sales_order_data)
# Check if the request was successful
if response.status_code == 200:
print("Sales Order Updated Successfully")
else:
print("Failed to Update Sales Order:", response.json())
Replace SALES_ORDER_ID
, YOUR_ORG_ID
, and YOUR_ACCESS_TOKEN
with the appropriate values. The updated_sales_order_data
dictionary contains the fields you wish to update.
Verifying API Call Success and Handling Errors
After making an API call, verify the success by checking the response status code. A status code of 201
indicates successful creation, while 200
indicates a successful update. If the request fails, the response will contain error details, which you can log or display for debugging purposes.
Common error codes include:
400
- Bad request401
- Unauthorized (Invalid AuthToken)404
- URL Not Found429
- Rate Limit Exceeded
For more information on error codes, refer to the Zoho Books Error Documentation.
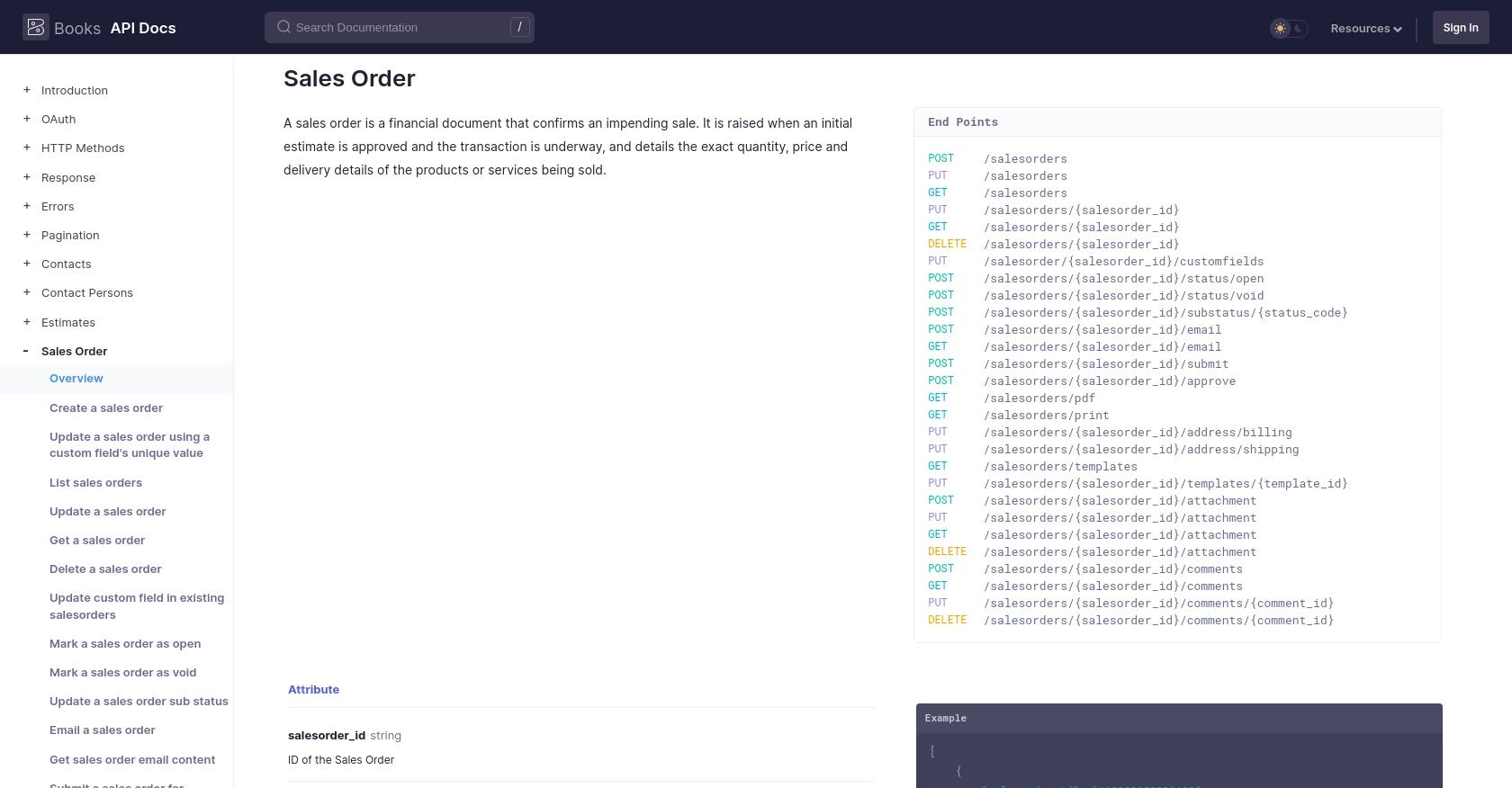
Conclusion and Best Practices for Zoho Books API Integration
Integrating with the Zoho Books API to manage sales orders can significantly enhance your business's operational efficiency. By automating the creation and updating of sales orders, you can reduce manual errors, ensure real-time inventory updates, and streamline your sales process.
Best Practices for Secure and Efficient Zoho Books API Usage
- Secure Storage of Credentials: Always store your OAuth credentials, including client ID, client secret, access tokens, and refresh tokens, securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handle Rate Limiting: Zoho Books API has a rate limit of 100 requests per minute per organization. Implement logic to handle rate limiting gracefully, such as retrying requests after a delay. For more details, refer to the API Call Limit Documentation.
- Error Handling: Implement robust error handling to manage API responses effectively. Log errors for debugging and provide user-friendly messages for known issues like authentication failures or invalid requests.
- Data Standardization: Ensure that data fields are standardized and validated before making API calls. This helps maintain data consistency across your systems.
Enhance Your Integration Strategy with Endgrate
While integrating with Zoho Books API can be a powerful tool for your business, managing multiple integrations can become complex. Endgrate offers a unified API solution that simplifies integration management across various platforms, including Zoho Books.
By leveraging Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product development.
- Build once for each use case instead of multiple times for different integrations, reducing redundancy and maintenance efforts.
- Provide an intuitive and seamless integration experience for your customers, enhancing user satisfaction and retention.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/sales-order/#overview
Ready to get started?