Using the Klaviyo API to Get Profiles (with PHP examples)
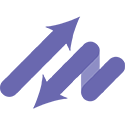
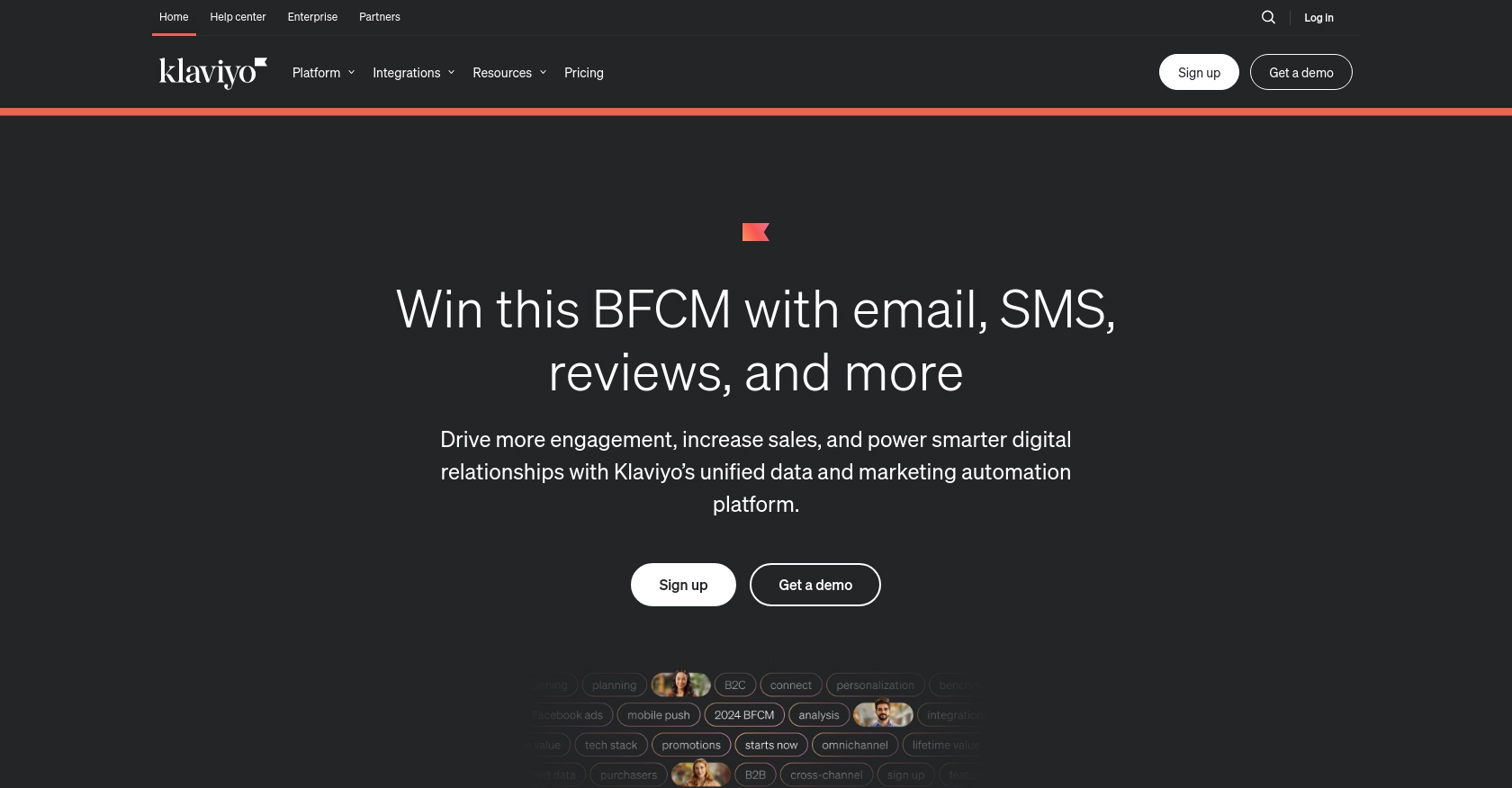
Introduction to Klaviyo and Its API
Klaviyo is a powerful email and SMS marketing platform that leverages data science to deliver personalized marketing experiences. It offers a robust API that allows developers to integrate Klaviyo's capabilities into their applications, enabling seamless access to customer data and marketing tools.
Connecting with Klaviyo's API can be highly beneficial for developers looking to enhance their marketing automation processes. For example, you might want to retrieve customer profiles to segment your audience more effectively or to personalize marketing campaigns based on user behavior and preferences.
This article will guide you through using the Klaviyo API to get profiles using PHP, providing you with the necessary steps and code examples to efficiently interact with Klaviyo's platform.
Setting Up Your Klaviyo Test Account and API Credentials
Before you can start using the Klaviyo API to retrieve profiles, you need to set up a test account and obtain the necessary API credentials. This process involves creating a Klaviyo account and generating an API key that will allow you to authenticate your requests.
Create a Klaviyo Account
If you don't already have a Klaviyo account, you can sign up for a free account on the Klaviyo website. This will give you access to the platform's features and allow you to test API interactions without any cost.
- Visit the Klaviyo website and click on the "Sign Up" button.
- Fill out the registration form with your details and complete the sign-up process.
- Once your account is created, log in to access the Klaviyo dashboard.
Generate API Credentials in Klaviyo
To interact with the Klaviyo API, you'll need to generate API credentials. Klaviyo uses API keys for authentication, and you can create these keys from your account settings.
- Navigate to the "Account" section in the top-right corner of the Klaviyo dashboard.
- Select "Settings" from the dropdown menu, then click on "API Keys" under the "Settings" tab.
- Click on the "Create API Key" button to generate a new private API key.
- Copy the generated API key and store it securely, as it will be used to authenticate your API requests.
For more detailed instructions, refer to the Klaviyo API documentation.
Understanding API Key Scopes
When creating an API key, you can define scopes to restrict access to specific data. For retrieving profiles, ensure that your API key has the profiles:read
scope. This will allow you to access profile data securely.
For more information on setting scopes, visit the Klaviyo documentation on API key scopes.
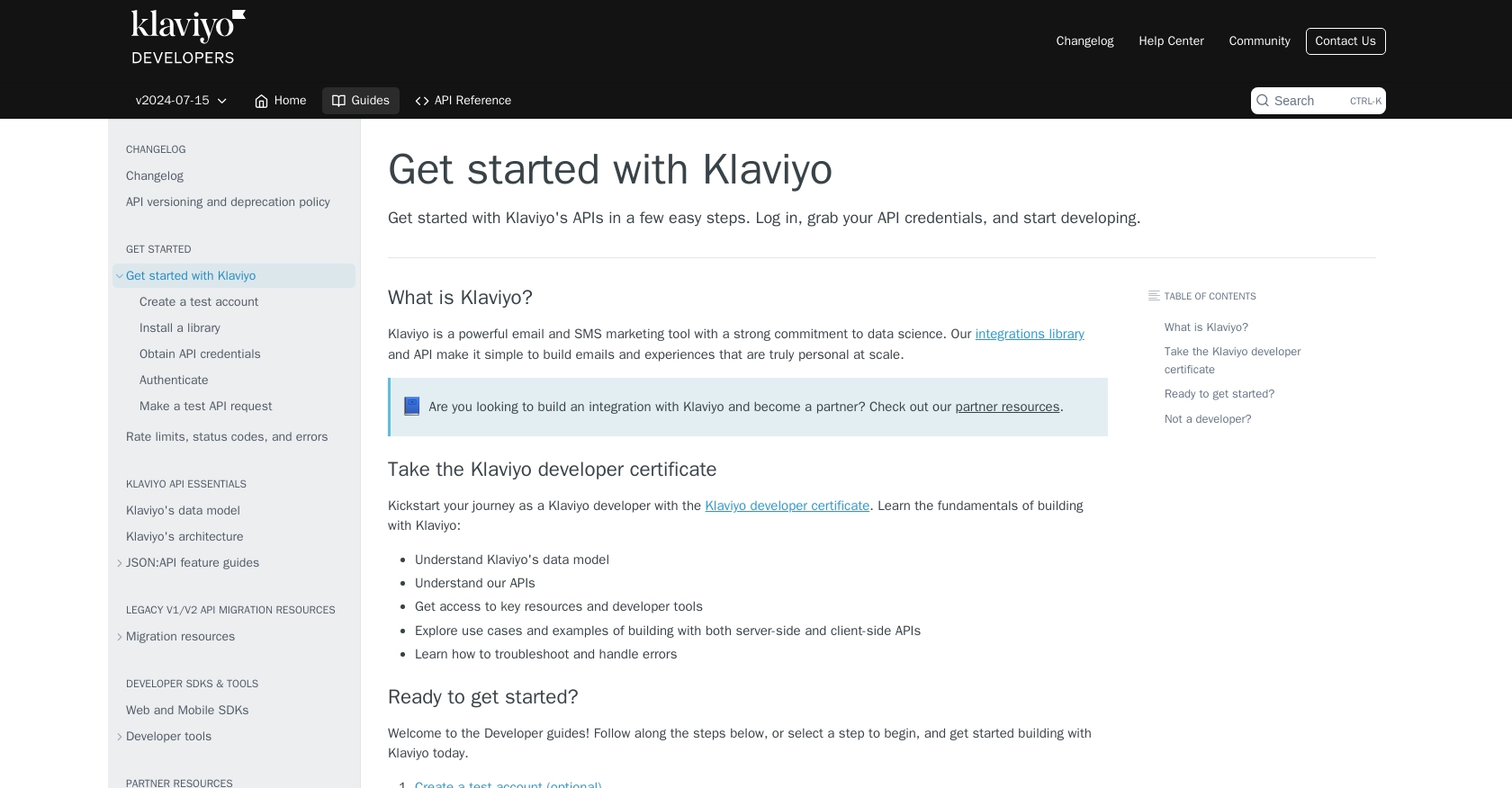
sbb-itb-96038d7
Making API Calls to Retrieve Klaviyo Profiles Using PHP
To effectively interact with the Klaviyo API and retrieve profiles, you'll need to use PHP to make HTTP requests. This section will guide you through the necessary steps, including setting up your PHP environment, writing the code to make API calls, and handling potential errors.
Setting Up Your PHP Environment for Klaviyo API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need PHP 7.4 or later and the cURL
extension enabled to handle HTTP requests.
- Verify your PHP version by running
php -v
in your terminal. - Ensure the
cURL
extension is enabled by checking yourphp.ini
file or runningphp -m | grep curl
.
Writing PHP Code to Retrieve Profiles from Klaviyo
With your environment set up, you can now write the PHP code to retrieve profiles from Klaviyo. The following example demonstrates how to make a GET request to the Klaviyo API to fetch profiles.
<?php
// Set the API endpoint and headers
$endpoint = 'https://a.klaviyo.com/api/profiles/';
$headers = [
'Authorization: Klaviyo-API-Key your-private-api-key',
'accept: application/json',
'revision: 2024-07-15'
];
// Initialize cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error:' . curl_error($ch);
} else {
// Parse and display the response
$data = json_decode($response, true);
foreach ($data['data'] as $profile) {
echo 'Email: ' . $profile['attributes']['email'] . '<br>';
echo 'First Name: ' . $profile['attributes']['first_name'] . '<br>';
echo 'Last Name: ' . $profile['attributes']['last_name'] . '<br><br>';
}
}
// Close cURL session
curl_close($ch);
?>
Replace your-private-api-key
with the API key you generated earlier. This script initializes a cURL session, sets the necessary headers, and sends a GET request to the Klaviyo API endpoint. It then parses the JSON response to display profile information.
Handling Errors and Verifying API Call Success
It's crucial to handle errors effectively when making API calls. The Klaviyo API uses standard HTTP status codes to indicate success or failure. Here are some common status codes you might encounter:
- 200 OK: The request was successful.
- 400 Bad Request: The request was malformed.
- 401 Not Authorized: Authentication failed. Check your API key.
- 429 Rate Limit: Too many requests. Implement rate limiting strategies.
For more detailed error handling, refer to the Klaviyo documentation on rate limits and error handling.
After executing your script, verify the profiles retrieved by checking the output against your Klaviyo account data. This ensures the API call was successful and the data is accurate.
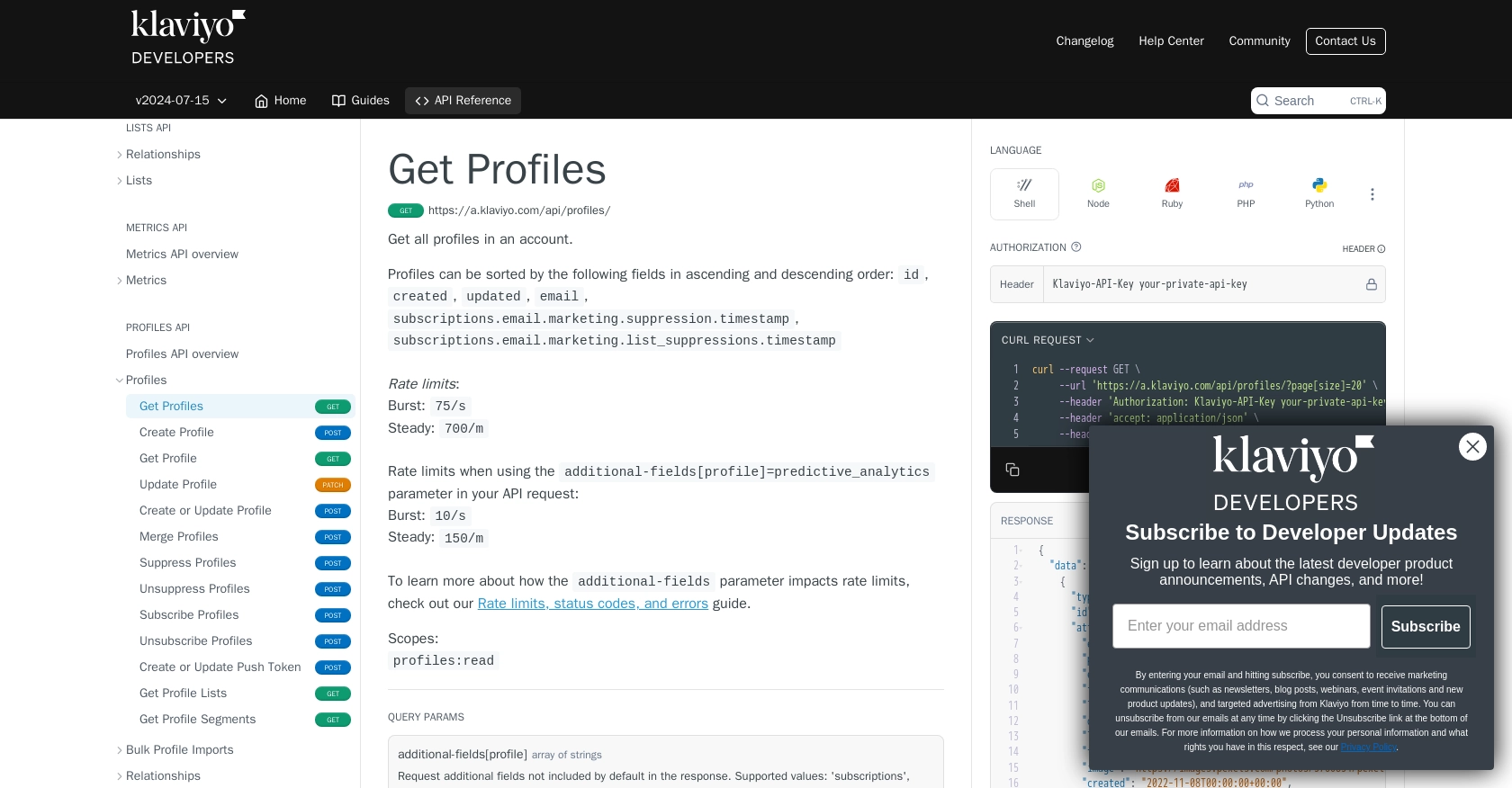
Conclusion and Best Practices for Using Klaviyo API with PHP
Integrating with the Klaviyo API using PHP allows developers to efficiently manage and retrieve customer profiles, enhancing marketing automation and personalization efforts. By following the steps outlined in this guide, you can seamlessly connect to Klaviyo's platform and leverage its powerful features.
Best Practices for Secure and Efficient API Integration
- Secure API Keys: Always store your API keys securely and avoid exposing them in client-side code or public repositories.
- Implement Rate Limiting: Be mindful of Klaviyo's rate limits to avoid disruptions. Implement strategies like exponential backoff to handle HTTP 429 errors gracefully. For more details, refer to the Klaviyo rate limits documentation.
- Data Transformation: Consider transforming and standardizing data fields to ensure consistency across different systems and applications.
Enhance Your Integration Strategy with Endgrate
While integrating with Klaviyo's API can significantly enhance your marketing capabilities, managing multiple integrations can be complex and time-consuming. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to various platforms, including Klaviyo.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development. Build once for each use case and enjoy an intuitive integration experience for your customers. Visit Endgrate to learn more about how you can simplify your integration processes.
Read More
Ready to get started?