How to Get Tasks with the Zoho CRM API in Javascript
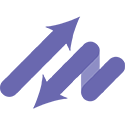
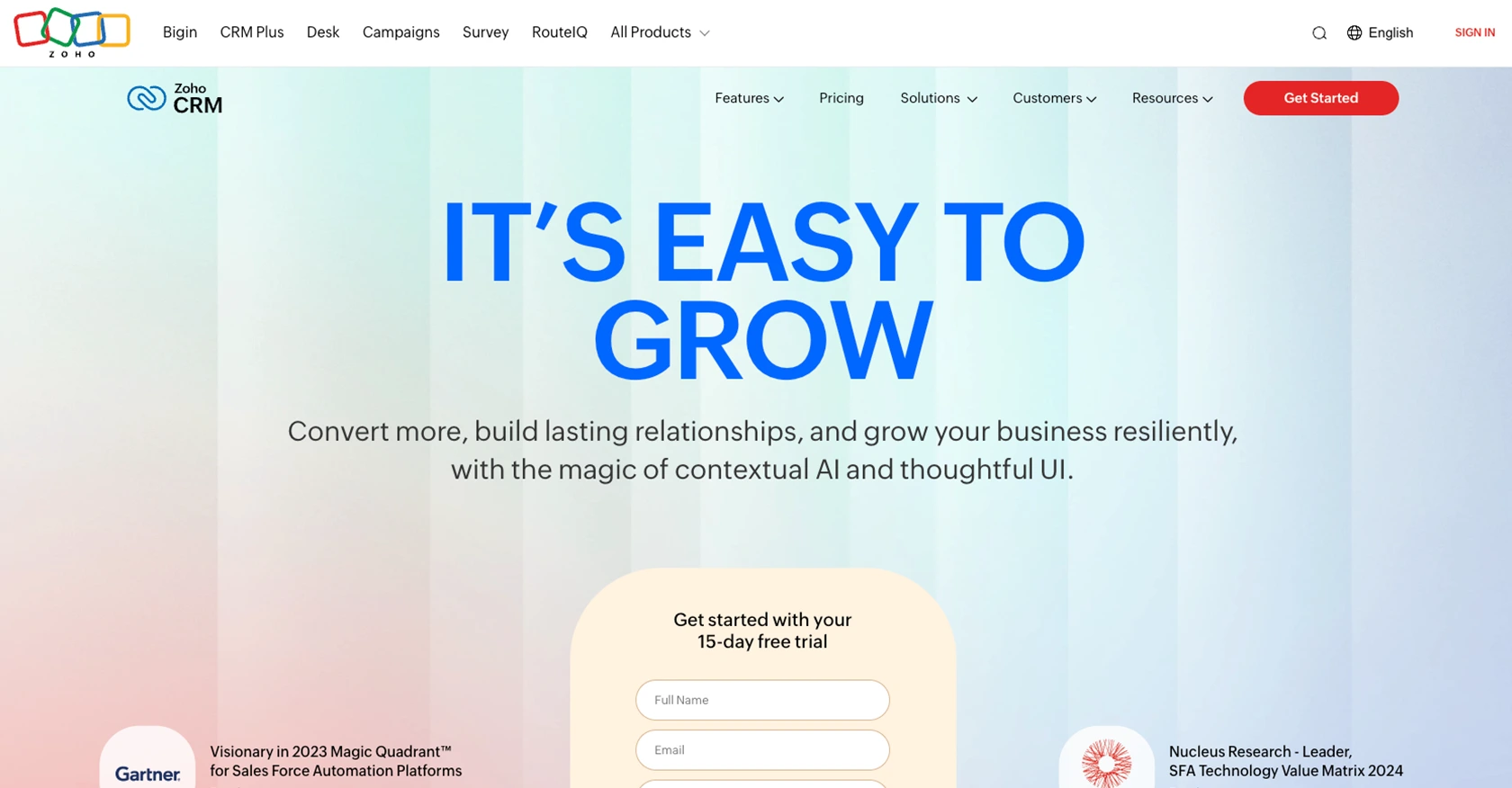
Introduction to Zoho CRM and Its API Integration
Zoho CRM is a comprehensive customer relationship management platform that empowers businesses to manage their sales, marketing, and support in a single system. Known for its flexibility and extensive features, Zoho CRM helps organizations streamline their operations and improve customer interactions.
For developers, integrating with the Zoho CRM API offers the ability to automate and enhance various business processes. By connecting with Zoho CRM, developers can access and manipulate data such as tasks, contacts, and deals, enabling seamless integration with other business tools and applications.
One practical use case is retrieving tasks from Zoho CRM using JavaScript. This can be particularly useful for developers looking to synchronize task data with other systems, automate task management, or create custom dashboards that display task information in real-time.
Setting Up Your Zoho CRM Sandbox Account for API Integration
Before you can start interacting with the Zoho CRM API using JavaScript, you'll need to set up a sandbox account. This allows you to test API calls without affecting your live data, providing a safe environment for development and experimentation.
Creating a Zoho CRM Sandbox Account
- Visit the Zoho Developer Console.
- Sign in with your Zoho account credentials or create a new account if you don't have one.
- Navigate to the "Sandbox" section and follow the prompts to create a new sandbox environment.
- Once your sandbox is set up, you can begin testing API integrations without impacting your live CRM data.
Registering Your Application for OAuth Authentication
Zoho CRM uses OAuth 2.0 for authentication, which requires you to register your application to obtain the necessary credentials.
- In the Zoho Developer Console, select "Register Client" to create a new application.
- Choose "JavaScript" as the client type, as your application will run in the browser.
- Fill in the required fields:
- Client Name: Enter a name for your application.
- Homepage URL: Provide the URL of your application's homepage.
- Authorized Redirect URIs: Specify the URL where Zoho will redirect users after authentication.
- Click "Create" to register your application. You will receive a Client ID and Client Secret.
Generating OAuth Tokens for API Access
With your application registered, you can now generate OAuth tokens to authenticate API requests.
- Direct users to the Zoho authorization URL with the appropriate scopes, such as
scope=ZohoCRM.modules.tasks.READ
. - After the user grants permission, Zoho will redirect them to your specified redirect URI with an authorization code.
- Exchange the authorization code for access and refresh tokens by making a POST request to Zoho's token endpoint.
Managing Access and Refresh Tokens
Access tokens are valid for a limited time, while refresh tokens can be used to obtain new access tokens without user intervention.
- Store the access and refresh tokens securely in your application.
- Use the refresh token to request a new access token when the current one expires.
- Ensure that tokens are not exposed in client-side code to prevent unauthorized access.
With your sandbox account and OAuth setup complete, you're ready to start making API calls to Zoho CRM and retrieve task data using JavaScript.
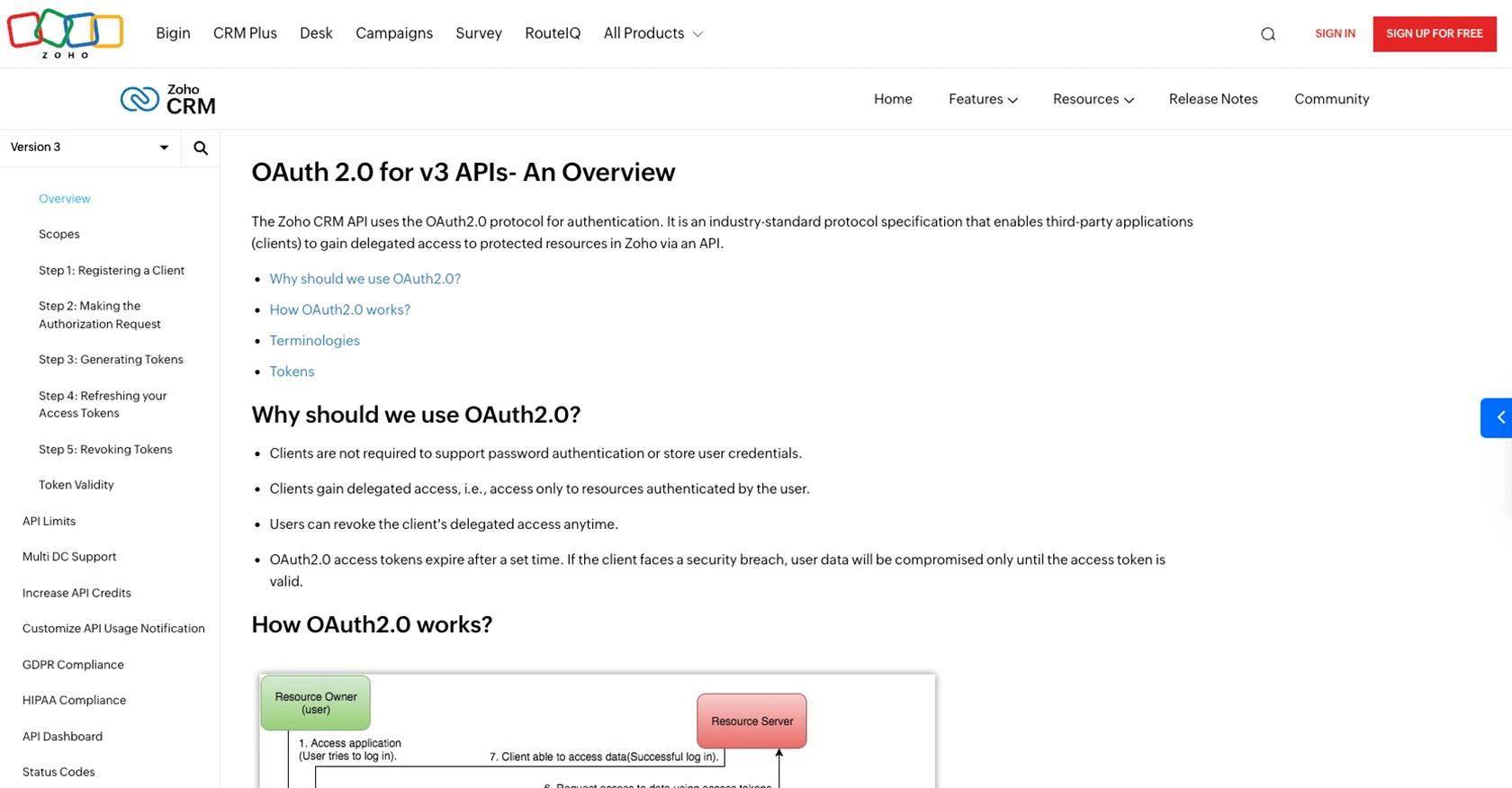
sbb-itb-96038d7
Making API Calls to Retrieve Tasks from Zoho CRM Using JavaScript
To interact with the Zoho CRM API and retrieve tasks using JavaScript, you'll need to ensure your development environment is properly set up. This involves using the right version of JavaScript and installing necessary dependencies.
Setting Up Your JavaScript Environment for Zoho CRM API Integration
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine, which includes npm (Node Package Manager).
- A text editor or IDE for writing your JavaScript code.
To manage HTTP requests, you can use the popular axios
library. Install it using npm with the following command:
npm install axios
Writing JavaScript Code to Fetch Tasks from Zoho CRM
Once your environment is ready, you can write the JavaScript code to fetch tasks from Zoho CRM. Create a file named getZohoTasks.js
and add the following code:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://www.zohoapis.com/crm/v3/Tasks';
const headers = {
'Authorization': 'Zoho-oauthtoken YOUR_ACCESS_TOKEN'
};
// Function to get tasks from Zoho CRM
async function getTasks() {
try {
const response = await axios.get(endpoint, { headers });
const tasks = response.data.data;
tasks.forEach(task => {
console.log(`Task ID: ${task.id}, Subject: ${task.Subject}`);
});
} catch (error) {
console.error('Error fetching tasks:', error.response ? error.response.data : error.message);
}
}
// Execute the function
getTasks();
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth authentication process.
Understanding the API Response and Handling Errors
When the API call is successful, the response will contain a list of tasks with details such as Task ID and Subject. You can verify the request's success by checking the console output for the task details.
If an error occurs, the catch block will handle it, and you can inspect the error message for troubleshooting. Common errors include invalid access tokens or exceeding API rate limits.
Verifying API Call Success in Zoho CRM Sandbox
To ensure the API call was successful, log in to your Zoho CRM sandbox account and navigate to the Tasks module. The tasks retrieved by your JavaScript code should match those displayed in the sandbox.
By following these steps, you can efficiently retrieve tasks from Zoho CRM using JavaScript, enabling seamless integration with your applications and systems.
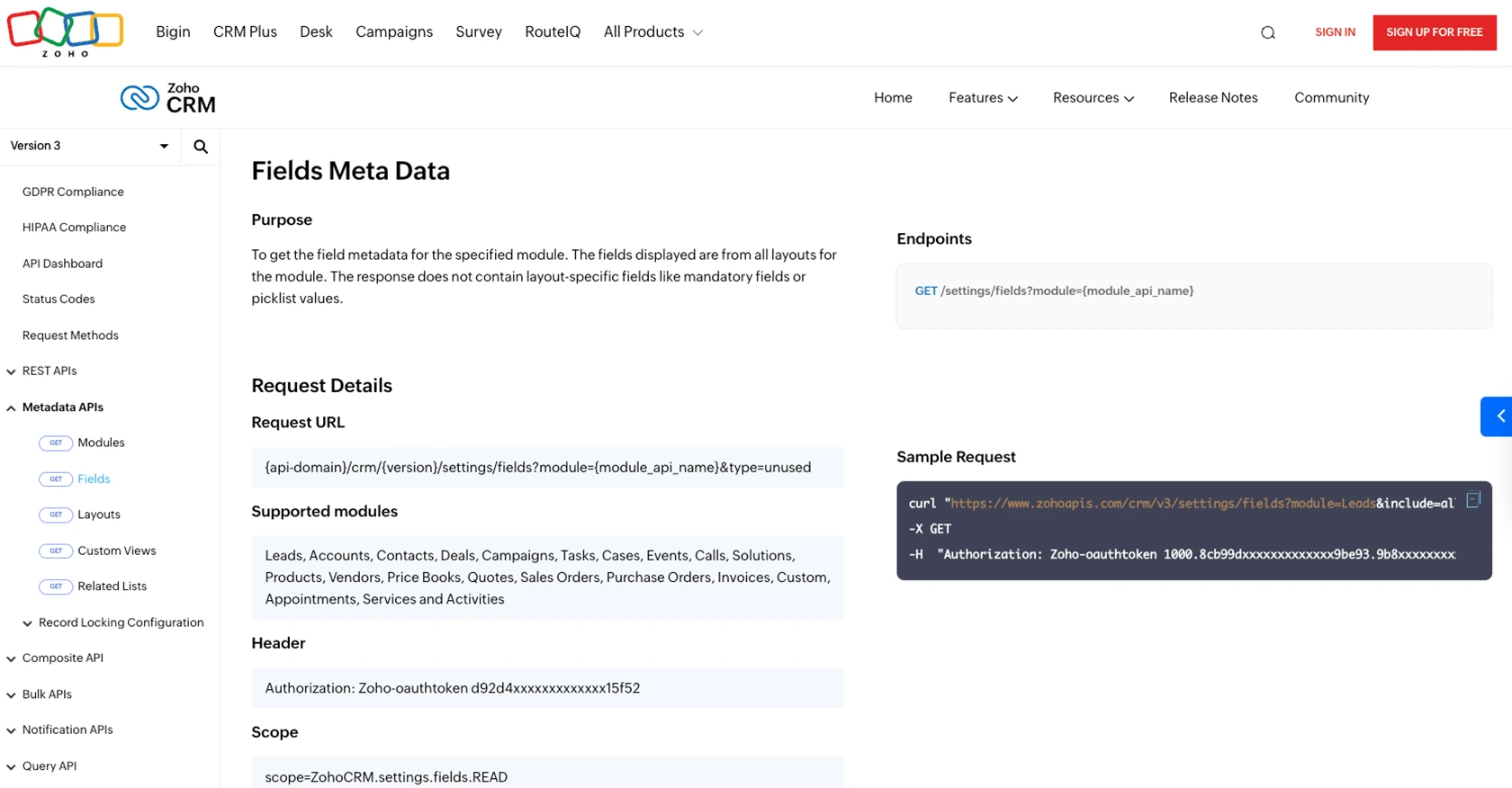
Conclusion: Best Practices for Zoho CRM API Integration Using JavaScript
Integrating with the Zoho CRM API using JavaScript provides developers with powerful capabilities to automate and enhance business processes. By following the steps outlined in this guide, you can efficiently retrieve tasks and integrate them with other systems, creating a seamless workflow.
Best Practices for Secure and Efficient Zoho CRM API Usage
- Secure Token Management: Always store your access and refresh tokens securely. Avoid exposing them in client-side code to prevent unauthorized access.
- Handle Rate Limits: Zoho CRM imposes API rate limits to ensure fair usage. Be mindful of these limits and implement retry logic to handle rate limit errors gracefully. For more details, refer to the Zoho CRM API Limits documentation.
- Data Standardization: When integrating data from Zoho CRM with other systems, ensure consistent data formats to maintain data integrity across platforms.
- Error Handling: Implement robust error handling to manage API errors effectively. Refer to the Zoho CRM Status Codes documentation for guidance on handling specific error codes.
Streamlining Integrations with Endgrate
While integrating with Zoho CRM can be highly beneficial, managing multiple integrations can become complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Zoho CRM. This allows you to build once for each use case and leverage an intuitive integration experience for your customers.
Explore how Endgrate can help you save time and resources by outsourcing integrations, allowing you to focus on your core product. Visit Endgrate to learn more about our solutions.
Read More
- https://endgrate.com/provider/zohocrm
- https://www.zoho.com/crm/developer/docs/api/v3/oauth-overview.html
- https://www.zoho.com/crm/developer/docs/api/v3/scopes.html
- https://www.zoho.com/crm/developer/docs/api/v3/register-client.html
- https://www.zoho.com/crm/developer/docs/api/v3/api-limits.html
- https://www.zoho.com/crm/developer/docs/api/v3/status-codes.html
- https://www.zoho.com/crm/developer/docs/api/v3/field-meta.html
- https://www.zoho.com/crm/developer/docs/api/v3/get-records.html
Ready to get started?