Using the Monday.com API to Get Users in Javascript
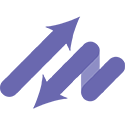
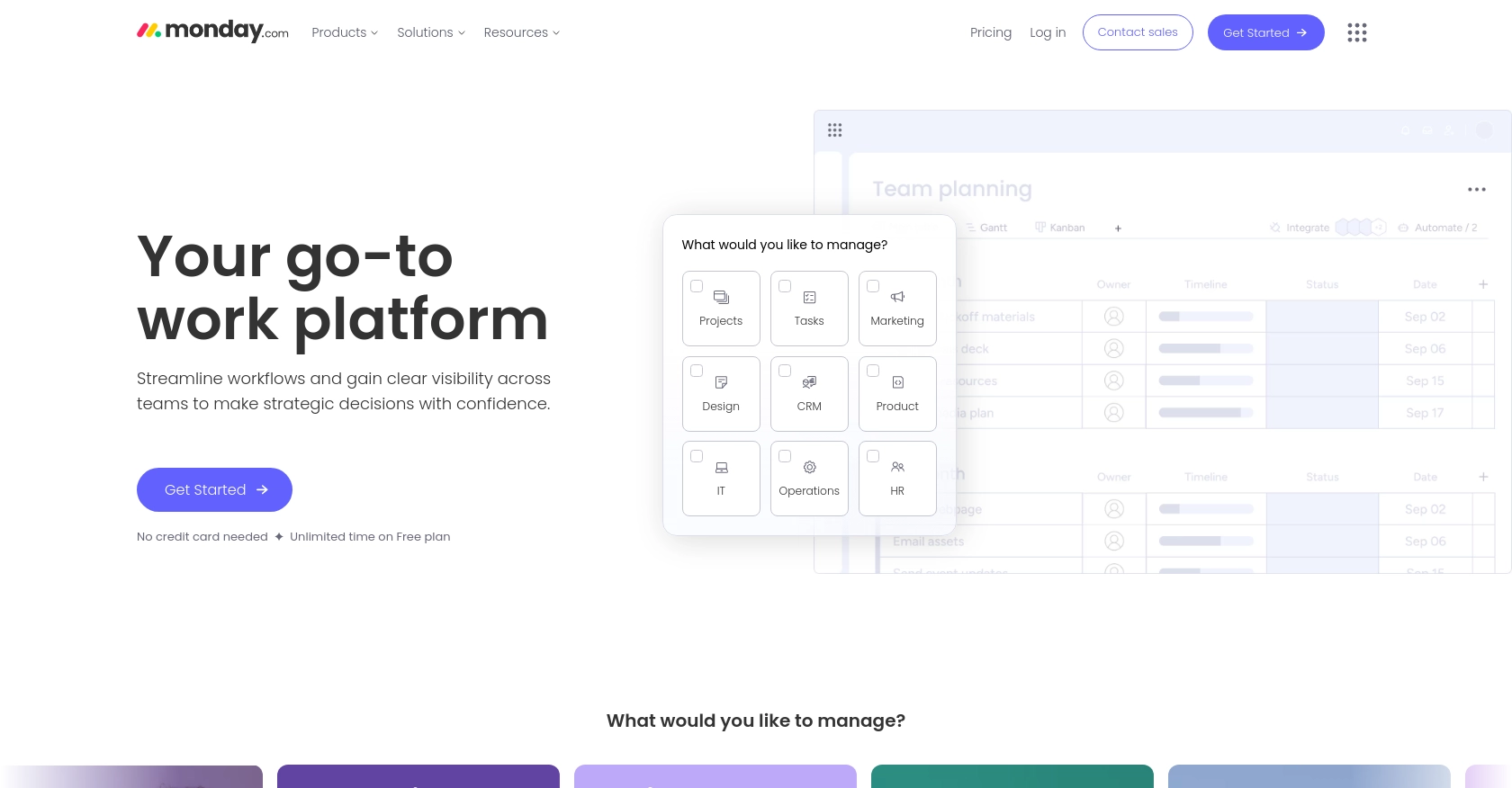
Introduction to Monday.com API
Monday.com is a versatile work operating system that empowers teams to run projects and workflows with confidence. It offers a highly customizable platform that integrates seamlessly with various tools, enhancing productivity and collaboration across organizations.
Developers often seek to integrate with Monday.com's API to streamline data management and automate tasks. For example, using the Monday.com API, a developer can retrieve user information to synchronize team data across different platforms, ensuring that all team members have access to the most up-to-date information.
Setting Up Your Monday.com API Test Account
Before you can start interacting with the Monday.com API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting your live data. Follow these steps to get started:
Create a Monday.com Account
- Visit the Monday.com website and sign up for a free trial or use an existing account.
- Once registered, log in to your account to access the dashboard.
Generate an API Token
To authenticate your API requests, you'll need an API token. Here's how to obtain it:
- Click on your profile picture in the top right corner of the Monday.com dashboard.
- Select Administration > Connections > API.
- Copy your personal API token. Note that regenerating a token will invalidate the previous one.
For more details, refer to the Monday.com API authentication documentation.
Set Up OAuth Authentication
If you're using OAuth for authentication, follow these steps to create an app:
- Navigate to the Developers section from your profile menu.
- Click on My Access Tokens and then Show to view your token.
- Ensure you have the necessary permission scopes for your app.
Verify Your Setup
With your API token ready, you can now make test API calls. Ensure that your token is included in the Authorization
header of your requests:
fetch("https://api.monday.com/v2", {
method: 'post',
headers: {
'Content-Type': 'application/json',
'Authorization': 'YOUR_API_TOKEN'
},
body: JSON.stringify({
query: "{ users { id name email } }"
})
})
.then(res => res.json())
.then(res => console.log(JSON.stringify(res, null, 2)));
Check the response to confirm that your setup is correct. You should see user data returned from your Monday.com account.
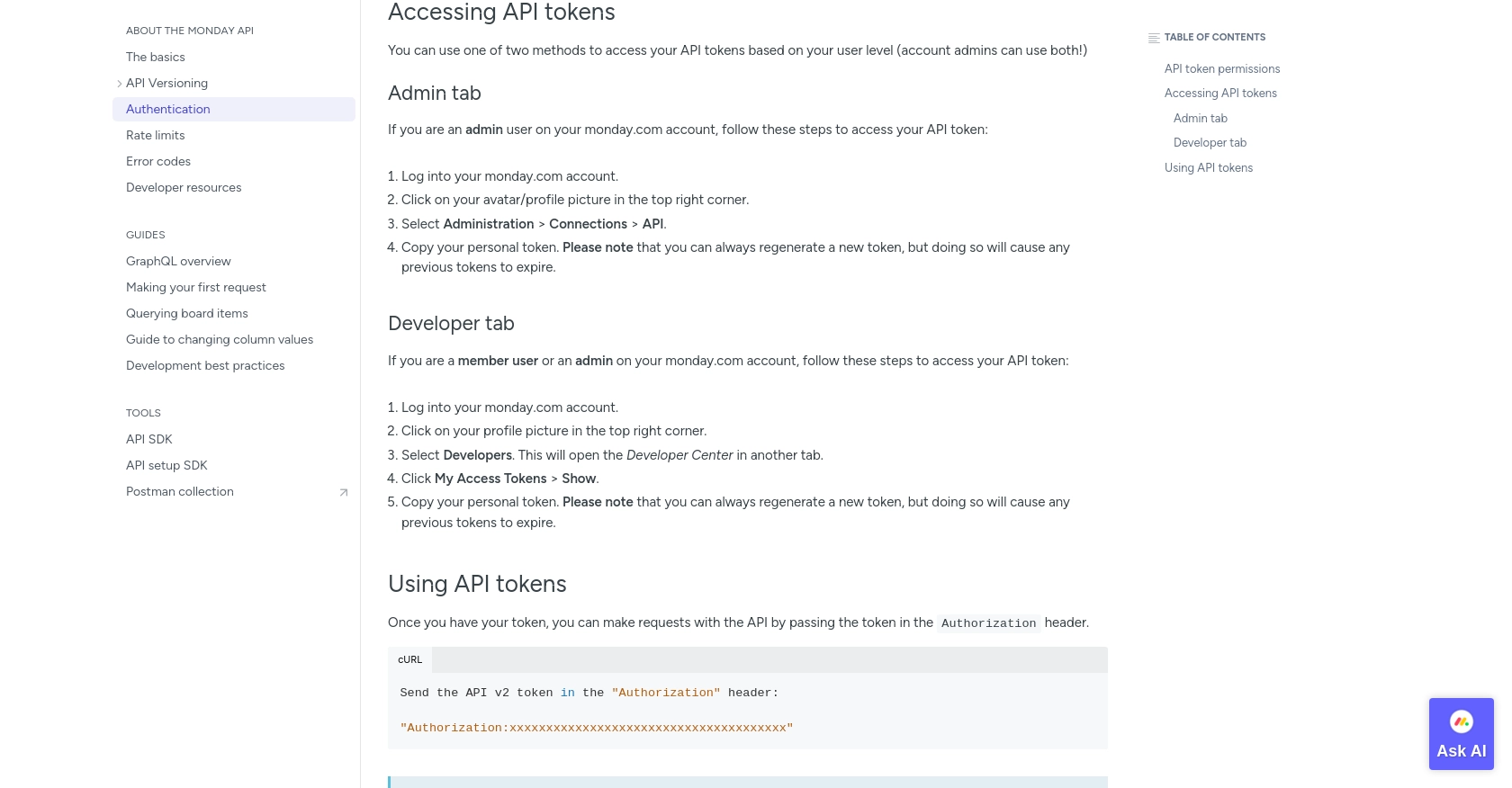
sbb-itb-96038d7
Making API Calls to Retrieve Users from Monday.com Using JavaScript
To interact with the Monday.com API and retrieve user information, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the necessary code, and handling potential errors.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A code editor like Visual Studio Code.
- Basic knowledge of JavaScript and asynchronous programming.
Installing Required Dependencies
To make HTTP requests, you can use the native fetch
API available in modern browsers. If you're working in a Node.js environment, consider using the node-fetch
package:
npm install node-fetch
Writing the JavaScript Code to Fetch Users
Create a new JavaScript file named getMondayUsers.js
and add the following code:
const fetch = require('node-fetch');
const query = `query {
users (limit: 50) {
id
name
email
}
}`;
fetch("https://api.monday.com/v2", {
method: 'post',
headers: {
'Content-Type': 'application/json',
'Authorization': 'YOUR_API_TOKEN'
},
body: JSON.stringify({ query })
})
.then(res => res.json())
.then(res => console.log(JSON.stringify(res, null, 2)))
.catch(error => console.error('Error fetching users:', error));
Replace YOUR_API_TOKEN
with the API token you obtained earlier. This script sends a POST request to the Monday.com API to retrieve user data.
Running the Script and Verifying the Output
Execute the script using Node.js:
node getMondayUsers.js
You should see a JSON response containing user information, such as IDs, names, and emails. Verify that the data matches the users in your Monday.com account.
Handling Errors and Understanding Error Codes
When making API calls, it's crucial to handle potential errors. The Monday.com API may return various error codes, such as:
- 401 Unauthorized: Ensure your API token is valid and included in the request header.
- 429 Rate Limit Exceeded: Reduce the number of requests sent per minute. Refer to the rate limits documentation.
- 500 Internal Server Error: Check for invalid arguments or malformed JSON.
For more detailed error handling, consult the Monday.com error codes documentation.
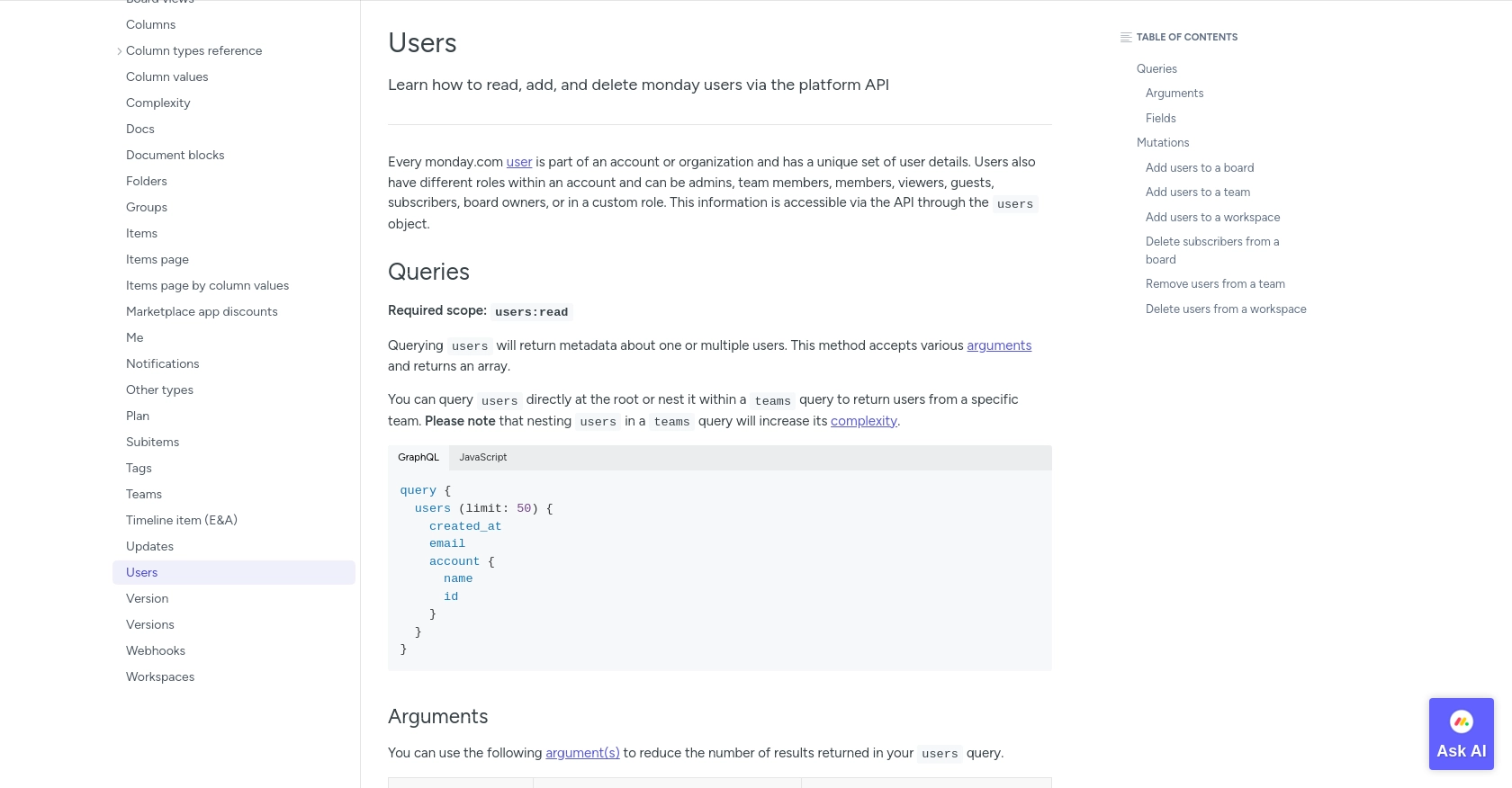
Conclusion and Best Practices for Using the Monday.com API in JavaScript
Integrating with the Monday.com API using JavaScript provides a powerful way to manage and automate workflows, ensuring that your team has access to the most current data. By following the steps outlined in this guide, you can efficiently retrieve user information and enhance your application's capabilities.
Best Practices for Secure and Efficient API Integration with Monday.com
- Securely Store API Tokens: Always store your API tokens securely and avoid hardcoding them in your scripts. Consider using environment variables or a secure vault.
- Handle Rate Limits: Be mindful of the rate limits imposed by Monday.com. Implement retry logic and backoff strategies to handle
429 Rate Limit Exceeded
errors. For more information, refer to the rate limits documentation. - Optimize Query Complexity: Use pagination and limit the fields you request to reduce query complexity and avoid hitting complexity limits. This will help maintain efficient API usage.
- Implement Error Handling: Ensure robust error handling in your application to manage various API errors gracefully. Refer to the error codes documentation for guidance.
Enhance Your Integration Strategy with Endgrate
While integrating with Monday.com can significantly boost your productivity, managing multiple integrations can be time-consuming. Endgrate offers a unified API solution that simplifies integration processes across various platforms, including Monday.com. By leveraging Endgrate, you can focus on your core product development while ensuring a seamless integration experience for your users.
Visit Endgrate to learn more about how you can streamline your integration strategy and save valuable time and resources.
Read More
Ready to get started?