How to Get Users with the Salesloft API in Javascript
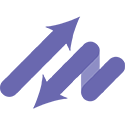
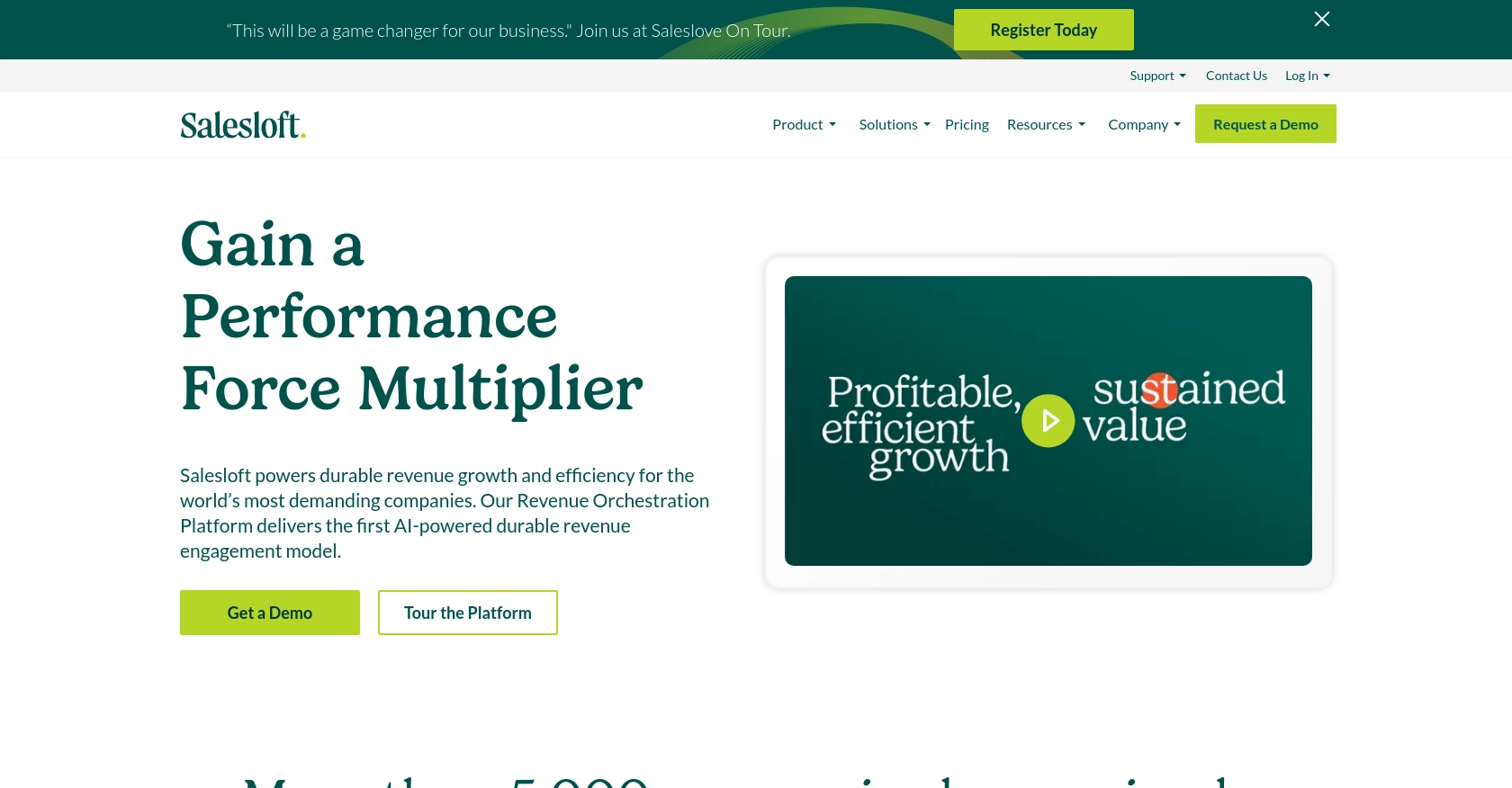
Introduction to Salesloft API Integration
Salesloft is a powerful sales engagement platform that enables sales teams to enhance their communication and workflow processes. By providing tools for email tracking, call logging, and analytics, Salesloft helps sales professionals connect with prospects more effectively and efficiently.
Integrating with the Salesloft API allows developers to access and manage user data, automate tasks, and streamline sales operations. For example, a developer might use the Salesloft API to retrieve a list of users within their organization, enabling them to build custom dashboards or automate reporting processes.
Setting Up Your Salesloft Test Account
Before you can start interacting with the Salesloft API, you need to set up a test account. This involves creating an OAuth application within your Salesloft account to obtain the necessary credentials for API access.
Creating a Salesloft OAuth Application
- Log in to your Salesloft account.
- Navigate to Your Applications under the account settings.
- Select OAuth Applications and click on Create New.
- Fill in the required fields, such as the application name and description, and click Save.
- After saving, you will receive your Application Id (Client Id) and Secret (Client Secret). Make sure to note these down as they will be needed for authentication.
Obtaining Authorization Code and Access Tokens
To interact with the Salesloft API, you need to obtain an access token using the OAuth 2.0 authorization flow. Follow these steps:
- Generate a request to the authorization endpoint by replacing
YOUR_CLIENT_ID
andYOUR_REDIRECT_URI
in the following URL: - Authorize the application when prompted. Upon approval, your redirect URI will receive a query parameter
code
. - Use this code to request an access token by making a POST request to the token endpoint:
- You will receive a JSON response containing the
access_token
andrefresh_token
. Store these securely as they are required for making API requests.
https://accounts.salesloft.com/oauth/authorize?client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&response_type=code
POST https://accounts.salesloft.com/oauth/token { "client_id": "YOUR_CLIENT_ID", "client_secret": "YOUR_CLIENT_SECRET", "code": "YOUR_AUTHORIZATION_CODE", "grant_type": "authorization_code", "redirect_uri": "YOUR_REDIRECT_URI" }
For more detailed information, refer to the Salesloft OAuth Authentication documentation.
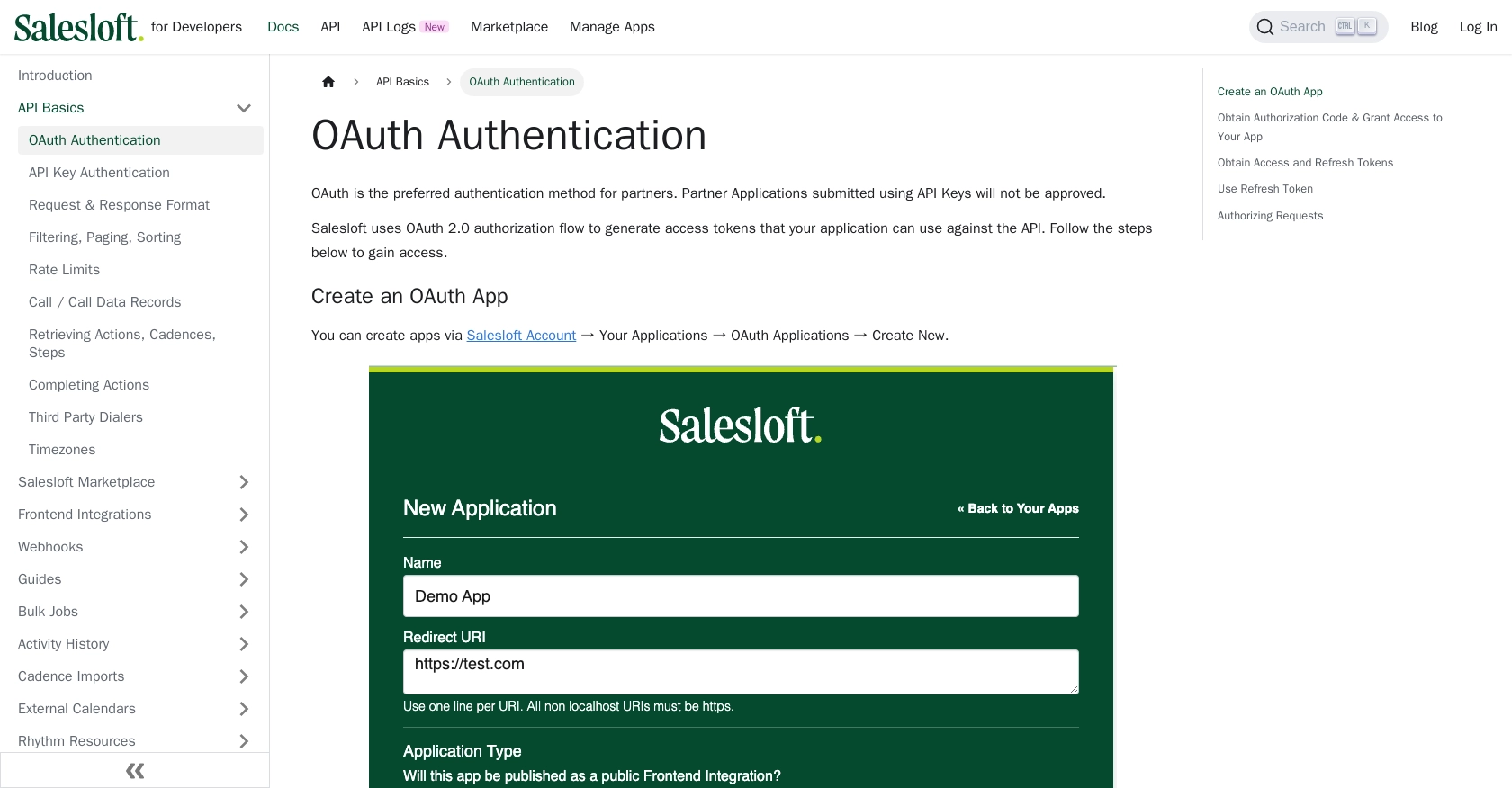
sbb-itb-96038d7
Making API Calls to Retrieve Users with Salesloft API in JavaScript
To interact with the Salesloft API and retrieve user data, you'll need to make HTTP requests using JavaScript. This section will guide you through the process of setting up your environment, writing the code, and handling responses effectively.
Setting Up Your JavaScript Environment for Salesloft API
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides the runtime environment for executing JavaScript code outside a browser. You can download it from the official Node.js website.
Once Node.js is installed, create a new project directory and navigate to it in your terminal. Initialize a new Node.js project by running:
npm init -y
Next, install the axios
library, which simplifies making HTTP requests:
npm install axios
Writing JavaScript Code to Fetch Users from Salesloft API
Create a new file named get_salesloft_users.js
and add the following code:
const axios = require('axios'); // Set the API endpoint and headers const endpoint = 'https://api.salesloft.com/v2/users'; const headers = { 'Authorization': 'Bearer YOUR_ACCESS_TOKEN', 'Accept': 'application/json' }; // Function to fetch users async function fetchUsers() { try { const response = await axios.get(endpoint, { headers }); const users = response.data.data; // Display user information users.forEach(user => { console.log(`ID: ${user.id}, Name: ${user.name}, Email: ${user.email}`); }); } catch (error) { console.error('Error fetching users:', error.response ? error.response.data : error.message); } } // Execute the function fetchUsers();
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth authentication process.
Running the JavaScript Code and Verifying Results
To execute the code, run the following command in your terminal:
node get_salesloft_users.js
If successful, the script will output a list of users with their IDs, names, and email addresses.
Handling Errors and Validating API Responses
When making API calls, it's crucial to handle potential errors. The code above includes a try-catch
block to catch and log any errors that occur during the request. Common error codes include:
- 403 Forbidden: Access is denied. Check your authorization token.
- 404 Not Found: The requested resource does not exist.
- 422 Unprocessable Entity: Invalid request parameters.
Refer to the Salesloft Request & Response Format documentation for more details on handling errors.
By following these steps, you can efficiently retrieve user data from Salesloft using JavaScript, enabling you to integrate and automate your sales processes seamlessly.
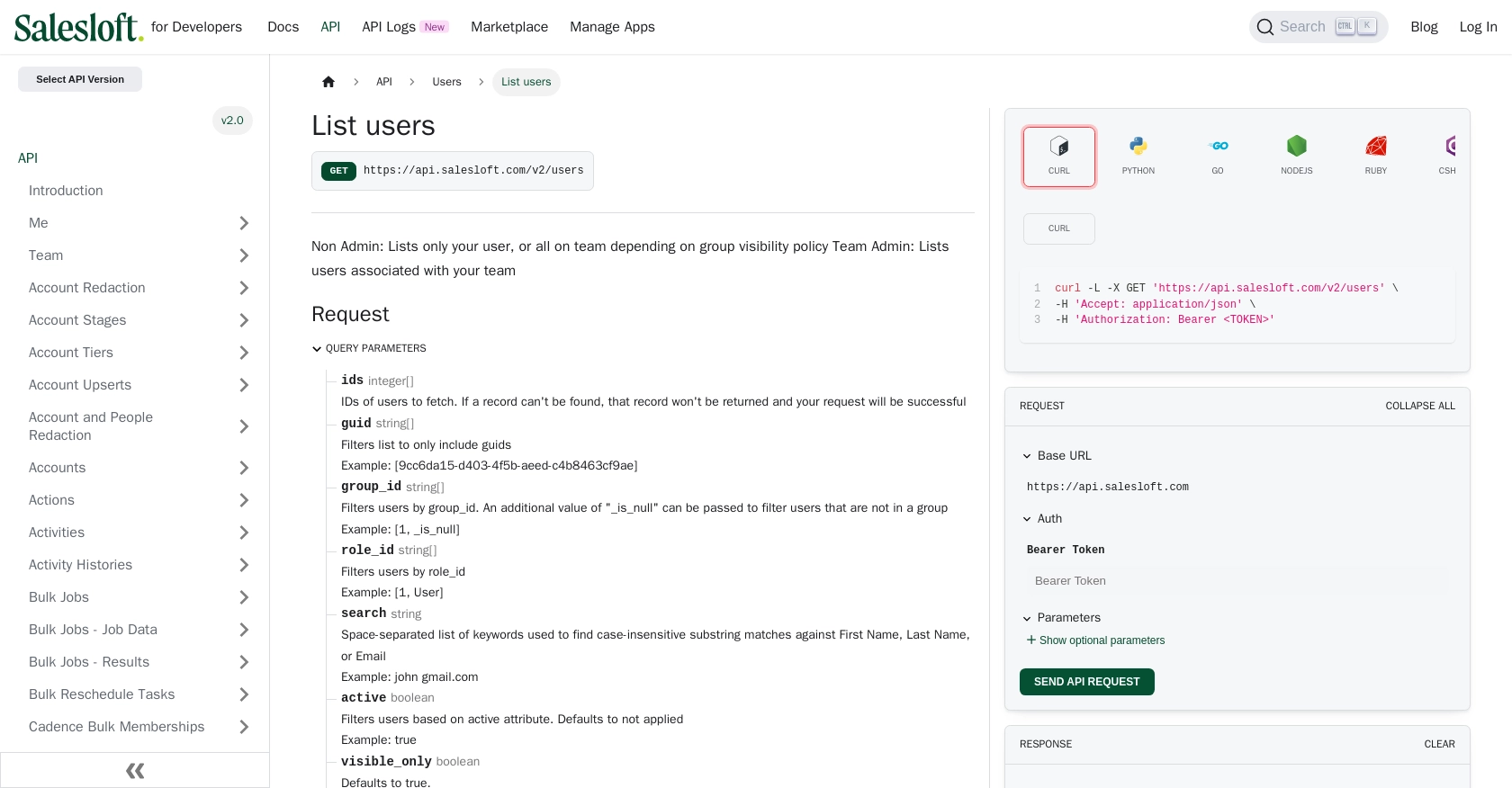
Conclusion and Best Practices for Integrating with Salesloft API Using JavaScript
Integrating with the Salesloft API using JavaScript provides a powerful way to automate and enhance your sales processes. By retrieving user data, you can build custom solutions that streamline operations and improve efficiency.
Best Practices for Secure and Efficient Salesloft API Integration
- Securely Store Credentials: Always store your access and refresh tokens securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limits: Be mindful of Salesloft's rate limits, which are set at 600 cost per minute. Implement logic to handle rate limiting gracefully, such as retry mechanisms or backoff strategies. For more details, refer to the Salesloft Rate Limits documentation.
- Manage Token Expiry: Use the refresh token to obtain a new access token when the current one expires. This ensures uninterrupted access to the API.
- Data Transformation: Consider transforming and standardizing data fields to match your application's requirements, ensuring consistency across different systems.
Enhance Your Integration Strategy with Endgrate
While integrating with Salesloft can significantly boost your sales operations, managing multiple integrations can be complex and time-consuming. Endgrate offers a streamlined solution by providing a unified API endpoint for various platforms, including Salesloft.
With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers. Explore how Endgrate can simplify your integration strategy by visiting Endgrate.
Read More
- https://endgrate.com/provider/salesloft
- https://developers.salesloft.com/docs/platform/api-basics/oauth-authentication/
- https://developers.salesloft.com/docs/platform/api-basics/request-response-format/
- https://developers.salesloft.com/docs/platform/api-basics/filtering-paging-sorting/
- https://developers.salesloft.com/docs/platform/api-basics/rate-limits/
- https://developers.salesloft.com/docs/api/users-index/
Ready to get started?