How to Create Products with the DDI API in PHP
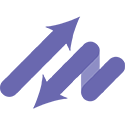
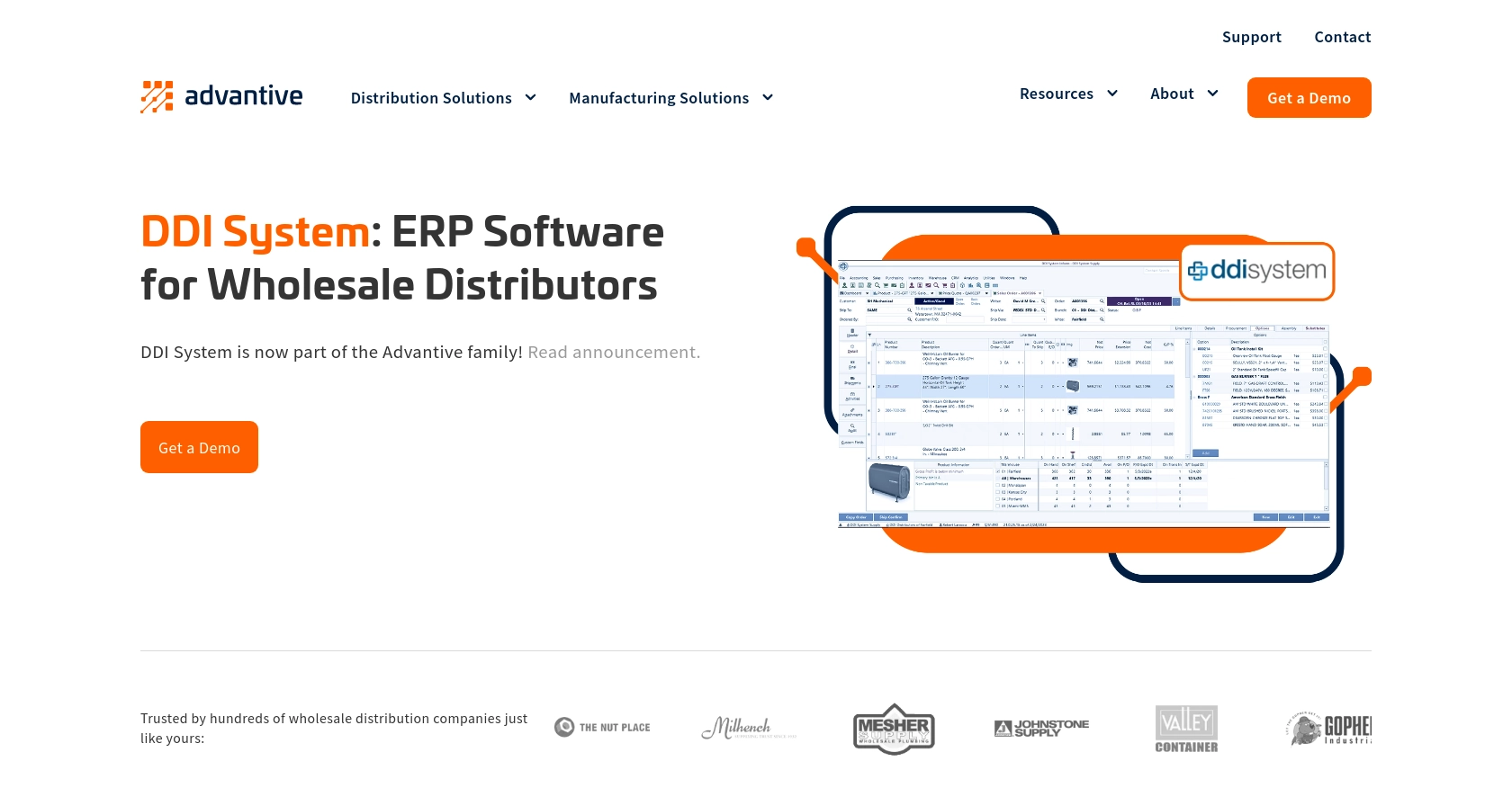
Introduction to DDI Product Integration
DDI is a robust platform that facilitates efficient product management and distribution for businesses. It offers a comprehensive suite of tools designed to streamline inventory, pricing, and vendor management, making it an essential resource for companies looking to optimize their supply chain operations.
Developers may seek to integrate with DDI to automate the process of managing product data. For example, a developer might want to push product information such as SKU, price, and inventory levels to DDI's system to ensure real-time updates and accuracy across platforms.
This article will guide you through the process of creating products using the DDI API with PHP, providing detailed steps to efficiently manage product data on the DDI platform.
Setting Up Your DDI Test/Sandbox Account for Product Integration
Before you begin integrating with the DDI platform, it's essential to set up a test or sandbox account. This allows you to safely experiment with the integration process without affecting live data. Unfortunately, DDI does not provide a publicly accessible API. Instead, a custom FTP integration is used to push product data to their FTP server.
Creating a Custom FTP Integration for DDI
To interact with DDI, you need to establish a custom FTP integration. Follow these steps to set up your environment:
- Contact DDI Support: Reach out to DDI's support team to request access to their FTP server. They will provide you with the necessary credentials and server details.
- Configure FTP Client: Use an FTP client like FileZilla or WinSCP to connect to the DDI FTP server using the credentials provided by DDI.
- Prepare Product Data: Structure your product data according to the schema required by DDI. The schema includes fields such as Variant SKU, Variant Price, and Product Description.
Understanding DDI's Product Data Schema
When preparing your product data, ensure it aligns with DDI's schema. Here is a brief overview of the required fields:
- Variant SKU: A unique identifier for each product variant.
- Variant Price: The price of the product variant.
- Variant Inventory Quantity: The available stock quantity for the variant.
- Vendor Name: The name of the product vendor.
- Item Cost: The cost price of the item.
- Product Category: The category under which the product falls.
- Product Type: The type of product.
- UPC: The Universal Product Code for the product.
- Unit of Measure: The unit in which the product is measured.
- Product Description: A detailed description of the product.
- Thumbnail URL: A URL pointing to the product's thumbnail image.
- Weight: The weight of the product.
- Specs File PDF URL: A URL to the PDF file containing product specifications.
Ensure that your data is formatted correctly and matches the types specified in the schema. This will facilitate a smooth integration process with DDI's system.
Implementing Product Data Transfer to DDI Using PHP
To effectively push product data to the DDI platform, you'll need to utilize PHP to handle the FTP integration. This section will guide you through the necessary steps to set up your PHP environment and execute the data transfer.
Setting Up Your PHP Environment for DDI FTP Integration
Before proceeding, ensure your PHP environment is correctly configured. You'll need PHP installed on your system, along with the FTP extension enabled. Follow these steps to prepare your environment:
- Install PHP: Ensure PHP is installed on your server or local machine. You can download it from the official PHP website.
- Enable FTP Extension: Verify that the FTP extension is enabled in your
php.ini
file. Look for the lineextension=ftp
and ensure it is uncommented. - Install Dependencies: Use Composer to manage any additional dependencies your project might require.
Writing PHP Code to Transfer Product Data to DDI
With your environment set up, you can now write the PHP code to transfer product data to DDI's FTP server. Below is a sample script to guide you:
<?php
// FTP server details
$ftp_server = "ftp.ddi.com";
$ftp_username = "your_username";
$ftp_password = "your_password";
// Establish an FTP connection
$conn_id = ftp_connect($ftp_server);
// Login to the FTP server
$login_result = ftp_login($conn_id, $ftp_username, $ftp_password);
// Check connection and login result
if ((!$conn_id) || (!$login_result)) {
die("FTP connection has failed!");
}
// Prepare product data in CSV format
$product_data = "Variant SKU,Variant Price,Variant Inventory Quantity,Vendor Name,Item Cost,Product Category,Product Type,UPC,Unit of Measure,Product Description,Thumbnail URL,Weight,Specs File PDF URL\n";
$product_data .= "12345,19.99,100,Vendor A,15.00,Electronics,Phone,0123456789012,Piece,Smartphone with 4G,https://example.com/image.jpg,0.5,https://example.com/specs.pdf\n";
// Create a temporary file
$temp_file = tmpfile();
fwrite($temp_file, $product_data);
fseek($temp_file, 0);
// Upload the file to the FTP server
$upload = ftp_fput($conn_id, "products.csv", $temp_file, FTP_ASCII);
// Check upload status
if (!$upload) {
echo "FTP upload has failed!";
} else {
echo "Product data uploaded successfully.";
}
// Close the FTP connection
ftp_close($conn_id);
// Close the temporary file
fclose($temp_file);
?>
In this script, you establish a connection to the DDI FTP server using the provided credentials. The product data is formatted as a CSV string and written to a temporary file, which is then uploaded to the server. Ensure that your product data aligns with the schema required by DDI.
Verifying Successful Data Transfer to DDI
After executing the script, you should verify that the product data has been successfully uploaded to the DDI FTP server. You can do this by:
- Checking FTP Logs: Review the FTP logs to confirm the successful transfer of the file.
- Contacting DDI Support: Reach out to DDI support to ensure the data has been processed correctly on their end.
By following these steps, you can efficiently manage product data integration with DDI using PHP, ensuring real-time updates and accuracy across your platforms.
Conclusion and Best Practices for DDI Product Integration Using PHP
Integrating with DDI through a custom FTP setup allows developers to automate and streamline product data management, ensuring that inventory, pricing, and vendor information are consistently updated across platforms. By leveraging PHP for this integration, you can efficiently handle data transfers and maintain data accuracy.
Best Practices for Secure and Efficient DDI Integration
- Secure Credentials: Always store FTP credentials securely, using environment variables or encrypted storage solutions to prevent unauthorized access.
- Data Validation: Ensure that all product data is validated against DDI's schema before uploading to avoid errors and ensure data integrity.
- Rate Limiting: Although DDI's FTP integration does not have explicit rate limits, it's best to schedule uploads during off-peak hours to avoid potential server overloads.
- Error Handling: Implement robust error handling in your PHP scripts to catch and log any issues during the FTP connection or file upload process.
Enhance Your Integration Strategy with Endgrate
While integrating with DDI using PHP and FTP is effective, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes across various platforms, including DDI.
By using Endgrate, you can:
- Save time and resources by outsourcing integration tasks and focusing on your core product development.
- Build once for each use case, reducing the need for multiple custom integrations.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate today.
Read More
Ready to get started?