How to Get Meetings with the Zoom API in PHP
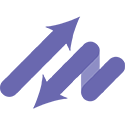
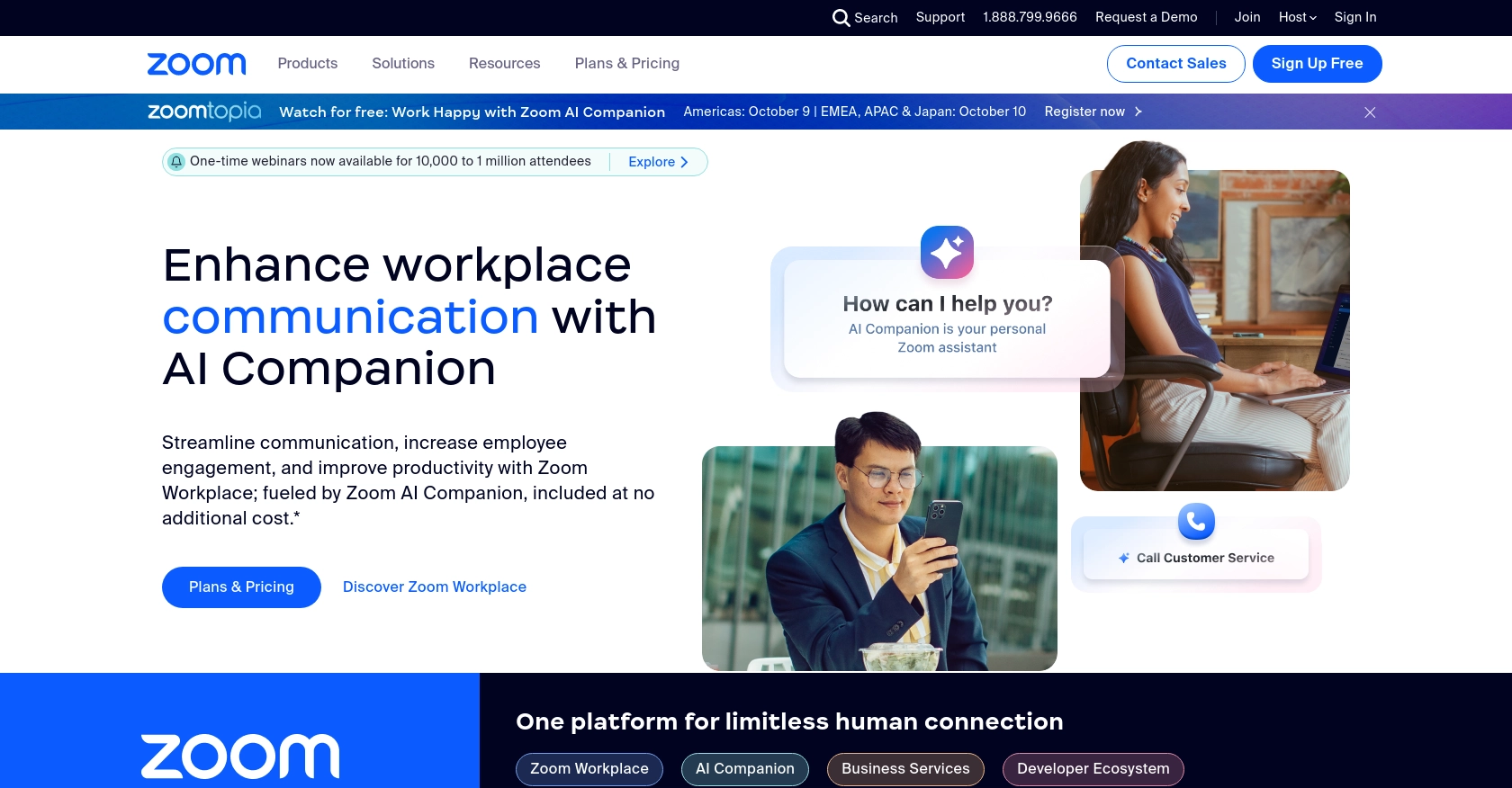
Introduction to Zoom API Integration
Zoom is a widely-used video conferencing platform that offers robust APIs for developers to enhance and automate meeting functionalities. With the Zoom API, developers can access a variety of features, such as scheduling meetings, retrieving meeting details, and managing participants, all of which can be seamlessly integrated into B2B SaaS products.
Connecting with the Zoom API can significantly streamline communication processes within applications. For example, a developer might want to retrieve upcoming meetings to display them in a custom dashboard, enabling users to manage their schedules more effectively without leaving the application.
Setting Up Your Zoom Developer Account and OAuth App
Before you can start integrating with the Zoom API, you need to set up a Zoom Developer account and create an OAuth app. This will allow you to authenticate API requests and access Zoom's resources securely.
Creating a Zoom Developer Account
If you don't have a Zoom Developer account, follow these steps to create one:
- Visit the Zoom App Marketplace and sign up for a developer account.
- Once registered, log in to your account to access the Zoom Developer Dashboard.
Creating an OAuth App for Zoom API
To interact with the Zoom API, you need to create an OAuth app. Follow these steps:
- In the Zoom Developer Dashboard, click on "Develop" in the top navigation bar and select "Build App".
- Choose the "OAuth" app type and click "Create".
- Fill in the required information such as the app name, company name, and developer contact information.
- Under "App Credentials", note down the Client ID and Client Secret. These will be used for authentication.
- Set the "Redirect URL for OAuth" to the URL where you want users to be redirected after they authorize your app.
- Define the necessary scopes for your app. For accessing meetings, ensure you include scopes like
meeting:read
andmeeting:write
. - Click "Continue" and complete any additional setup steps required by Zoom.
Generating Access Tokens Using OAuth
With your OAuth app set up, you can now generate access tokens to authenticate API requests:
- Direct users to the Zoom authorization URL, which includes your Client ID and redirect URI.
- Once the user authorizes your app, Zoom will redirect them to your specified redirect URI with an authorization code.
- Exchange this authorization code for an access token by making a POST request to Zoom's token endpoint.
// Example PHP code to exchange authorization code for access token
$client_id = 'YOUR_CLIENT_ID';
$client_secret = 'YOUR_CLIENT_SECRET';
$authorization_code = 'AUTHORIZATION_CODE';
$redirect_uri = 'YOUR_REDIRECT_URI';
$url = 'https://zoom.us/oauth/token';
$data = [
'grant_type' => 'authorization_code',
'code' => $authorization_code,
'redirect_uri' => $redirect_uri
];
$options = [
'http' => [
'header' => "Authorization: Basic " . base64_encode("$client_id:$client_secret"),
'method' => 'POST',
'content' => http_build_query($data)
]
];
$context = stream_context_create($options);
$response = file_get_contents($url, false, $context);
$token_data = json_decode($response, true);
echo 'Access Token: ' . $token_data['access_token'];
Ensure you securely store the access token and refresh token, as they will be used to authenticate future API requests.
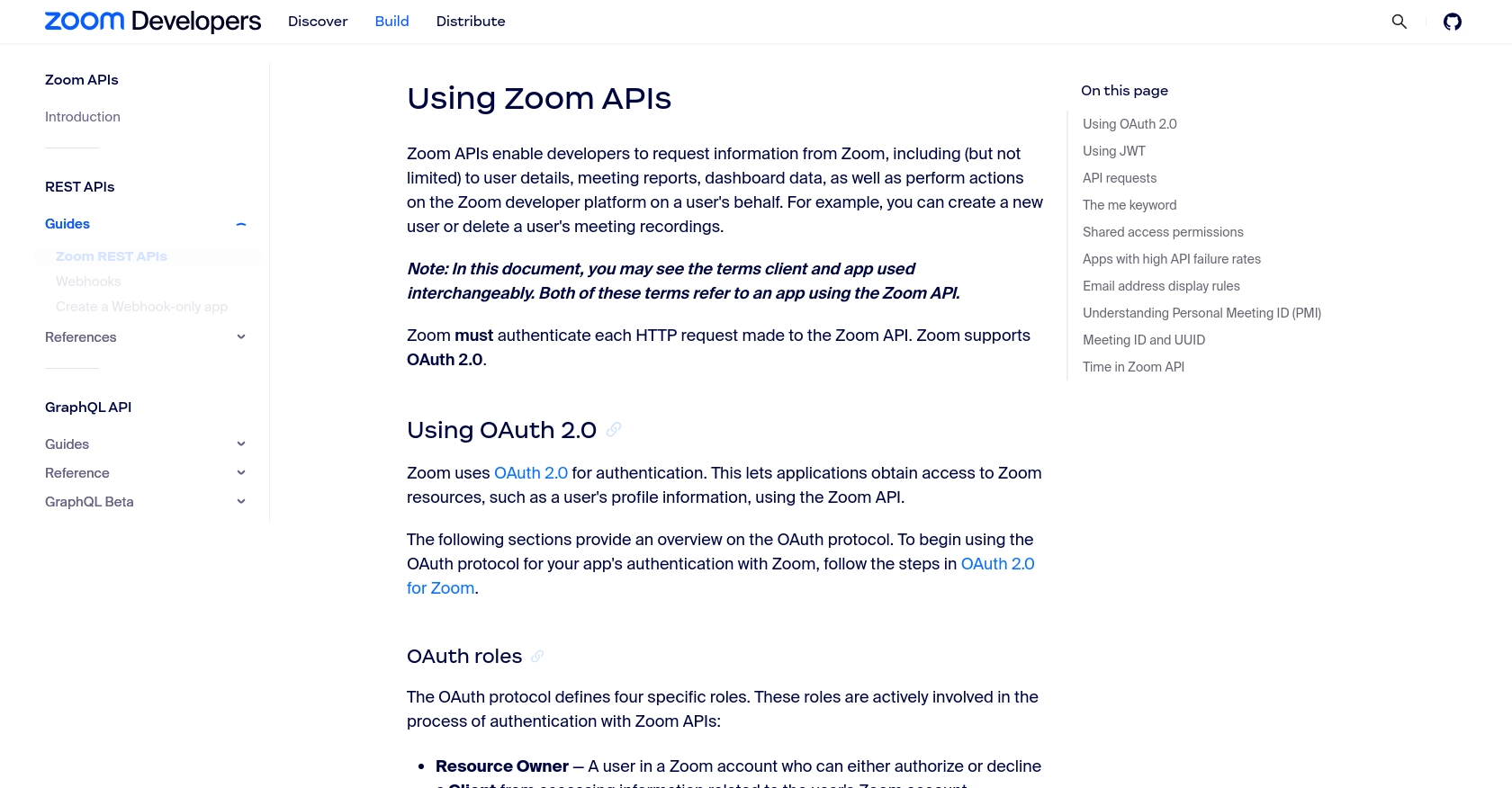
sbb-itb-96038d7
Making API Calls to Retrieve Zoom Meetings Using PHP
Now that you have set up your Zoom OAuth app and obtained the necessary access tokens, you can proceed to make API calls to retrieve meeting information. This section will guide you through the process of using PHP to interact with the Zoom API and fetch meeting details.
Prerequisites for Zoom API Integration in PHP
Before making API calls, ensure you have the following prerequisites set up on your development environment:
- PHP 7.4 or higher
- cURL extension enabled in PHP
Installing Required PHP Dependencies
To interact with the Zoom API, you will use PHP's cURL library. Ensure that the cURL extension is enabled in your php.ini
file. You can verify this by running the following command:
php -m | grep curl
If cURL is not listed, enable it by uncommenting the line extension=curl
in your php.ini
file and restarting your web server.
Fetching Zoom Meetings with PHP
To retrieve meetings, you will make a GET request to the Zoom API endpoint. Below is an example of how to fetch meetings using PHP:
// Set the API endpoint and access token
$endpoint = 'https://api.zoom.us/v2/users/me/meetings';
$access_token = 'YOUR_ACCESS_TOKEN';
// Initialize cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Bearer ' . $access_token,
'Content-Type: application/json'
]);
// Execute cURL request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error:' . curl_error($ch);
} else {
// Parse and display the response
$meetings = json_decode($response, true);
foreach ($meetings['meetings'] as $meeting) {
echo 'Meeting ID: ' . $meeting['id'] . ' - Topic: ' . $meeting['topic'] . '<br>';
}
}
// Close cURL session
curl_close($ch);
Handling API Response and Errors
After executing the API call, you should verify the response to ensure the request was successful. The Zoom API uses HTTP status codes to indicate success or failure:
- 2XX: Successful request
- 4XX: Client error (e.g., 401 Unauthorized, 403 Forbidden)
- 5XX: Server error
For detailed error handling, refer to the Zoom API Error Definitions.
Verifying API Call Success in Zoom Dashboard
To confirm that your API call was successful, you can log in to your Zoom account and navigate to the Meetings section. The meetings retrieved by your API call should match those listed in your Zoom dashboard.
Handling Rate Limits and Best Practices
Zoom API enforces rate limits to ensure fair usage. For more information on rate limits, visit the Zoom API Rate Limits page. Implement retry mechanisms and error handling to manage rate limit errors gracefully.
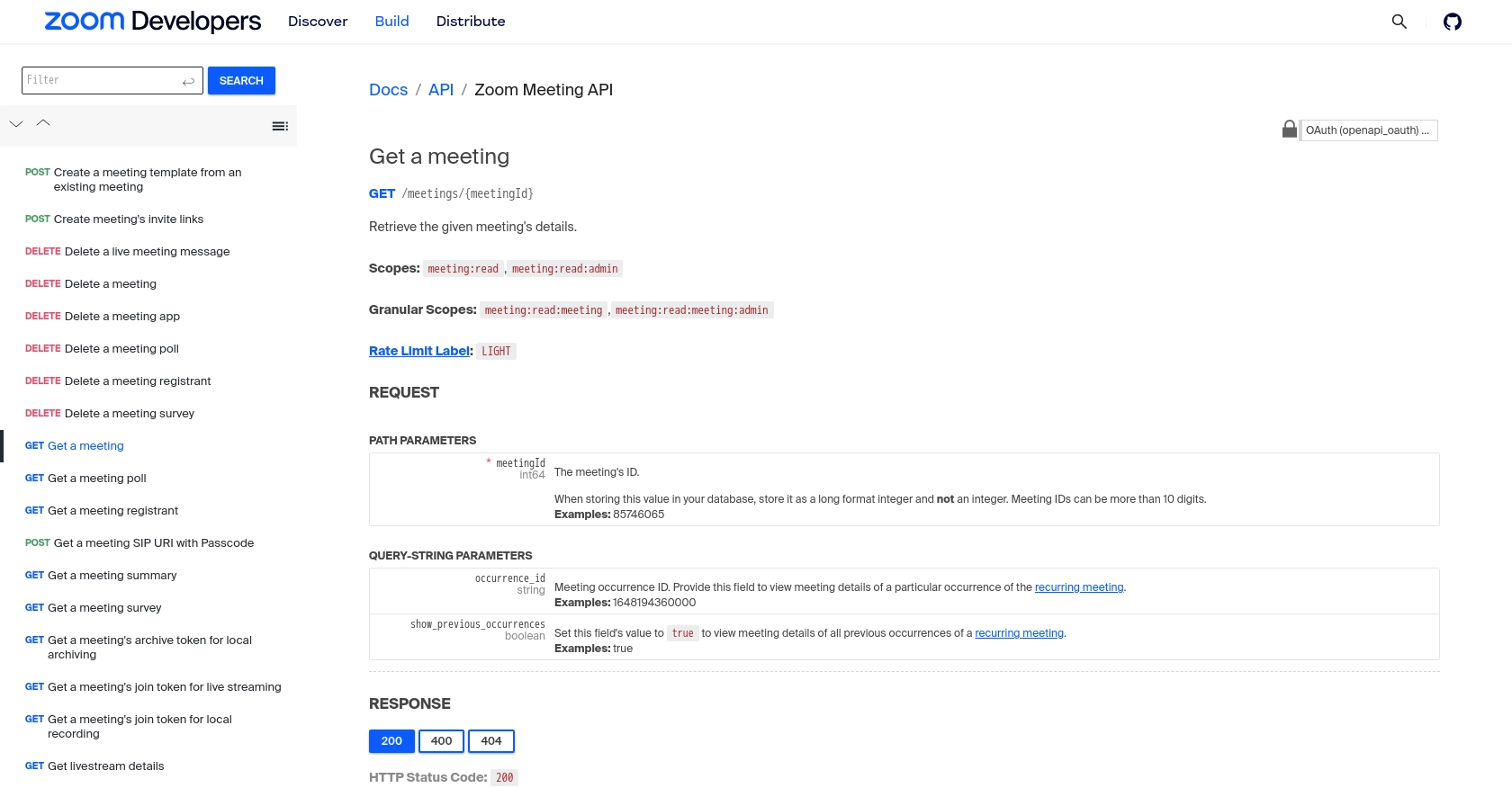
Conclusion and Best Practices for Zoom API Integration in PHP
Integrating with the Zoom API using PHP allows developers to enhance their applications with robust video conferencing features. By following the steps outlined in this guide, you can efficiently retrieve meeting data and integrate it into your B2B SaaS products, providing users with seamless access to their Zoom meetings.
Best Practices for Secure and Efficient Zoom API Usage
- Secure Storage of Credentials: Always store your access tokens and client credentials securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limits Gracefully: Implement retry mechanisms and exponential backoff strategies to handle rate limit errors. Refer to the Zoom API Rate Limits documentation for more details.
- Data Transformation and Standardization: Ensure that the data retrieved from the Zoom API is transformed and standardized to fit your application's data model, enhancing data consistency and usability.
Leverage Endgrate for Streamlined Integration Solutions
For developers seeking to simplify and accelerate their integration processes, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product development. Endgrate provides a unified API endpoint that connects to multiple platforms, including Zoom, offering an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration capabilities by visiting Endgrate today.
Read More
- https://endgrate.com/provider/zoom
- https://developers.zoom.us/docs/api/rest/using-zoom-apis/
- https://developers.zoom.us/docs/api/rest/pagination/
- https://developers.zoom.us/docs/api/rest/error-definitions/
- https://developers.zoom.us/docs/api/rest/rate-limits/
- https://developers.zoom.us/docs/api/rest/reference/zoom-api/methods/#operation/meeting
- https://developers.zoom.us/docs/api/rest/reference/zoom-api/methods/#operation/pastMeetingParticipants
- https://developers.zoom.us/docs/api/rest/reference/zoom-api/methods/#operation/recordingsList
Ready to get started?