How to Get Records with the Notion API in Python
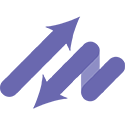
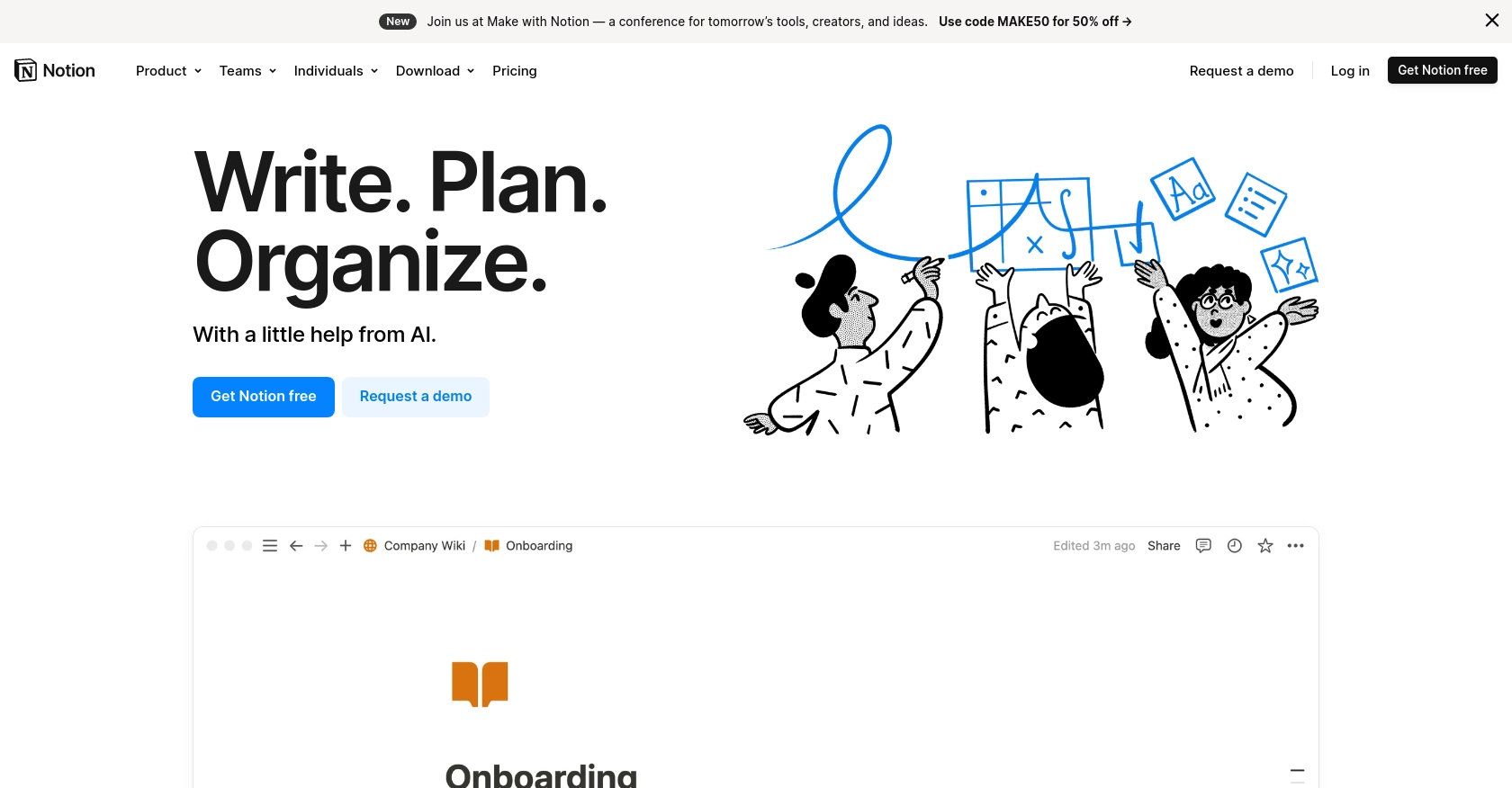
Introduction to Notion API Integration
Notion is a versatile all-in-one workspace that allows teams to organize tasks, manage projects, and store information in a highly customizable environment. Its flexibility and user-friendly interface make it a popular choice for businesses and individuals looking to streamline their workflows.
Integrating with the Notion API enables developers to programmatically interact with Notion workspaces, allowing for automation and enhanced functionality. For example, a developer might use the Notion API to retrieve records from a database and display them in a custom dashboard, providing real-time insights and analytics.
This article will guide you through the process of using Python to interact with the Notion API, specifically focusing on retrieving records from a Notion database. By the end of this tutorial, you'll have a clear understanding of how to set up your environment, authenticate with the Notion API, and execute API calls to fetch data efficiently.
Setting Up Your Notion Test/Sandbox Account for API Integration
Before diving into the Notion API, you need to set up a test or sandbox account to safely experiment with API calls. Notion offers a flexible environment where you can create a personal workspace for free, which is ideal for testing purposes.
Creating a Notion Account
If you don't already have a Notion account, follow these steps to create one:
- Visit the Notion signup page.
- Enter your email address and follow the instructions to create your account.
- Once your account is set up, you'll have access to a personal workspace where you can create pages and databases.
Setting Up a Notion Integration for API Access
To interact with the Notion API, you'll need to create an integration and obtain an integration token. This token will allow your application to authenticate API requests.
- Navigate to the My Integrations page in Notion.
- Click on New Integration and fill out the required information, such as the integration name and associated workspace.
- Once created, you'll find your integration token in the integration's settings. Keep this token secure, as it will be used to authenticate your API requests.
Sharing a Database with Your Integration
For your integration to access a Notion database, you must share the database with the integration:
- Open the database page in your Notion workspace.
- Click on the ••• menu at the top right corner of the page.
- Select Add connections and search for your integration in the dropdown list.
- Once selected, your integration will have access to the database, allowing you to make API calls to retrieve records.
OAuth Authentication for Notion API
The Notion API uses OAuth 2.0 for authentication. Here’s a brief overview of the steps involved:
- Direct users to the integration’s authorization URL to grant access to their Notion workspace.
- After authorization, Notion redirects users to your specified redirect URI with a temporary authorization code.
- Exchange this code for an access token by making a POST request to the Notion API.
- Use the access token to authenticate subsequent API requests.
For detailed instructions on OAuth setup, refer to the Notion API Authorization Guide.
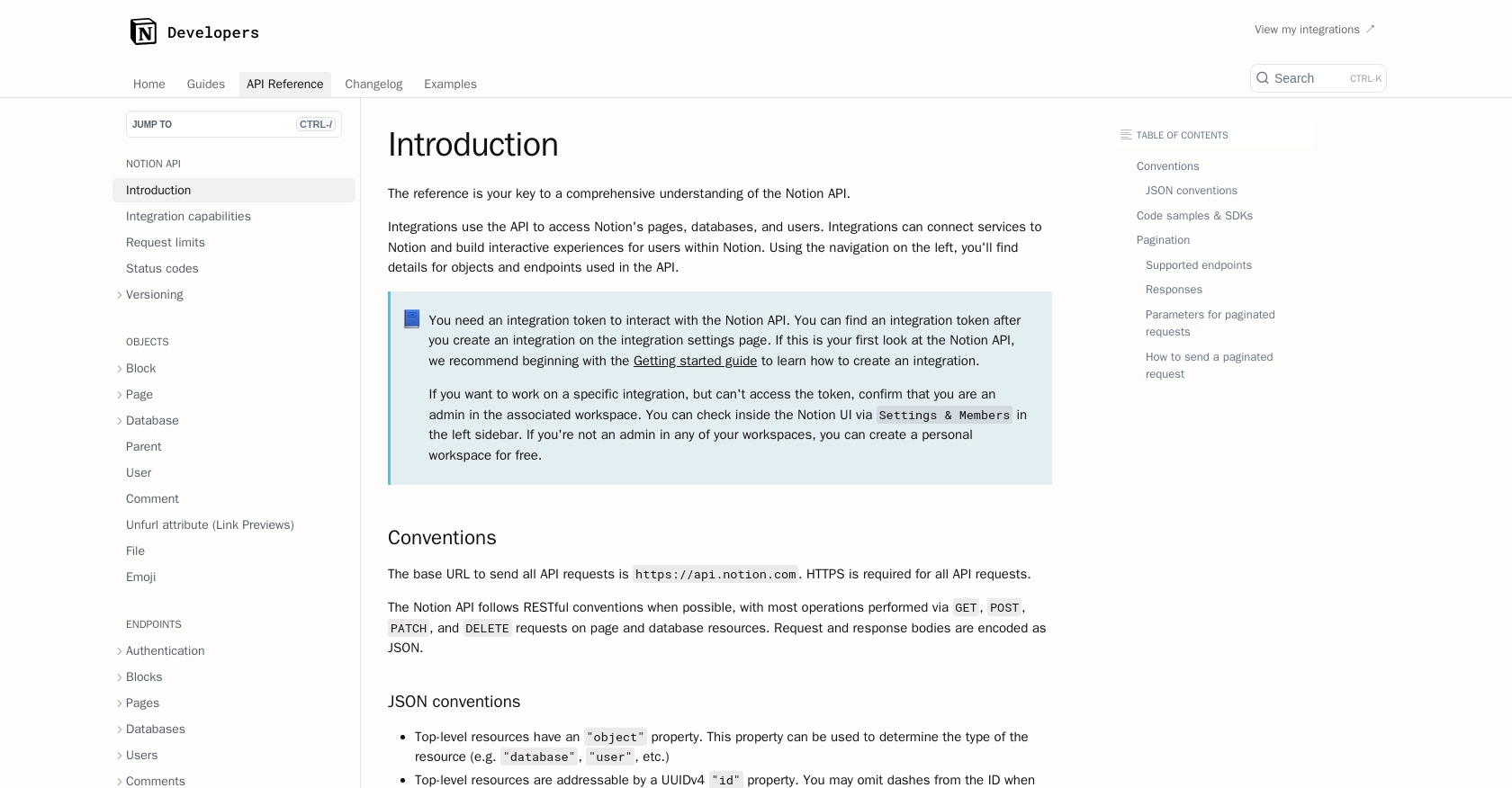
sbb-itb-96038d7
Setting Up Your Python Environment for Notion API Integration
Before making API calls to the Notion API using Python, you need to ensure that your development environment is properly configured. This involves installing the necessary Python version and dependencies.
Python Version and Dependencies
To interact with the Notion API, you'll need Python 3.11.1 or later. Additionally, you'll need the requests
library to handle HTTP requests.
- Ensure Python 3.11.1 is installed on your machine. You can download it from the official Python website.
- Install the
requests
library by running the following command in your terminal:
pip install requests
Executing API Calls to Retrieve Notion Database Records
With your environment set up, you can now proceed to make API calls to retrieve records from a Notion database. This involves constructing the appropriate HTTP request and handling the response.
Constructing the API Request
To query a Notion database, you'll need to make a POST request to the Notion API's database query endpoint. Here's a step-by-step guide:
- Define the API endpoint URL using your database ID:
endpoint = "https://api.notion.com/v1/databases/{database_id}/query"
- Set up the request headers, including the authorization token and content type:
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Content-Type": "application/json",
"Notion-Version": "2022-06-28"
}
Making the API Call
With the endpoint and headers defined, you can now make the API call to retrieve records:
import requests
# Make the POST request to query the database
response = requests.post(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
for page in data["results"]:
print(page)
else:
print(f"Failed to retrieve records: {response.status_code} - {response.json()}")
Handling API Response and Errors
Upon making the API call, you should verify the response to ensure the request was successful. The Notion API returns various status codes that indicate the outcome of the request:
- 200: Request was successful, and records are returned.
- 400: Invalid request, possibly due to malformed JSON or incorrect parameters.
- 401: Unauthorized access, check your access token.
- 429: Rate limit exceeded, wait and retry the request.
For more details on error codes, refer to the Notion API Status Codes documentation.
Verifying Retrieved Data in Notion
After successfully retrieving records, you can verify the data by checking the corresponding database in your Notion workspace. Ensure the records match the data returned by the API call.
Handling Rate Limits
The Notion API enforces rate limits of three requests per second. If you encounter a rate limit error (HTTP 429), respect the Retry-After
header value before retrying. For more information, see the Notion API Request Limits documentation.
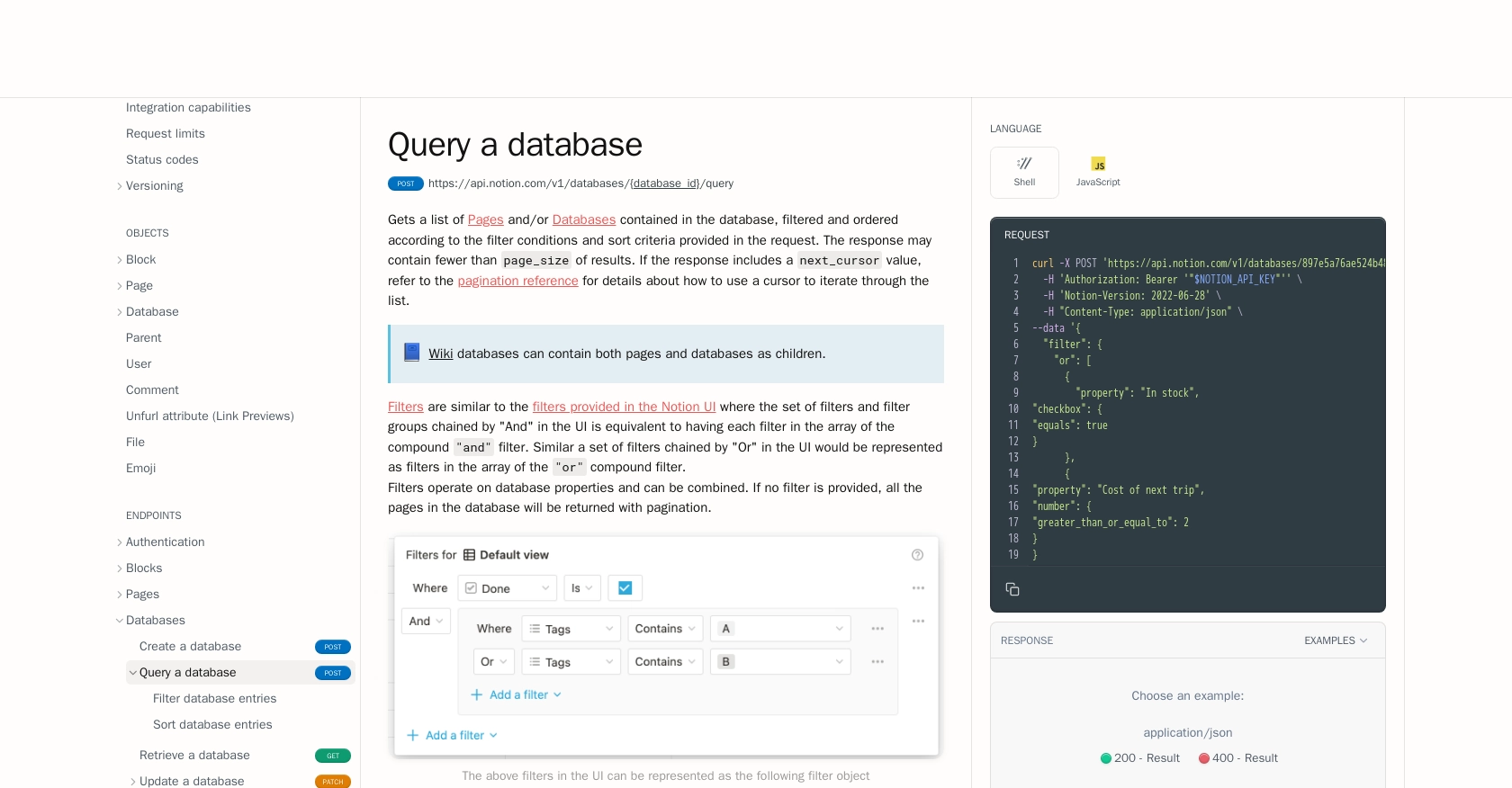
Best Practices for Using the Notion API in Python
When working with the Notion API, it's essential to follow best practices to ensure secure and efficient integration. Here are some key recommendations:
- Securely Store Access Tokens: Always keep your access tokens secure by storing them in environment variables or a secure vault. Avoid hardcoding them in your source code.
- Handle Rate Limits Gracefully: Implement logic to handle HTTP 429 errors by respecting the
Retry-After
header. This ensures your application remains compliant with Notion's rate limits. - Validate API Responses: Always check the status codes and response data to handle errors effectively. This helps in debugging and maintaining a robust integration.
- Optimize Data Handling: Consider transforming and standardizing data fields to match your application's requirements, ensuring seamless data integration.
Enhancing Integration Efficiency with Endgrate
Building and maintaining multiple integrations can be time-consuming and resource-intensive. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to various platforms, including Notion.
With Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction.
Explore how Endgrate can simplify your integration processes by visiting Endgrate.
Read More
- https://endgrate.com/provider/Notion
- https://developers.notion.com/reference/intro
- https://developers.notion.com/docs/getting-started
- https://developers.notion.com/docs/authorization
- https://developers.notion.com/reference/request-limits
- https://developers.notion.com/reference/status-codes
- https://developers.notion.com/reference/post-database-query
- https://developers.notion.com/reference/post-search
Ready to get started?