How to Create Records with the VTiger API in Python
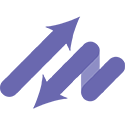
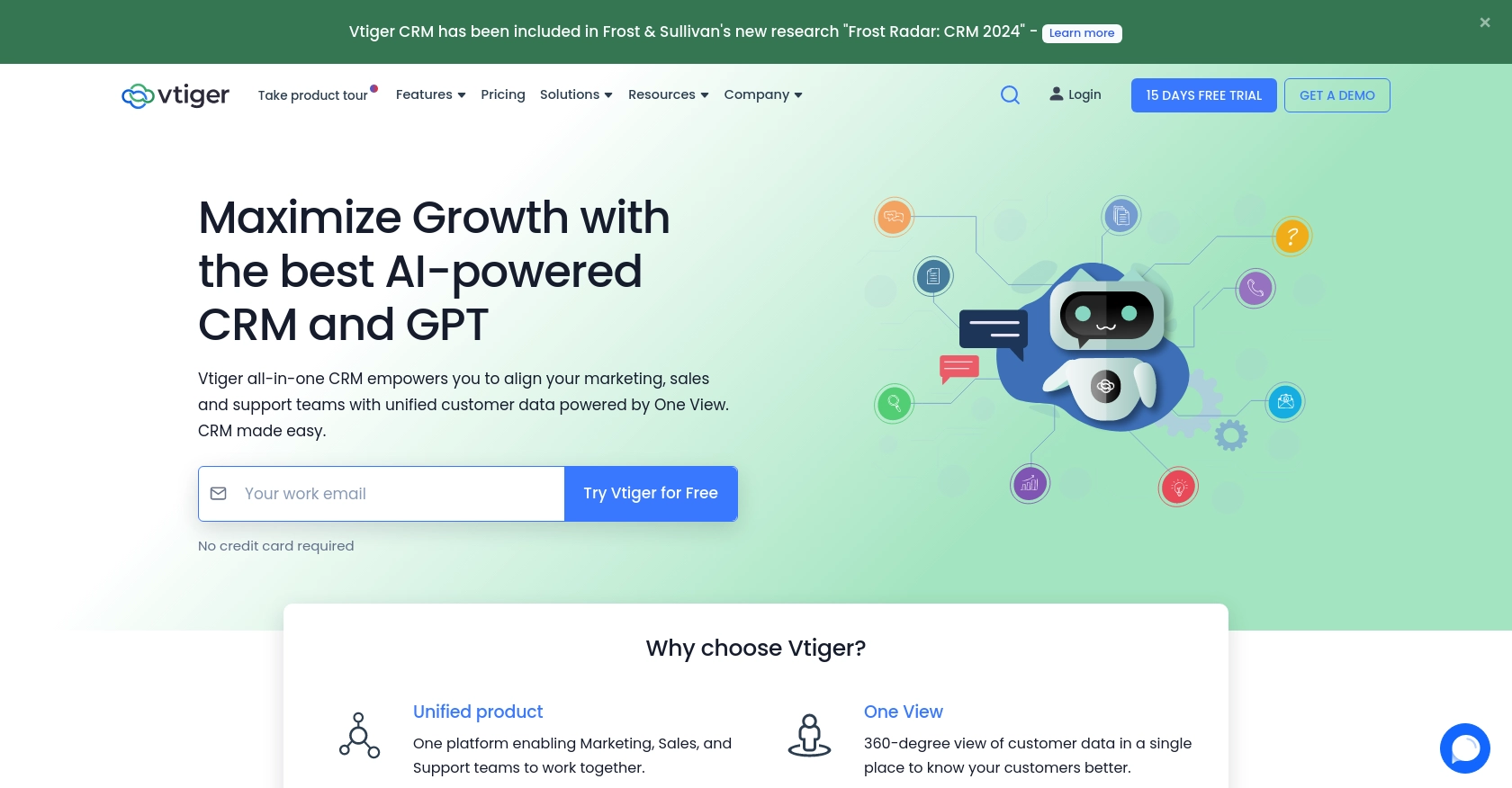
Introduction to VTiger CRM Integration
VTiger CRM is a robust customer relationship management platform that offers a wide array of tools to help businesses manage their sales, marketing, and support operations. Known for its flexibility and comprehensive features, VTiger is a popular choice among businesses looking to streamline their customer interactions and improve efficiency.
Integrating with VTiger's API allows developers to automate and enhance CRM functionalities, such as creating and managing records. For example, a developer might want to automate the creation of new customer records in VTiger when a user signs up on their website, ensuring that all customer data is centralized and easily accessible.
Setting Up Your VTiger CRM Test Account
Before you can start integrating with the VTiger API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data.
Creating a VTiger CRM Account
If you don't already have a VTiger account, you can sign up for a free trial or demo account on the VTiger website. This will give you access to the CRM features and the ability to test API integrations.
- Visit the VTiger website and navigate to the sign-up page.
- Follow the instructions to create your account. Once registered, log in to access your CRM dashboard.
Generating API Access Credentials
VTiger uses HTTP Basic Authentication, requiring a username and an access key. Follow these steps to obtain your credentials:
- Log in to your VTiger account and navigate to My Preferences.
- Locate the Access Key section. This key is a unique token generated for your user account.
- Note down your username and access key, as you'll need these for API authentication.
Configuring API Access in VTiger
To ensure your API calls are successful, you may need to configure certain settings within VTiger:
- Ensure that your user role has the necessary permissions to access and modify records via the API.
- Check the API limits based on your VTiger edition to avoid exceeding the allowed number of API calls per day.
With your VTiger account set up and credentials in hand, you're ready to start making API calls to create records. In the next section, we'll walk through the process of making these calls using Python.
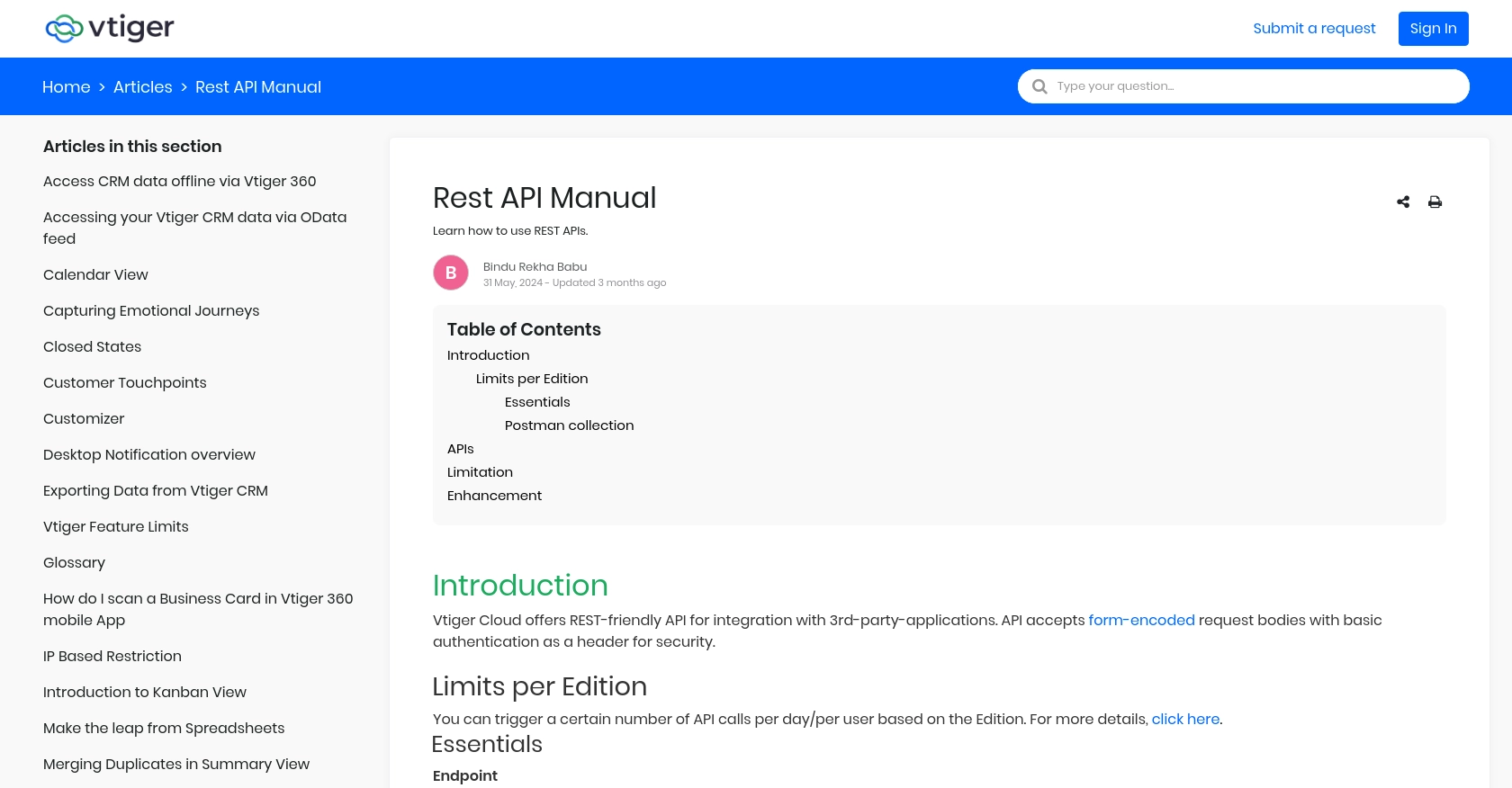
sbb-itb-96038d7
Making API Calls to Create Records in VTiger Using Python
To interact with the VTiger API and create records, you'll need to use Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your Python Environment for VTiger API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python 3.11.1 from the official Python website.
- Use pip to install the
requests
library by running the following command in your terminal:
pip install requests
Writing Python Code to Create Records in VTiger
Now that your environment is set up, you can write the Python code to create records in VTiger. The following example demonstrates how to create a contact record.
import requests
import json
# Define the VTiger API endpoint
url = "https://your_instance.odx.vtiger.com/restapi/v1/vtiger/default/create"
# Set your VTiger credentials
username = "your_username"
access_key = "your_access_key"
# Set the request headers
headers = {
"Content-Type": "application/json"
}
# Define the contact data to be created
data = {
"elementType": "Contacts",
"element": json.dumps({
"firstname": "John",
"lastname": "Doe",
"email": "johndoe@example.com",
"assigned_user_id": "19x1" # Example user ID
})
}
# Make the POST request to create the record
response = requests.post(url, headers=headers, auth=(username, access_key), json=data)
# Check if the request was successful
if response.status_code == 200:
print("Record created successfully:", response.json())
else:
print("Failed to create record:", response.status_code, response.text)
Replace your_instance
, your_username
, and your_access_key
with your actual VTiger instance details and credentials.
Verifying Record Creation in VTiger
After running the script, you should verify that the record was created successfully in your VTiger CRM. Log in to your VTiger account and navigate to the Contacts module to see the newly created contact.
Handling Errors and Common Issues with VTiger API
When making API calls, it's crucial to handle potential errors. The VTiger API returns specific HTTP status codes to indicate success or failure:
- 200 OK: The request was successful, and the record was created.
- 400 Bad Request: There was an error with the request, such as missing required fields.
- 500 Internal Server Error: The server encountered an error processing the request.
Always check the response status code and handle errors appropriately to ensure robust integration.
For more details on error codes and handling, refer to the VTiger API documentation.
Best Practices for VTiger API Integration
When integrating with the VTiger API, it's essential to follow best practices to ensure a secure and efficient implementation. Here are some key recommendations:
- Securely Store Credentials: Always store your VTiger username and access key securely. Avoid hardcoding them in your scripts. Use environment variables or secure vaults to manage sensitive information.
- Handle Rate Limiting: Be aware of the API call limits based on your VTiger edition. Implement logic to handle rate limiting gracefully, such as retry mechanisms with exponential backoff.
- Data Standardization: Ensure that data fields are standardized and validated before sending them to the API. This helps maintain data integrity and consistency across your CRM.
Streamlining VTiger Integrations with Endgrate
Building and maintaining multiple integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including VTiger.
With Endgrate, you can:
- Save Time and Resources: Focus on your core product while outsourcing integration complexities to Endgrate.
- Build Once, Deploy Everywhere: Create a single integration for each use case and apply it across multiple platforms effortlessly.
- Enhance User Experience: Offer your customers a seamless and intuitive integration experience.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?