Using the OnePageCRM API to Get Users in Javascript
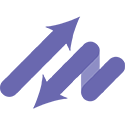
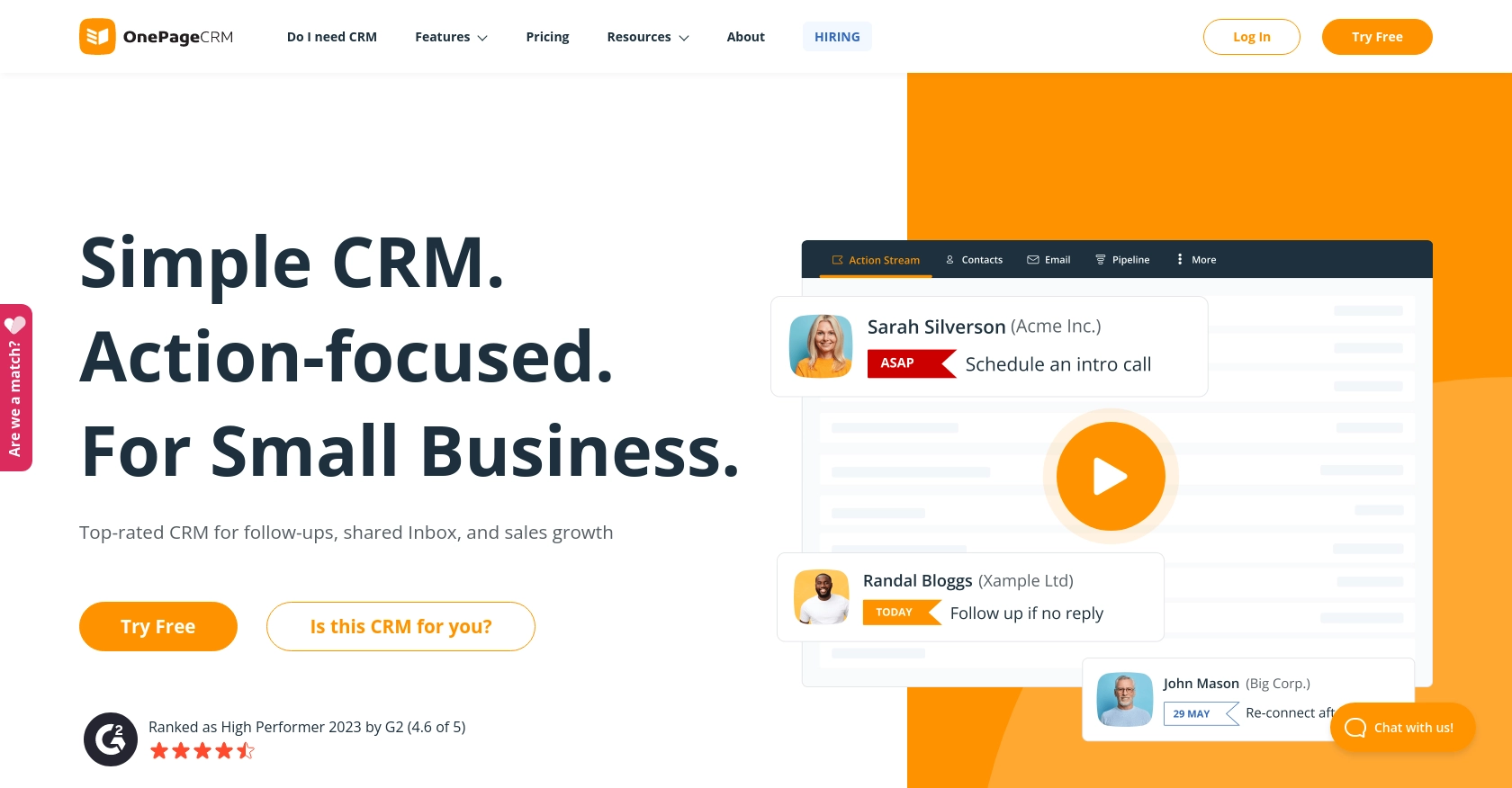
Introduction to OnePageCRM
OnePageCRM is a dynamic customer relationship management (CRM) tool designed to simplify sales processes for businesses. It offers a streamlined approach to managing contacts, tasks, and sales pipelines, making it an ideal choice for sales teams looking to enhance productivity and efficiency.
Integrating with OnePageCRM's API allows developers to access and manage user data seamlessly. For example, you might want to retrieve a list of users within your organization to synchronize with another system or to generate reports. This capability can be particularly useful for businesses aiming to maintain up-to-date user information across multiple platforms.
Setting Up Your OnePageCRM Test Account for API Integration
Before you can start using the OnePageCRM API to retrieve user data, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting your live data.
Creating a OnePageCRM Account
If you don't already have a OnePageCRM account, you can sign up for a free trial on their website. This trial will give you access to all the features you need to test the API.
- Visit the OnePageCRM website and click on the "Free Trial" button.
- Fill in the required information to create your account.
- Once your account is set up, log in to access the dashboard.
Generating API Credentials for OnePageCRM
OnePageCRM uses a custom authentication method to secure API requests. Follow these steps to generate the necessary credentials:
- Navigate to the "Settings" section in your OnePageCRM dashboard.
- Locate the "API & Integrations" tab and click on it.
- Under the API section, you will find options to generate your API key and secret.
- Copy these credentials and store them securely, as you will need them to authenticate your API requests.
Configuring Your Environment for API Testing
With your API credentials in hand, you can now configure your development environment to interact with the OnePageCRM API:
- Ensure you have a modern web browser and a code editor installed.
- Set up a local server if needed, or use an online code editor to test your JavaScript code.
- Keep your API key and secret accessible, as you will need to include them in your API requests.
With your OnePageCRM account and API credentials ready, you're all set to start making API calls to retrieve user data. In the next section, we'll explore how to use JavaScript to interact with the OnePageCRM API.
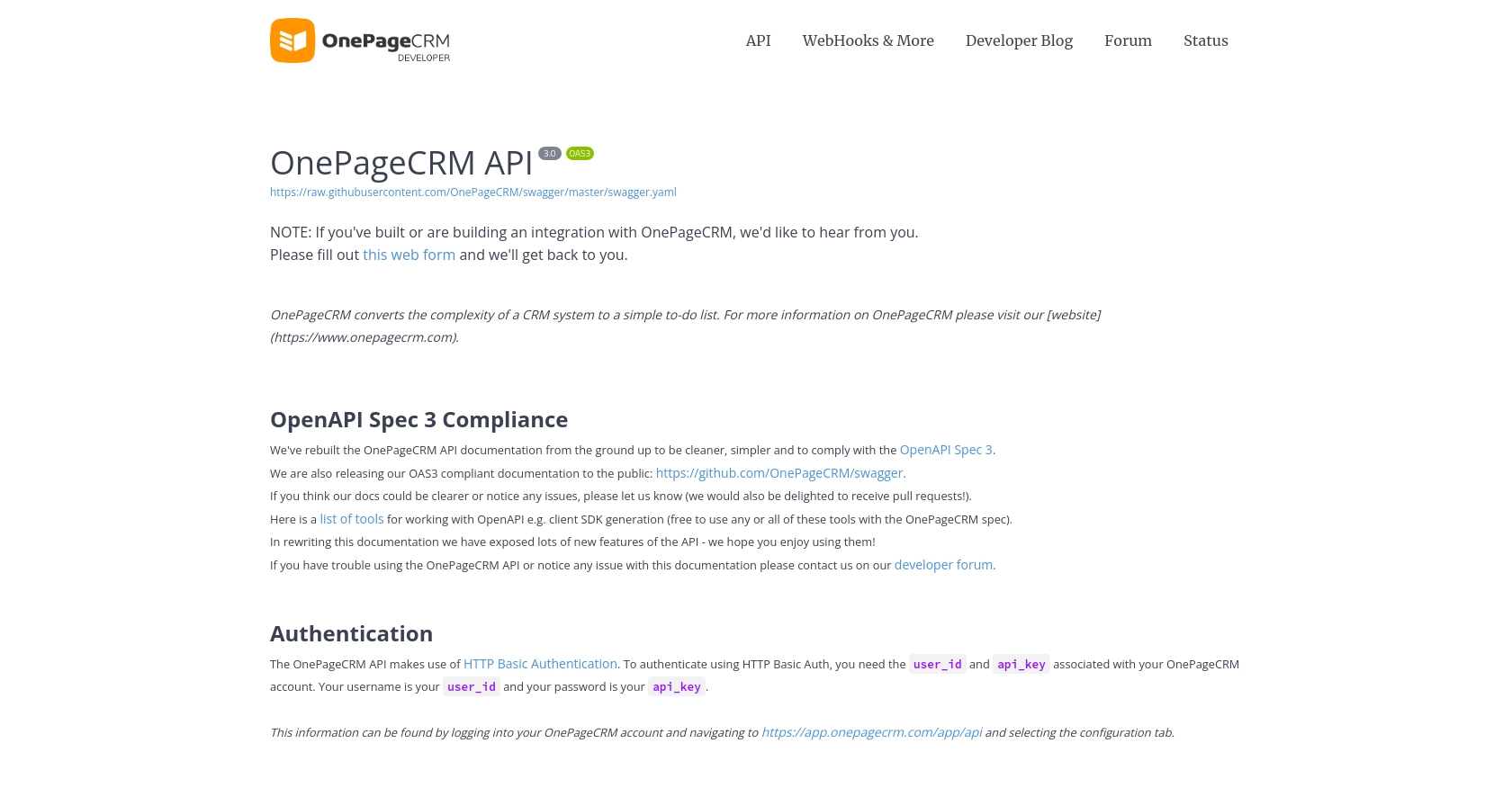
sbb-itb-96038d7
Making API Calls to Retrieve Users with OnePageCRM in JavaScript
Now that you have your OnePageCRM account and API credentials set up, it's time to dive into making API calls using JavaScript. This section will guide you through the process of retrieving user data from OnePageCRM, ensuring you have all the necessary tools and knowledge to interact with the API effectively.
Setting Up Your JavaScript Environment for OnePageCRM API
Before making API calls, ensure your JavaScript environment is ready:
- Ensure you have Node.js installed on your machine to run JavaScript code outside the browser.
- Use a code editor like Visual Studio Code to write and manage your scripts.
- Install the
axios
library, which simplifies making HTTP requests. You can install it using npm:
npm install axios
Writing JavaScript Code to Retrieve Users from OnePageCRM
With your environment set up, you can now write the JavaScript code to interact with the OnePageCRM API:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://app.onepagecrm.com/api/v3/users';
const headers = {
'Authorization': 'Bearer YOUR_API_KEY',
'Content-Type': 'application/json'
};
// Function to get users
async function getUsers() {
try {
const response = await axios.get(endpoint, { headers });
if (response.status === 200) {
console.log('Users retrieved successfully:', response.data.data);
}
} catch (error) {
console.error('Error fetching users:', error.response ? error.response.data : error.message);
}
}
// Call the function
getUsers();
Replace YOUR_API_KEY
with the API key you generated earlier. This script uses the axios
library to send a GET request to the OnePageCRM API endpoint for users. If successful, it logs the user data to the console.
Verifying API Call Success and Handling Errors
After running the script, you should see the list of users printed in the console. To verify the success of your API call:
- Check the console output for the user data.
- Ensure the response status is
200 OK
, indicating a successful request.
Handle potential errors by checking the error response. Common error codes include:
400 Bad Request
: Indicates a generic error in the request.401 Unauthorized
: Authentication data is missing or incorrect.403 Forbidden
: Access to the resource is denied.404 Resource Not Found
: The requested resource does not exist.500 Internal Server Error
: A server-side error occurred.
For more detailed error handling, refer to the OnePageCRM API documentation.
Conclusion and Best Practices for Using OnePageCRM API in JavaScript
Integrating with the OnePageCRM API using JavaScript provides a powerful way to manage user data and enhance your CRM capabilities. By following the steps outlined in this article, you can efficiently retrieve user information and synchronize it with other systems or generate insightful reports.
Best Practices for Secure and Efficient API Integration
- Securely Store API Credentials: Always store your API key and secret securely. Avoid hardcoding them in your scripts and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement exponential backoff strategies to handle rate limit errors gracefully.
- Standardize Data Formats: Ensure that the data retrieved from OnePageCRM is transformed and standardized to match your application's data structures for seamless integration.
- Error Handling: Implement robust error handling to manage different HTTP status codes and provide meaningful feedback to users or logs for debugging.
Streamlining Integrations with Endgrate
While integrating with OnePageCRM is straightforward, managing multiple integrations can become complex. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including OnePageCRM. By using Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate and discover how it can streamline your development process, providing an intuitive and efficient integration experience for your customers.
Read More
Ready to get started?