Using the Insightly API to Get Organizations (with Javascript examples)
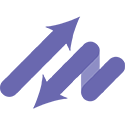
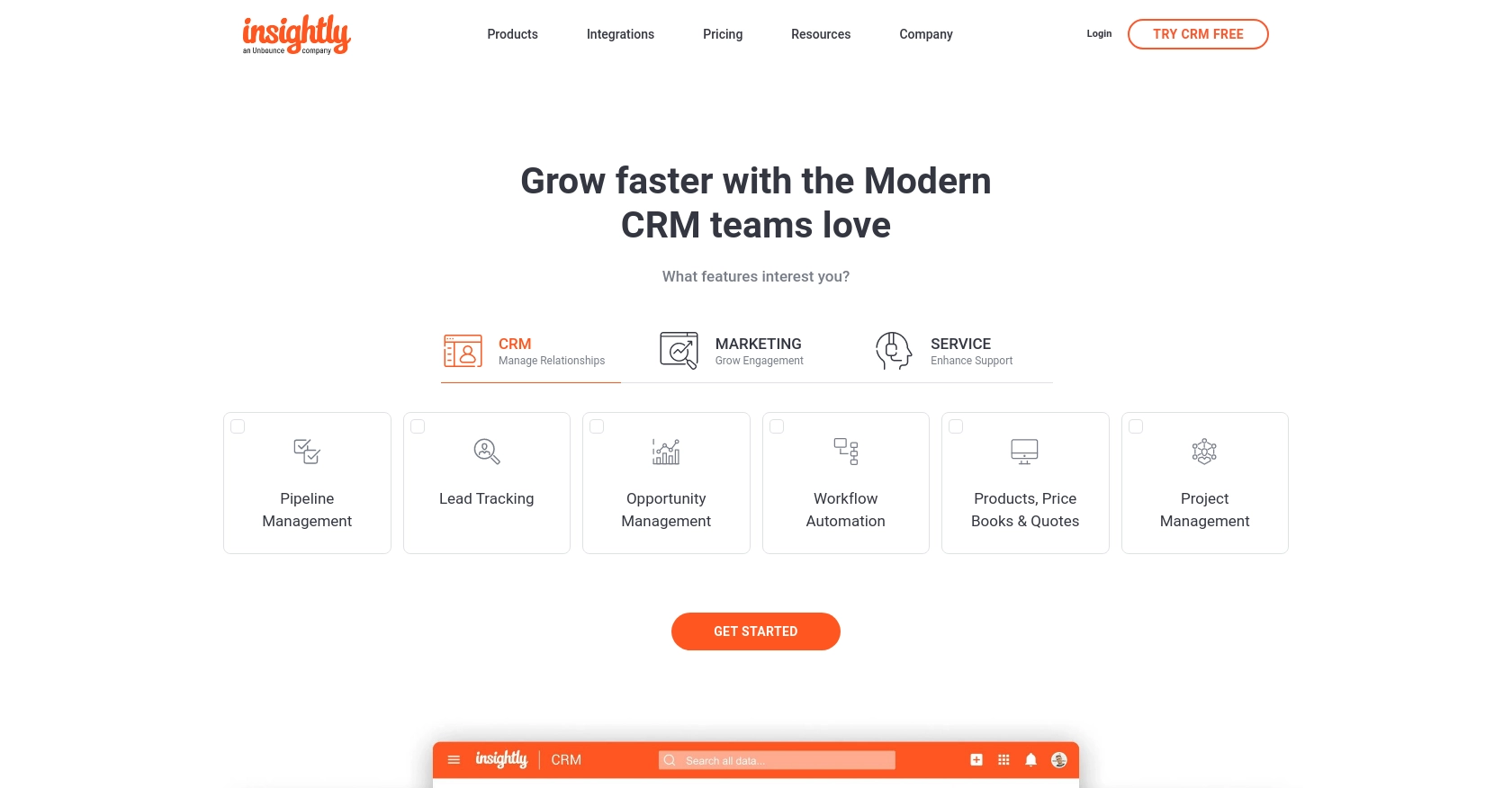
Introduction to Insightly CRM and API Integration
Insightly is a powerful CRM platform that helps businesses manage customer relationships, streamline workflows, and enhance productivity. With features like project management, contact tracking, and sales automation, Insightly is a popular choice for organizations looking to improve their customer engagement strategies.
Developers may want to integrate with Insightly's API to access and manipulate organizational data, enabling seamless data synchronization and automation of business processes. For example, using the Insightly API, a developer could retrieve a list of organizations to analyze customer demographics and tailor marketing strategies accordingly.
Setting Up Your Insightly API Test Account
Before you can start interacting with the Insightly API, you need to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Insightly offers a straightforward process to get started with their API using an API key for authentication.
Creating an Insightly Account
If you don't already have an Insightly account, you can sign up for a free trial on their website. This will give you access to the necessary features to test API interactions.
- Visit the Insightly website and click on the "Free Trial" button.
- Fill in the required information and follow the prompts to create your account.
- Once your account is set up, log in to access the Insightly dashboard.
Finding Your Insightly API Key
Insightly uses HTTP Basic authentication, which requires an API key. This key identifies the user making API calls and determines their permissions.
- Navigate to your user profile by clicking on your avatar in the upper right corner of the Insightly dashboard.
- Select "User Settings" from the dropdown menu.
- In the "API Key" section, you will find your API key. Copy this key as you will need it for authentication in your API requests.
For more detailed instructions, refer to the Insightly support article.
Configuring API Key Authentication
To authenticate your API requests, you will use the API key as a Base64-encoded username, leaving the password blank. This ensures that your API calls are securely associated with your Insightly account.
For testing purposes, you can directly use your API key without Base64 encoding when using tools like Postman or the Insightly API sandbox.
Testing Your Insightly API Setup
Once you have your API key, you can start testing API calls. Use tools like Postman to make GET requests to the Insightly API and verify that you can successfully retrieve data.
Ensure that you are familiar with the API endpoints and response schemas by reviewing the Insightly API documentation.
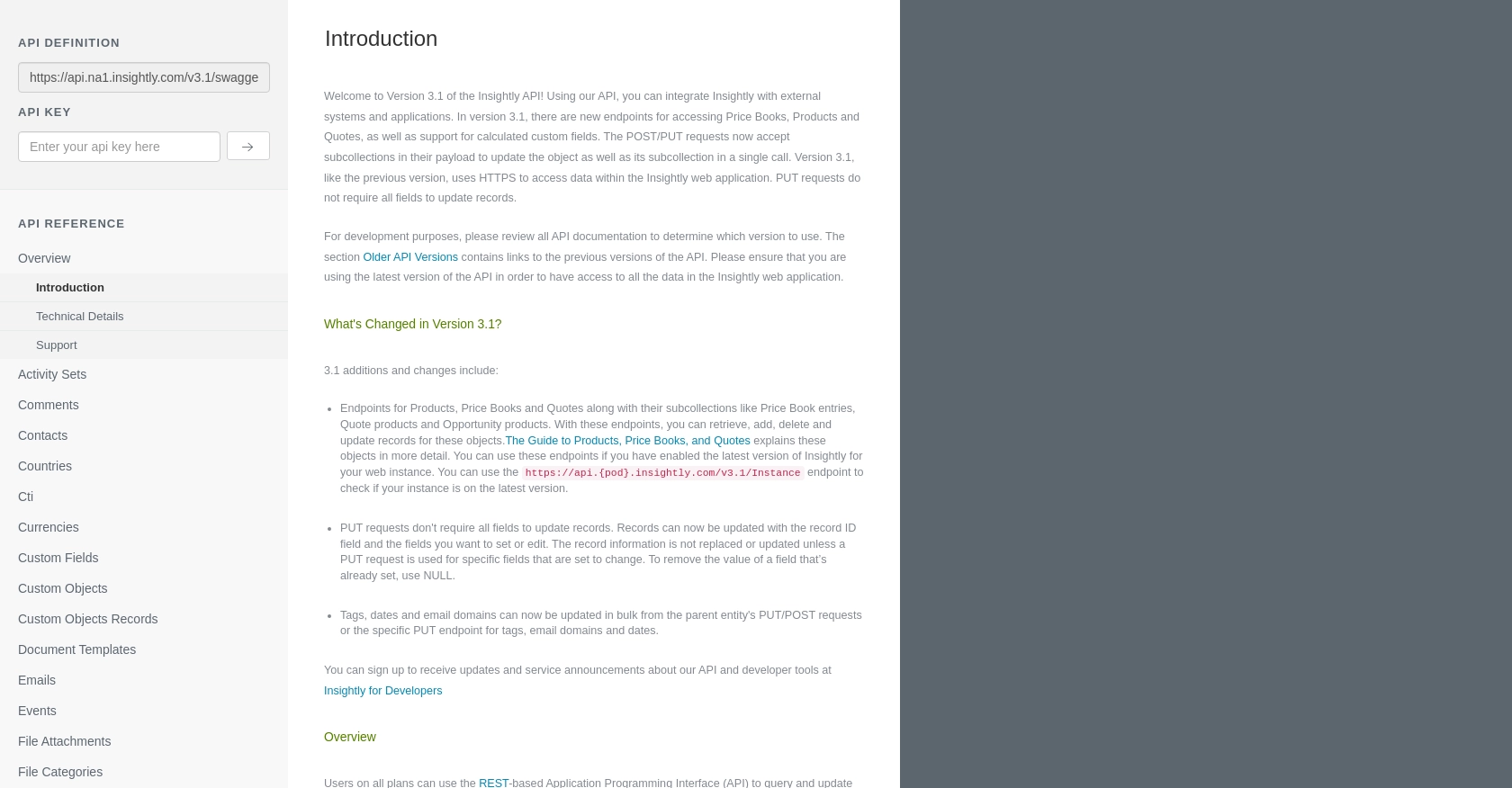
sbb-itb-96038d7
Making API Calls to Retrieve Organizations from Insightly Using JavaScript
To interact with the Insightly API and retrieve a list of organizations, you'll need to use JavaScript to make HTTP requests. This section will guide you through the process of setting up your environment, writing the code, and handling responses effectively.
Setting Up Your JavaScript Environment for Insightly API Integration
Before making API calls, ensure you have a working JavaScript environment. You can use Node.js or a browser-based environment for this purpose. Additionally, you'll need the axios
library to simplify HTTP requests.
- Ensure you have Node.js installed on your machine. You can download it from the official Node.js website.
- Initialize a new Node.js project and install
axios
by running the following command in your terminal:
npm install axios
Writing JavaScript Code to Retrieve Organizations from Insightly
With your environment set up, you can now write the JavaScript code to make an API call to Insightly and retrieve organizations. Below is a sample code snippet to get you started:
const axios = require('axios');
// Your Insightly API key
const apiKey = 'Your_API_Key';
// Base64 encode the API key
const encodedApiKey = Buffer.from(apiKey + ':').toString('base64');
// Set up the API endpoint and headers
const endpoint = 'https://api.na1.insightly.com/v3.1/Organisations';
const headers = {
'Authorization': `Basic ${encodedApiKey}`,
'Accept-Encoding': 'gzip'
};
// Make a GET request to the Insightly API
axios.get(endpoint, { headers })
.then(response => {
// Handle the successful response
console.log('Organizations:', response.data);
})
.catch(error => {
// Handle errors
if (error.response) {
console.error('Error:', error.response.status, error.response.data);
} else {
console.error('Error:', error.message);
}
});
Replace Your_API_Key
with your actual Insightly API key. This code sets up the necessary headers for authentication and makes a GET request to the organizations endpoint.
Understanding and Handling API Responses from Insightly
After executing the code, you should receive a response containing the list of organizations. The response data will be logged to the console. Ensure that the API call is successful by checking the response status code.
If the request fails, the error handling block will log the error details, including the status code and error message. Refer to the Insightly API documentation for more information on error codes and troubleshooting tips.
Verifying API Call Success in Insightly Sandbox
To verify that your API call is successful, you can cross-check the retrieved data with the organizations listed in your Insightly sandbox account. This ensures that the data synchronization is accurate and complete.
By following these steps, you can efficiently retrieve and manage organizational data from Insightly using JavaScript, streamlining your business processes and enhancing data-driven decision-making.
Conclusion and Best Practices for Insightly API Integration
Integrating with the Insightly API using JavaScript allows developers to efficiently manage organizational data, enhancing business processes and decision-making capabilities. By following the steps outlined in this article, you can seamlessly retrieve and handle data from Insightly, ensuring that your applications are both robust and scalable.
Best Practices for Secure and Efficient Insightly API Usage
- Secure Storage of API Keys: Always store your API keys securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of Insightly's rate limits, which allow up to 10 requests per second and vary daily based on your plan. Implement retry logic to handle HTTP 429 errors gracefully. For more details, refer to the Insightly API documentation.
- Data Standardization: Ensure that data retrieved from Insightly is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement comprehensive error handling to manage different HTTP status codes and provide meaningful feedback to users.
Streamlining Integrations with Endgrate
While integrating with Insightly directly offers flexibility, using a tool like Endgrate can significantly simplify the process. Endgrate provides a unified API endpoint for multiple platforms, allowing you to focus on your core product while outsourcing complex integrations. This approach not only saves time and resources but also enhances the integration experience for your customers.
Explore how Endgrate can streamline your integration processes by visiting Endgrate and discover how companies like yours have successfully scaled their integrations.
Read More
Ready to get started?