Using the Capsule API to Get Parties in Python
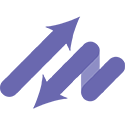
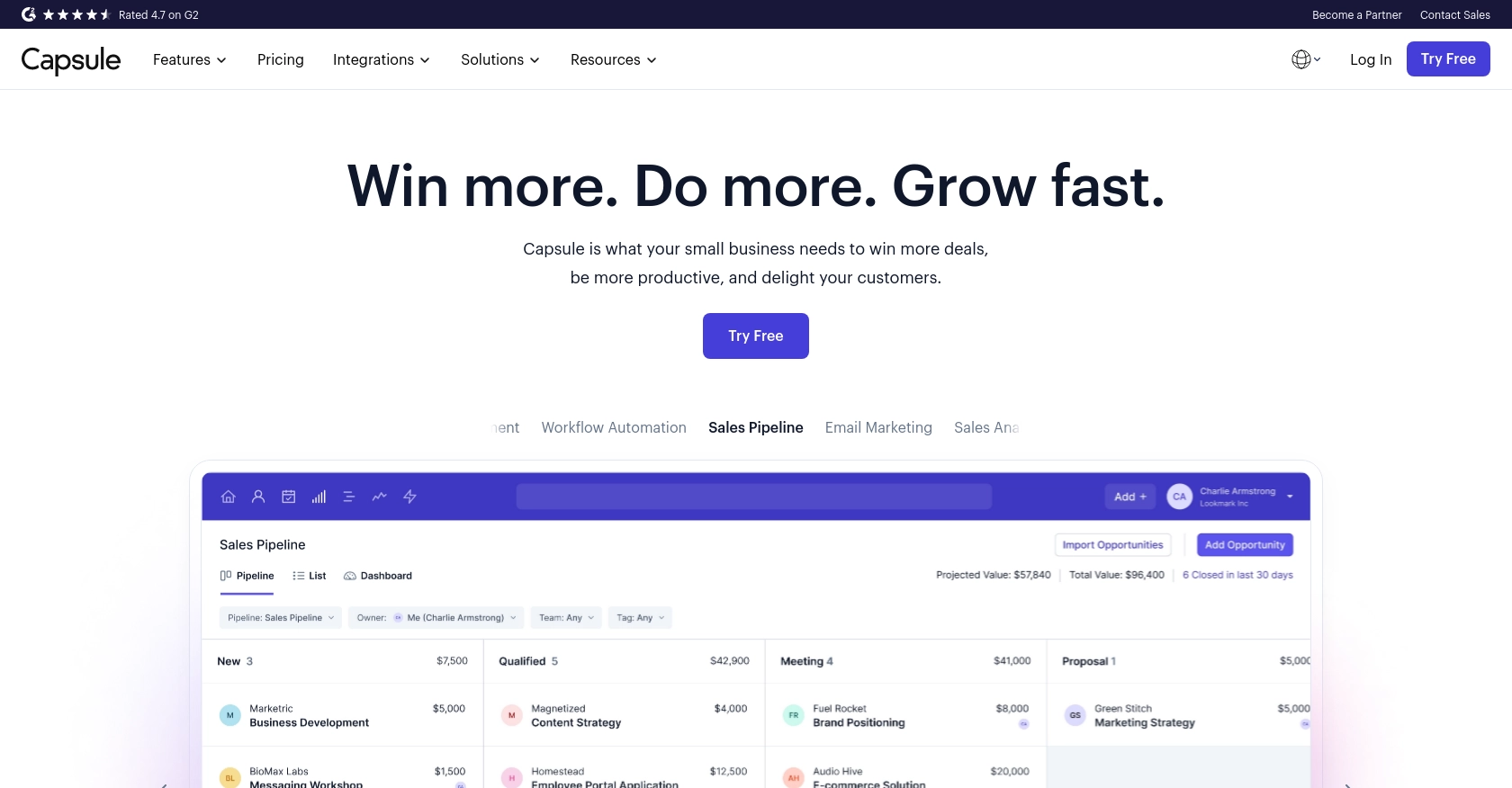
Introduction to Capsule CRM API
Capsule CRM is a versatile customer relationship management platform that helps businesses manage their contacts, track sales, and nurture customer relationships. Known for its simplicity and powerful features, Capsule CRM is a popular choice for businesses looking to streamline their CRM processes.
Developers may want to integrate with Capsule CRM to access and manage data related to people and organizations, known as "parties" in Capsule. For example, a developer might use the Capsule API to retrieve a list of parties and synchronize this data with another application, ensuring that customer information is always up-to-date across platforms.
This article will guide you through using Python to interact with the Capsule API, specifically focusing on retrieving party data. By the end of this tutorial, you'll be able to efficiently access and manage parties within Capsule CRM using Python.
Setting Up Your Capsule CRM Test Account
Before diving into the Capsule API, you'll need to set up a test account to experiment with API calls without affecting live data. Capsule CRM offers a straightforward process to get started with their API using OAuth 2.0 authentication.
Creating a Capsule CRM Account
If you don't already have a Capsule CRM account, you can sign up for a free trial on their website. This will give you access to the necessary features to test the API integration.
- Visit the Capsule CRM signup page.
- Fill in the required details and create your account.
- Once registered, log in to your Capsule CRM dashboard.
Registering Your Application for OAuth 2.0
To interact with the Capsule API, you need to register your application to obtain the client ID and client secret required for OAuth 2.0 authentication.
- Navigate to the My Preferences section in your Capsule account.
- Select API Authentication Tokens to create a new application.
- Provide a name and description for your application.
- Set the Application Type to "web" or "installed" based on your use case.
- Note down the Client ID and Client Secret generated for your application.
Obtaining an OAuth 2.0 Bearer Token
With your application registered, you can now obtain a bearer token to authenticate API requests.
- Redirect users to the Capsule authorization URL to grant access to your application:
- After authorization, Capsule will redirect to your specified URL with a temporary code.
- Exchange this code for an access token by making a POST request:
- Store the received access token securely, as it will be used in the Authorization header for API requests.
GET https://api.capsulecrm.com/oauth/authorise?response_type=code&client_id=YOUR_CLIENT_ID&redirect_uri=YOUR_REDIRECT_URI&scope=read%20write
POST https://api.capsulecrm.com/oauth/token
{
"code": "YOUR_AUTHORIZATION_CODE",
"client_id": "YOUR_CLIENT_ID",
"client_secret": "YOUR_CLIENT_SECRET",
"grant_type": "authorization_code"
}
For more detailed information on authentication, refer to the Capsule API Authentication documentation.
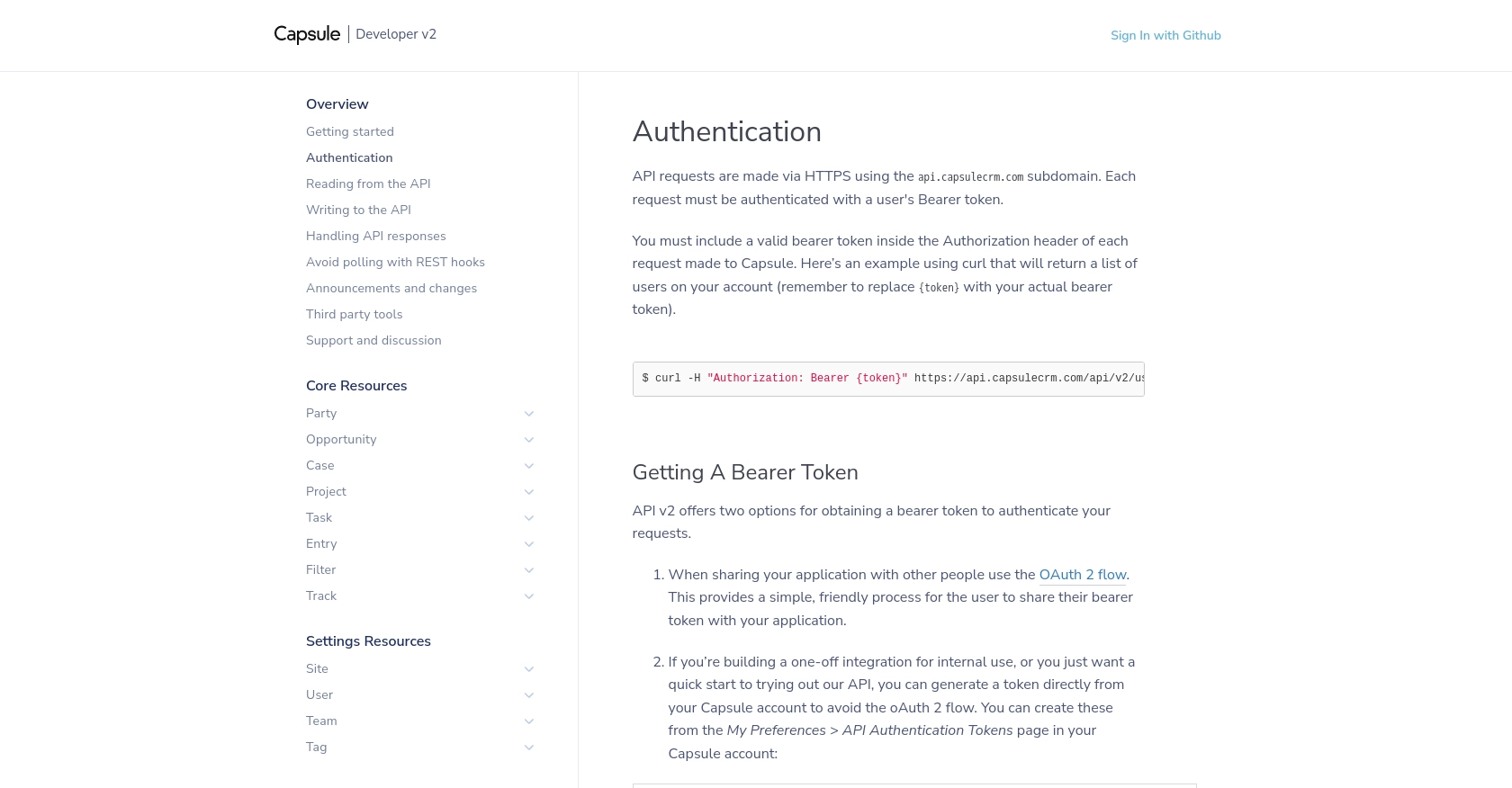
sbb-itb-96038d7
Making API Calls to Retrieve Parties from Capsule CRM Using Python
Now that you have set up your Capsule CRM account and obtained the necessary OAuth 2.0 credentials, it's time to make API calls to retrieve party data. This section will guide you through the process of using Python to interact with the Capsule API and fetch information about parties.
Setting Up Your Python Environment for Capsule API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using the following command:
pip install requests
Writing Python Code to Fetch Parties from Capsule CRM
With your environment ready, you can now write the Python code to retrieve parties from Capsule CRM. Create a file named get_parties.py
and add the following code:
import requests
# Define the API endpoint and headers
url = "https://api.capsulecrm.com/api/v2/parties"
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN"
}
# Make a GET request to the Capsule API
response = requests.get(url, headers=headers)
# Check if the request was successful
if response.status_code == 200:
parties = response.json()["parties"]
for party in parties:
print(f"ID: {party['id']}, Name: {party.get('firstName', '')} {party.get('lastName', '')}")
else:
print(f"Failed to retrieve parties: {response.status_code} - {response.text}")
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth 2.0 process.
Running the Python Script and Verifying the Output
Execute the script using the following command:
python get_parties.py
If successful, the script will print out the list of parties retrieved from your Capsule CRM account. You can verify the data by checking the parties listed in your Capsule dashboard.
Handling Errors and Understanding API Response Codes
When interacting with the Capsule API, it's crucial to handle potential errors gracefully. The API may return various status codes indicating the success or failure of your request:
- 200 OK: The request was successful, and the response contains the requested data.
- 401 Unauthorized: The access token is invalid or expired. Ensure your token is correct and hasn't expired.
- 403 Forbidden: The token lacks the necessary scope to perform the request.
- 429 Too Many Requests: You have exceeded the rate limit of 4,000 requests per hour. Implement rate limiting strategies to avoid this error.
For more details on handling API responses, refer to the Capsule API Handling API Responses documentation.
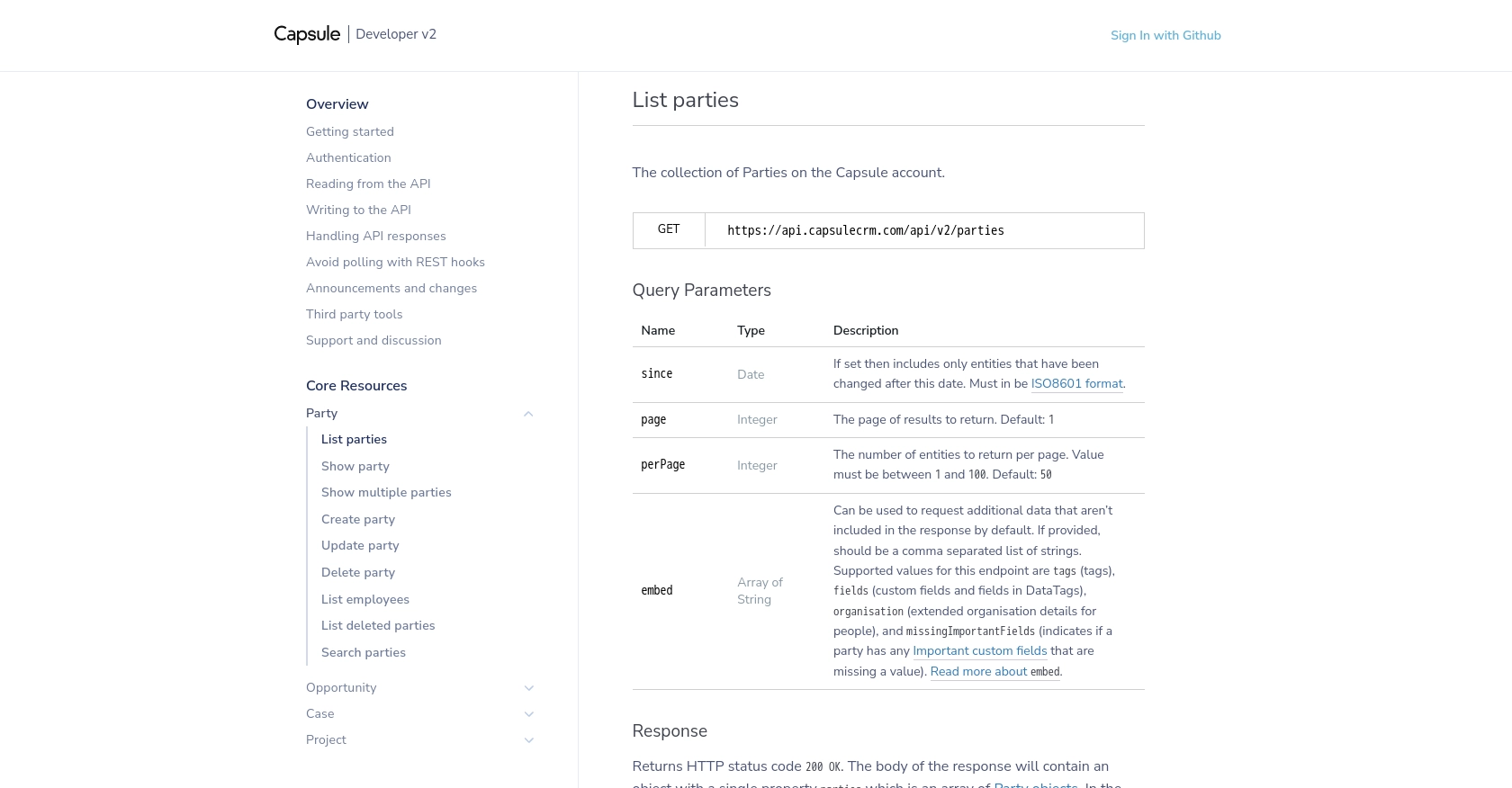
Best Practices for Capsule API Integration and Error Handling
Successfully integrating with the Capsule API involves more than just making API calls. Here are some best practices to ensure a robust and efficient integration:
- Securely Store Credentials: Always store your client ID, client secret, and access tokens securely. Avoid hardcoding them in your source code. Use environment variables or secure vaults to manage sensitive information.
- Implement Rate Limiting: Capsule API allows up to 4,000 requests per hour. To avoid hitting this limit, implement rate limiting strategies such as throttling requests or caching responses where possible. Monitor the
X-RateLimit-Remaining
header to manage your request flow. - Handle Token Expiry: Access tokens have a limited lifespan. Use the refresh token to obtain new access tokens before they expire to maintain seamless API interactions.
- Graceful Error Handling: Anticipate and handle various HTTP status codes. Implement retry logic for transient errors and provide meaningful error messages to users for better troubleshooting.
- Data Standardization: Ensure that data retrieved from Capsule is transformed and standardized to fit your application's data model. This helps maintain consistency across different systems.
Leveraging Endgrate for Streamlined Capsule Integrations
While building integrations with Capsule CRM can be rewarding, it can also be time-consuming and complex. This is where Endgrate can make a significant difference.
Endgrate offers a unified API that simplifies the integration process by providing a single endpoint to connect with multiple platforms, including Capsule CRM. By using Endgrate, you can:
- Save Time and Resources: Focus on your core product development while Endgrate handles the intricacies of API integrations.
- Build Once, Deploy Everywhere: Create integration logic once and apply it across various platforms, reducing redundancy and maintenance efforts.
- Enhance Customer Experience: Provide your users with a seamless and intuitive integration experience, boosting satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined integration process.
Read More
- https://endgrate.com/provider/capsulecrm
- https://developer.capsulecrm.com/v2/overview/authentication
- https://developer.capsulecrm.com/v2/overview/handling-api-responses
- https://developer.capsulecrm.com/v2/overview/avoid-polling-with-rest-hooks
- https://developer.capsulecrm.com/v2/operations/Party#listParties
Ready to get started?