Using the Woodpecker API to Get Prospects in Javascript
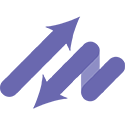
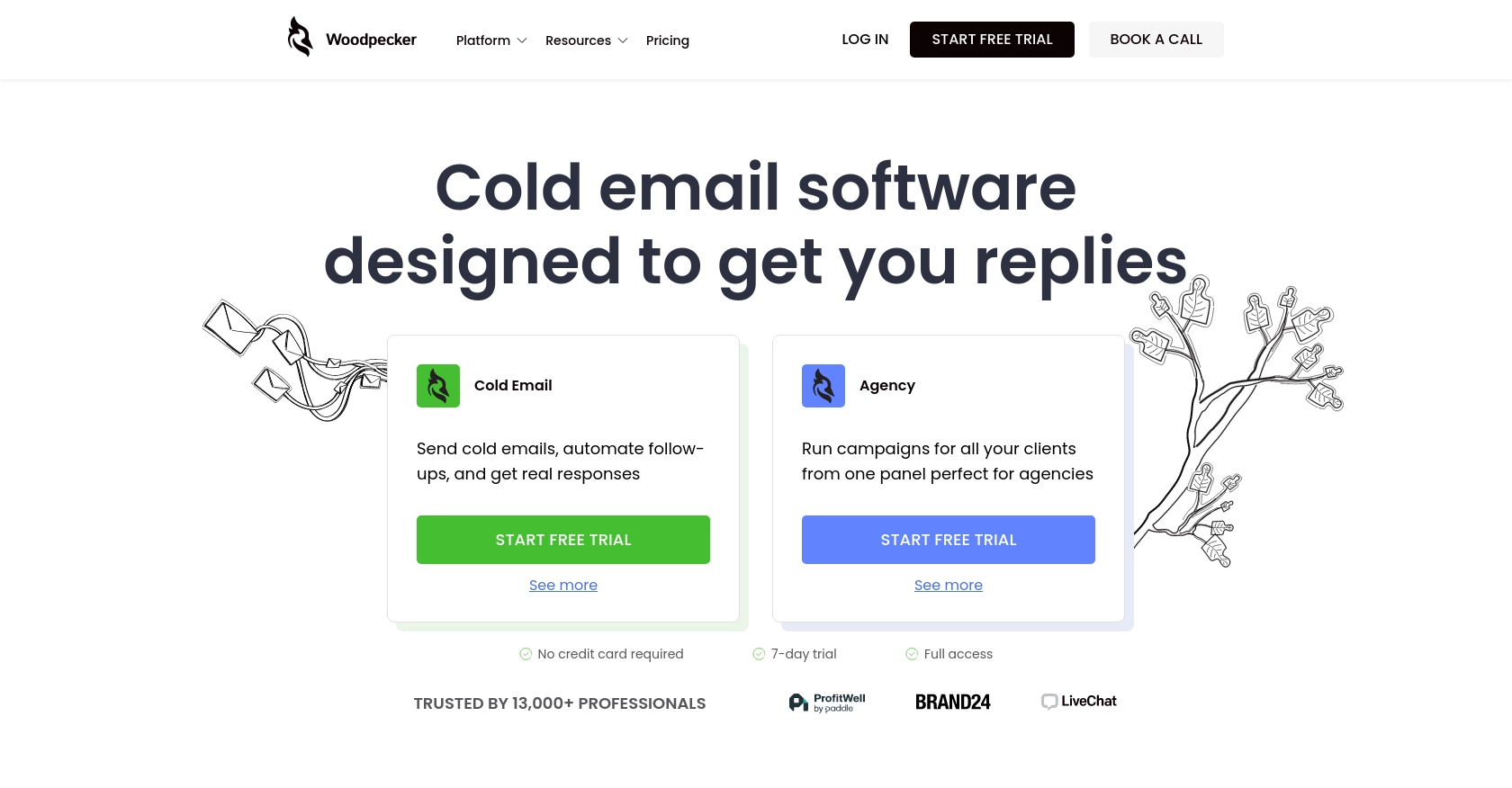
Introduction to Woodpecker API
Woodpecker is a powerful tool designed to enhance cold email outreach and automate follow-up processes for businesses. It offers a suite of features that streamline lead generation and engagement, making it a popular choice for sales teams, lead generation agencies, and business owners.
Integrating with Woodpecker's API allows developers to efficiently manage prospects and automate email campaigns. For example, you can use the Woodpecker API to retrieve a list of prospects and tailor your outreach strategies based on their engagement levels, ultimately improving your campaign's effectiveness.
Setting Up Your Woodpecker Account for API Access
Before you can start using the Woodpecker API to manage prospects, you need to set up your Woodpecker account and generate an API key. This key will allow you to authenticate your requests and interact with the Woodpecker platform programmatically.
Creating a Woodpecker Account
If you don't already have a Woodpecker account, you can sign up for a free trial on the Woodpecker website. Follow the on-screen instructions to create your account and log in.
Generating Your Woodpecker API Key
Once you have access to your Woodpecker account, follow these steps to generate an API key:
- Log into your Woodpecker account at app.woodpecker.co.
- Navigate to the Marketplace and select INTEGRATIONS.
- Click on API keys and use the green button to CREATE A KEY.
- Copy the generated API key and keep it secure, as it will be used to authenticate your API requests.
For more detailed instructions, you can refer to the Woodpecker API Key Generation Guide.
Understanding Woodpecker API Authentication
The Woodpecker API uses API key-based authentication. When making API requests, you need to include your API key in the request headers. Here's how you can set up the headers in your JavaScript code:
// Example of setting up headers with API key
const headers = {
"Authorization": "Basic "
};
Ensure that your API key is encoded in Base64 format before using it in requests. For more information, visit the Woodpecker Authentication Documentation.
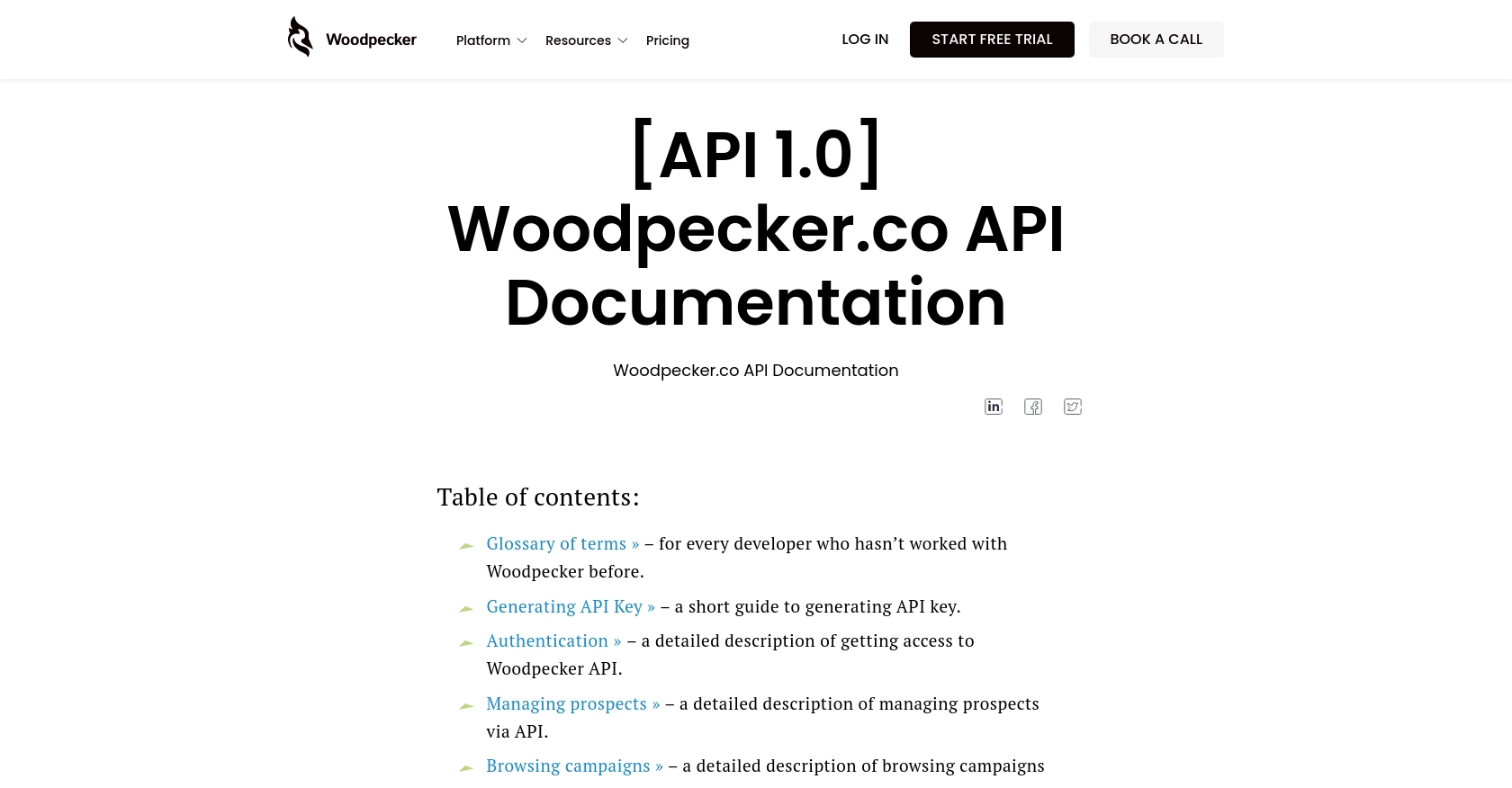
sbb-itb-96038d7
Making API Calls to Retrieve Prospects Using Woodpecker API in JavaScript
To interact with the Woodpecker API and retrieve a list of prospects, you'll need to write JavaScript code that makes HTTP requests to the API endpoints. This section will guide you through the process of setting up your environment, writing the code, and handling the responses.
Setting Up Your JavaScript Environment
Before making API calls, ensure you have Node.js installed on your machine. Node.js allows you to run JavaScript code outside of a browser. You can download it from the official Node.js website.
Once Node.js is installed, you can use npm (Node Package Manager) to install the axios
library, which simplifies making HTTP requests:
npm install axios
Writing JavaScript Code to Access Woodpecker Prospects
Now, let's write the JavaScript code to make a GET request to the Woodpecker API to retrieve prospects:
const axios = require('axios');
// Set the API endpoint and headers
const endpoint = 'https://api.woodpecker.co/rest/v1/prospects';
const headers = {
'Authorization': 'Basic '
};
// Function to get prospects
async function getProspects() {
try {
const response = await axios.get(endpoint, { headers });
const prospects = response.data;
console.log('Prospects:', prospects);
} catch (error) {
console.error('Error fetching prospects:', error.response ? error.response.data : error.message);
}
}
// Call the function
getProspects();
Replace <API_KEY>
with your actual API key. This code uses the axios
library to send a GET request to the Woodpecker API endpoint. The response is logged to the console, displaying the list of prospects.
Handling API Responses and Errors
After executing the code, you should see a list of prospects in the console. If the request fails, an error message will be displayed. Common error codes include:
- 401 Unauthorized: The API key is missing or invalid. Ensure your API key is correct and properly encoded.
- 404 Not Found: The endpoint URL is incorrect. Double-check the URL for typos.
For more detailed error information, refer to the Woodpecker API Documentation.
Verifying Successful API Calls in Woodpecker
To verify that your API call was successful, log into your Woodpecker account and navigate to the prospects section. The data retrieved via the API should match the prospects listed in your account.
By following these steps, you can efficiently retrieve and manage prospects using the Woodpecker API in JavaScript, enhancing your cold email outreach capabilities.
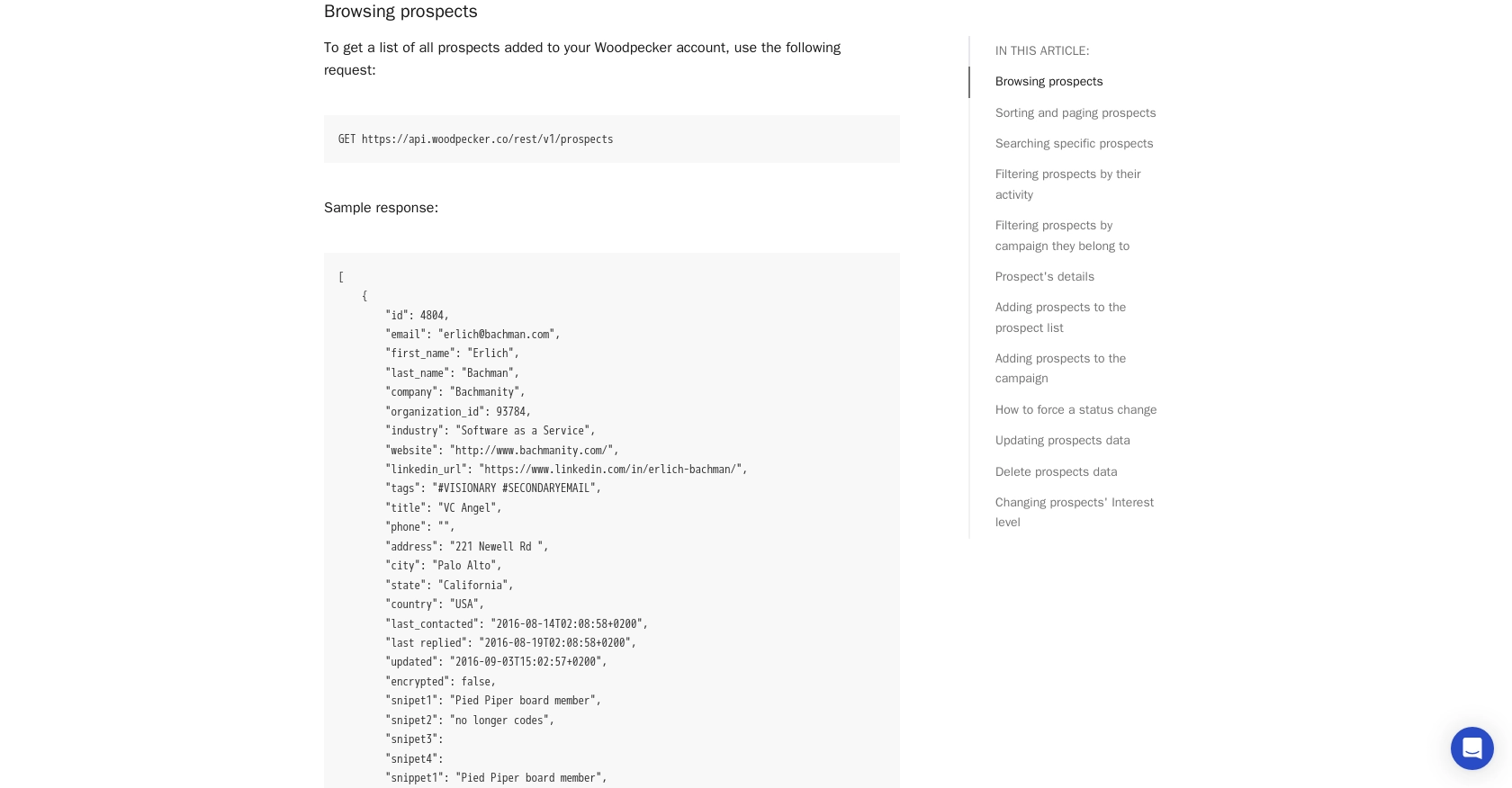
Conclusion and Best Practices for Using Woodpecker API in JavaScript
Integrating with the Woodpecker API using JavaScript can significantly enhance your cold email outreach and prospect management capabilities. By automating the retrieval and management of prospects, you can streamline your workflow and focus on crafting effective email campaigns.
Best Practices for Secure and Efficient API Integration
- Secure Storage of API Keys: Always store your API keys securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limits: Although Woodpecker allows unlimited API calls per month, remember that only one request can be processed at a time, with a maximum queue of six requests. Plan your requests accordingly to avoid dropped requests.
- Data Standardization: Ensure that the data retrieved from the API is standardized to fit your application's requirements. This may involve transforming fields or normalizing data formats.
- Error Handling: Implement robust error handling to manage potential issues such as network failures or invalid API keys. This will help maintain the stability of your application.
Enhancing Integration Capabilities with Endgrate
While integrating with the Woodpecker API can be highly beneficial, managing multiple integrations can become complex. Endgrate offers a unified API solution that simplifies integration processes across various platforms, including Woodpecker. By using Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate's website and discover the advantages of a seamless integration experience for your business.
Read More
Ready to get started?