Using the igloohome API to Get Devices in PHP
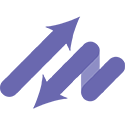
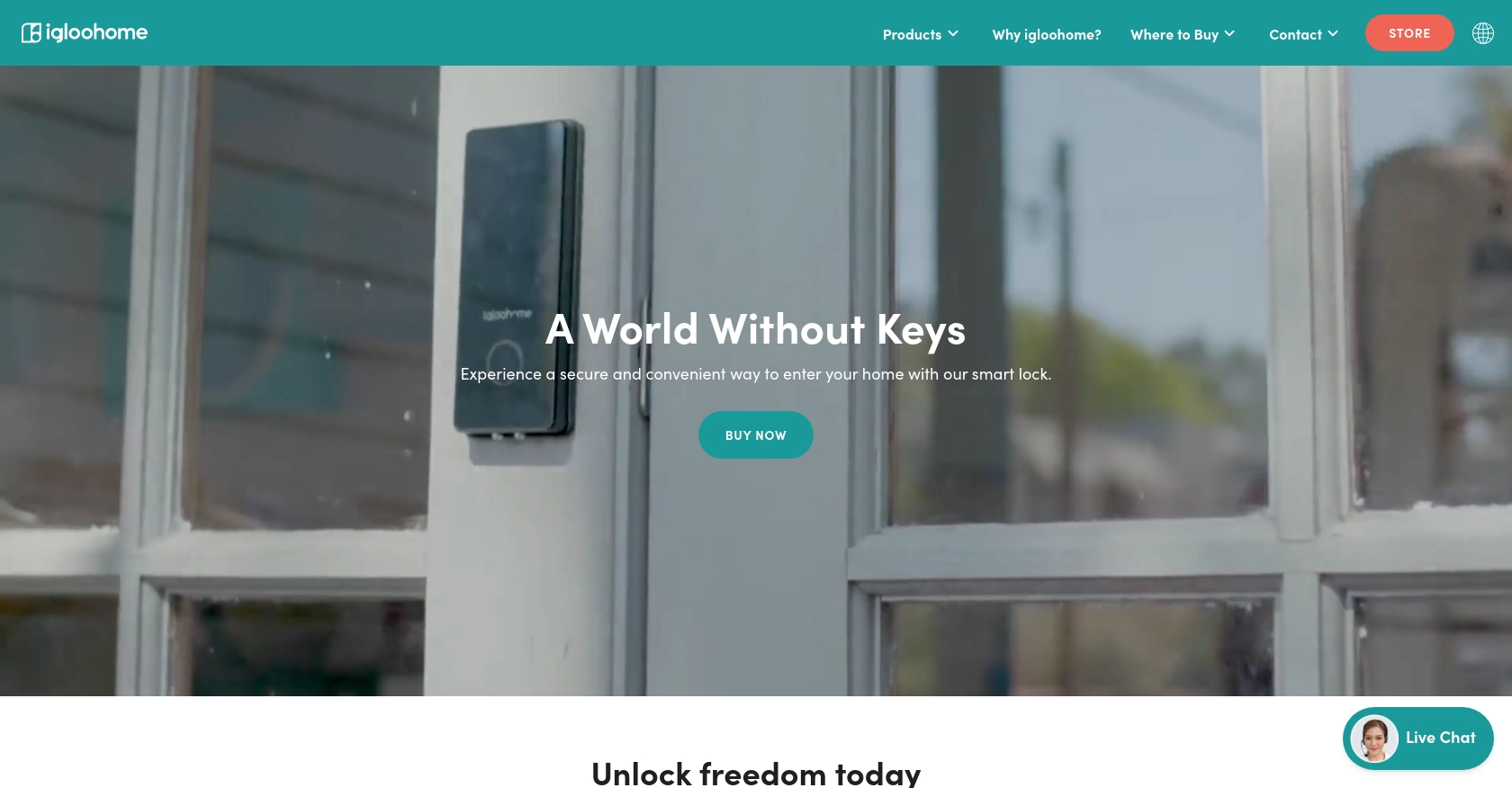
Introduction to igloohome API Integration
igloohome is a cutting-edge provider of smart access solutions, offering a range of devices that enable secure and convenient property access. Their products are widely used in residential, commercial, and vacation rental properties, providing keyless entry and remote access management.
For developers, integrating with the igloohome API opens up opportunities to manage these smart devices programmatically. By connecting with the API, developers can automate tasks such as retrieving device information, managing access permissions, and monitoring device status.
An example use case of the igloohome API is retrieving a list of devices associated with an account. This can be particularly useful for property managers who need to keep track of multiple smart locks and access points across various locations.
Setting Up Your igloohome API Test Account
Before you can start integrating with the igloohome API, you'll need to set up a test account. This will allow you to experiment with the API's features in a controlled environment.
Register for an igloohome API Trial
To begin, sign up for a 30-day trial of the igloohome API. Visit the igloohome registration page and follow the instructions to create your account. This trial provides access to the API's full functionality, enabling you to test and develop your integration.
Create an igloohome App for OAuth Authentication
igloohome uses OAuth for authentication, which requires you to create an app within your test account. Follow these steps to set up your app:
- Log in to your igloohome account.
- Navigate to the "Developer" section in the dashboard.
- Click on "Create App" and fill in the required details, such as the app name and description.
- Once your app is created, you'll receive a Client ID and Client Secret. Keep these credentials secure, as they are essential for authenticating API requests.
Configure OAuth Scopes and Permissions
Next, you'll need to configure the OAuth scopes to ensure your app has the necessary permissions to access device information:
- Go to the "Scopes" section of your app settings.
- Select the scopes related to device management, such as "read:devices" and "write:devices".
- Save your changes to update the app's permissions.
Generate an Access Token
With your app set up, you can now generate an access token to authenticate your API requests:
- Use the Client ID and Client Secret to request an access token from the igloohome OAuth server.
- Refer to the igloohome API documentation for detailed instructions on obtaining an access token.
- Store the access token securely, as it will be used in the authorization header of your API requests.
With your test account and app configured, you're ready to start making API calls to retrieve device information using PHP.
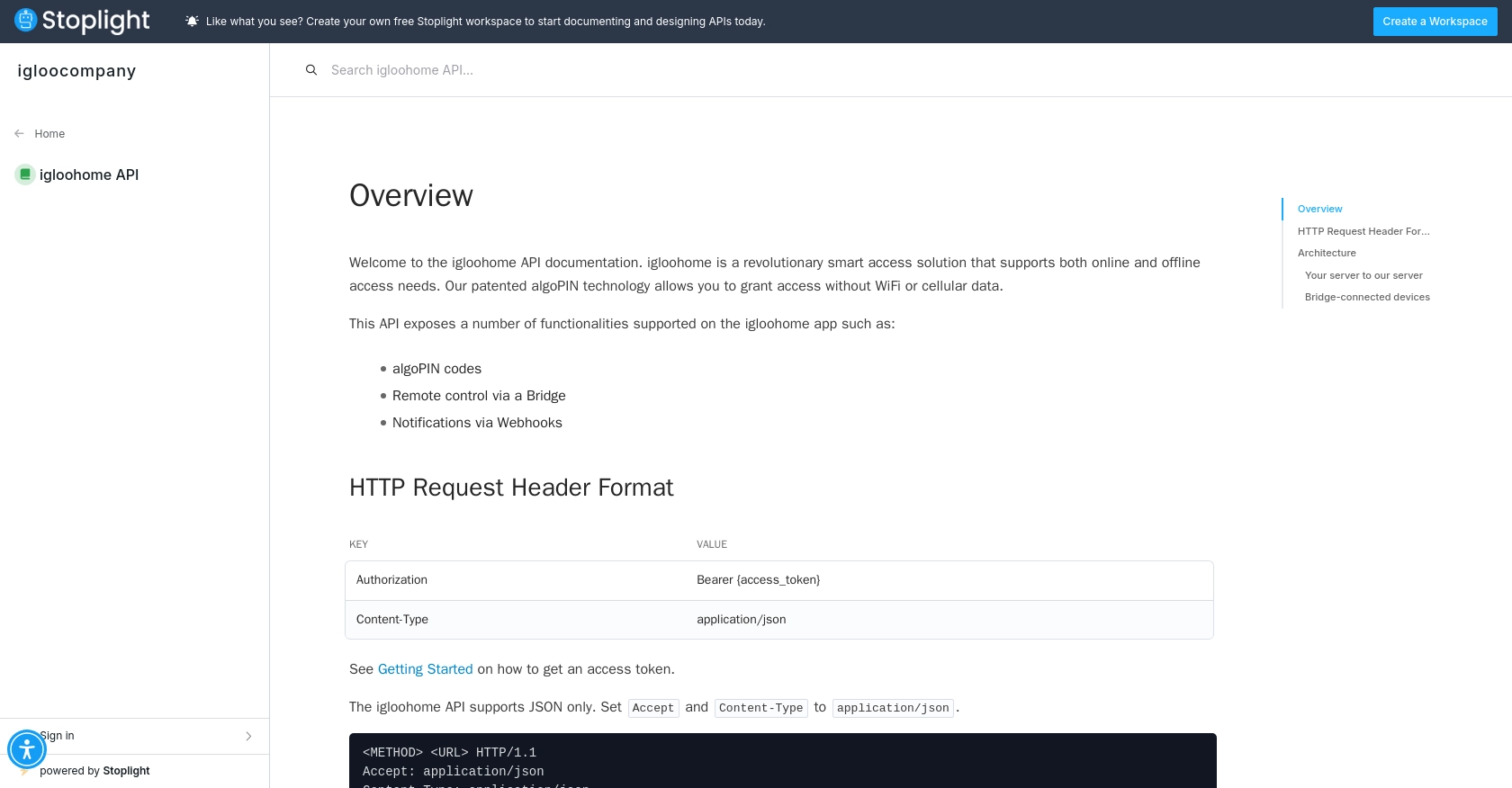
sbb-itb-96038d7
Making API Calls to Retrieve igloohome Devices Using PHP
To interact with the igloohome API and retrieve device information, you'll need to make HTTP requests using PHP. This section will guide you through the process of setting up your PHP environment, making the API call, and handling the response.
Setting Up Your PHP Environment for igloohome API Integration
Before you begin, ensure that you have PHP installed on your machine. You can download the latest version from the official PHP website. Additionally, you'll need the cURL
extension enabled to make HTTP requests.
To verify that cURL
is enabled, create a PHP file with the following content and run it:
<?php
phpinfo();
?>
Look for the cURL
section in the output. If it's not enabled, you may need to uncomment the line extension=curl
in your php.ini
file and restart your server.
Installing Dependencies for igloohome API Calls
To simplify HTTP requests, you can use the Guzzle library. Install it using Composer by running the following command in your project directory:
composer require guzzlehttp/guzzle
Writing PHP Code to Retrieve Devices from igloohome API
With your environment set up, you can now write the PHP code to make the API call. Create a file named get_igloohome_devices.php
and add the following code:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$accessToken = 'Your_Access_Token'; // Replace with your actual access token
try {
$response = $client->request('GET', 'https://api.igloohome.co/v1/devices', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Accept' => 'application/json',
],
]);
$devices = json_decode($response->getBody(), true);
foreach ($devices as $device) {
echo 'Device ID: ' . $device['id'] . '<br>';
echo 'Device Name: ' . $device['name'] . '<br>';
echo 'Device Status: ' . $device['status'] . '<br><br>';
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
?>
Replace Your_Access_Token
with the access token you obtained during the OAuth setup. This script uses Guzzle to send a GET request to the igloohome API endpoint for devices. It then parses the JSON response and outputs the device details.
Running the PHP Script and Verifying igloohome API Response
Run the script from the command line or through a web server. If successful, you should see a list of devices with their IDs, names, and statuses. Verify the data by cross-referencing it with your igloohome test account.
Handling Errors and igloohome API Response Codes
When making API calls, it's crucial to handle potential errors. The igloohome API may return various HTTP status codes indicating success or failure. Common codes include:
- 200 OK: The request was successful.
- 401 Unauthorized: Authentication failed. Check your access token.
- 404 Not Found: The requested resource could not be found.
- 500 Internal Server Error: An error occurred on the server side.
In the provided PHP code, exceptions are caught and displayed, allowing you to diagnose issues quickly.
For more detailed information on error handling, refer to the igloohome API documentation.
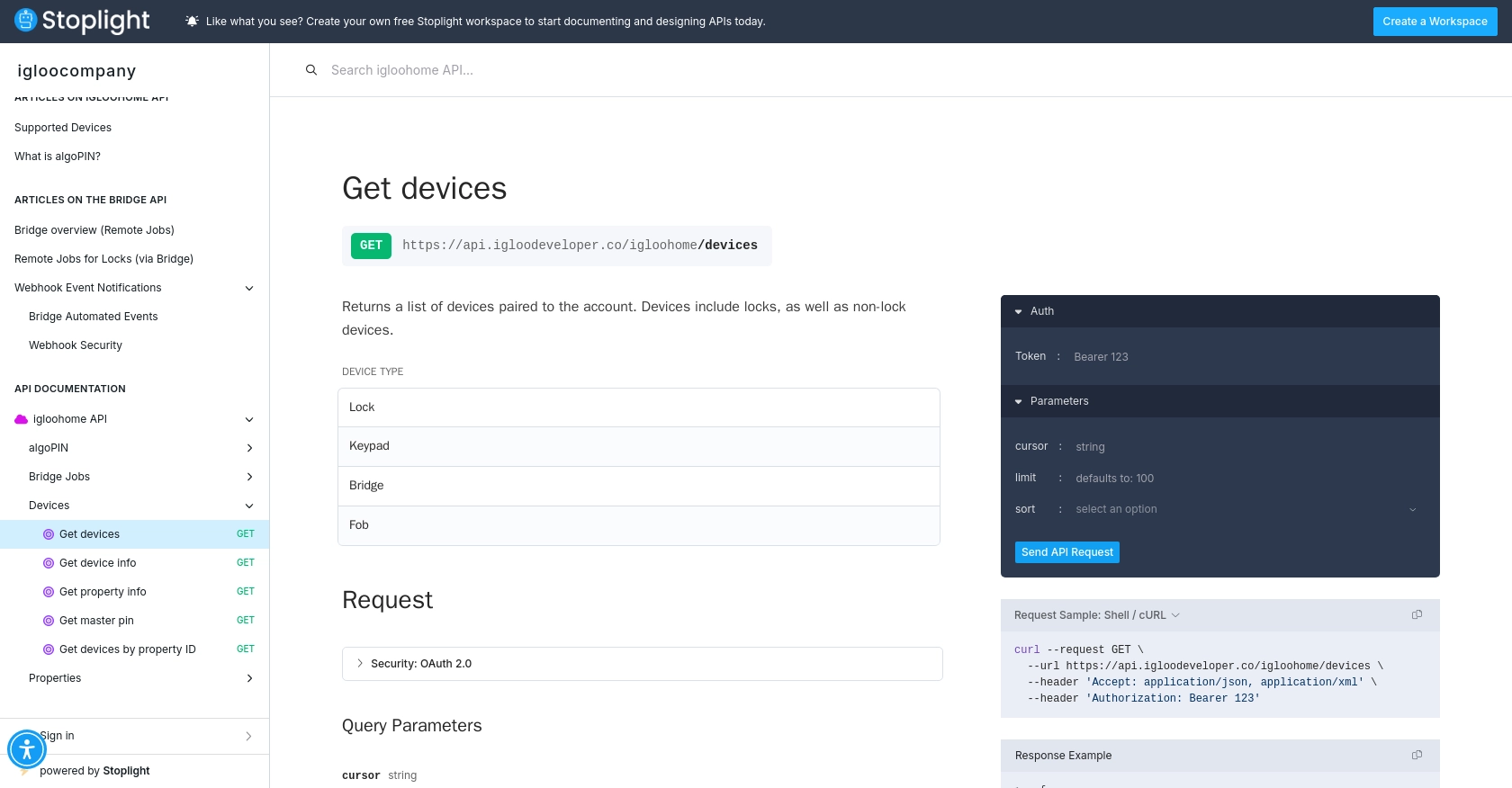
Conclusion and Best Practices for igloohome API Integration
Integrating with the igloohome API using PHP provides a powerful way to manage smart access devices programmatically. By following the steps outlined in this article, you can efficiently retrieve device information and automate access management tasks.
Best Practices for Secure and Efficient igloohome API Usage
- Secure Storage of Credentials: Always store your Client ID, Client Secret, and access tokens securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of the API's rate limits to avoid throttling. Implement exponential backoff strategies to manage retries gracefully.
- Data Standardization: Ensure that data retrieved from the API is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage different HTTP status codes and exceptions, ensuring a smooth user experience.
Streamline Your Integrations with Endgrate
While integrating with individual APIs like igloohome can be rewarding, it can also be time-consuming and complex, especially when dealing with multiple platforms. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage a single API endpoint to connect with various platforms, including igloohome. This not only saves time and resources but also provides an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the benefits of outsourcing your integration needs.
Read More
Ready to get started?