Using the Mailshake API to Create Leads in PHP
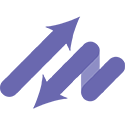
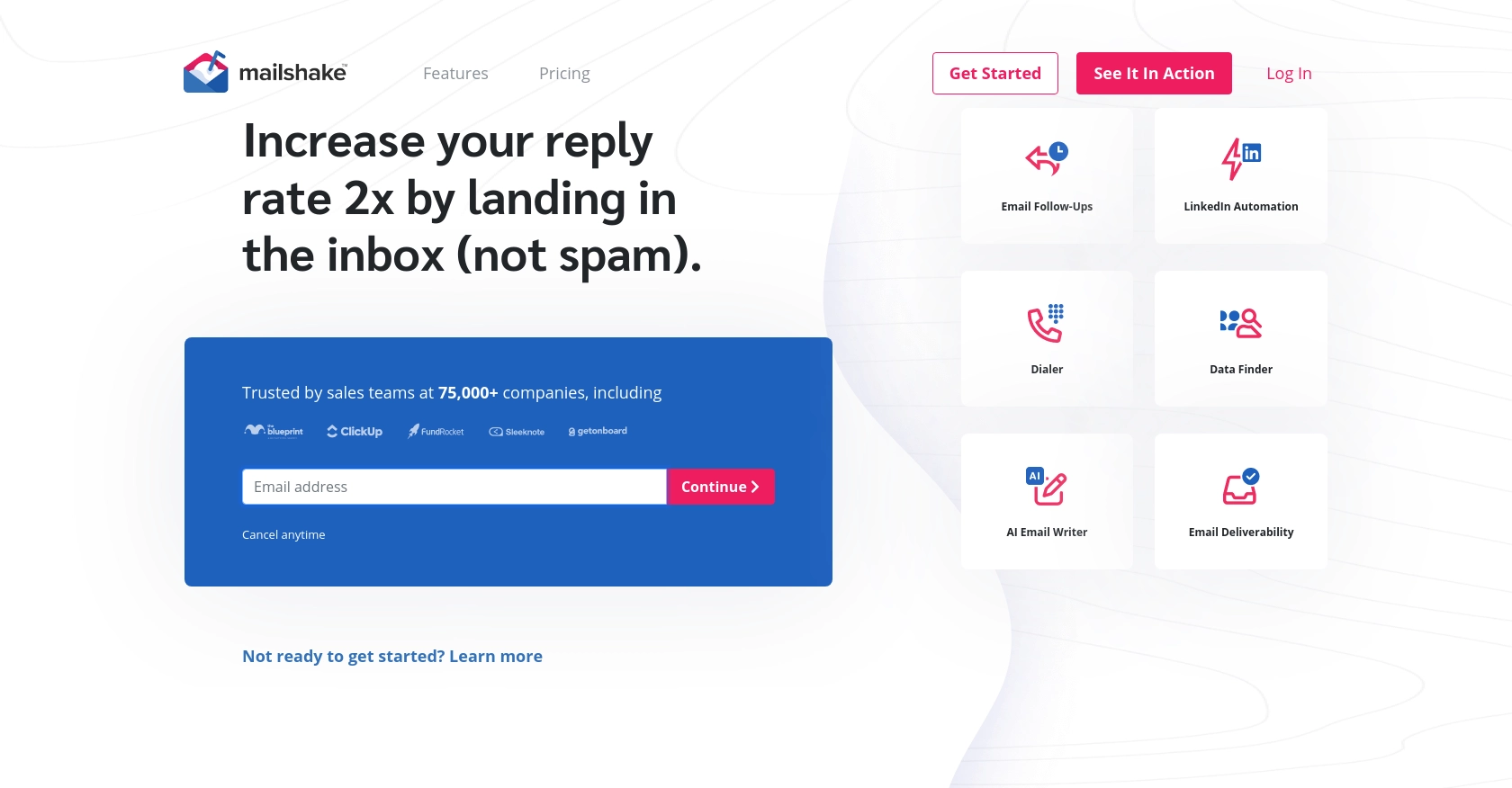
Introduction to Mailshake API
Mailshake is a powerful sales engagement platform designed to streamline outreach efforts through email campaigns, social media, and phone calls. It provides a comprehensive suite of tools to help sales teams automate and personalize their communication strategies, ultimately driving more leads and conversions.
Integrating with the Mailshake API allows developers to automate lead management processes, such as creating leads directly from email campaigns. For example, you could use the Mailshake API to automatically generate leads from recipients who engage with your email campaigns, ensuring that your sales team can follow up promptly and efficiently.
Setting Up Your Mailshake API Test Account
Before you can start using the Mailshake API to create leads, you need to set up a test account. Mailshake offers a straightforward process to obtain an API key, which is essential for authenticating your requests.
Creating a Mailshake Account
If you don't already have a Mailshake account, you can sign up for a free trial on the Mailshake website. This will give you access to the platform's features and allow you to test API integrations.
- Visit the Mailshake website and click on the "Sign Up" button.
- Follow the on-screen instructions to create your account.
- Once your account is set up, log in to access the dashboard.
Generating Your Mailshake API Key
To interact with the Mailshake API, you'll need an API key. Follow these steps to generate one:
- Navigate to the "Extensions" section in the Mailshake dashboard.
- Select "API" from the dropdown menu.
- Click on "Create API Key" to generate a new key.
- Copy the API key and store it securely, as you'll need it to authenticate your API requests.
For more details on obtaining your API key, refer to the Mailshake API documentation.
Authenticating with the Mailshake API
Mailshake uses simple API key-based authentication. Include your API key in the request header to authenticate your API calls. Here's an example of how to set up the authentication in PHP:
// Set the API endpoint and headers
$endpoint = "https://api.mailshake.com/2017-04-01/leads/create";
$headers = [
"Authorization: Basic " . base64_encode("your-api-key:")
];
// Initialize cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request
$response = curl_exec($ch);
// Close the cURL session
curl_close($ch);
// Output the response
echo $response;
Replace your-api-key
with the API key you generated earlier. This setup ensures that your requests are authenticated and can interact with the Mailshake API.
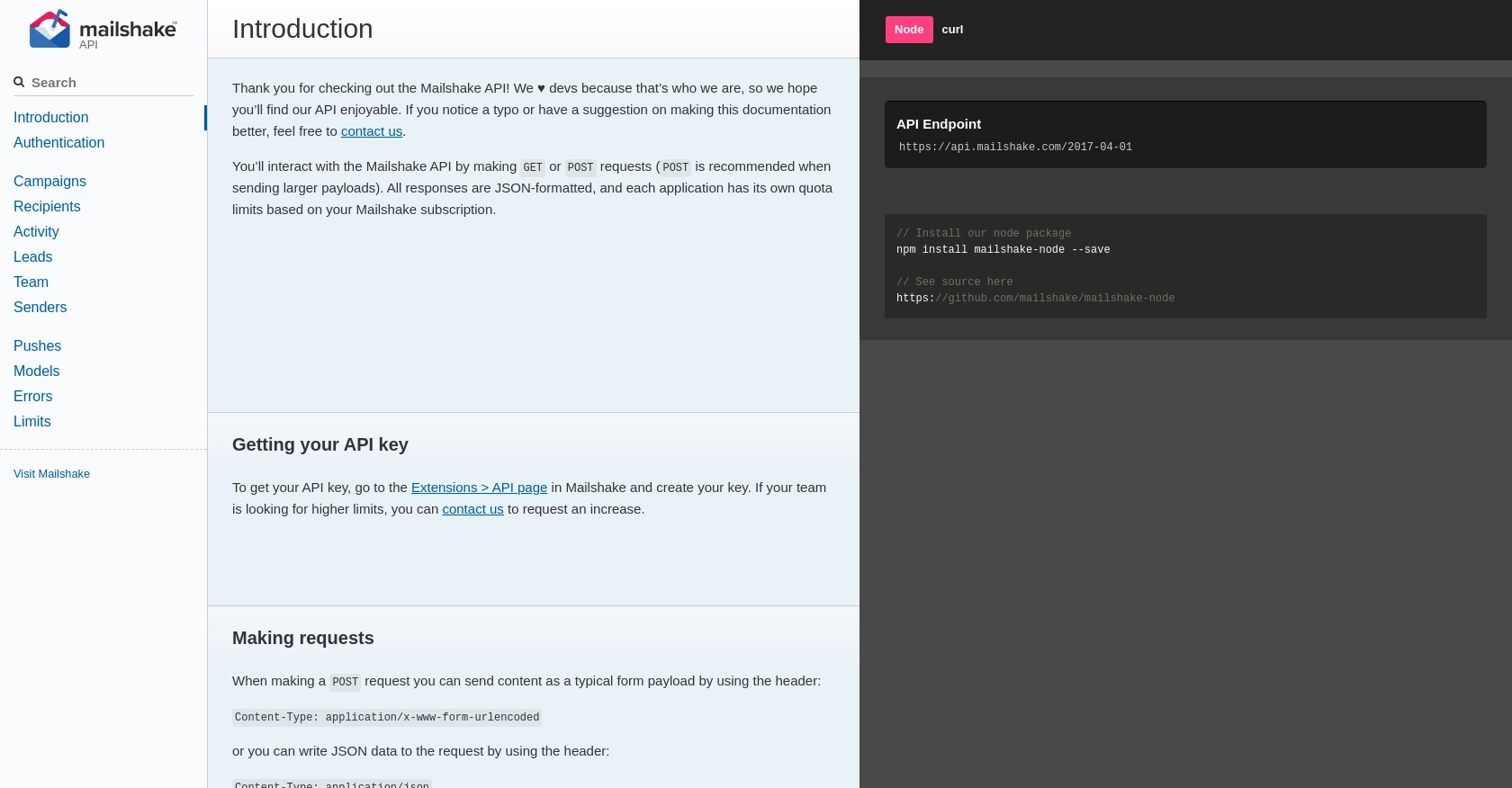
sbb-itb-96038d7
Making API Calls to Create Leads with Mailshake in PHP
To create leads using the Mailshake API in PHP, you'll need to set up your development environment and write the necessary code to interact with the API. This section will guide you through the process, ensuring you can efficiently create leads from your email campaigns.
Setting Up Your PHP Environment for Mailshake API Integration
Before making API calls, ensure your PHP environment is ready. You'll need PHP 7.4 or later and the cURL extension enabled. If you're unsure about your setup, you can check your PHP version and extensions using the following command:
php -v
Ensure cURL is enabled by checking your PHP configuration:
php -m | grep curl
Installing Necessary PHP Dependencies for Mailshake API
For this tutorial, we'll use PHP's built-in cURL functions to make HTTP requests. No additional libraries are required, but ensure your server has internet access to reach the Mailshake API endpoint.
Creating Leads with Mailshake API Using PHP
Now, let's write the PHP code to create leads using the Mailshake API. We'll use the curl
functions to send a POST request to the Mailshake API endpoint.
// Set the API endpoint and headers
$endpoint = "https://api.mailshake.com/2017-04-01/leads/create";
$headers = [
"Authorization: Basic " . base64_encode("your-api-key:"),
"Content-Type: application/x-www-form-urlencoded"
];
// Set the data for the API request
$data = http_build_query([
'campaignID' => 1,
'emailAddresses' => ['example1@domain.com', 'example2@domain.com']
]);
// Initialize cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $data);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
// Execute the request and capture the response
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
echo 'Response:' . $response;
}
// Close the cURL session
curl_close($ch);
Replace your-api-key
with the API key you generated earlier. This code sends a POST request to the Mailshake API to create leads from specified email addresses.
Verifying Successful Mailshake API Requests
After executing the code, check the response to verify the success of your API call. A successful response will include details of the created leads. You can also log in to your Mailshake account to confirm that the leads appear in your campaign.
Handling Errors and Mailshake API Error Codes
It's crucial to handle potential errors when making API calls. The Mailshake API may return various error codes, such as invalid_api_key
or missing_parameter
. Ensure your code checks for errors and handles them appropriately. For a full list of error codes, refer to the Mailshake API documentation.
Conclusion and Best Practices for Using Mailshake API in PHP
Integrating with the Mailshake API using PHP can significantly enhance your sales engagement strategy by automating lead creation and management. By following the steps outlined in this guide, you can efficiently create leads from your email campaigns, ensuring your sales team can focus on converting these leads into customers.
Best Practices for Secure and Efficient Mailshake API Integration
- Securely Store API Keys: Always store your API keys securely, avoiding hardcoding them in your source code. Consider using environment variables or a secure vault.
- Handle Rate Limits: Be mindful of Mailshake's rate limits to avoid disruptions. Implement logic to handle the
limit_reached
error and retry requests after the specified wait time. For more details, refer to the Mailshake API documentation. - Validate API Responses: Always check the API responses for errors and handle them appropriately. This ensures your application can gracefully manage issues like invalid parameters or authentication failures.
- Optimize Data Handling: When dealing with large datasets, consider implementing pagination to efficiently manage API responses and reduce load times.
Streamline Your Integrations with Endgrate
While integrating with the Mailshake API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes, allowing you to focus on your core product. With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Visit Endgrate to learn more about how you can save time and resources by outsourcing your integrations.
Read More
Ready to get started?