Using the Hubspot API to Create or Update Custom Objects in Python
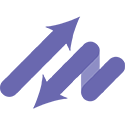
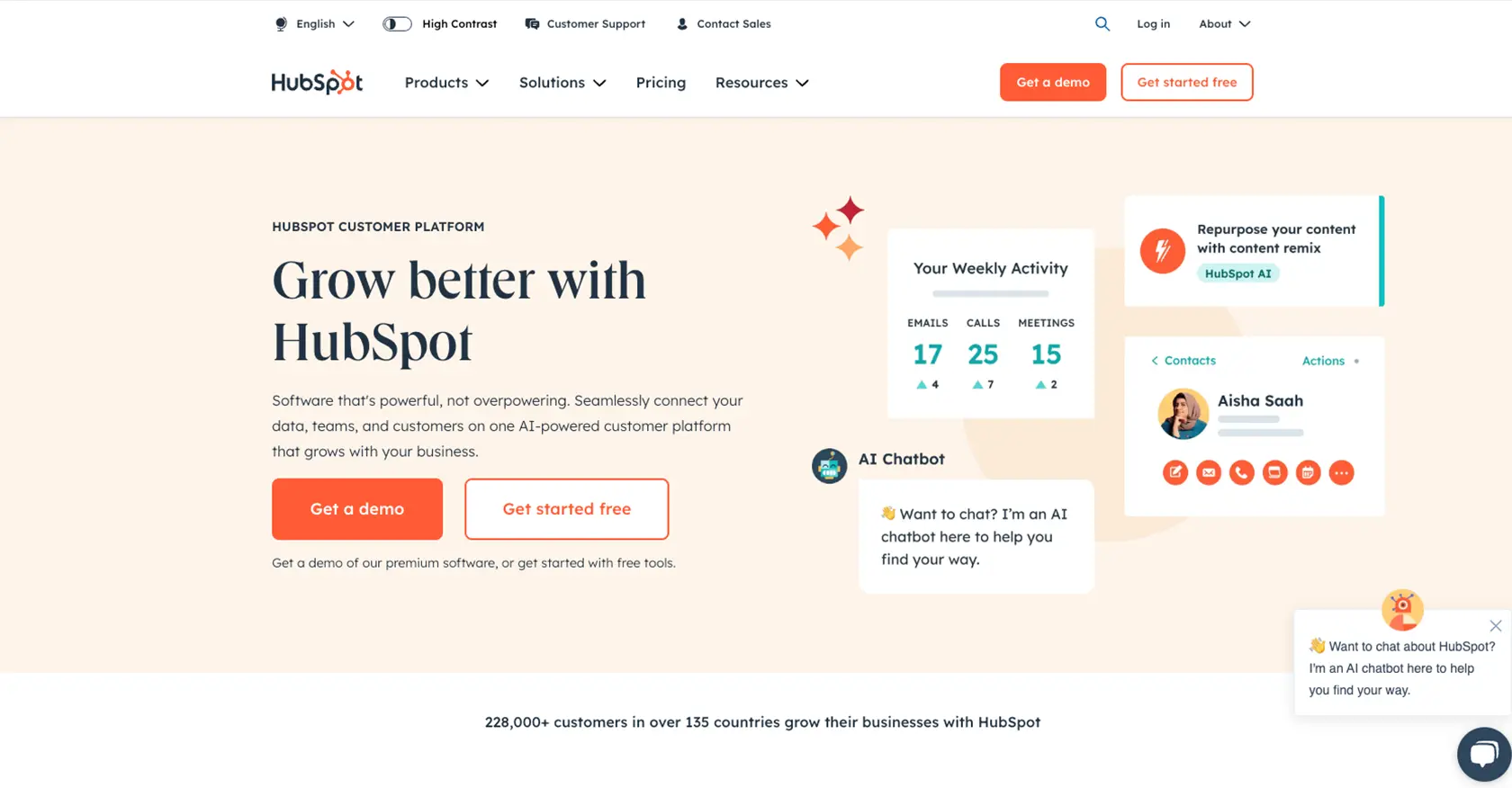
Introduction to HubSpot Custom Objects API
HubSpot is a versatile CRM platform that offers a wide range of tools to help businesses manage their marketing, sales, and customer service efforts. Its flexibility and comprehensive features make it a popular choice for companies looking to streamline their operations.
Developers often need to interact with HubSpot's API to customize and extend the platform's capabilities. One powerful feature is the ability to create and update custom objects, which allows businesses to tailor their CRM data structure to fit specific needs. For example, a developer might create a custom object to track inventory items, linking them to customer records for better sales insights.
This article will guide you through using Python to create or update custom objects in HubSpot, providing detailed steps and code examples to help you efficiently manage your CRM data.
Setting Up Your HubSpot Test/Sandbox Account for Custom Objects
Before diving into creating or updating custom objects using the HubSpot API, it's essential to set up a test or sandbox account. This environment allows you to experiment with API calls without affecting your live data, providing a safe space to develop and test your integration.
Creating a HubSpot Developer Account
To begin, you'll need a HubSpot developer account. If you don't have one, follow these steps:
- Visit the HubSpot Developer Portal.
- Click on "Create a developer account" and fill in the required information.
- Once registered, log in to your developer account.
Setting Up a HubSpot Sandbox Account
HubSpot provides sandbox accounts for testing purposes. Here's how to set one up:
- Navigate to the "Settings" section in your HubSpot account.
- Under "Account Setup," select "Sandbox Accounts."
- Follow the prompts to create a new sandbox environment.
Creating a HubSpot App for OAuth Authentication
Since HubSpot uses OAuth for authentication, you'll need to create an app to obtain the necessary credentials:
- In your developer account, go to "Apps" and click "Create App."
- Provide a name and description for your app.
- Under "Auth," select "OAuth" and configure the redirect URL.
- Save your app to generate a client ID and client secret.
Configuring OAuth Scopes for Custom Objects
To interact with custom objects, you'll need to set the appropriate OAuth scopes:
- In your app settings, navigate to "Scopes."
- Select the scopes related to custom objects, such as
crm.objects.custom.write
andcrm.objects.custom.read
. - Save your changes to update the app's permissions.
Generating an OAuth Access Token
With your app configured, you can now generate an OAuth access token:
- Direct users to the authorization URL provided in your app settings.
- Upon user consent, you'll receive an authorization code.
- Exchange this code for an access token using the following Python code:
import requests
# Set the token endpoint and your app credentials
token_url = "https://api.hubapi.com/oauth/v1/token"
client_id = "Your_Client_ID"
client_secret = "Your_Client_Secret"
redirect_uri = "Your_Redirect_URI"
authorization_code = "Your_Authorization_Code"
# Prepare the payload
payload = {
"grant_type": "authorization_code",
"client_id": client_id,
"client_secret": client_secret,
"redirect_uri": redirect_uri,
"code": authorization_code
}
# Make the POST request to get the access token
response = requests.post(token_url, data=payload)
access_token = response.json().get("access_token")
print("Access Token:", access_token)
Replace placeholders with your actual client ID, client secret, redirect URI, and authorization code. The access token will be used in subsequent API calls.
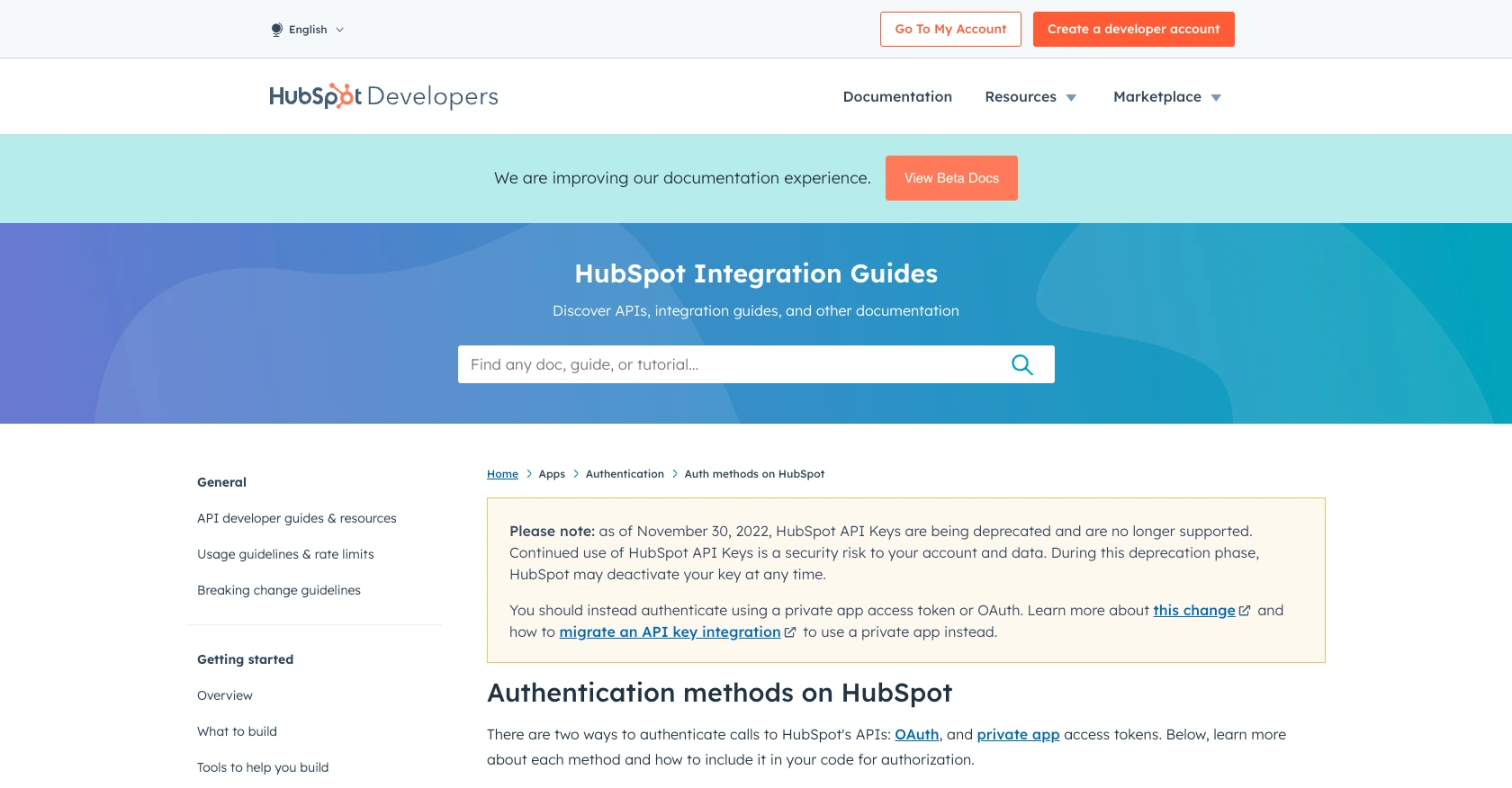
sbb-itb-96038d7
Making API Calls to Create or Update HubSpot Custom Objects Using Python
To interact with HubSpot's API for creating or updating custom objects, you'll need to use Python and ensure you have the necessary dependencies installed. This section will guide you through the process of making API calls to manage custom objects effectively.
Setting Up Your Python Environment for HubSpot API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using the following command:
pip install requests
Creating a Custom Object in HubSpot Using Python
To create a custom object, you'll need to define its schema, including properties and associations. Use the following Python code to make a POST request to the HubSpot API:
import requests
# Set the API endpoint and headers
url = "https://api.hubapi.com/crm/v3/schemas"
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Define the custom object schema
custom_object_schema = {
"name": "inventory_item",
"labels": {
"singular": "Inventory Item",
"plural": "Inventory Items"
},
"primaryDisplayProperty": "item_name",
"properties": [
{"name": "item_name", "label": "Item Name", "type": "string", "fieldType": "text"},
{"name": "quantity", "label": "Quantity", "type": "number", "fieldType": "number"}
],
"associatedObjects": ["CONTACT"]
}
# Make the POST request to create the custom object
response = requests.post(url, json=custom_object_schema, headers=headers)
# Check the response
if response.status_code == 201:
print("Custom Object Created Successfully")
else:
print("Failed to Create Custom Object:", response.json())
Replace Your_Access_Token
with the OAuth access token you generated earlier. This code defines a custom object schema for an inventory item and sends a POST request to create it in HubSpot.
Updating a Custom Object in HubSpot Using Python
To update an existing custom object, you'll need its objectTypeId
. Use the following Python code to make a PATCH request:
import requests
# Set the API endpoint and headers
object_type_id = "2-1234567" # Replace with your custom object's ID
url = f"https://api.hubapi.com/crm/v3/schemas/{object_type_id}"
headers = {
"Content-Type": "application/json",
"Authorization": "Bearer Your_Access_Token"
}
# Define the updated properties
updated_properties = {
"properties": [
{"name": "price", "label": "Price", "type": "number", "fieldType": "number"}
]
}
# Make the PATCH request to update the custom object
response = requests.patch(url, json=updated_properties, headers=headers)
# Check the response
if response.status_code == 200:
print("Custom Object Updated Successfully")
else:
print("Failed to Update Custom Object:", response.json())
Ensure you replace object_type_id
with your actual custom object's ID. This code updates the custom object by adding a new property for price.
Verifying API Call Success and Handling Errors
After making API calls, verify the success by checking the response status code. A status code of 201
indicates successful creation, while 200
indicates a successful update. If the request fails, the response will contain error details.
HubSpot's API documentation provides detailed information on error codes and their meanings. For instance, a 429
error indicates rate limiting, which means you've exceeded the allowed number of requests. Refer to the HubSpot API usage guidelines for more information on handling rate limits and errors.
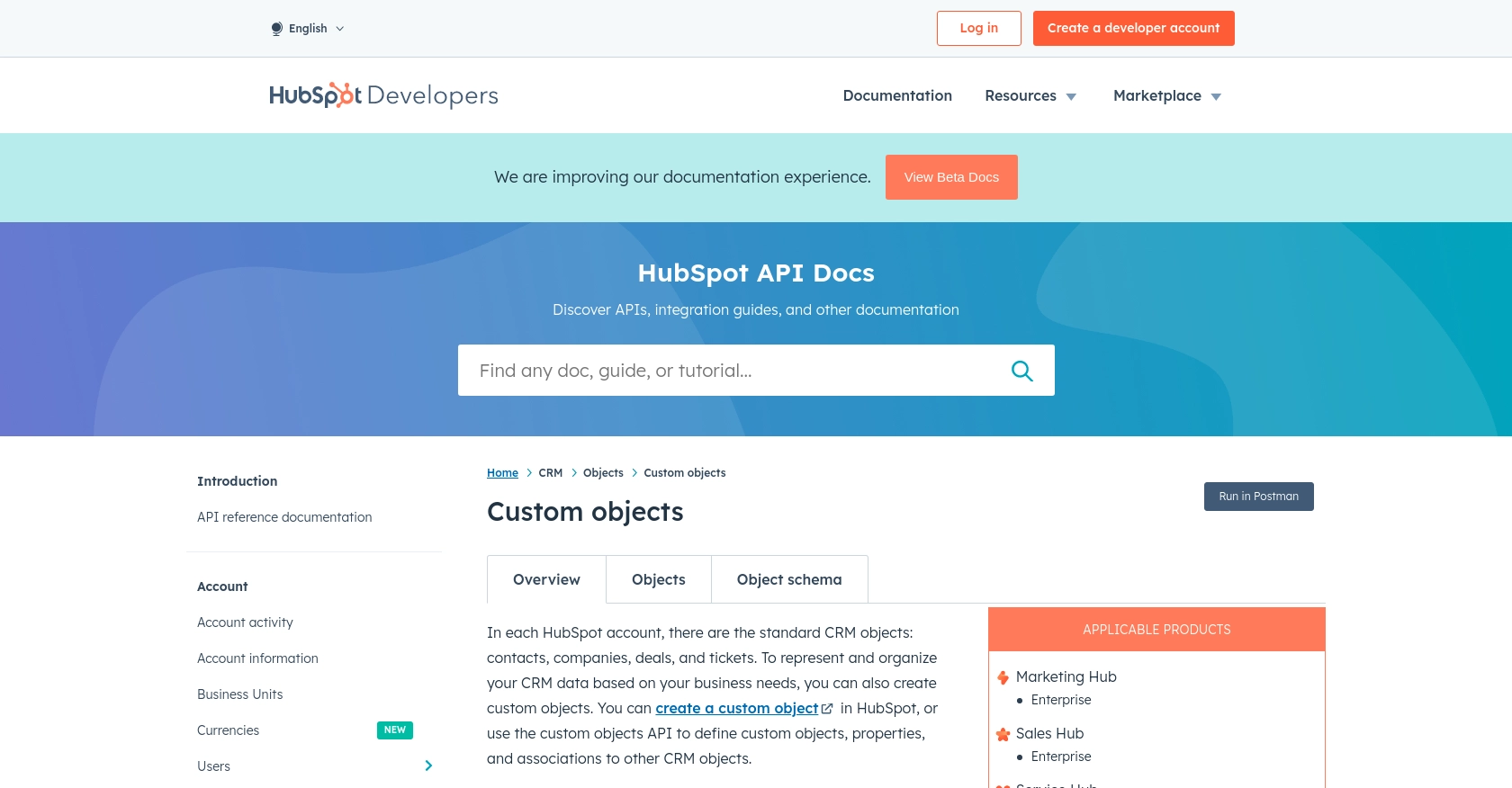
Conclusion and Best Practices for Using HubSpot API with Python
Integrating with HubSpot's API to create or update custom objects using Python can significantly enhance your CRM capabilities, allowing you to tailor data structures to meet specific business needs. By following the steps outlined in this guide, you can efficiently manage custom objects and ensure seamless data integration within your HubSpot environment.
Best Practices for Secure and Efficient HubSpot API Integration
- Secure Storage of Credentials: Always store your OAuth credentials securely. Avoid hardcoding them in your scripts. Use environment variables or secure vaults to manage sensitive information.
- Handling Rate Limits: Be mindful of HubSpot's API rate limits. For public apps using OAuth, the limit is 100 requests every 10 seconds per account. Implement retry logic and exponential backoff strategies to handle
429
rate limit errors gracefully. For more details, refer to the HubSpot API usage guidelines. - Data Standardization: Ensure that data fields are standardized across your custom objects to maintain consistency and improve data quality.
- Error Handling: Implement robust error handling to manage API call failures. Log errors for troubleshooting and consider using alerting mechanisms to notify your team of critical issues.
Leverage Endgrate for Streamlined Integration Management
While building custom integrations with HubSpot's API can be rewarding, it can also be time-consuming and complex. Endgrate offers a unified API solution that simplifies integration management across multiple platforms, including HubSpot. By using Endgrate, you can:
- Save time and resources by outsourcing integration tasks and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations, reducing redundancy and maintenance efforts.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined, efficient integration process.
Read More
- https://endgrate.com/provider/hubspot
- https://developers.hubspot.com/docs/api/overview
- https://developers.hubspot.com/docs/api/intro-to-auth
- https://developers.hubspot.com/docs/api/usage-details
- https://developers.hubspot.com/docs/api/scopes
- https://developers.hubspot.com/docs/api/crm/crm-custom-objects
- https://developers.hubspot.com/docs/api/crm/properties
Ready to get started?