Using the Customer.io App API to Get Customers (with Javascript examples)
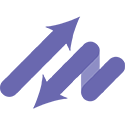
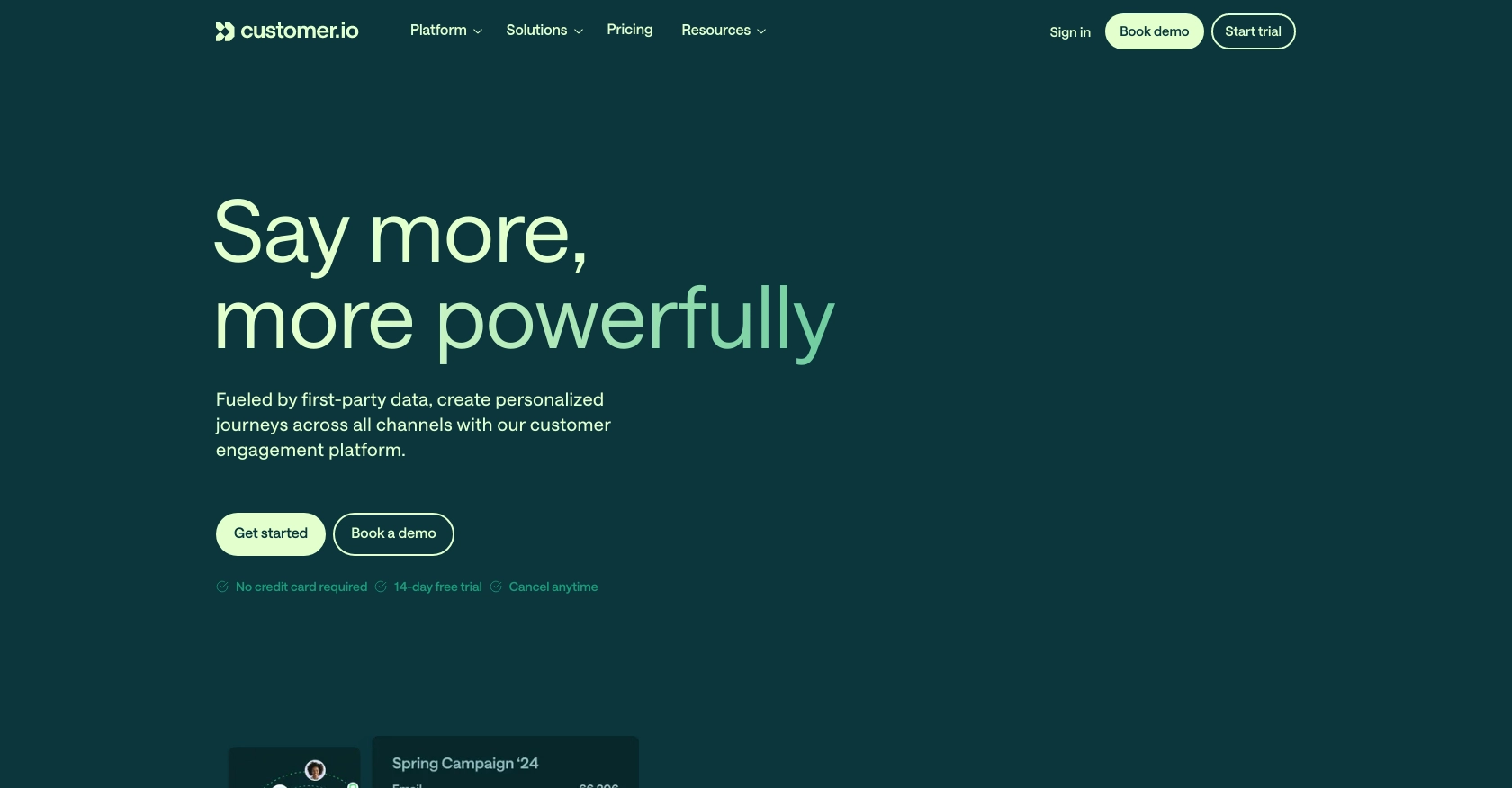
Introduction to Customer.io App API
Customer.io is a powerful platform designed to help businesses manage and automate their customer engagement through targeted messaging. With its robust API, Customer.io enables developers to seamlessly integrate customer data and messaging capabilities into their applications, enhancing communication strategies and improving customer experiences.
Connecting with the Customer.io App API allows developers to access and manage customer data efficiently. For example, you can retrieve customer information to personalize marketing campaigns, ensuring that each message resonates with the recipient's preferences and behaviors.
In this article, we will explore how to use JavaScript to interact with the Customer.io App API, focusing on retrieving customer data. This integration can significantly streamline your marketing efforts by providing real-time access to customer insights.
Setting Up Your Customer.io App API Test Account
Before diving into the integration with the Customer.io App API, it's essential to set up a test account. This will allow you to safely experiment with API calls and understand how the platform works without affecting live data.
Create a Customer.io Account
If you don't have a Customer.io account, start by signing up for a free trial on the Customer.io website. Follow the on-screen instructions to complete the registration process. If you already have an account, simply log in.
Generate an API Key for Customer.io App API
To interact with the Customer.io App API, you'll need an API key. Follow these steps to generate one:
- Log in to your Customer.io account.
- Navigate to the Account Settings section.
- Select API Keys from the menu.
- Click on Create API Key.
- Provide a name for your API key and select the appropriate permissions.
- Click Generate to create the key.
- Copy the generated API key and store it securely, as you will need it for authentication in your API requests.
Configure Your Sandbox Environment
Customer.io provides a sandbox environment for testing purposes. This allows you to simulate API interactions without impacting your production data. To set up your sandbox environment:
- Ensure that your API key has access to the sandbox environment.
- Use the sandbox-specific endpoints provided by Customer.io for testing your API calls.
Authenticate Your API Requests
All requests to the Customer.io App API require authentication using the API key. Here's how you can set up authentication in your JavaScript code:
// Example of setting up authentication in JavaScript
const apiKey = 'Your_API_Key_Here';
const headers = {
'Authorization': `Bearer ${apiKey}`,
'Content-Type': 'application/json'
};
// Example API request
fetch('https://api.customer.io/v1/customers', {
method: 'GET',
headers: headers
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
Replace Your_API_Key_Here
with the API key you generated earlier. This setup will allow you to authenticate and make requests to the Customer.io App API.
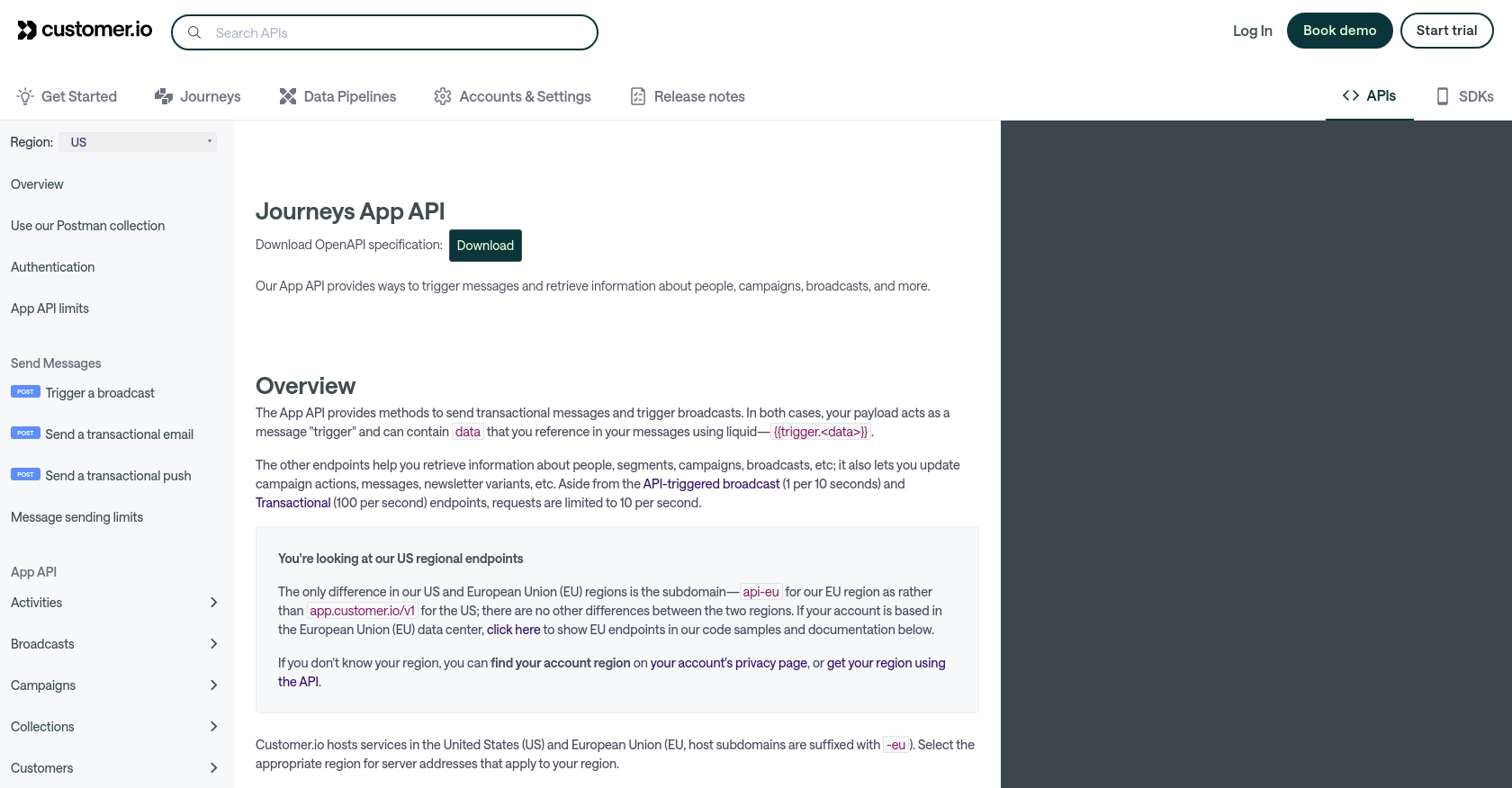
sbb-itb-96038d7
Making API Calls to Retrieve Customer Data from Customer.io App
To effectively interact with the Customer.io App API using JavaScript, it's crucial to understand the process of making API calls. This section will guide you through setting up your environment, writing the necessary code, and handling responses and errors.
JavaScript Environment Setup for Customer.io App API
Before making API calls, ensure you have a suitable JavaScript environment. You can use Node.js or a browser-based environment. Make sure you have access to the fetch
API or a similar HTTP client like Axios.
Dependencies and Tools for Customer.io App API Integration
- Ensure you have Node.js installed if you're working in a server environment.
- Use a package manager like npm to install any necessary libraries, such as Axios, if you prefer it over
fetch
.
Example Code for Fetching Customer Data from Customer.io App API
Below is an example of how to use JavaScript to fetch customer data from the Customer.io App API:
// Set up the API endpoint and headers
const apiKey = 'Your_API_Key_Here';
const endpoint = 'https://api.customer.io/v1/customers';
const headers = {
'Authorization': `Bearer ${apiKey}`,
'Content-Type': 'application/json'
};
// Function to fetch customer data
async function fetchCustomers() {
try {
const response = await fetch(endpoint, {
method: 'GET',
headers: headers
});
if (!response.ok) {
throw new Error(`Error: ${response.status} ${response.statusText}`);
}
const data = await response.json();
console.log('Customer Data:', data);
} catch (error) {
console.error('Failed to fetch customer data:', error);
}
}
// Call the function to fetch data
fetchCustomers();
Replace Your_API_Key_Here
with your actual API key. This code sets up the necessary headers and makes a GET request to the Customer.io App API to retrieve customer data.
Handling API Responses and Errors
When making API calls, it's essential to handle responses and potential errors effectively. The example above includes error handling using a try-catch block. If the response is not successful, an error is thrown, and the catch block logs the error message.
Check the response status code to determine if the request was successful. A status code of 200 indicates success, while other codes may indicate errors. Refer to the Customer.io API documentation for more details on error codes and their meanings.
Verifying API Call Success in Customer.io App Sandbox
After making API calls, verify their success by checking the sandbox environment in Customer.io. Ensure that the data retrieved matches the expected results and that no errors occurred during the process.
By following these steps, you can efficiently interact with the Customer.io App API using JavaScript, enabling seamless integration of customer data into your applications.
Conclusion and Best Practices for Customer.io App API Integration
Integrating with the Customer.io App API using JavaScript provides a powerful way to enhance your customer engagement strategies. By accessing real-time customer data, you can tailor your marketing efforts to meet individual preferences, ultimately improving customer satisfaction and retention.
Best Practices for Secure and Efficient API Usage
- Secure API Keys: Always store your API keys securely. Avoid hardcoding them in your source code and consider using environment variables or secure vaults.
- Handle Rate Limits: Be mindful of the API rate limits. The Customer.io App API allows up to 10 requests per second. Implement retry logic to handle rate limit errors gracefully.
- Data Transformation: Standardize and transform data fields as needed to ensure consistency across your applications.
- Error Handling: Implement robust error handling to manage different response codes and exceptions effectively. Refer to the Customer.io API documentation for detailed error code descriptions.
Enhance Your Integration with Endgrate
For developers looking to streamline their integration processes, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration needs by visiting Endgrate.
Read More
Ready to get started?