Using the Sage Accounting API to Get Vendors (with PHP examples)
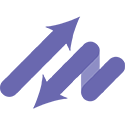
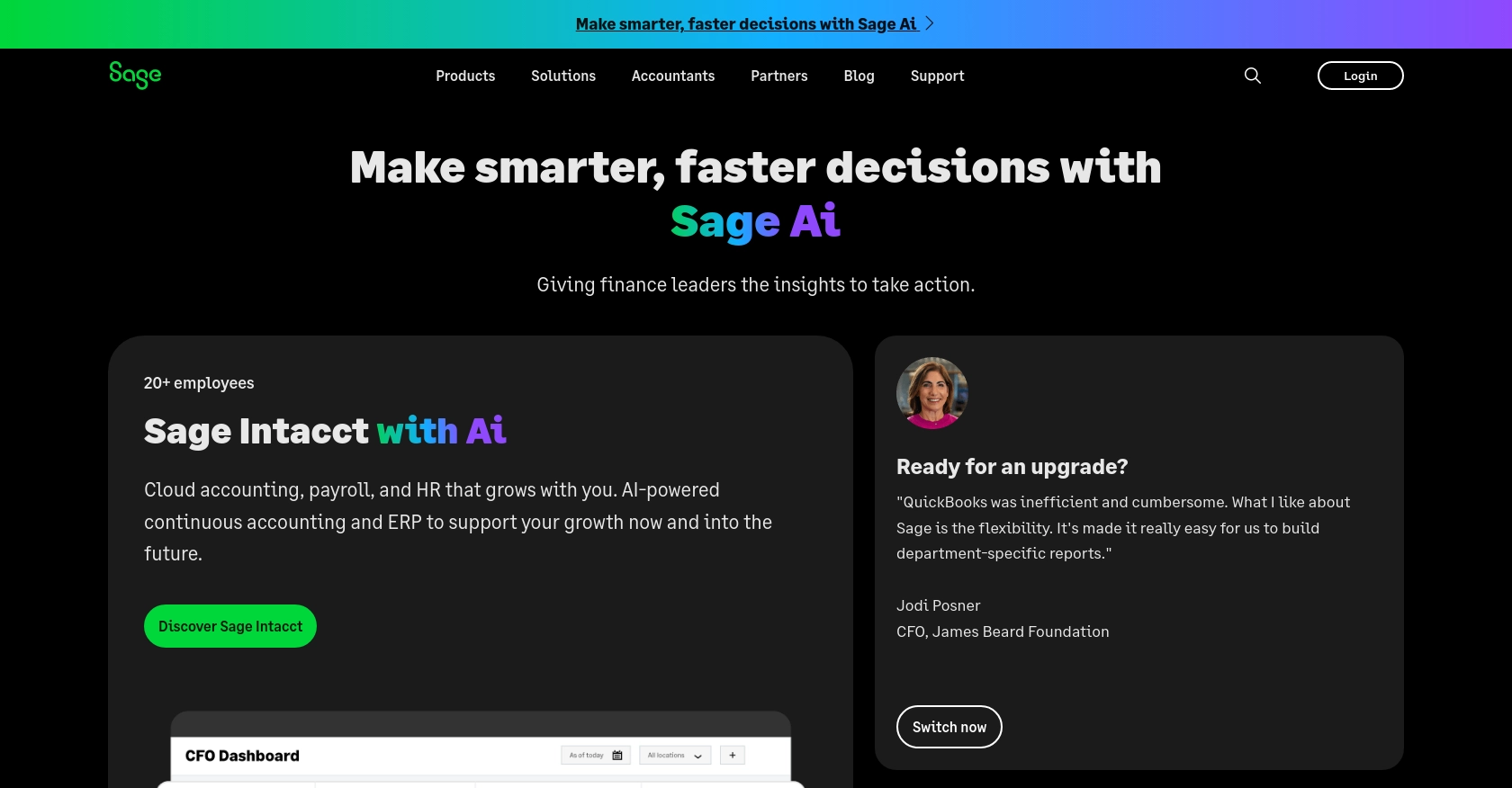
Introduction to Sage Accounting API
Sage Accounting is a robust cloud-based accounting solution tailored for small and medium-sized businesses. It offers a comprehensive suite of tools to manage finances, track expenses, and streamline accounting processes. With its user-friendly interface and powerful features, Sage Accounting is a preferred choice for businesses aiming to enhance their financial management.
Integrating with the Sage Accounting API allows developers to automate and optimize various accounting tasks. For example, by accessing vendor data through the API, developers can streamline procurement processes, ensuring that vendor information is always up-to-date and easily accessible. This integration can significantly reduce manual data entry and improve overall efficiency.
Setting Up Your Sage Accounting Test/Sandbox Account
To begin integrating with the Sage Accounting API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with the API without affecting live data. Follow these steps to get started:
Create a Sage Developer Account
First, you'll need a Sage Developer account to access the API and manage your applications.
- Visit the Sage Developer portal and sign up using your GitHub account or email address.
- Once registered, you'll have access to the developer dashboard where you can manage your applications and obtain API credentials.
Set Up a Trial Business for Development
Sage allows you to create trial businesses for development purposes. This is essential for testing your integration.
- Navigate to the Set up the Basics page.
- Select the appropriate region and subscription tier for your trial business.
- Follow the instructions to create your trial business account.
Create a Sage Accounting App for OAuth Authentication
Since the Sage Accounting API uses OAuth for authentication, you need to create an app to obtain your client credentials.
- Log in to your Sage Developer account and click on "Create App."
- Provide a name and callback URL for your app. Refer to the Create an App guide for more details.
- Save your app to generate a Client ID and Client Secret, which you'll use for OAuth authentication.
Upgrade to a Developer Account
To fully utilize the Sage Accounting API, upgrade your trial account to a developer account.
- Submit a request using the Upgrade your account form.
- Provide the necessary details, including your app name and Client ID.
- Wait for confirmation from the Sage team, which typically takes 3-5 working days.
Once these steps are completed, you'll be ready to interact with the Sage Accounting API in your test environment, allowing you to develop and test your integrations efficiently.
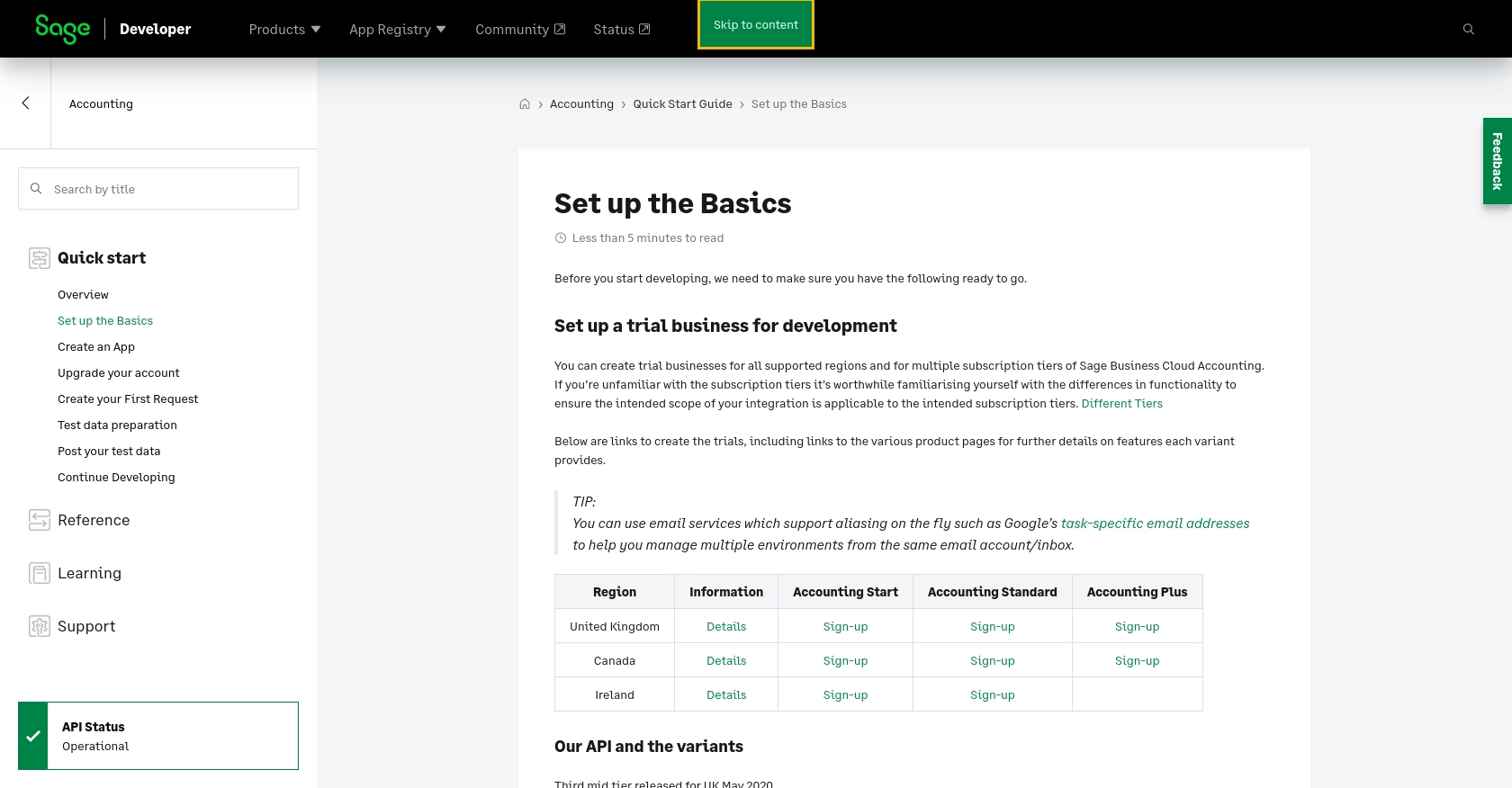
sbb-itb-96038d7
Making API Calls to Retrieve Vendors from Sage Accounting Using PHP
In this section, we'll explore how to interact with the Sage Accounting API to retrieve vendor data using PHP. This integration can help automate vendor management processes, ensuring that your vendor information is always current and accessible.
Prerequisites for Using PHP with Sage Accounting API
Before you begin, ensure you have the following installed on your system:
- PHP 7.4 or higher
- Composer for dependency management
You'll also need to install the Guzzle HTTP client, which simplifies making HTTP requests in PHP. Run the following command to install Guzzle:
composer require guzzlehttp/guzzle
Setting Up OAuth Authentication for Sage Accounting API
Since the Sage Accounting API uses OAuth for authentication, you'll need to authenticate your requests using the Client ID and Client Secret obtained during the app setup. Here's a basic example of how to set up OAuth authentication:
use GuzzleHttp\Client;
$client = new Client();
$response = $client->post('https://oauth.accounting.sage.com/token', [
'form_params' => [
'grant_type' => 'client_credentials',
'client_id' => 'Your_Client_ID',
'client_secret' => 'Your_Client_Secret',
],
]);
$accessToken = json_decode($response->getBody(), true)['access_token'];
Replace Your_Client_ID
and Your_Client_Secret
with your actual credentials.
Retrieving Vendor Data from Sage Accounting API
Once authenticated, you can make a GET request to the Sage Accounting API to retrieve vendor data. Here's how you can do it using PHP:
use GuzzleHttp\Client;
$client = new Client();
$response = $client->get('https://api.accounting.sage.com/v3.1/contacts', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Accept' => 'application/json',
],
'query' => [
'contact_type_id' => 'VENDOR_TYPE_ID', // Replace with actual vendor type ID
],
]);
$vendors = json_decode($response->getBody(), true);
foreach ($vendors['data'] as $vendor) {
echo 'Vendor Name: ' . $vendor['name'] . "\n";
}
Ensure you replace VENDOR_TYPE_ID
with the actual ID for vendors in your Sage Accounting setup.
Handling API Response and Errors
After making the API call, it's crucial to handle the response and any potential errors. Here's an example of how to manage errors:
try {
$response = $client->get('https://api.accounting.sage.com/v3.1/contacts', [
'headers' => [
'Authorization' => 'Bearer ' . $accessToken,
'Accept' => 'application/json',
],
]);
$vendors = json_decode($response->getBody(), true);
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Check the Sage Accounting API documentation for specific error codes and their meanings: Sage API Documentation.
Verifying API Call Success in Sage Accounting Sandbox
To verify that your API call was successful, log in to your Sage Accounting sandbox account and check the vendor data. The retrieved data should match the information in your sandbox environment.
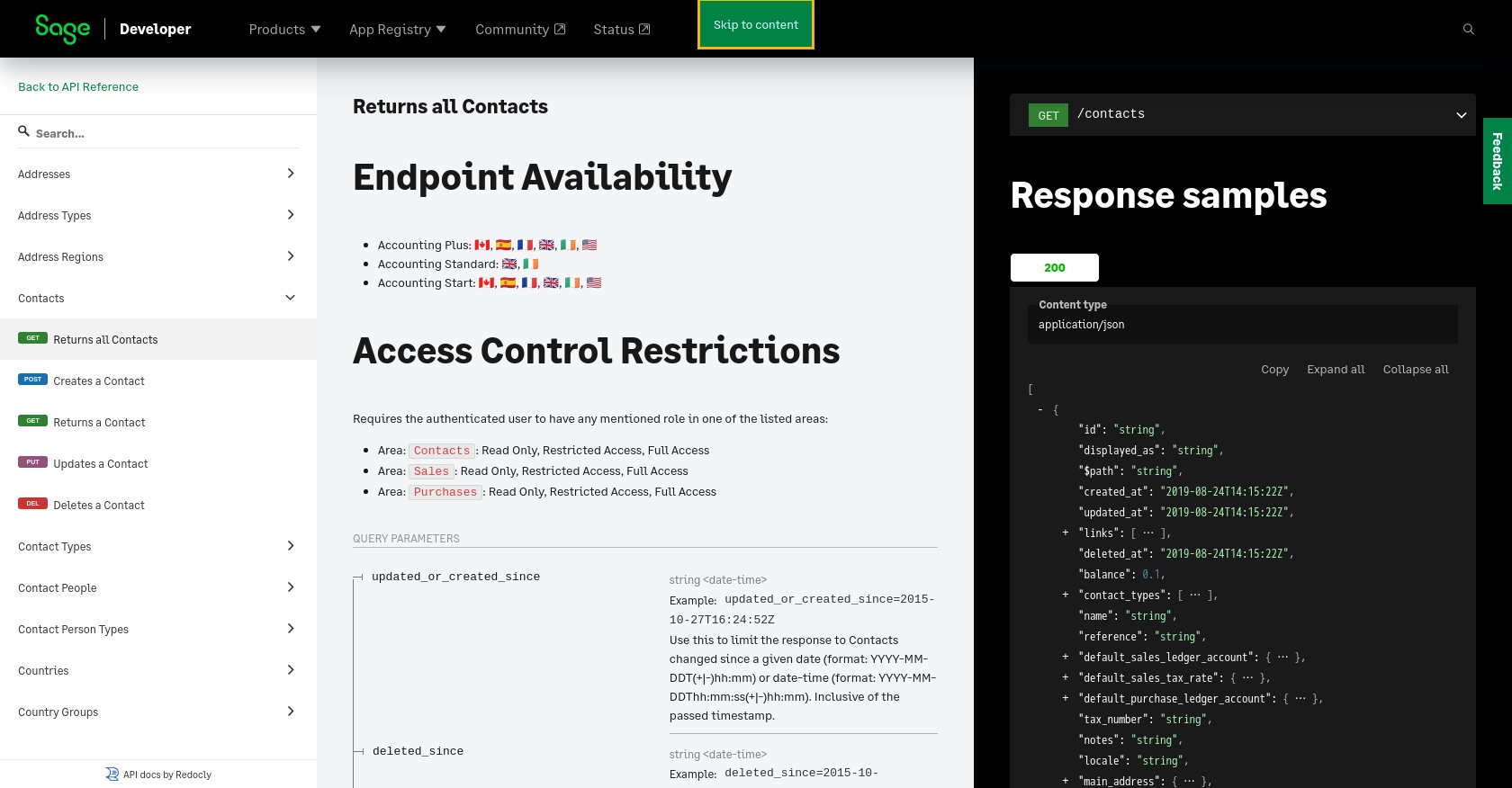
Conclusion and Best Practices for Using Sage Accounting API with PHP
Integrating with the Sage Accounting API using PHP can significantly enhance your business's financial management by automating vendor data retrieval and management. By following the steps outlined in this guide, you can efficiently set up OAuth authentication, make API calls, and handle responses and errors.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your Client ID and Client Secret securely, using environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be aware of any rate limits imposed by the Sage Accounting API. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data retrieved from the API is standardized and transformed as needed to fit your application's requirements.
- Monitor API Usage: Regularly monitor your API usage to optimize performance and identify any potential issues early.
By adhering to these best practices, you can ensure a robust and secure integration with the Sage Accounting API, enhancing your application's capabilities and providing a seamless experience for your users.
Streamline Your Integrations with Endgrate
If managing multiple integrations is becoming a challenge, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, allowing you to focus on your core product. Build once for each use case and enjoy an intuitive integration experience for your customers. Visit Endgrate to learn more about how it can simplify your integration process.
Read More
- https://endgrate.com/provider/sage
- https://developer.sage.com/accounting/quick-start/set-up-the-basics/
- https://developer.sage.com/accounting/quick-start/create-an-app/
- https://developer.sage.com/accounting/quick-start/upgrade-your-account/
- https://developer.sage.com/accounting/reference/contacts/#tag/Contacts/operation/getContacts
Ready to get started?