Using the Mailshake API to Get Leads in PHP
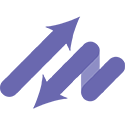
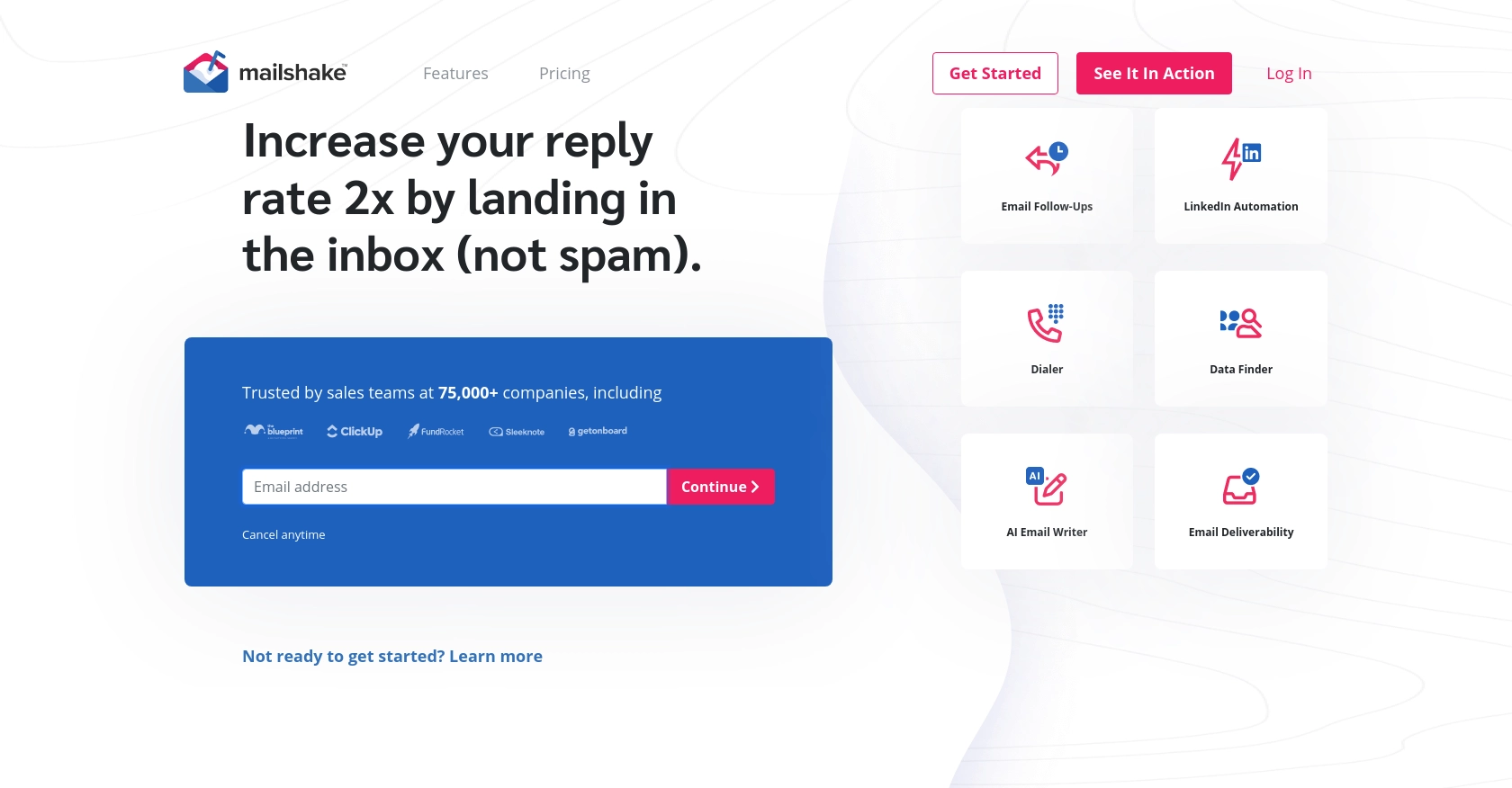
Introduction to Mailshake API for Lead Generation
Mailshake is a powerful sales engagement platform designed to streamline outreach efforts and enhance lead generation. It offers a suite of tools for email campaigns, social media engagement, and phone calls, making it an essential tool for sales teams aiming to boost their productivity and efficiency.
Integrating with the Mailshake API allows developers to automate and optimize lead management processes. For example, using the Mailshake API, you can programmatically retrieve leads generated from your email campaigns, enabling seamless integration with your CRM system and enhancing your sales workflow.
Setting Up Your Mailshake API Account for Lead Retrieval
Before you can start using the Mailshake API to retrieve leads, you need to set up your Mailshake account and obtain an API key. This key will allow you to authenticate your requests and interact with the Mailshake API seamlessly.
Creating a Mailshake Account
If you don't already have a Mailshake account, you can sign up for a free trial or choose a subscription plan that suits your needs. Visit the Mailshake website to create your account.
Generating Your Mailshake API Key
Once your account is set up, follow these steps to generate your API key:
- Log in to your Mailshake account.
- Navigate to the Extensions section in the dashboard.
- Select API from the menu.
- Click on Create API Key to generate a new key.
- Copy the API key and store it securely, as you'll need it to authenticate your API requests.
Authenticating with the Mailshake API Using an API Key
The Mailshake API uses simple API key-based authentication. You can include your API key in your requests in one of the following ways:
- As a query string parameter:
?apiKey=your_api_key
- In the request body as JSON:
{"apiKey": "your_api_key"}
- Via an HTTP Authorization header:
Authorization: Basic [base-64 encoded version of your api key]
Ensure you replace your_api_key
with the actual API key you generated.
For more information on authentication and API usage, refer to the Mailshake API documentation.
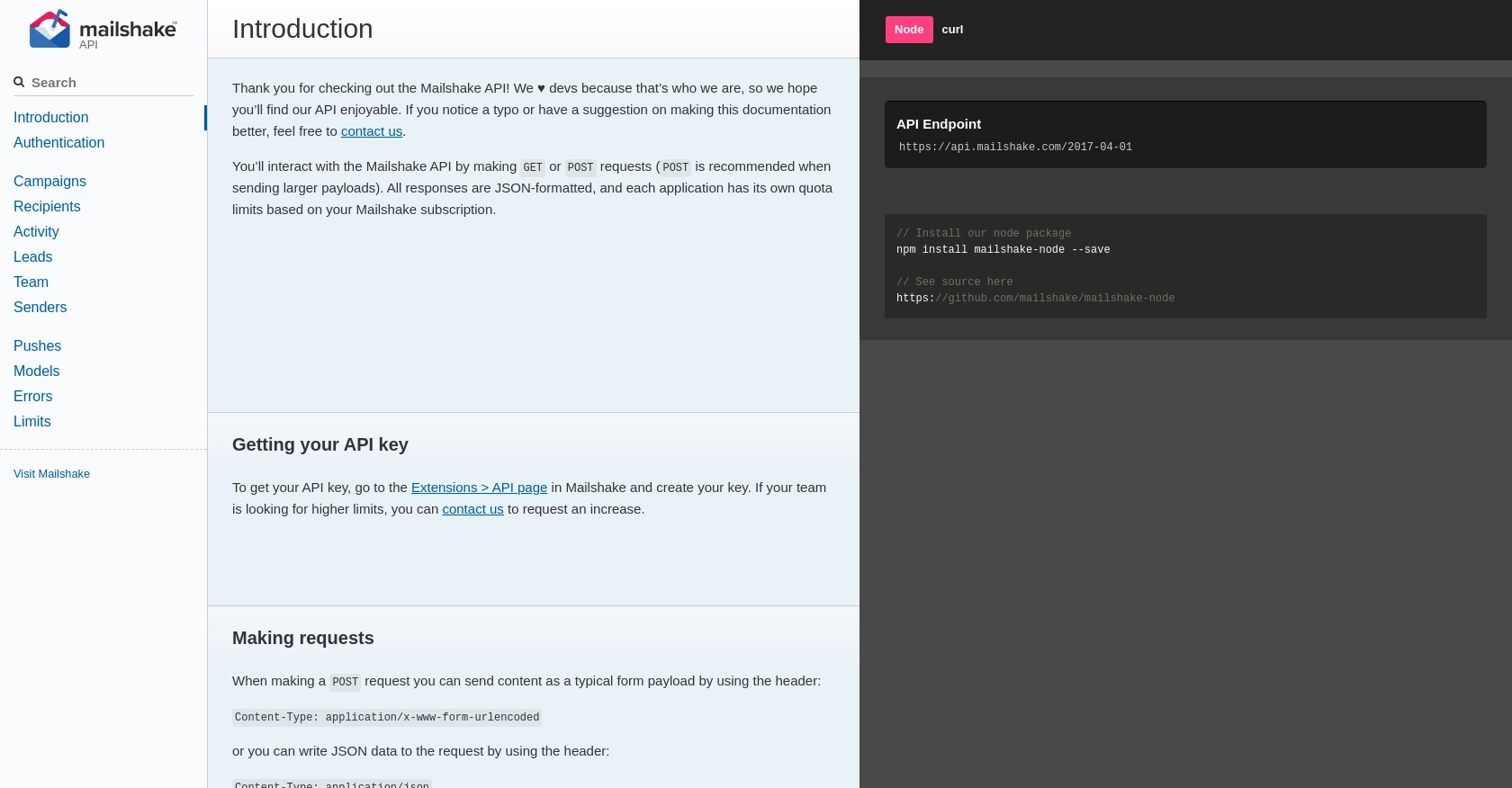
sbb-itb-96038d7
Making API Calls to Retrieve Leads Using Mailshake API in PHP
To interact with the Mailshake API and retrieve leads, you need to set up your PHP environment and make the necessary API calls. This section will guide you through the process of setting up PHP, installing dependencies, and executing API requests to fetch leads from Mailshake.
Setting Up Your PHP Environment for Mailshake API Integration
Before making API calls, ensure that your PHP environment is properly configured. You will need:
- PHP 7.4 or higher
- Composer for dependency management
To install Composer, follow the instructions on the Composer website.
Installing Required PHP Dependencies for Mailshake API
To make HTTP requests, you will need the Guzzle HTTP client. Install it using Composer with the following command:
composer require guzzlehttp/guzzle
Example Code to Retrieve Leads from Mailshake API
Once your environment is set up, you can use the following PHP code to retrieve leads from the Mailshake API:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$apiKey = 'your_api_key'; // Replace with your actual API key
try {
$response = $client->request('GET', 'https://api.mailshake.com/2017-04-01/leads/list', [
'headers' => [
'Authorization' => 'Basic ' . base64_encode($apiKey . ':')
],
'query' => [
'perPage' => 100 // Adjust the number of leads per page as needed
]
]);
$data = json_decode($response->getBody(), true);
foreach ($data['results'] as $lead) {
echo 'Lead ID: ' . $lead['id'] . ' - Email: ' . $lead['recipient']['emailAddress'] . "\n";
}
} catch (Exception $e) {
echo 'Error: ' . $e->getMessage();
}
Replace your_api_key
with the API key you generated earlier. This code initializes a Guzzle client, sets up the request with the necessary headers, and retrieves a list of leads. The results are then parsed and displayed.
Verifying Successful API Requests and Handling Errors
After running the code, verify that the leads are correctly retrieved by checking the output. If the request is successful, you should see a list of lead IDs and email addresses.
In case of errors, the Mailshake API provides specific error codes. For example, an invalid_api_key
error indicates an issue with your API key. Refer to the Mailshake API documentation for a complete list of error codes and their meanings.
Conclusion and Best Practices for Using Mailshake API in PHP
Integrating the Mailshake API into your PHP applications can significantly enhance your lead management processes by automating the retrieval and handling of leads. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate your requests, and interact with the Mailshake API to streamline your sales workflow.
Best Practices for Secure and Efficient Mailshake API Integration
- Secure Storage of API Keys: Always store your API keys securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limits: Be mindful of Mailshake's rate limits. Implement logic to handle the
limit_reached
error by retrying requests after the specified wait time. - Data Standardization: Ensure that the data retrieved from Mailshake is standardized before integrating it into your CRM or other systems.
- Error Handling: Implement robust error handling to manage API errors gracefully and log them for further analysis.
Enhance Your Integration Experience with Endgrate
While integrating with the Mailshake API can be straightforward, managing multiple integrations can become complex and time-consuming. Consider using Endgrate to simplify your integration processes. With Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations.
- Provide an easy and intuitive integration experience for your customers.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate.
Read More
Ready to get started?