Using the MongoDB API to Get Records (with Python examples)
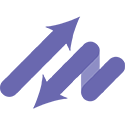
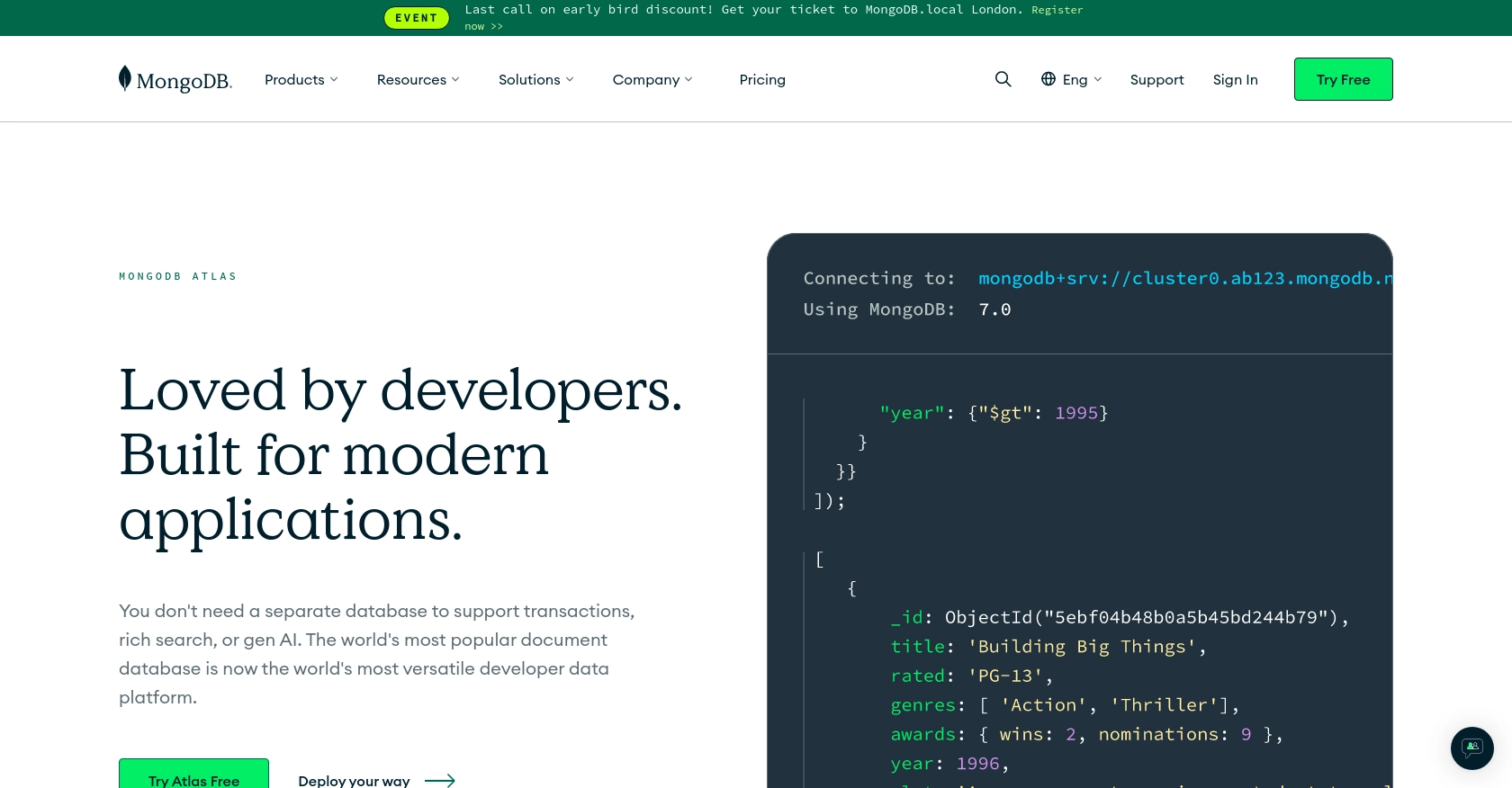
Introduction to MongoDB
MongoDB is a powerful, flexible NoSQL database platform that allows developers to store and manage data in a document-oriented format. Known for its scalability and high performance, MongoDB is widely used in modern applications that require handling large volumes of data efficiently.
Developers may want to integrate with MongoDB to leverage its robust data management capabilities. For example, using the MongoDB API, a developer can retrieve records from a database to display user-specific data in a web application, enhancing the user experience by providing personalized content.
Setting Up Your MongoDB Test/Sandbox Account
Before you can start interacting with MongoDB using its API, you need to set up a test or sandbox environment. MongoDB offers several options for developers to get started, including MongoDB Atlas, a fully managed cloud database service, and the Community Edition for local development.
Creating a MongoDB Atlas Free Tier Cluster
MongoDB Atlas provides a free tier that allows you to create a cloud-based MongoDB cluster. Follow these steps to set up your Atlas account:
- Visit the MongoDB Atlas website and sign up for a free account.
- Once registered, log in to your Atlas dashboard.
- Click on "Build a Cluster" and select the free tier option.
- Choose your preferred cloud provider and region, then click "Create Cluster."
- Wait for the cluster to be provisioned, which may take a few minutes.
Generating MongoDB API Credentials
To interact with your MongoDB cluster via the API, you'll need to generate API credentials:
- In the Atlas dashboard, navigate to the "Database Access" section.
- Click on "Add New Database User" and enter a username and password.
- Assign the user appropriate roles, such as "Read and Write to any database."
- Save the user credentials, as you'll need them to authenticate API requests.
Setting Up Network Access
Ensure your local machine can access the MongoDB cluster:
- Go to the "Network Access" section in the Atlas dashboard.
- Click on "Add IP Address" and enter your local machine's IP address.
- Alternatively, you can allow access from anywhere by adding "0.0.0.0/0," but this is not recommended for production environments.
Configuring MongoDB for API Access
MongoDB uses a custom authentication mechanism. Ensure your API client is configured to use the correct credentials:
- Install the necessary MongoDB drivers for Python, such as
pymongo
. - Use the connection string provided in the Atlas dashboard to connect to your cluster.
With your MongoDB test environment set up, you're ready to start making API calls to retrieve records and perform other operations.
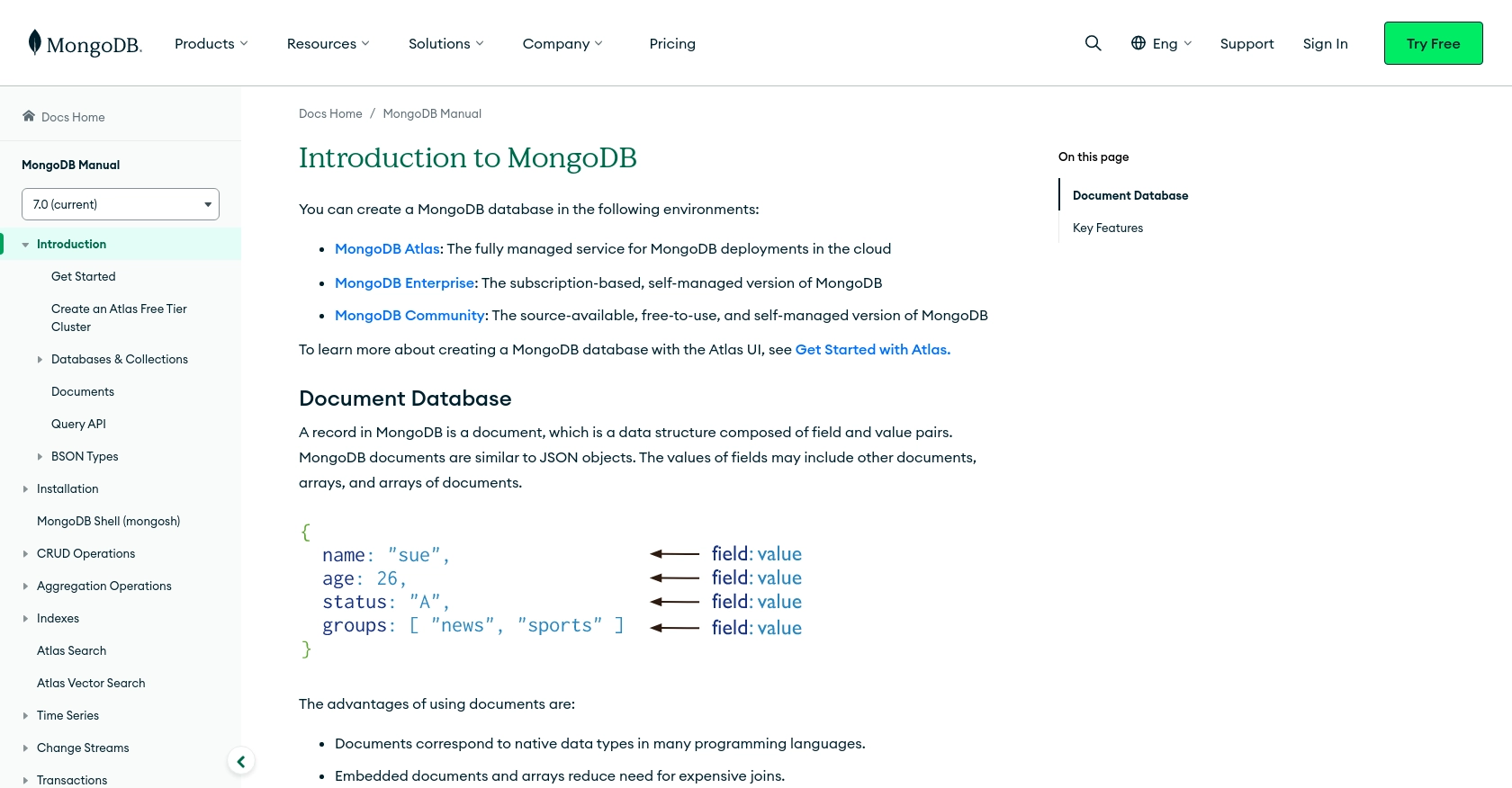
sbb-itb-96038d7
Making API Calls to Retrieve Records from MongoDB Using Python
To interact with MongoDB and retrieve records, you'll need to set up your Python environment and use the appropriate libraries. This section will guide you through the process of making API calls to MongoDB using Python, ensuring you can efficiently access your data.
Setting Up Python Environment for MongoDB API Integration
Before making API calls, ensure your Python environment is properly configured. You'll need Python 3.x and the pymongo
library to interact with MongoDB.
- Ensure Python 3.x is installed on your machine. You can download it from the official Python website.
- Install the
pymongo
library using pip:
pip install pymongo
Connecting to MongoDB Cluster Using Python
Once your environment is set up, you can connect to your MongoDB cluster using the connection string provided in the Atlas dashboard. Here's a sample code snippet to establish a connection:
from pymongo import MongoClient
# Replace the following with your MongoDB connection string
connection_string = "your_connection_string_here"
# Create a MongoClient to the running MongoDB instance
client = MongoClient(connection_string)
# Access the database
db = client['your_database_name']
# Access the collection
collection = db['your_collection_name']
Replace your_connection_string_here
, your_database_name
, and your_collection_name
with your actual MongoDB connection string, database name, and collection name.
Retrieving Records from MongoDB Collection
With the connection established, you can now retrieve records from your MongoDB collection. Here's how you can fetch and display records:
# Retrieve all documents from the collection
documents = collection.find()
# Iterate over the documents and print them
for document in documents:
print(document)
This code snippet retrieves all documents from the specified collection and prints each document to the console.
Handling Errors and Verifying Successful API Calls
When making API calls, it's crucial to handle potential errors and verify the success of your requests. Use try-except blocks to catch exceptions and ensure your connection and queries are successful:
try:
# Attempt to retrieve documents
documents = collection.find()
for document in documents:
print(document)
except Exception as e:
print(f"An error occurred: {e}")
By implementing error handling, you can diagnose issues and ensure your application runs smoothly.
Verifying Data in MongoDB Atlas
After executing your API calls, you can verify the data in your MongoDB Atlas dashboard. Navigate to your cluster, select the database and collection, and ensure the records match the output from your Python script.
For more detailed information on MongoDB and its features, refer to the MongoDB Manual.
Conclusion and Best Practices for MongoDB API Integration Using Python
Integrating with MongoDB using its API and Python can significantly enhance your application's data management capabilities. By following the steps outlined in this guide, you can efficiently retrieve and manipulate records from your MongoDB database, leveraging its powerful NoSQL features.
Best Practices for Secure and Efficient MongoDB API Usage
- Secure Credential Storage: Always store your MongoDB credentials securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Be mindful of any rate limits imposed by your MongoDB setup. Implement retry logic and exponential backoff strategies to handle API rate limits gracefully.
- Data Transformation and Standardization: Ensure that data retrieved from MongoDB is transformed and standardized to fit your application's requirements. This can help maintain consistency and improve data quality.
- Error Handling: Implement robust error handling to catch and manage exceptions, ensuring that your application can recover gracefully from unexpected issues.
Streamlining Integrations with Endgrate
While integrating with MongoDB directly can be rewarding, it can also be time-consuming and complex, especially when dealing with multiple integrations. Endgrate offers a streamlined solution by providing a unified API endpoint that connects to various platforms, including MongoDB. This allows you to focus on your core product while Endgrate handles the intricacies of integration.
By using Endgrate, you can save time and resources, build once for each use case, and provide an intuitive integration experience for your customers. Explore how Endgrate can simplify your integration needs by visiting Endgrate.
Read More
Ready to get started?