How to Send Messages (Channel) with the Slack API in Javascript
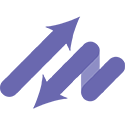
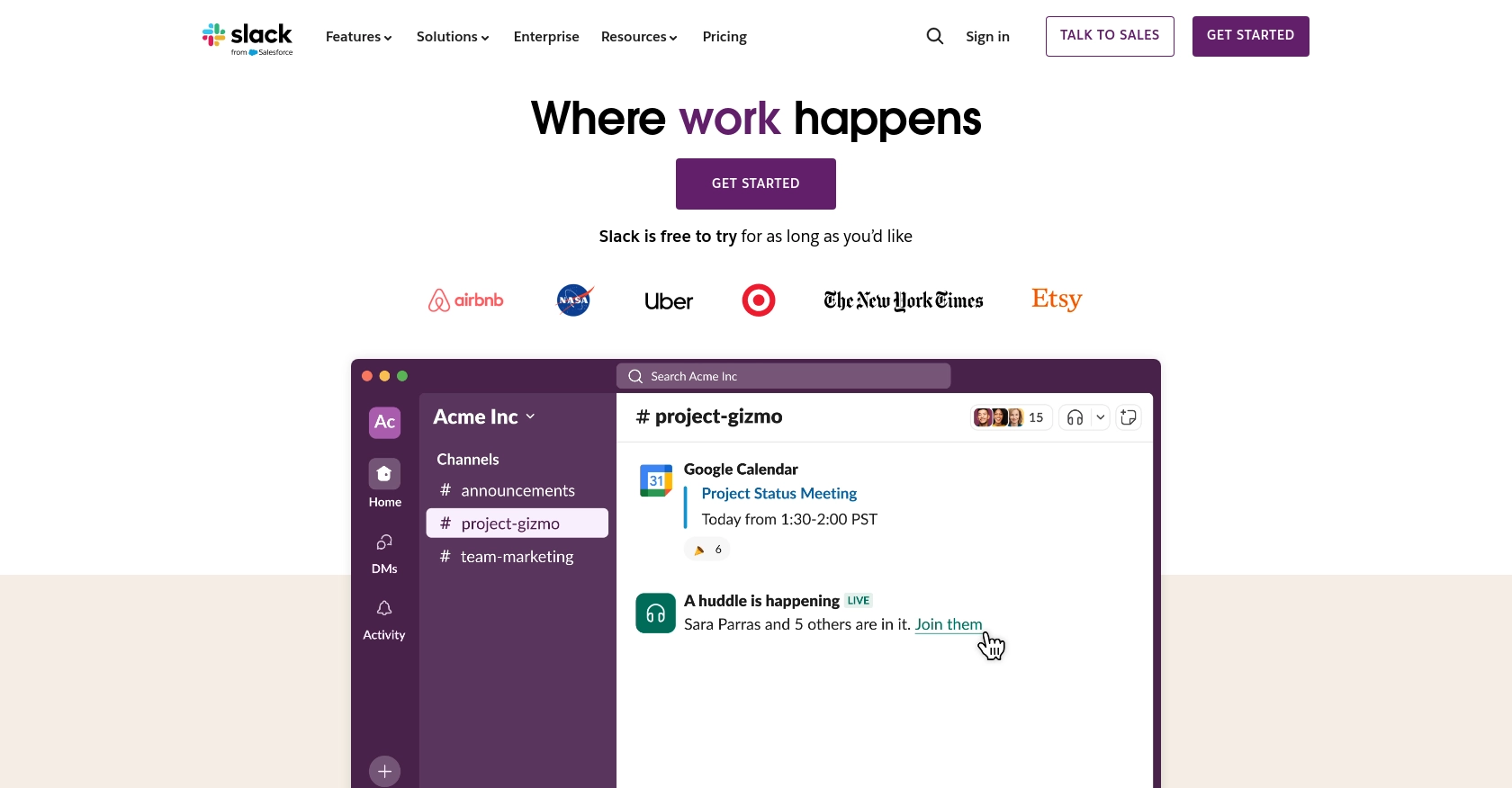
Introduction to Slack API Integration
Slack is a powerful collaboration platform that enables teams to communicate and work together more effectively. With features like channels, direct messaging, and integrations with various tools, Slack has become an essential part of many organizations' workflows.
Developers often need to integrate with Slack's API to automate tasks, enhance communication, or build custom applications. For example, you might want to send automated notifications to a Slack channel whenever a specific event occurs in your application, keeping your team informed in real-time.
This article will guide you through the process of using JavaScript to send messages to a Slack channel via the Slack API. You'll learn how to set up your Slack app, authenticate using OAuth, and make API calls to post messages, enabling seamless integration with your team's communication hub.
Setting Up Your Slack Test or Sandbox Account
Before you can start sending messages to a Slack channel using the Slack API, you'll need to set up a Slack app and configure it for testing. This involves creating a Slack app, requesting the necessary permissions, and obtaining an OAuth token for authentication. Follow these steps to get started:
Create a Slack App for API Integration
- Navigate to the Slack Apps page and click on "Create New App."
- Select "From scratch" and enter your app's name, such as "Message Bot."
- Choose the workspace where you want to develop your app and click "Create App."
Request Necessary OAuth Scopes for Slack API
To send messages to a Slack channel, your app needs specific permissions. You'll need to request these scopes:
- chat:write - Allows your app to send messages to channels.
- channels:read - Enables your app to retrieve a list of public channels.
- Go to the "OAuth & Permissions" section in your app settings.
- Under "Scopes," add the required scopes to the "Bot Token Scopes" section.
Install and Authorize Your Slack App
- Return to the "Basic Information" section and click "Install to Workspace."
- Follow the OAuth flow and click "Allow" to authorize your app.
- After installation, you'll receive an OAuth access token. Store this token securely, as it will be used to authenticate your API requests.
Invite Your App to a Slack Channel
Your app must be a member of the channel where you want to send messages. To invite your app:
- Open Slack and navigate to the desired channel.
- Use the slash command
/invite @YourAppName
to add your app to the channel.
With your Slack app set up and authorized, you're ready to start sending messages using the Slack API. In the next section, we'll cover how to make API calls using JavaScript to post messages to your Slack channel.
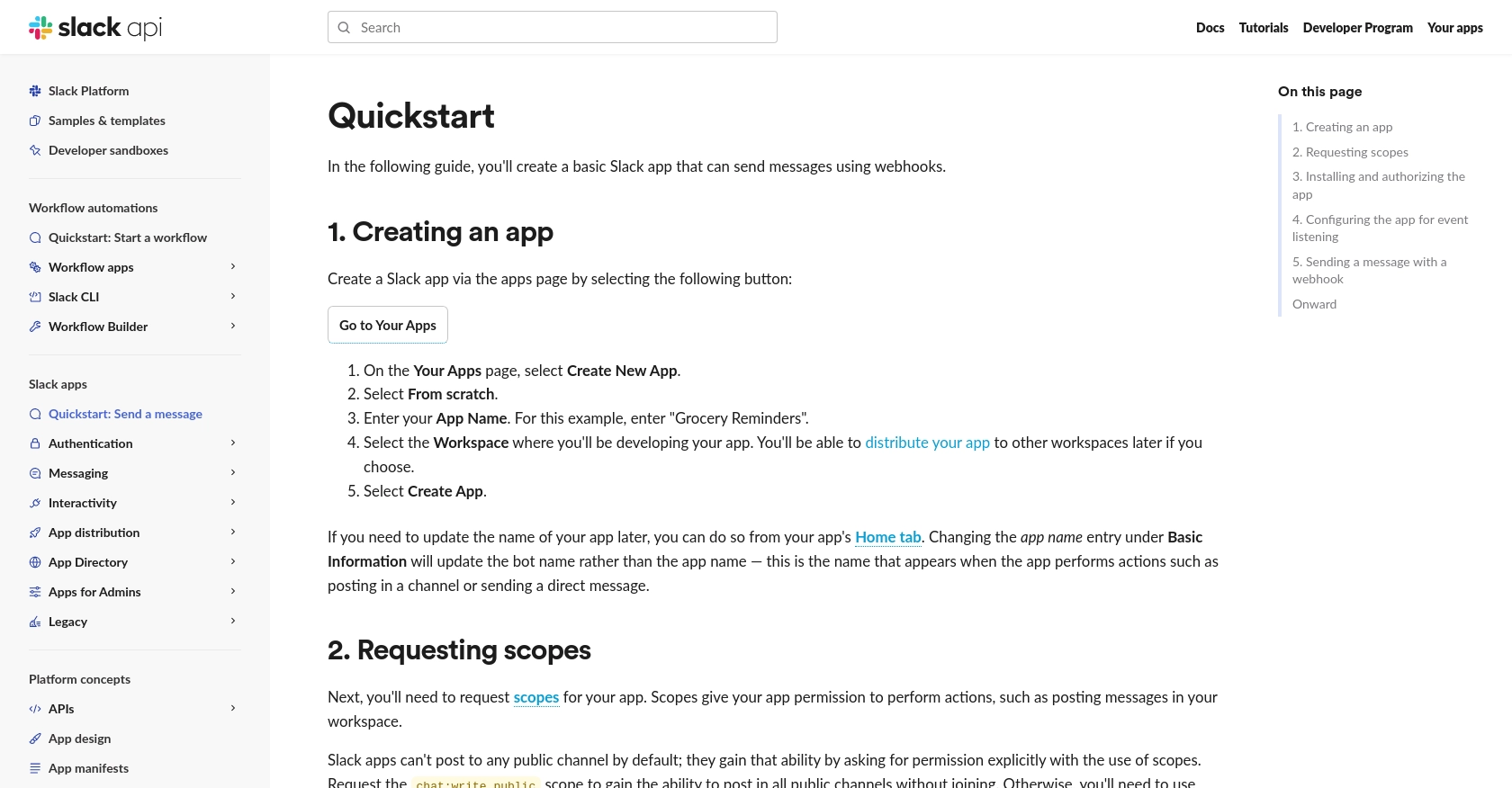
sbb-itb-96038d7
Making API Calls to Send Messages with Slack API in JavaScript
Now that your Slack app is set up and authorized, it's time to dive into making API calls to send messages to a Slack channel using JavaScript. This section will guide you through the necessary steps, including setting up your development environment, writing the code, and handling potential errors.
Setting Up Your JavaScript Environment for Slack API Integration
Before you begin coding, ensure you have the following prerequisites installed on your machine:
- Node.js (version 14 or higher)
- NPM (Node Package Manager)
Once you have Node.js and NPM installed, you can set up your project by creating a new directory and initializing it:
mkdir slack-message-bot
cd slack-message-bot
npm init -y
Next, install the Slack Web API client for Node.js:
npm install @slack/web-api
Writing JavaScript Code to Send Messages Using Slack API
With your environment ready, you can now write the JavaScript code to send messages to a Slack channel. Create a new file named sendMessage.js
and add the following code:
const { WebClient } = require('@slack/web-api');
// Initialize Slack Web API client with your OAuth token
const token = 'YOUR_OAUTH_ACCESS_TOKEN';
const web = new WebClient(token);
// Function to send a message to a Slack channel
async function sendMessage(channelId, text) {
try {
const result = await web.chat.postMessage({
channel: channelId,
text: text,
});
console.log('Message sent: ', result.ts);
} catch (error) {
console.error('Error sending message: ', error);
}
}
// Replace 'YOUR_CHANNEL_ID' with the actual channel ID
sendMessage('YOUR_CHANNEL_ID', 'Hello, Slack!');
Replace YOUR_OAUTH_ACCESS_TOKEN
with the OAuth token you obtained earlier, and YOUR_CHANNEL_ID
with the ID of the channel where you want to send the message.
Running the JavaScript Code to Post Messages on Slack
To execute your code and send a message, run the following command in your terminal:
node sendMessage.js
If successful, you should see the message "Hello, Slack!" appear in the specified Slack channel, along with a confirmation of the message timestamp in your terminal.
Handling Errors and Verifying Slack API Requests
When making API calls, it's crucial to handle potential errors gracefully. The Slack API provides error codes and messages that can help you diagnose issues. Common errors include invalid_auth, channel_not_found, and rate_limited.
To verify that your request succeeded, check the response object returned by the chat.postMessage
method. A successful request will have an ok
property set to true
.
For more information on error handling, refer to the Slack Web API documentation.
.webp)
Conclusion and Best Practices for Integrating Slack API with JavaScript
Integrating with the Slack API using JavaScript provides developers with powerful capabilities to enhance team communication and automate workflows. By following the steps outlined in this guide, you can effectively send messages to Slack channels, ensuring your team stays informed and connected.
Best Practices for Secure and Efficient Slack API Integration
- Securely Store OAuth Tokens: Always store your OAuth tokens securely, using environment variables or secure vaults, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of Slack's rate limits, which are typically 1 request per second per method. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Monitor API Usage: Regularly monitor your API usage and logs to identify any unusual activity or potential issues.
- Standardize Data Formats: Ensure consistent data formats when sending messages to maintain clarity and readability across your Slack channels.
Enhance Your Integration Strategy with Endgrate
While building custom integrations with Slack can be rewarding, it can also be time-consuming and complex. Endgrate offers a streamlined solution for managing integrations, allowing you to focus on your core product. With Endgrate, you can build once for each use case and enjoy an intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration process by visiting Endgrate and discover how companies like yours are leveraging its capabilities to scale their integrations efficiently.
Read More
Ready to get started?