Using the Zoom API to Get Meetings in Python
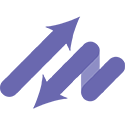
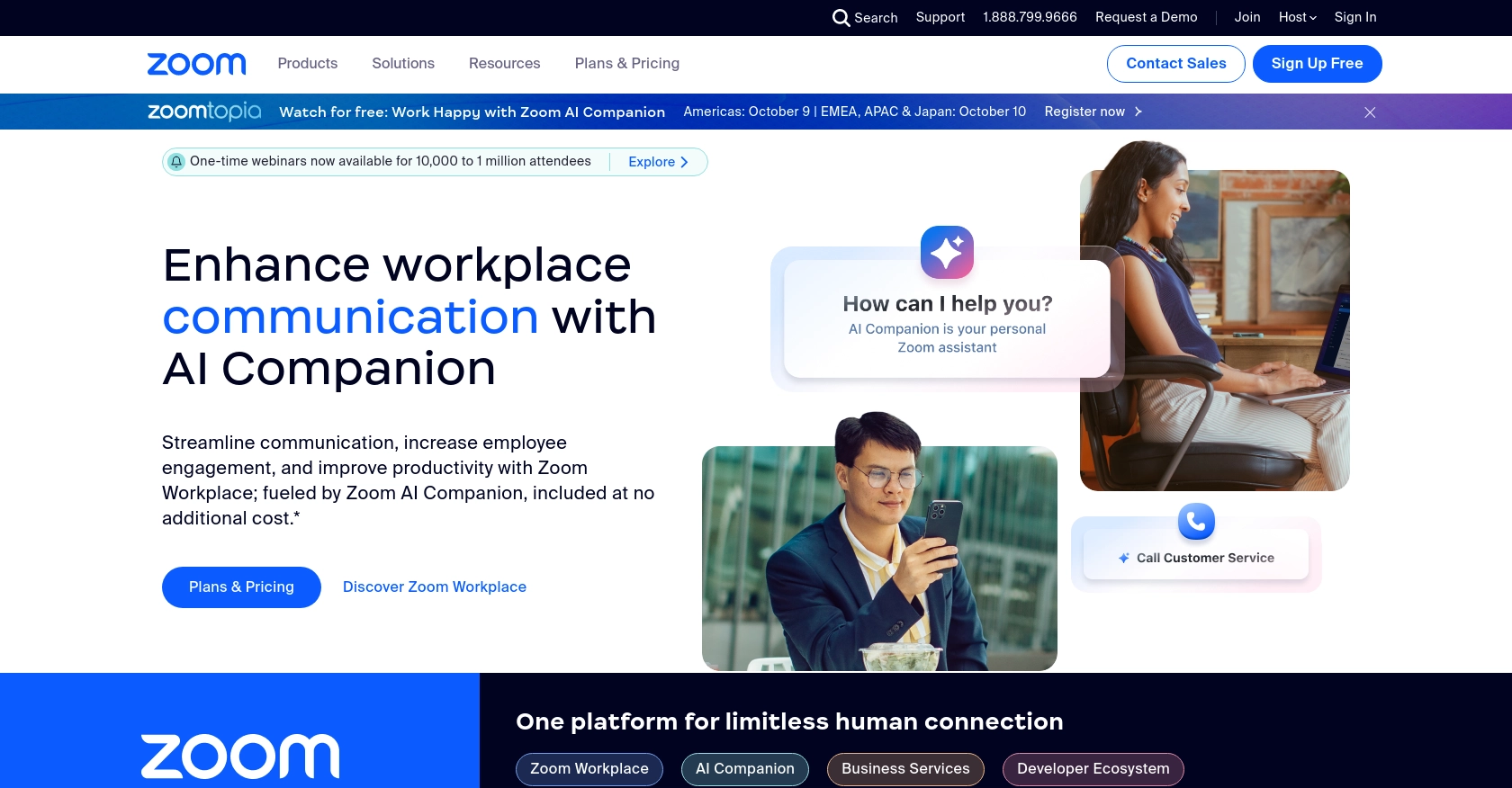
Introduction to Zoom API for Meeting Management
Zoom is a widely-used video conferencing platform that offers robust features for hosting virtual meetings, webinars, and collaborative sessions. Its API provides developers with the ability to integrate Zoom's functionalities into their own applications, enabling seamless meeting management and enhanced user experiences.
By connecting with the Zoom API, developers can automate tasks such as scheduling meetings, retrieving meeting details, and managing participants. For example, a developer might use the Zoom API to automatically fetch upcoming meetings and display them within a custom dashboard, streamlining the workflow for remote teams.
Setting Up Your Zoom Developer Account for API Access
Before you can start using the Zoom API to manage meetings, you'll need to set up a Zoom Developer account. This will allow you to create an app and obtain the necessary credentials for OAuth 2.0 authentication.
Creating a Zoom Developer Account
If you don't already have a Zoom Developer account, follow these steps to create one:
- Visit the Zoom App Marketplace.
- Click on "Sign In" and log in with your Zoom credentials. If you don't have a Zoom account, you'll need to create one first.
- Once logged in, navigate to the "Develop" dropdown and select "Build App."
Creating an OAuth App in Zoom
To interact with the Zoom API, you'll need to create an OAuth app. Follow these steps:
- In the Zoom App Marketplace, click on "Create" under the OAuth section.
- Choose "OAuth" as the app type and click "Create."
- Fill in the required information, such as the app name and company details.
- Under "Redirect URL for OAuth," enter the URL where users will be redirected after authentication.
- Set the "Scopes" to include permissions for accessing meeting information.
- Click "Continue" to save your app settings.
Obtaining Your Client ID and Client Secret
After creating your OAuth app, you'll receive a Client ID and Client Secret. These credentials are essential for authenticating API requests:
- Navigate to the "App Credentials" tab within your app's settings.
- Copy the Client ID and Client Secret and store them securely. You'll use these in your Python code to authenticate API requests.
Testing with a Zoom Sandbox Environment
Zoom provides a sandbox environment for testing API integrations without affecting live data:
- Use the sandbox environment to test API calls and ensure your integration works as expected.
- Refer to the Zoom API documentation for details on using the sandbox environment.
With your Zoom Developer account set up and your OAuth app configured, you're ready to start making API calls to manage meetings using Python.
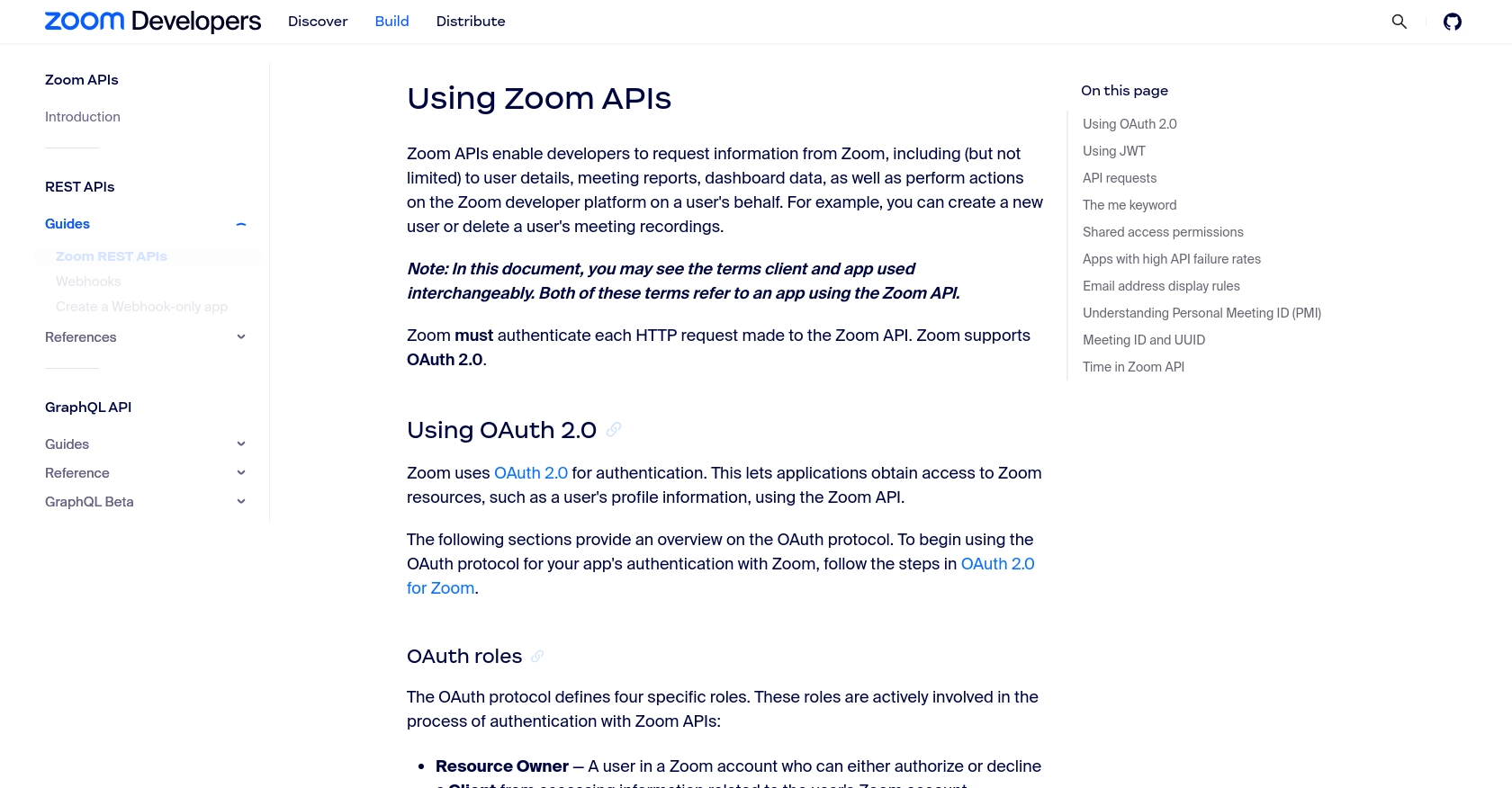
sbb-itb-96038d7
Making API Calls to Retrieve Zoom Meetings Using Python
To interact with the Zoom API and retrieve meeting details, you'll need to use Python. This section will guide you through the process of setting up your environment, writing the code, and handling responses effectively.
Setting Up Your Python Environment for Zoom API Integration
Before making API calls, ensure you have the following prerequisites installed:
- Python 3.11.1
- The Python package installer, pip
Install the requests
library, which is essential for making HTTP requests:
pip install requests
Writing Python Code to Fetch Zoom Meetings
Create a Python script named get_zoom_meetings.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://api.zoom.us/v2/users/me/meetings"
headers = {
"Authorization": "Bearer Your_Access_Token"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
meetings = response.json().get("meetings", [])
for meeting in meetings:
print(f"Meeting ID: {meeting['id']}, Topic: {meeting['topic']}")
else:
print(f"Failed to retrieve meetings: {response.status_code} - {response.text}")
Replace Your_Access_Token
with the access token obtained from your OAuth app setup.
Understanding the Zoom API Response and Error Handling
After executing the script, you should see a list of meetings with their IDs and topics. If the request fails, the script will print an error message with the status code and response text.
Common error codes include:
- 401 Unauthorized: Invalid or missing credentials.
- 403 Forbidden: Insufficient permissions.
- 429 Too Many Requests: Rate limit exceeded. Refer to the Zoom API rate limits documentation for more details.
Verifying API Call Success in Zoom Sandbox
To ensure your API call is successful, verify the retrieved meeting details in your Zoom sandbox environment. This helps confirm that your integration is functioning as expected without affecting live data.
By following these steps, you can effectively use Python to interact with the Zoom API and manage meetings programmatically. For more information, refer to the Zoom API documentation.
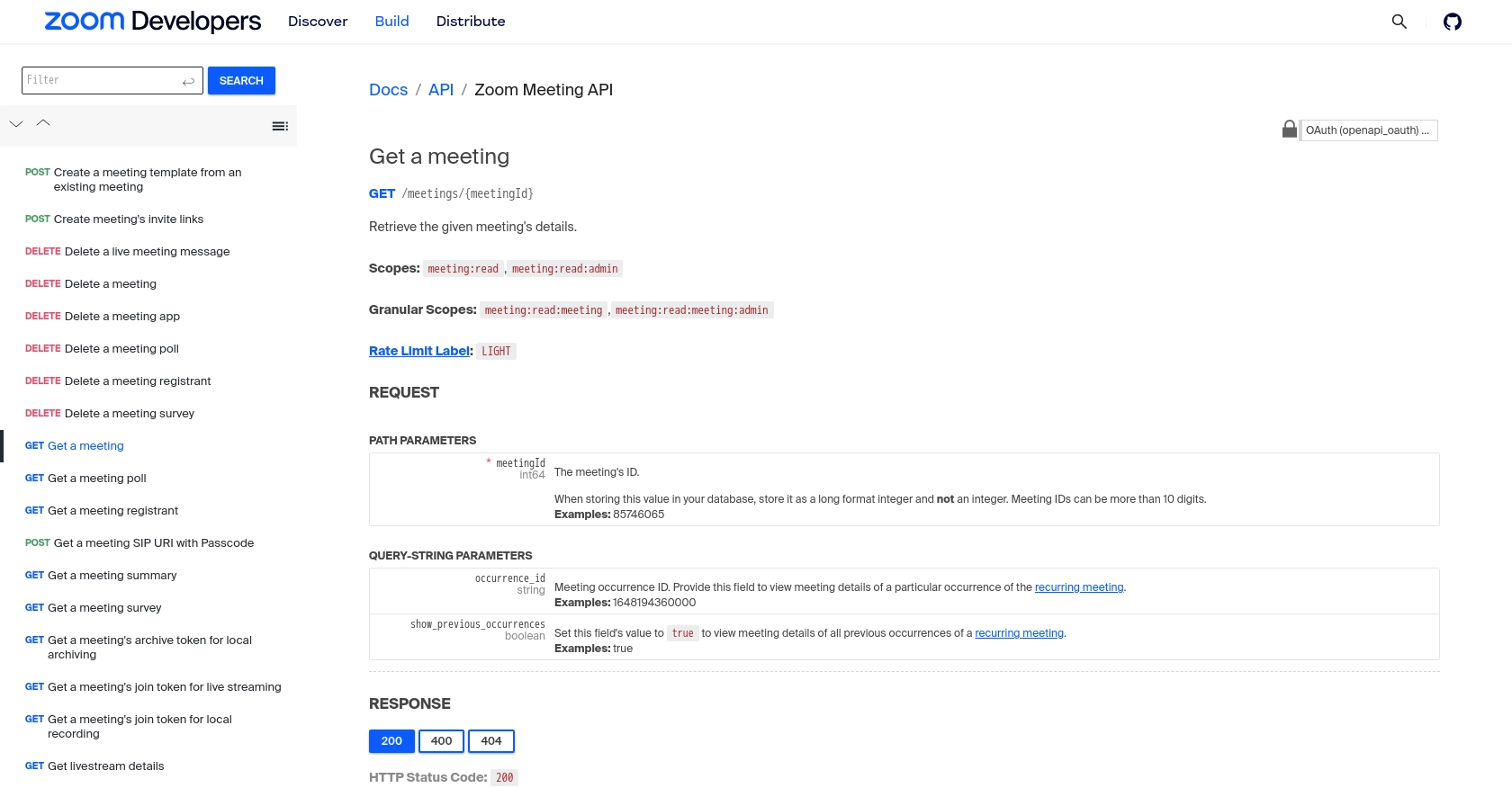
Conclusion and Best Practices for Zoom API Integration Using Python
Integrating with the Zoom API using Python offers developers a powerful way to automate meeting management and enhance user experiences. By following the steps outlined in this guide, you can efficiently retrieve and manage Zoom meetings, ensuring seamless integration within your applications.
Best Practices for Secure and Efficient Zoom API Usage
- Secure Storage of Credentials: Always store your Client ID, Client Secret, and Access Tokens securely. Consider using environment variables or secure vaults to protect sensitive information.
- Handling Rate Limits: Be mindful of Zoom's rate limits to avoid disruptions. Implement retry mechanisms and exponential backoff strategies to handle HTTP 429 errors gracefully. For more details, refer to the Zoom API rate limits documentation.
- Data Standardization: Ensure consistent data formats when integrating with other systems. This helps maintain data integrity and simplifies data processing across platforms.
Enhance Your Integration Strategy with Endgrate
While integrating with individual APIs like Zoom can be effective, managing multiple integrations can become complex and time-consuming. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Zoom. This allows you to focus on your core product while outsourcing integration complexities.
With Endgrate, you can build once for each use case, reducing development time and costs. Explore how Endgrate can streamline your integration efforts by visiting Endgrate today.
Read More
- https://endgrate.com/provider/zoom
- https://developers.zoom.us/docs/api/rest/using-zoom-apis/
- https://developers.zoom.us/docs/api/rest/pagination/
- https://developers.zoom.us/docs/api/rest/error-definitions/
- https://developers.zoom.us/docs/api/rest/rate-limits/
- https://developers.zoom.us/docs/api/rest/reference/zoom-api/methods/#operation/meeting
- https://developers.zoom.us/docs/api/rest/reference/zoom-api/methods/#operation/pastMeetingParticipants
- https://developers.zoom.us/docs/api/rest/reference/zoom-api/methods/#operation/recordingsList
Ready to get started?