Using the Webhook API to Push Data in Javascript
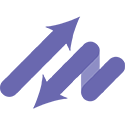
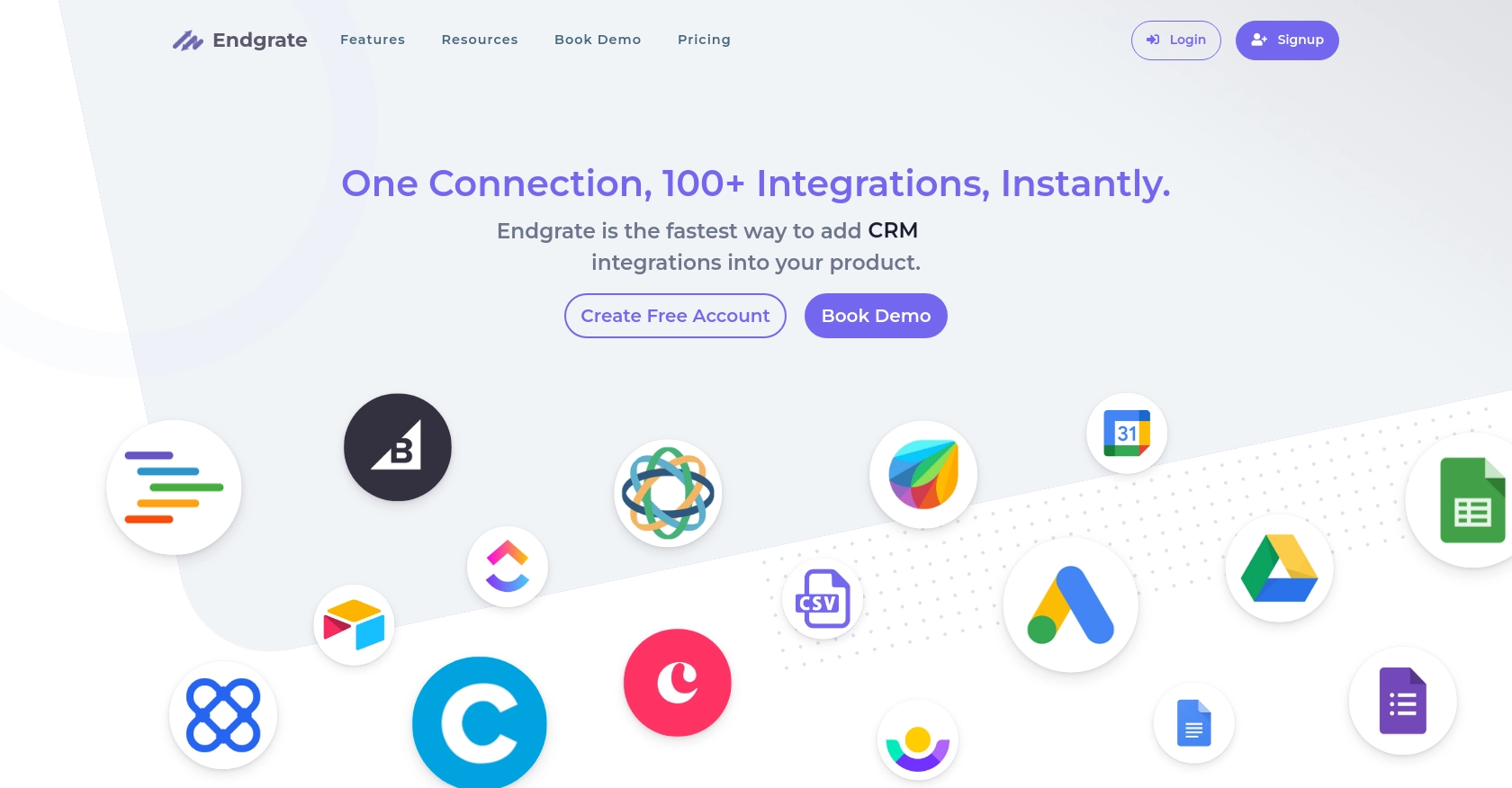
Introduction to Webhook API Integration
The Webhook API is a powerful tool that allows developers to automate and streamline data communication between different web services. By using webhooks, developers can push data to a specified URL, enabling real-time data transfer and integration across platforms.
Integrating with the Webhook API is essential for developers looking to enhance their applications' functionality by enabling seamless data exchange. For example, a developer might use the Webhook API to send real-time notifications from an e-commerce platform to a CRM system, ensuring that customer data is always up-to-date.
This article will guide you through the process of using JavaScript to interact with the Webhook API, providing detailed steps to efficiently push data and handle responses.
Setting Up Your Webhook API Test Environment
Before you begin integrating with the Webhook API using JavaScript, it's crucial to set up a test environment. This allows you to safely experiment with API calls without affecting live data. Fortunately, the Webhook API does not require authentication, simplifying the setup process.
Creating a Test URL for Webhook Data Transmission
To effectively test your integration, you'll need a URL to which you can send data. This can be a local server or a publicly accessible endpoint. Here’s how you can set up a simple test URL:
- Local Server: Use tools like ngrok to expose your local server to the internet. This is ideal for testing webhooks locally.
- Public Endpoint: Services like Webhook.site provide temporary URLs to receive and inspect webhook data.
Validating Webhook Configuration
Once you have your test URL, it's important to validate your webhook configuration. This ensures that the URL and parameters are correctly set before sending any data. Follow these steps:
- Ensure the URL is accessible and can receive POST requests.
- Send a test request to verify that the endpoint is functioning as expected.
- Check the response to confirm successful data reception.
With your test environment ready, you can proceed to implement the JavaScript code to interact with the Webhook API, ensuring a smooth and efficient integration process.
Making API Calls with JavaScript to Push Data Using Webhook API
To effectively interact with the Webhook API using JavaScript, you'll need to understand how to structure your API calls. This involves setting up the necessary environment, preparing the data, and handling responses efficiently. JavaScript provides a flexible and powerful way to manage these interactions, ensuring seamless data transfer.
Setting Up JavaScript Environment for Webhook API Integration
Before making API calls, ensure you have a suitable JavaScript environment. You can use Node.js for server-side scripting or a browser-based environment for client-side scripting. Ensure you have the latest version of Node.js installed if you're working server-side.
Installing Necessary Dependencies
For server-side JavaScript, you'll need to install the axios
library to simplify HTTP requests. Use the following command to install it:
npm install axios
Writing JavaScript Code to Send Data to Webhook API
Once your environment is set up, you can write the JavaScript code to send data to the Webhook API. Here's an example using axios
:
const axios = require('axios');
// Define the webhook URL
const webhookUrl = 'https://your-webhook-url.com';
// Prepare the data to be sent
const data = {
message: 'Hello, Webhook!',
timestamp: new Date().toISOString()
};
// Send a POST request to the webhook URL
axios.post(webhookUrl, data)
.then(response => {
console.log('Data sent successfully:', response.status);
})
.catch(error => {
console.error('Error sending data:', error.message);
});
Handling Responses and Errors in Webhook API Calls
Handling responses correctly is crucial for ensuring successful data transmission. The server will return a status code indicating the result of the request:
- Success: Status codes in the 200 range indicate successful data reception.
- Error: Codes outside the 200 range indicate an error. Implement error handling to log issues and retry if necessary.
Verifying Data Transmission to Webhook API
After sending data, verify that the transmission was successful by checking the response status and inspecting the data received at the endpoint. This ensures that your integration is functioning as expected.
By following these steps, you can efficiently push data to the Webhook API using JavaScript, enabling real-time data integration and enhancing your application's capabilities.
Conclusion and Best Practices for Webhook API Integration Using JavaScript
Integrating with the Webhook API using JavaScript offers a powerful way to automate data communication between web services. By following the steps outlined in this article, you can efficiently push data and handle responses, ensuring seamless integration and real-time data transfer.
Best Practices for Secure and Efficient Webhook API Integration
- Data Security: Always ensure that sensitive data is encrypted and transmitted securely. Use HTTPS for all webhook communications to protect data integrity.
- Error Handling: Implement robust error handling to manage failed requests. Log errors and consider implementing retry mechanisms to handle transient issues.
- Rate Limiting: Be mindful of any rate limits imposed by the webhook service. Implement throttling mechanisms to avoid exceeding these limits and ensure smooth operation.
- Data Validation: Validate data before sending it to the webhook to ensure it meets the expected format and requirements, reducing the risk of errors.
Enhancing Integration Capabilities with Endgrate
While building webhook integrations can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including webhooks. By using Endgrate, you can:
- Save time and resources by outsourcing integrations and focusing on your core product development.
- Build once for each use case instead of multiple times for different integrations, streamlining your development process.
- Offer an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate and discover the benefits of a unified integration approach.
Read More
Ready to get started?