Using the Zoho Books API to Create or Update Customers (with PHP examples)
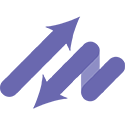
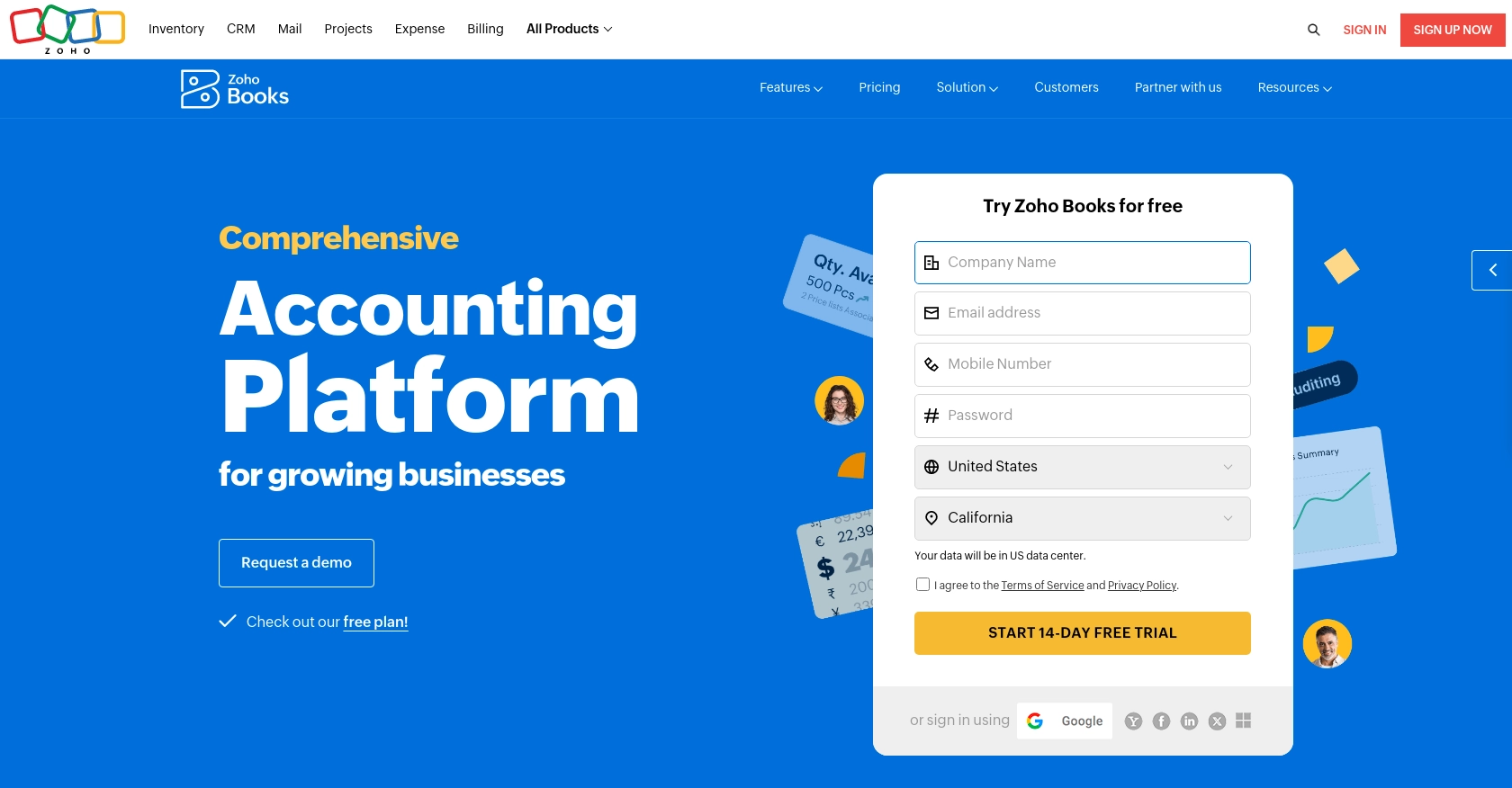
Introduction to Zoho Books API
Zoho Books is a comprehensive online accounting software designed to manage your finances, automate business workflows, and help you work collectively across departments. It offers a wide range of features including invoicing, expense tracking, and inventory management, making it a popular choice for businesses of all sizes.
Integrating with the Zoho Books API allows developers to automate and streamline accounting processes. For example, you can use the API to create or update customer records directly from your application, ensuring that your customer data is always up-to-date and reducing manual data entry.
This article will guide you through using PHP to interact with the Zoho Books API, specifically focusing on creating or updating customer records. By following this tutorial, you can enhance your application’s functionality and improve your business's operational efficiency.
Setting Up Your Zoho Books Test/Sandbox Account
Before you can start integrating with the Zoho Books API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data.
Creating a Zoho Books Account
If you don't already have a Zoho Books account, you can sign up for a free trial on the Zoho Books website. Follow the instructions to create your account. Once your account is set up, you can access the Zoho Books dashboard.
Registering Your Application for OAuth Authentication
Zoho Books uses OAuth 2.0 for authentication. To interact with the API, you'll need to register your application and obtain the necessary credentials.
- Go to the Zoho Developer Console.
- Click on Add Client ID to register your application.
- Fill in the required details, such as the application name and redirect URI.
- Upon successful registration, you'll receive a Client ID and Client Secret. Keep these credentials secure.
Generating the OAuth Tokens
With your Client ID and Client Secret, you can now generate the OAuth tokens needed to authenticate API requests.
- Redirect users to the following authorization URL, replacing the placeholders with your details:
- After the user consents, Zoho will redirect to your specified URI with a code parameter.
- Exchange this code for an access token by making a POST request to:
- The response will include an access_token and a refresh_token. Use the access token in your API requests.
https://accounts.zoho.com/oauth/v2/auth?scope=ZohoBooks.contacts.CREATE,ZohoBooks.contacts.UPDATE&client_id=YOUR_CLIENT_ID&response_type=code&redirect_uri=YOUR_REDIRECT_URI&access_type=offline
https://accounts.zoho.com/oauth/v2/token?code=YOUR_CODE&client_id=YOUR_CLIENT_ID&client_secret=YOUR_CLIENT_SECRET&redirect_uri=YOUR_REDIRECT_URI&grant_type=authorization_code
For more detailed information, refer to the Zoho Books OAuth documentation.
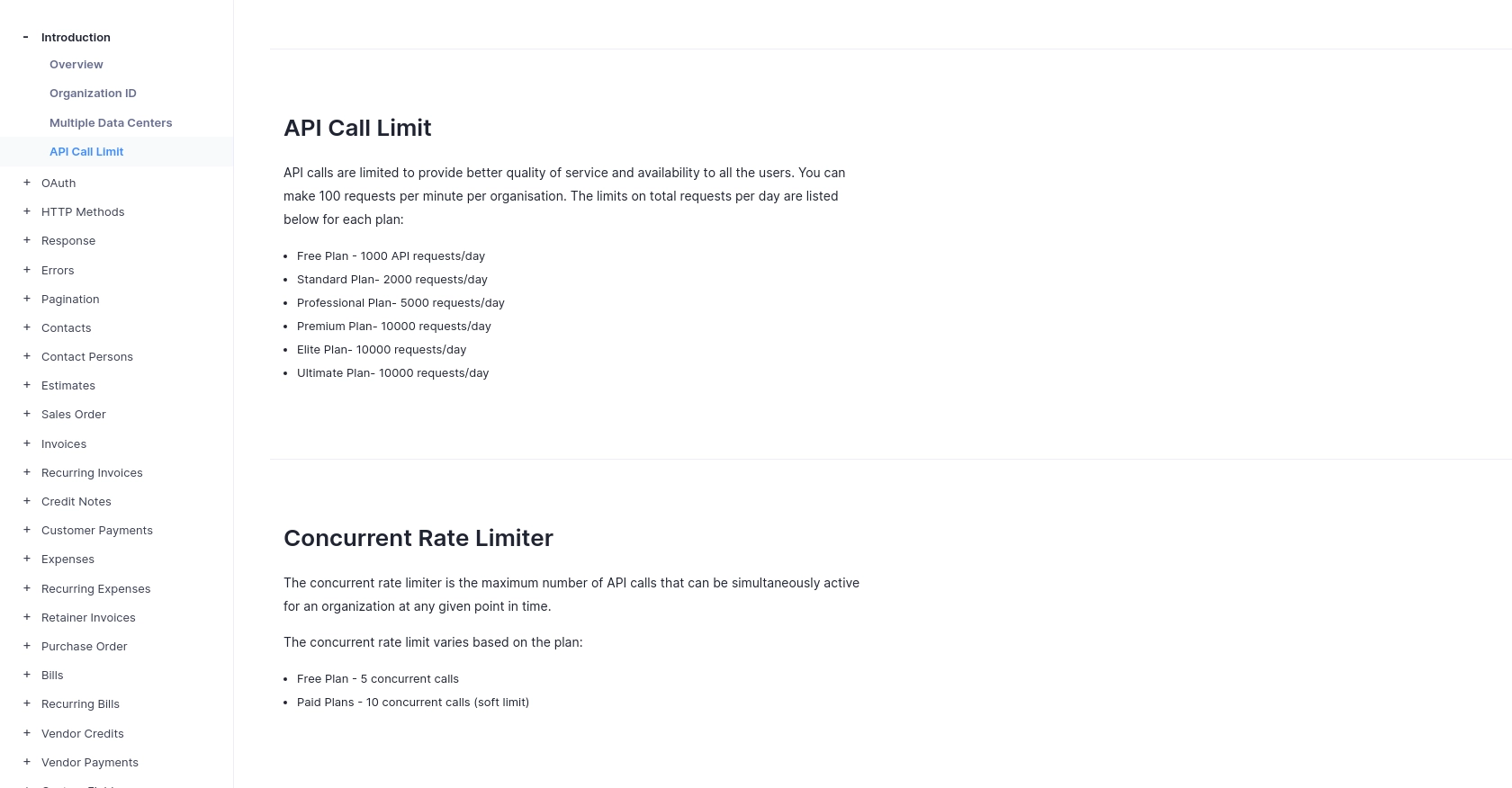
sbb-itb-96038d7
Making API Calls to Zoho Books for Creating or Updating Customers Using PHP
To interact with the Zoho Books API for creating or updating customer records, you'll need to use PHP to make HTTP requests. This section will guide you through the necessary steps, including setting up your PHP environment, writing the code, and handling responses.
Setting Up Your PHP Environment for Zoho Books API Integration
Before making API calls, ensure your PHP environment is properly configured. You'll need:
- PHP 7.4 or higher
- The
cURL
extension enabled for making HTTP requests
To install the cURL
extension, you can use the following command:
sudo apt-get install php-curl
Creating a Customer in Zoho Books Using PHP
To create a new customer, you'll need to send a POST request to the Zoho Books API endpoint. Here's an example of how to do this using PHP:
<?php
$accessToken = 'YOUR_ACCESS_TOKEN';
$organizationId = 'YOUR_ORGANIZATION_ID';
$url = "https://www.zohoapis.com/books/v3/contacts?organization_id=$organizationId";
$data = [
"contact_name" => "John Doe",
"company_name" => "Doe Enterprises",
"contact_type" => "customer"
];
$headers = [
"Authorization: Zoho-oauthtoken $accessToken",
"Content-Type: application/json"
];
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
?>
Replace YOUR_ACCESS_TOKEN
and YOUR_ORGANIZATION_ID
with your actual access token and organization ID. This script sends a POST request to create a customer named "John Doe" under "Doe Enterprises".
Updating a Customer in Zoho Books Using PHP
To update an existing customer, use a PUT request. Here's an example:
<?php
$accessToken = 'YOUR_ACCESS_TOKEN';
$organizationId = 'YOUR_ORGANIZATION_ID';
$contactId = 'CONTACT_ID_TO_UPDATE';
$url = "https://www.zohoapis.com/books/v3/contacts/$contactId?organization_id=$organizationId";
$data = [
"contact_name" => "John Doe Updated",
"company_name" => "Doe Enterprises Updated"
];
$headers = [
"Authorization: Zoho-oauthtoken $accessToken",
"Content-Type: application/json"
];
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_CUSTOMREQUEST, "PUT");
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data));
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
?>
Replace CONTACT_ID_TO_UPDATE
with the ID of the customer you wish to update. This script updates the customer's name and company details.
Handling API Responses and Errors
After making an API call, it's crucial to handle the response properly. Zoho Books uses HTTP status codes to indicate success or failure:
- 200: OK
- 201: Created
- 400: Bad Request
- 401: Unauthorized
- 404: Not Found
- 429: Rate Limit Exceeded
- 500: Internal Server Error
Check the response code and handle errors accordingly. For more details, refer to the Zoho Books Error Documentation.
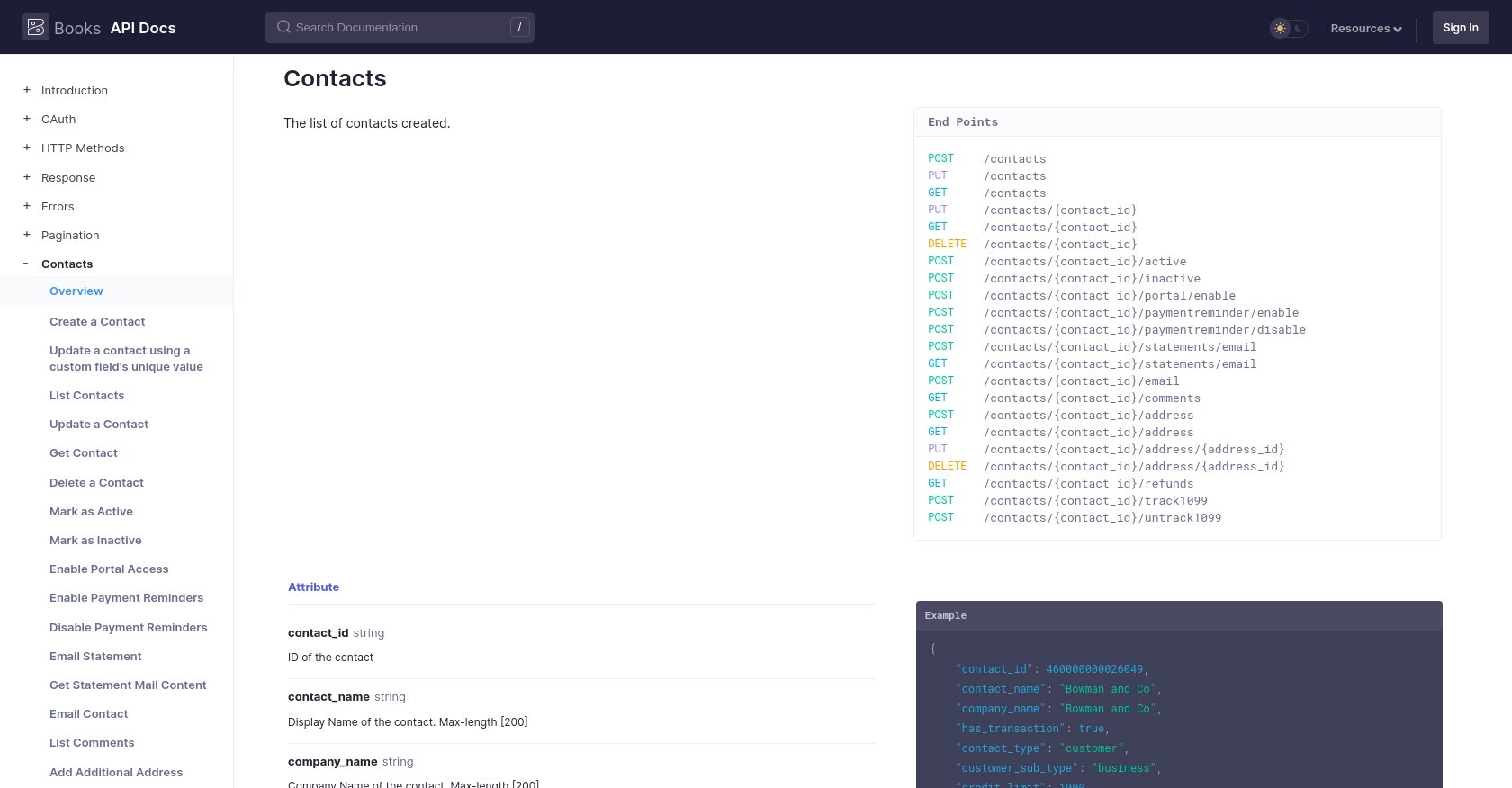
Best Practices for Using Zoho Books API in PHP Applications
When integrating with the Zoho Books API, it's essential to follow best practices to ensure security, efficiency, and scalability. Here are some recommendations:
- Securely Store Credentials: Always store your OAuth credentials, such as the Client ID, Client Secret, and tokens, securely. Use environment variables or a secure vault to prevent unauthorized access.
- Handle Rate Limiting: Zoho Books API has rate limits, such as 100 requests per minute per organization. Implement logic to handle rate limit responses (HTTP 429) by retrying requests after a delay. For more details, refer to the API Call Limit Documentation.
- Validate API Responses: Always check the HTTP status codes and response messages to handle errors gracefully. This ensures your application can recover from issues without crashing.
- Optimize Data Handling: Transform and standardize data fields to match your application's requirements. This helps maintain consistency and reduces errors during data processing.
Enhancing Your Integration with Endgrate
Building and maintaining integrations can be time-consuming and complex. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including Zoho Books. By using Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations.
- Provide an easy, intuitive integration experience for your customers.
Explore how Endgrate can simplify your integration needs by visiting Endgrate's website.
Conclusion on Zoho Books API Integration with PHP
Integrating with the Zoho Books API using PHP allows you to automate and streamline your accounting processes, enhancing your application's functionality. By following the steps outlined in this article, you can efficiently create or update customer records, ensuring your data remains accurate and up-to-date.
Remember to adhere to best practices for security and efficiency, and consider leveraging tools like Endgrate to further simplify your integration efforts. With these strategies, you can optimize your business operations and focus on delivering value to your customers.
Read More
- https://endgrate.com/provider/zohobooks
- https://www.zoho.com/books/api/v3/introduction/#api-call-limit
- https://www.zoho.com/books/api/v3/oauth/#overview
- https://www.zoho.com/books/api/v3/errors/#overview
- https://www.zoho.com/books/api/v3/pagination/#overview
- https://www.zoho.com/books/api/v3/contacts/#overview
Ready to get started?