How to Create or Update Leads with the Copper API in Javascript
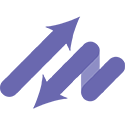
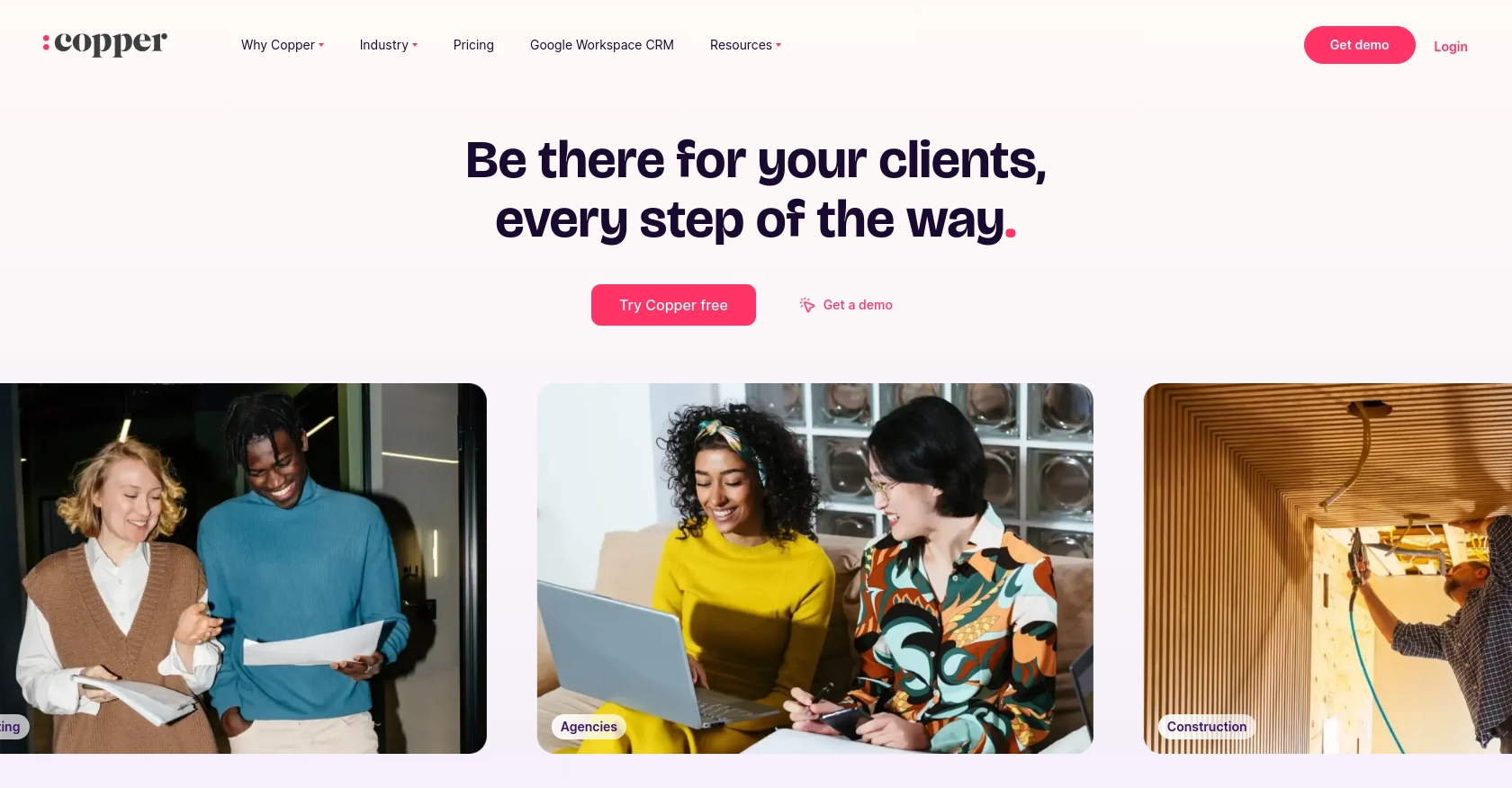
Introduction to Copper CRM API
Copper is a powerful CRM platform that seamlessly integrates with Google Workspace, providing businesses with a streamlined approach to managing customer relationships. Its intuitive interface and robust features make it an ideal choice for teams looking to enhance productivity and collaboration.
Developers may want to integrate with Copper's API to automate and optimize CRM processes, such as creating or updating leads. For example, you could use the Copper API to automatically update lead information from a web form submission, ensuring your sales team always has the most current data.
Setting Up a Copper CRM Test or Sandbox Account
Before diving into the integration with Copper's API, it's essential to set up a test or sandbox account. This allows developers to experiment with API calls without affecting live data, ensuring a safe environment for development and testing.
Creating a Copper CRM Account
If you don't already have a Copper account, you can sign up for a free trial on the Copper website. This trial provides access to most features, allowing you to explore the platform's capabilities.
- Visit the Copper website and click on "Try Free."
- Follow the prompts to create your account, providing necessary details such as your email and company information.
- Once your account is set up, you'll have access to the Copper dashboard.
Generating Copper API Keys for Authentication
Copper uses API keys for authentication, allowing secure access to its API. Follow these steps to generate your API keys:
- Log in to your Copper account and navigate to the "Settings" section.
- Under "Integrations," select "API Keys."
- Click on "Generate API Key" and provide a name for your key to identify it later.
- Copy the generated API key and secret. Store them securely, as you'll need them for API authentication.
Configuring OAuth for Copper API Access
For enhanced security, Copper supports OAuth-based authentication. To set this up:
- In the "API Keys" section, select "OAuth Apps."
- Click on "Create OAuth App" and fill in the required details, such as the app name and redirect URI.
- Once created, you'll receive a client ID and client secret. These are crucial for OAuth authentication.
Testing API Calls in Copper's Sandbox Environment
With your API keys or OAuth credentials ready, you can start testing API calls in Copper's sandbox environment:
- Use tools like Postman or cURL to make API requests to Copper's endpoints.
- Ensure you include the necessary headers, such as "Authorization" with your API key or OAuth token.
- Experiment with different API methods to create or update leads, verifying responses to ensure correct functionality.
By setting up a test environment and using Copper's API, developers can efficiently integrate CRM functionalities into their applications, enhancing productivity and data management.
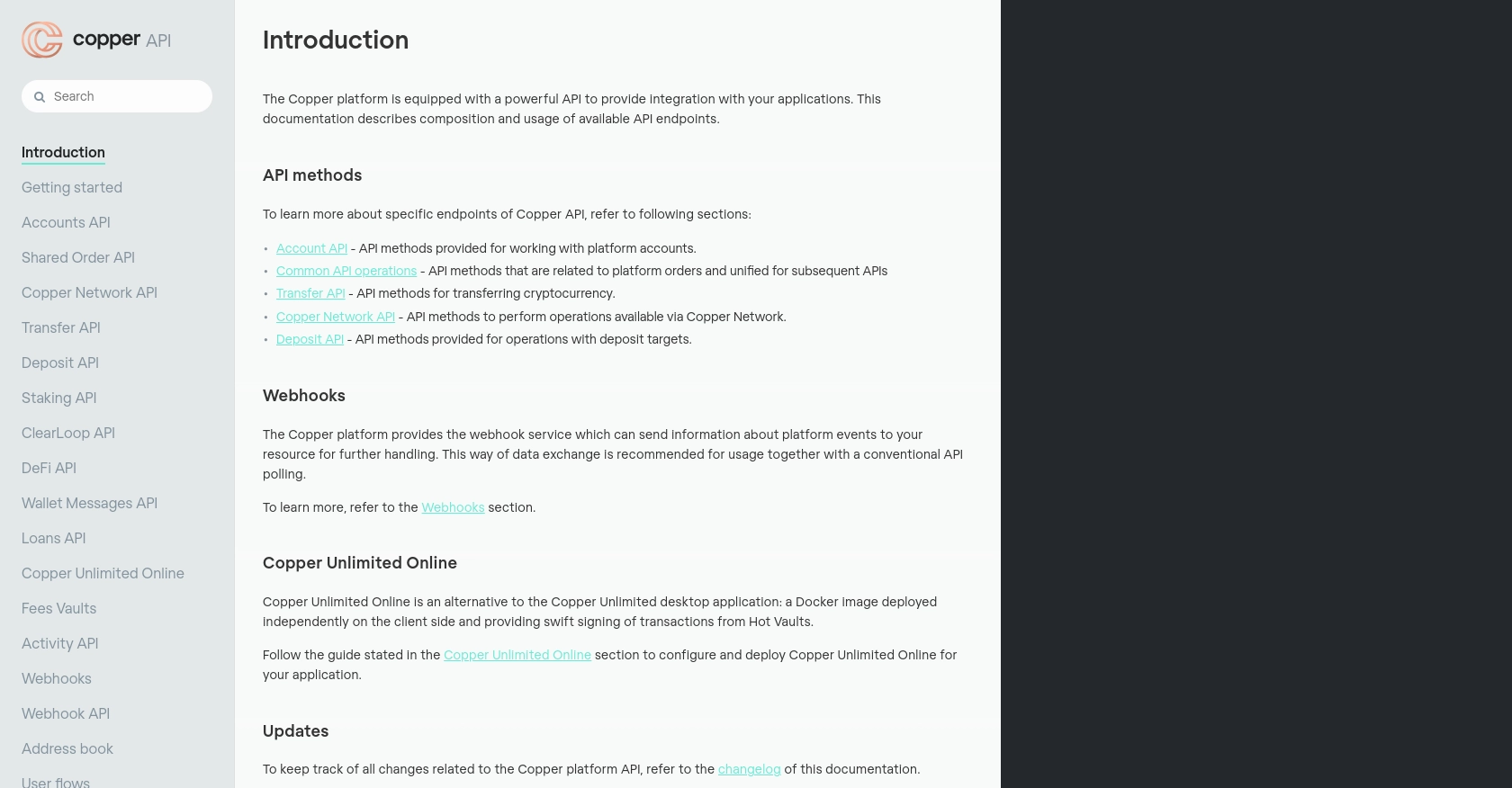
sbb-itb-96038d7
Making API Calls to Copper CRM Using JavaScript
To interact with Copper's API using JavaScript, you'll need to ensure you have the correct setup and dependencies. This section will guide you through the process of making API calls to create or update leads in Copper CRM.
Setting Up Your JavaScript Environment for Copper API Integration
Before making API calls, ensure your JavaScript environment is properly configured:
- Ensure you have Node.js installed on your machine. You can download it from the official Node.js website.
- Use npm (Node Package Manager) to install the necessary packages. For HTTP requests, you can use the popular
axios
library.
npm install axios
Creating and Updating Leads with Copper API in JavaScript
With your environment ready, you can now write JavaScript code to interact with Copper's API. Below is an example of how to create or update leads:
const axios = require('axios');
// Set your Copper API credentials
const apiKey = 'Your_API_Key';
const apiSecret = 'Your_API_Secret';
// Define the Copper API endpoint for leads
const endpoint = 'https://api.copper.com/developer_api/v1/leads';
// Function to create or update a lead
async function createOrUpdateLead(leadData) {
try {
const response = await axios.post(endpoint, leadData, {
headers: {
'Content-Type': 'application/json',
'X-PW-AccessToken': apiKey,
'X-PW-Application': 'developer_api',
'X-PW-UserEmail': 'your-email@example.com',
}
});
console.log('Lead created/updated successfully:', response.data);
} catch (error) {
console.error('Error creating/updating lead:', error.response.data);
}
}
// Sample lead data
const leadData = {
name: 'John Doe',
email: 'john.doe@example.com',
company_name: 'Example Corp',
phone_number: '123-456-7890'
};
// Call the function to create or update the lead
createOrUpdateLead(leadData);
Verifying API Call Success in Copper CRM
After executing the API call, verify the success of your request:
- Check the response data in your console to confirm the lead was created or updated successfully.
- Log in to your Copper CRM dashboard and navigate to the Leads section to see the changes reflected.
Handling Errors and Troubleshooting Copper API Requests
When making API calls, it's crucial to handle potential errors effectively:
- Use try-catch blocks in your JavaScript code to catch and log errors.
- Check the error response from Copper's API to understand the issue, such as authentication errors or invalid data.
By following these steps, you can efficiently integrate Copper CRM functionalities into your applications using JavaScript, enhancing your team's productivity and data management capabilities.
Conclusion: Best Practices for Integrating Copper CRM API with JavaScript
Integrating Copper CRM with JavaScript can significantly enhance your team's efficiency by automating lead management processes. Here are some best practices to ensure a smooth integration:
- Securely Store API Credentials: Always keep your API key and secret secure. Consider using environment variables or a secure vault to store sensitive information.
- Handle Rate Limiting: Copper API has rate limits. Implement logic to handle HTTP 429 errors by retrying requests after a delay.
- Standardize Data Formats: Ensure that data sent to Copper is standardized to prevent errors. Validate email formats, phone numbers, and other fields before making API calls.
- Monitor API Usage: Regularly monitor your API usage to ensure you stay within limits and maintain optimal performance.
By following these practices, you can effectively leverage Copper's API to streamline CRM processes, allowing your team to focus on core business activities.
Explore More with Endgrate for Seamless Integrations
For developers looking to simplify integration processes across multiple platforms, consider using Endgrate. With Endgrate, you can:
- Save time and resources by outsourcing complex integrations.
- Build once for each use case, reducing redundancy across different integrations.
- Provide an intuitive integration experience for your customers.
Visit Endgrate to learn more about how you can enhance your integration capabilities and focus on what truly matters—your core product.
Read More
Ready to get started?