Using the Active Campaign API to Get Users (with Javascript examples)
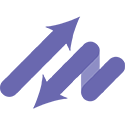
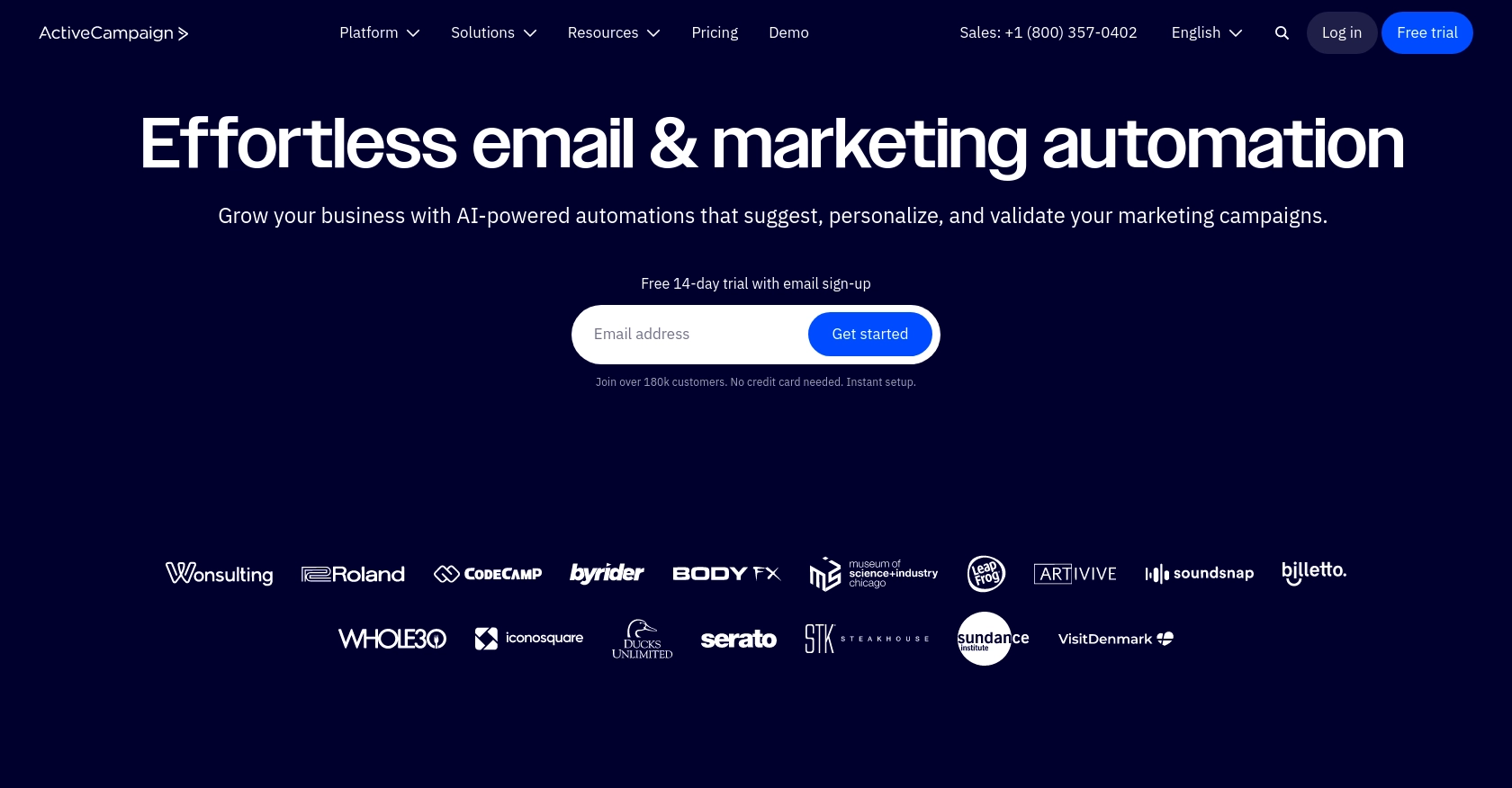
Introduction to Active Campaign API
Active Campaign is a powerful marketing automation platform that combines email marketing, CRM, and sales automation. It is designed to help businesses of all sizes engage with their customers more effectively and efficiently. With its robust set of features, Active Campaign enables businesses to create personalized customer experiences and streamline their marketing efforts.
Developers may want to integrate with Active Campaign's API to access and manage user data, automate marketing workflows, and enhance customer relationship management. For example, using the Active Campaign API, a developer can retrieve a list of users to analyze engagement metrics or synchronize user data with other applications.
Setting Up Your Active Campaign Test or Sandbox Account
Before you can start using the Active Campaign API, you'll need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting your live data.
Creating an Active Campaign Account
If you don't already have an Active Campaign account, you can sign up for a free trial on their website. This trial will give you access to all the features you need to test the API.
- Visit the Active Campaign website and click on "Try it Free."
- Fill out the registration form with your details and follow the instructions to create your account.
- Once your account is created, log in to access the dashboard.
Generating Your API Key
To authenticate API requests, you'll need an API key. Follow these steps to generate your API key:
- Log in to your Active Campaign account and navigate to the "Settings" page.
- Click on the "Developer" tab to find your API key.
- Copy the API key and keep it secure. You'll use this key to authenticate your API requests.
For more details, refer to the Active Campaign authentication documentation.
Understanding the Base URL
Each Active Campaign account has a unique base URL for API access. You can find your base URL in the "Developer" tab under "Settings." The URL format is typically https://youraccountname.api-us1.com/api/3/
. Ensure you use the correct base URL for your account.
For additional information, see the Active Campaign base URL documentation.
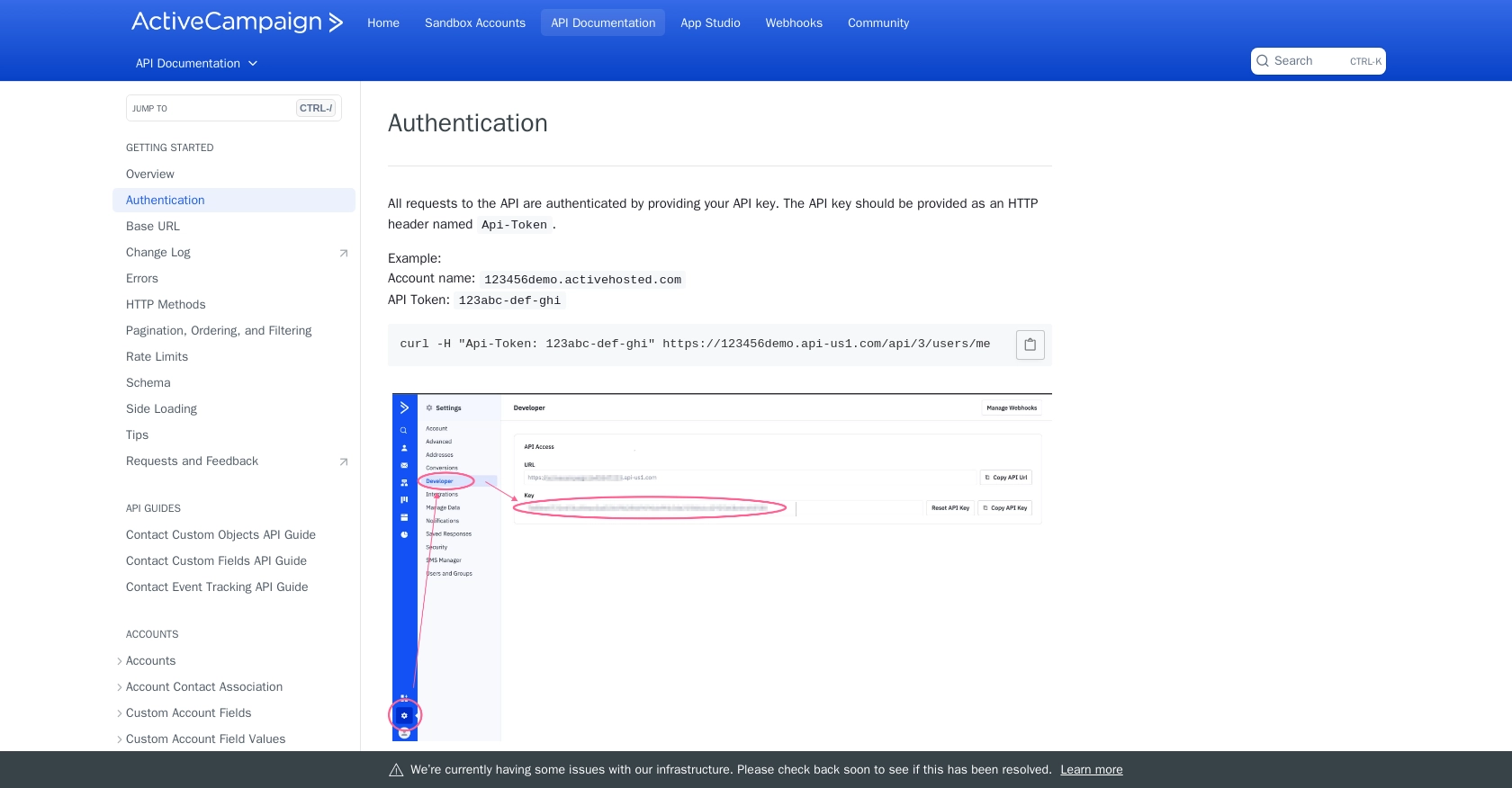
sbb-itb-96038d7
Making an API Call to Retrieve Users with Active Campaign API Using JavaScript
Prerequisites for Using JavaScript with Active Campaign API
To follow this tutorial, ensure you have the following installed on your machine:
- Node.js (version 14 or later)
- NPM (Node Package Manager)
Additionally, you'll need the axios
library to make HTTP requests. Install it using the following command:
npm install axios
Setting Up the JavaScript Environment for Active Campaign API
Create a new JavaScript file named getActiveCampaignUsers.js
and open it in your preferred code editor. This file will contain the code to interact with the Active Campaign API.
Writing the JavaScript Code to Fetch Users from Active Campaign
Add the following code to your getActiveCampaignUsers.js
file:
const axios = require('axios');
// Set the API endpoint and headers
const baseURL = 'https://youraccountname.api-us1.com/api/3/users';
const apiKey = 'Your_API_Key'; // Replace with your actual API key
// Configure the request headers
const headers = {
'Api-Token': apiKey,
'Accept': 'application/json'
};
// Function to fetch users
async function fetchUsers() {
try {
const response = await axios.get(baseURL, { headers });
const users = response.data.users;
// Display the retrieved users
users.forEach(user => {
console.log(`Username: ${user.username}, Email: ${user.email}`);
});
} catch (error) {
console.error('Error fetching users:', error.response ? error.response.data : error.message);
}
}
// Execute the function
fetchUsers();
Replace Your_API_Key
with the API key you obtained from the Active Campaign settings.
Running the JavaScript Code to Retrieve Users
To execute the code, open your terminal and run the following command:
node getActiveCampaignUsers.js
You should see a list of users printed in the console, similar to the following output:
Username: admin, Email: johndoe@activecampaign.com
Username: janedoe, Email: janedoe@activecampaign.com
Verifying the API Call Success in Active Campaign
To verify that the API call was successful, log in to your Active Campaign account and navigate to the "Users" section. Ensure that the users listed in your console output match those in your Active Campaign account.
Handling Errors and Understanding Error Codes
In the code above, errors are caught and logged to the console. Common error codes include:
- 400 Bad Request: The request was invalid or cannot be served.
- 401 Unauthorized: Authentication failed or user does not have permissions for the requested operation.
- 404 Not Found: The requested resource could not be found.
For more details on error handling, refer to the Active Campaign API documentation.
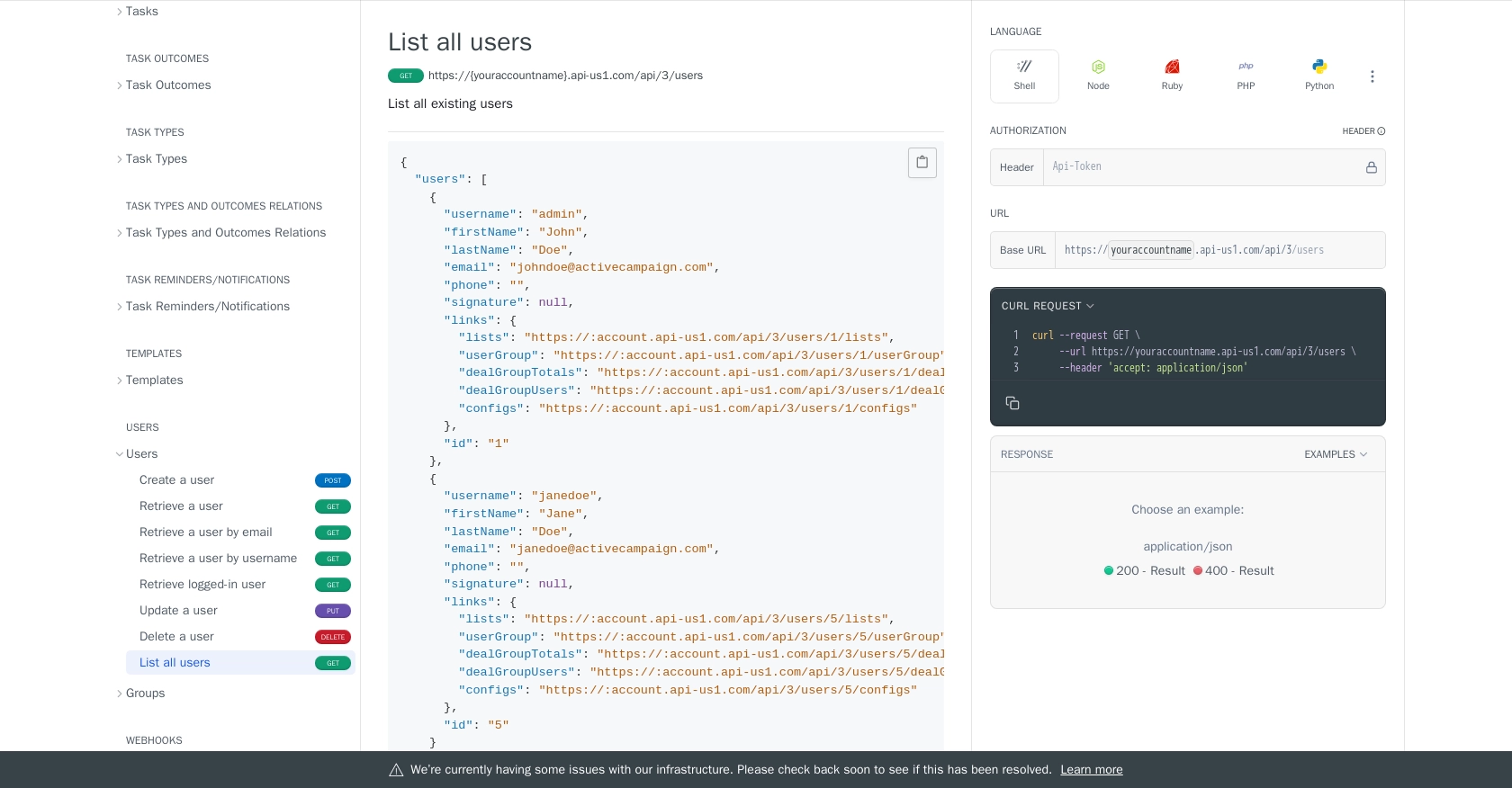
Best Practices for Using Active Campaign API with JavaScript
When working with the Active Campaign API, it's crucial to follow best practices to ensure security, efficiency, and maintainability of your integration. Here are some key recommendations:
- Secure Your API Key: Store your API key securely and avoid exposing it in client-side code. Consider using environment variables to manage sensitive information.
- Handle Rate Limits: Active Campaign's API has a rate limit of 5 requests per second per account. Implement logic to handle rate limiting gracefully, such as retrying requests after a delay. For more details, refer to the rate limits documentation.
- Manage Error Responses: Implement comprehensive error handling to manage different HTTP status codes and provide meaningful feedback to users or logs.
- Optimize Data Handling: Consider using pagination to handle large datasets efficiently. Use query parameters like
limit
andoffset
to control the amount of data retrieved per request.
Enhance Your Integration Experience with Endgrate
Building and maintaining integrations can be time-consuming and complex. With Endgrate, you can streamline your integration process by leveraging a unified API that connects to multiple platforms, including Active Campaign. This allows you to focus on your core product while Endgrate handles the intricacies of integration.
Endgrate offers a seamless integration experience, enabling you to build once for each use case and deploy across various platforms effortlessly. Explore how Endgrate can save you time and resources by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/activecampaign
- https://developers.activecampaign.com/reference/authentication
- https://developers.activecampaign.com/reference/url
- https://developers.activecampaign.com/reference/pagination
- https://developers.activecampaign.com/reference/rate-limits
- https://developers.activecampaign.com/reference/list-all-users
Ready to get started?