How to Get Custom Objects with the Hubspot API in PHP
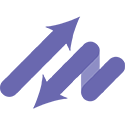
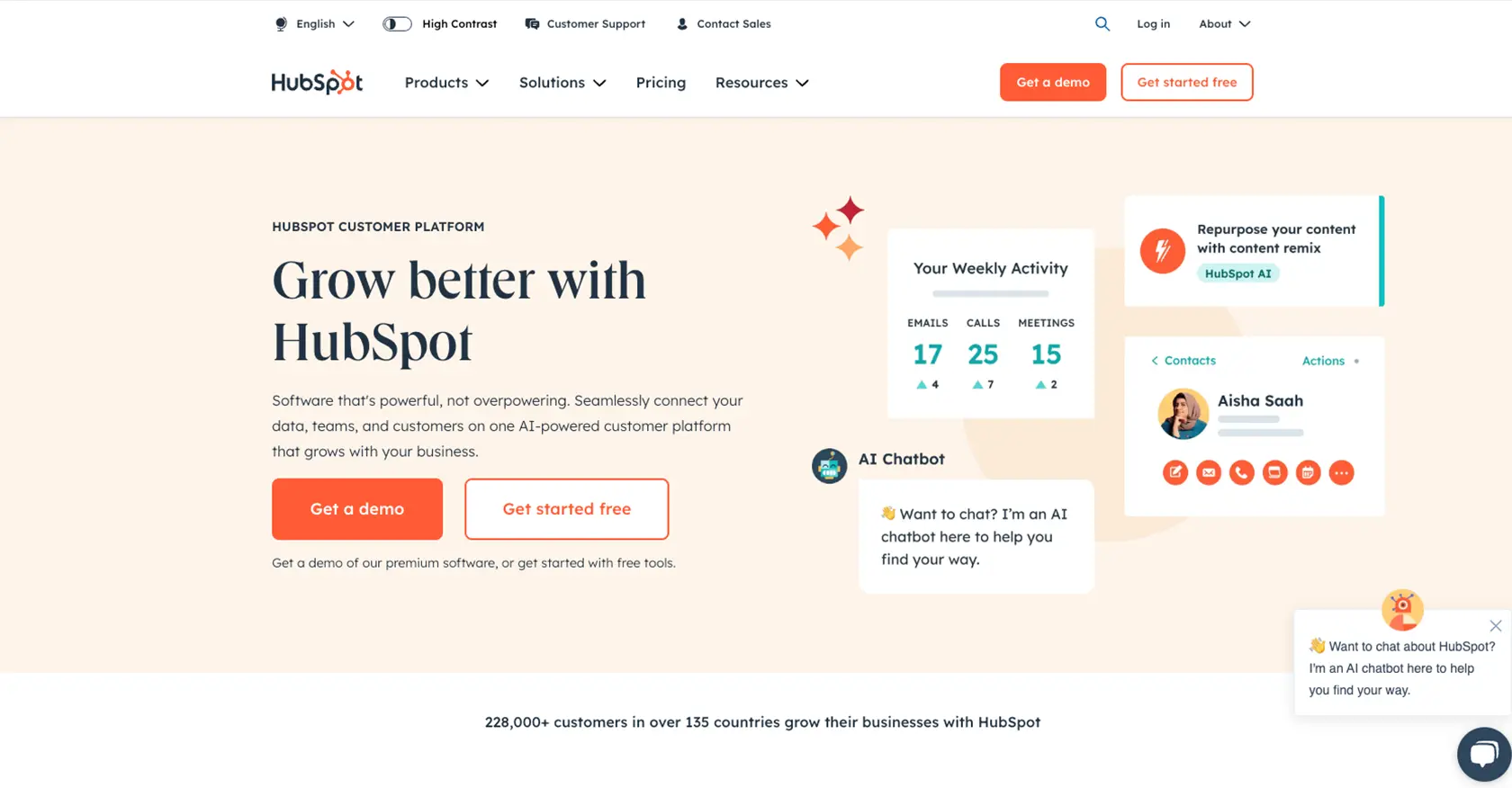
Introduction to HubSpot Custom Objects API
HubSpot is a comprehensive CRM platform that offers a wide range of tools to help businesses manage their marketing, sales, and customer service efforts. Known for its flexibility and user-friendly interface, HubSpot is a popular choice for businesses looking to streamline their operations and enhance customer engagement.
Developers often need to interact with HubSpot's API to access and manipulate data stored within the platform. One powerful feature of HubSpot's API is the ability to create and manage custom objects. Custom objects allow businesses to tailor their CRM data to fit specific business needs, beyond the standard objects like contacts and deals.
For example, a developer might use the HubSpot API to retrieve custom objects that represent unique business entities, such as inventory items or service requests. This capability enables businesses to maintain a more organized and customized CRM system, enhancing data management and operational efficiency.
Setting Up Your HubSpot Developer Account and Sandbox Environment
Before you can start working with HubSpot's Custom Objects API in PHP, you'll need to set up a HubSpot developer account and create a sandbox environment. This will allow you to test your API integrations without affecting live data.
Creating a HubSpot Developer Account
If you don't already have a HubSpot developer account, follow these steps to create one:
- Visit the HubSpot Developer Portal.
- Click on "Create a developer account" and fill in the required information.
- Once your account is created, log in to access the developer dashboard.
Setting Up a HubSpot Sandbox Account
To test your API interactions, you'll need a sandbox account. Follow these steps to set it up:
- In your HubSpot developer dashboard, navigate to the "Test Accounts" section.
- Click on "Create a test account" to generate a sandbox environment.
- Use this sandbox account to safely test API calls and integrations.
Configuring OAuth Authentication for HubSpot API
HubSpot uses OAuth for authentication, which requires setting up an app to obtain the necessary credentials. Here's how to do it:
- In your developer dashboard, go to "Apps" and click "Create app."
- Fill in the app details and configure the necessary scopes for accessing custom objects. For more information on scopes, refer to the HubSpot API Scopes Documentation.
- Once the app is created, you'll receive a client ID and client secret. Keep these credentials secure as they are required for OAuth authentication.
For detailed instructions on working with OAuth, visit the HubSpot OAuth Documentation.
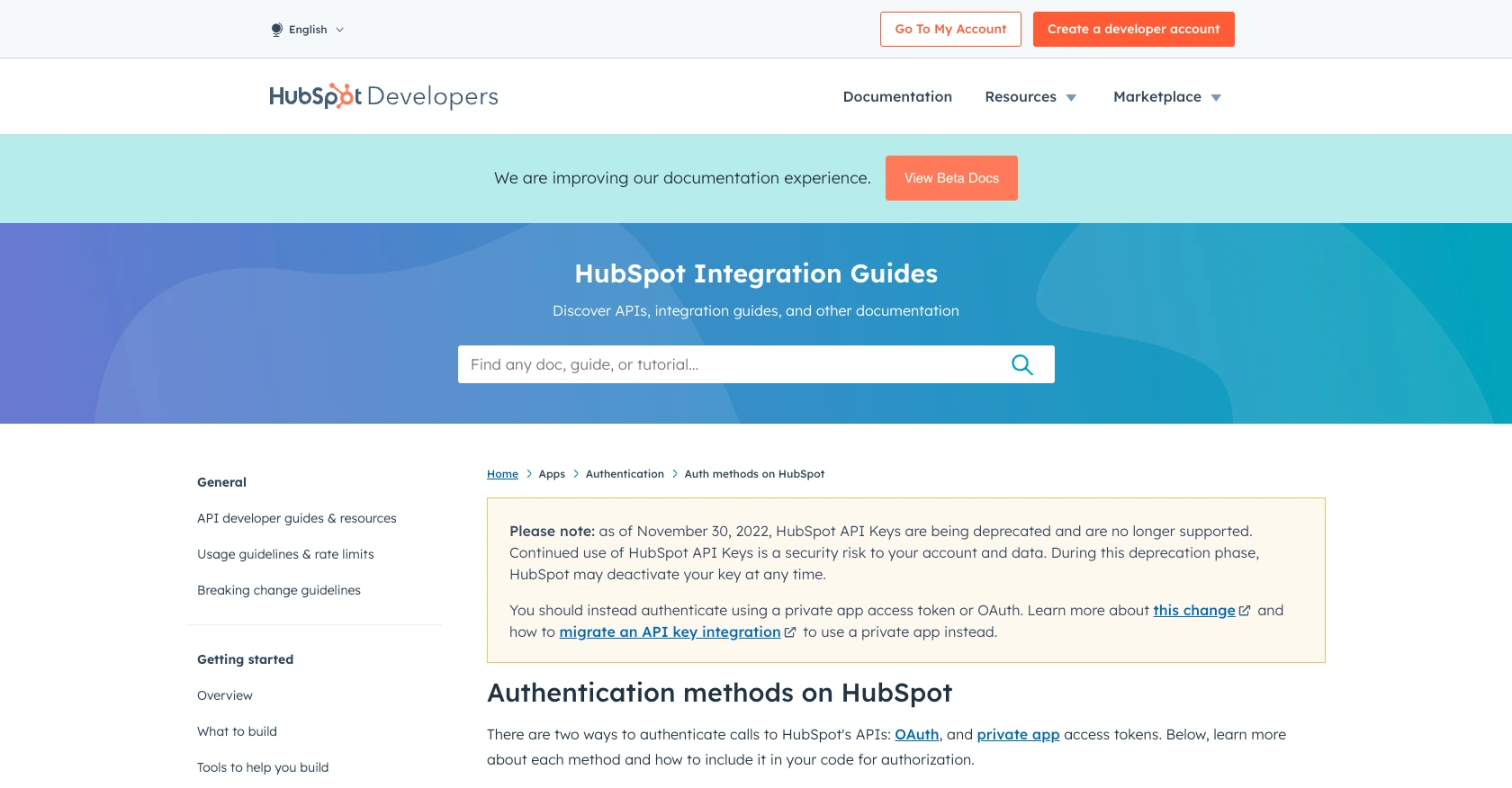
sbb-itb-96038d7
Making API Calls to Retrieve HubSpot Custom Objects Using PHP
To interact with HubSpot's Custom Objects API using PHP, you'll need to set up your environment and write code to make the necessary API calls. This section will guide you through the process of retrieving custom objects from HubSpot using PHP.
Setting Up Your PHP Environment
Before making API calls, ensure your PHP environment is properly configured. You'll need:
- PHP 7.4 or higher
- Composer for dependency management
Install the HubSpot API client for PHP using Composer:
composer require hubspot/api-client
Writing PHP Code to Retrieve HubSpot Custom Objects
With your environment set up, you can now write the PHP code to retrieve custom objects. Here's a step-by-step guide:
require 'vendor/autoload.php';
use HubSpot\Factory;
use HubSpot\Client\Crm\Schemas\ApiException;
// Initialize the HubSpot client
$client = Factory::createWithAccessToken('YOUR_ACCESS_TOKEN');
try {
// Retrieve custom object schemas
$response = $client->crm()->schemas()->coreApi()->getAll();
$customObjects = $response->getResults();
// Display custom object details
foreach ($customObjects as $object) {
echo "Object Name: " . $object->getName() . "\n";
echo "Object Type ID: " . $object->getObjectTypeId() . "\n";
}
} catch (ApiException $e) {
echo "Exception when calling HubSpot API: ", $e->getMessage(), PHP_EOL;
}
Replace YOUR_ACCESS_TOKEN
with the OAuth access token obtained from your HubSpot app. This code initializes the HubSpot client, retrieves all custom object schemas, and prints their details.
Verifying API Call Success and Handling Errors
After running the code, verify the API call's success by checking the output for the expected custom object details. If the API call fails, the ApiException
will provide error details.
Common error codes include:
- 401 Unauthorized: Check your access token and ensure it has the correct scopes.
- 429 Too Many Requests: You've hit the rate limit. Implement request throttling or retry logic.
For more information on error codes, refer to the HubSpot API Usage Details.
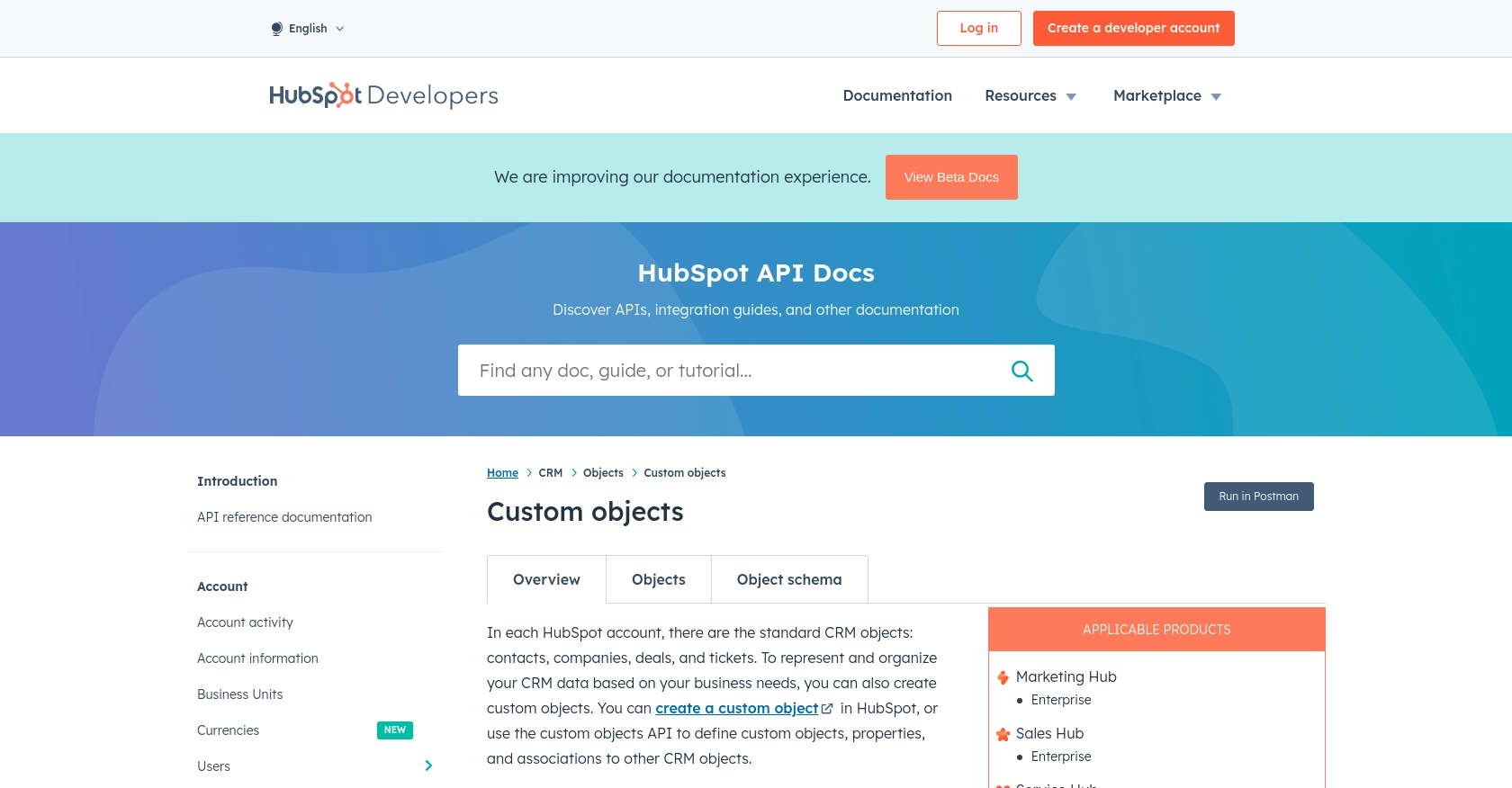
Conclusion and Best Practices for Using HubSpot Custom Objects API in PHP
Integrating with HubSpot's Custom Objects API using PHP allows developers to tailor CRM data to meet specific business needs, enhancing data management and operational efficiency. By following the steps outlined in this guide, you can effectively retrieve and manage custom objects within your HubSpot environment.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your OAuth access tokens securely. Consider using environment variables or secure vaults to manage sensitive information.
- Implement Rate Limiting: HubSpot's API has rate limits, such as 100 requests every 10 seconds for OAuth apps. Implement request throttling and retry logic to handle 429 errors gracefully. For more details, refer to the HubSpot API Usage Details.
- Standardize Data Fields: Ensure that custom object properties are standardized across your CRM to maintain data consistency and integrity.
Streamlining Integrations with Endgrate
If managing multiple integrations becomes overwhelming, consider using Endgrate to simplify the process. Endgrate offers a unified API endpoint that connects to various platforms, including HubSpot, allowing you to focus on your core product while outsourcing integration complexities.
By leveraging Endgrate, you can save time and resources, build once for each use case, and provide an intuitive integration experience for your customers. Visit Endgrate to learn more about how it can enhance your integration strategy.
Read More
- https://endgrate.com/provider/hubspot
- https://developers.hubspot.com/docs/api/overview
- https://developers.hubspot.com/docs/api/intro-to-auth
- https://developers.hubspot.com/docs/api/usage-details
- https://developers.hubspot.com/docs/api/scopes
- https://developers.hubspot.com/docs/api/crm/crm-custom-objects
- https://developers.hubspot.com/docs/api/crm/properties
Ready to get started?