How to Get Records with the Salesforce Sandbox API in Python
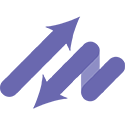
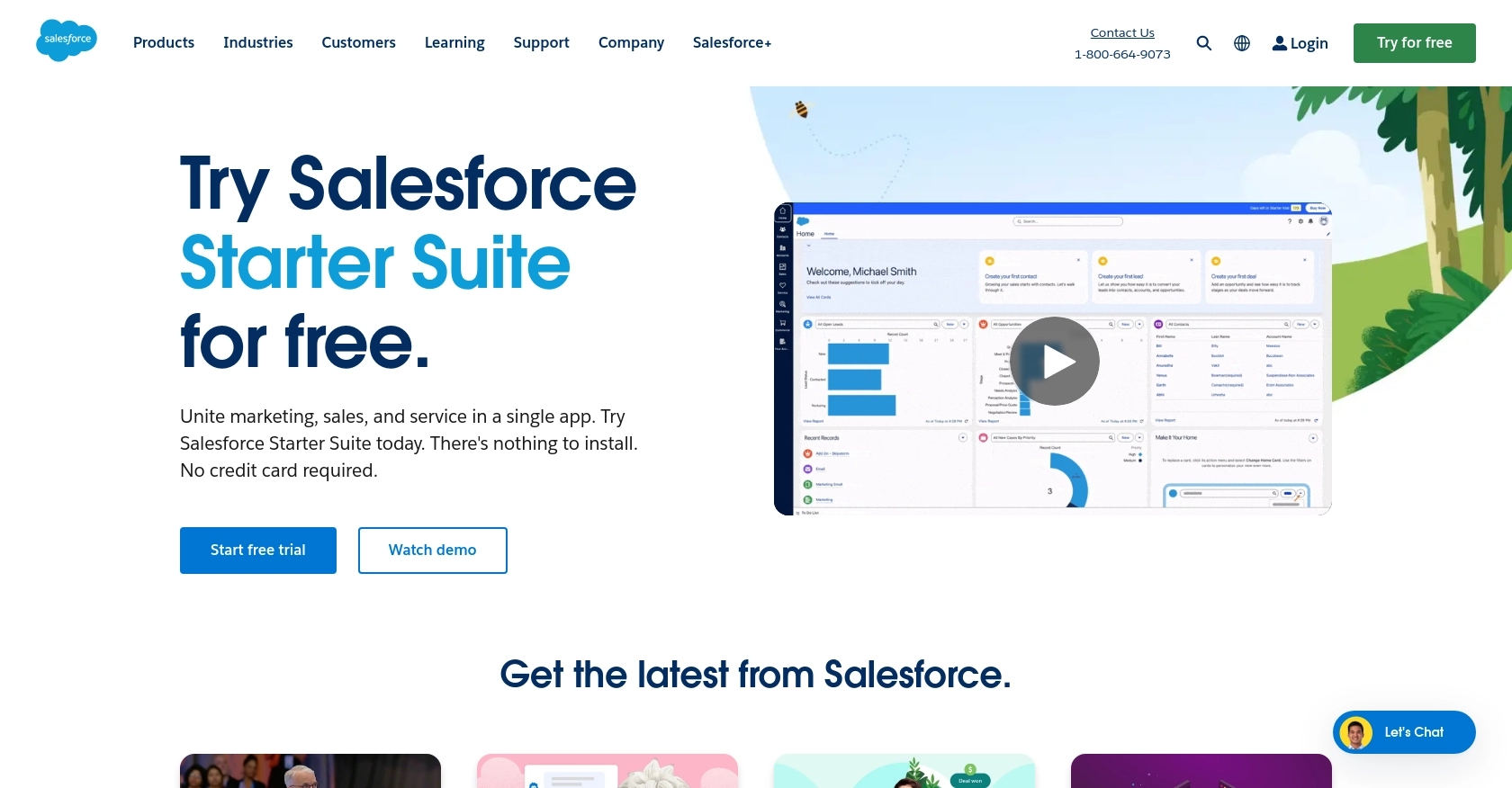
Introduction to Salesforce Sandbox API
Salesforce is a leading cloud-based customer relationship management (CRM) platform that empowers businesses to manage their sales, service, marketing, and more. The Salesforce Sandbox provides a testing environment where developers can safely build and test applications without affecting the production data.
Integrating with the Salesforce Sandbox API allows developers to interact with Salesforce data in a controlled setting. This is particularly useful for testing new features or integrations before deploying them to a live environment. For example, a developer might use the Salesforce Sandbox API to retrieve customer records and analyze sales trends without risking data integrity in the production environment.
Setting Up Your Salesforce Sandbox Account for API Integration
Before you can begin interacting with the Salesforce Sandbox API, you need to set up a Salesforce Sandbox account. This environment allows you to test and develop applications without impacting your live data.
Creating a Salesforce Sandbox Account
If you don't already have a Salesforce account, you'll need to sign up for one. Follow these steps to create a Salesforce Sandbox account:
- Visit the Salesforce Help Center and follow the instructions to create a test instance.
- Once your account is set up, log in to Salesforce and navigate to the Sandbox section under the Setup menu.
- Create a new Sandbox by selecting the appropriate options and configurations that suit your testing needs.
Setting Up OAuth Authentication for Salesforce API Access
Salesforce uses OAuth 2.0 for secure API authentication. To interact with the Salesforce Sandbox API, you'll need to create a connected app and obtain the necessary credentials:
- Navigate to the Setup menu in your Salesforce account.
- Under Platform Tools, find Apps and click on App Manager.
- Click on New Connected App and fill in the required fields, such as the app name and contact email.
- Enable OAuth settings by checking the Enable OAuth Settings box.
- Provide a callback URL and select the necessary OAuth scopes, such as api and refresh_token.
- Save the connected app and note down the Consumer Key and Consumer Secret. These will be used for API authentication.
For more detailed information on setting up OAuth, refer to the Salesforce Developer Guide.
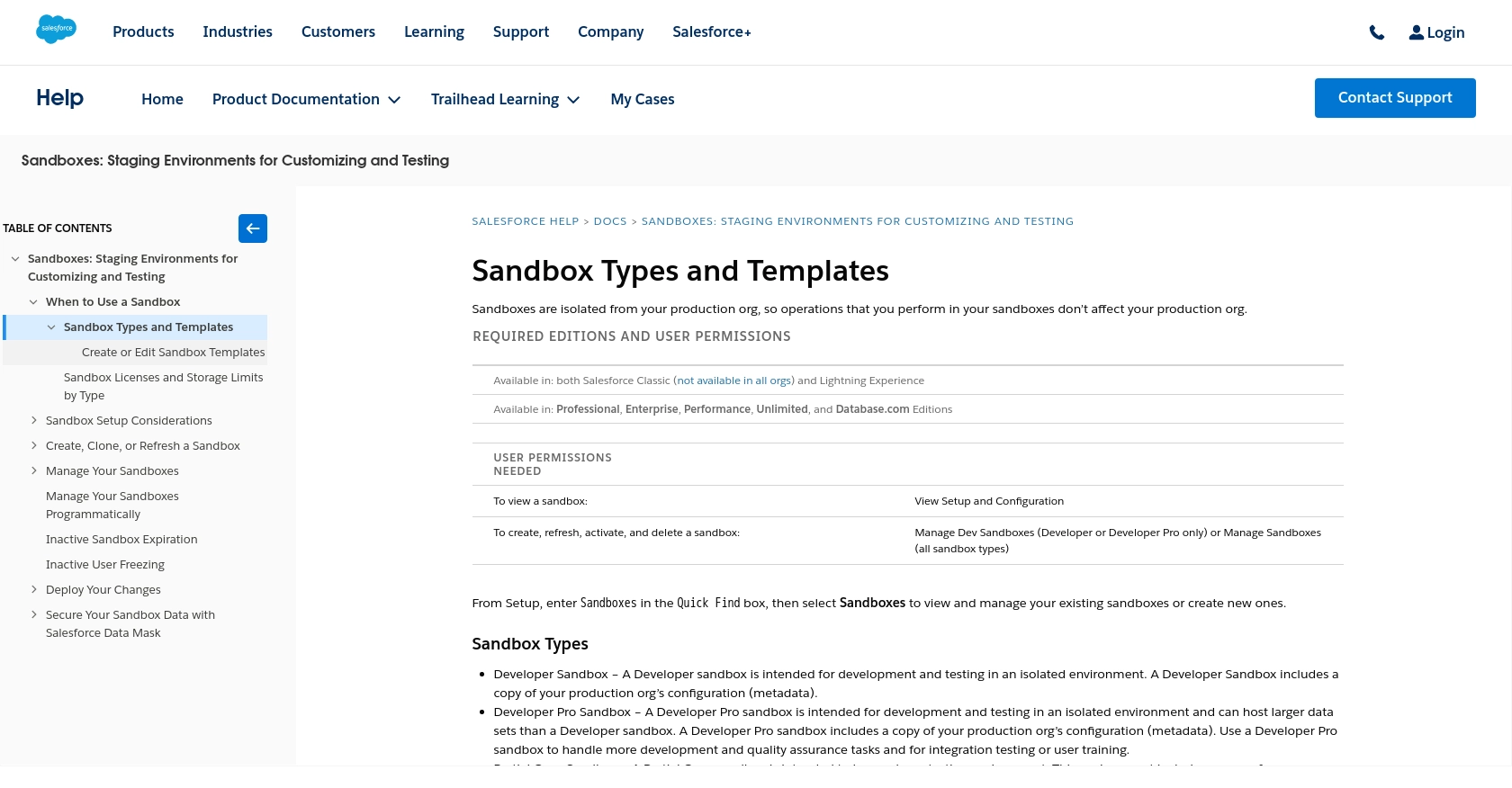
sbb-itb-96038d7
Making API Calls to Retrieve Records from Salesforce Sandbox Using Python
To interact with the Salesforce Sandbox API and retrieve records, you need to use Python, a versatile and widely-used programming language. This section will guide you through the process of setting up your Python environment and making API calls to Salesforce Sandbox.
Setting Up Your Python Environment for Salesforce Sandbox API
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1 or later
- The Python package installer, pip
Once you have these installed, open your terminal or command prompt and install the requests
library, which is essential for making HTTP requests:
pip install requests
Writing Python Code to Retrieve Records from Salesforce Sandbox
Now that your environment is set up, you can write a Python script to retrieve records from Salesforce Sandbox. Create a file named get_salesforce_records.py
and add the following code:
import requests
# Define the Salesforce Sandbox API endpoint
endpoint = "https://your_instance.salesforce.com/services/data/vXX.X/sobjects/Your_Object"
# Set up the headers with your OAuth token
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json"
}
# Make a GET request to the Salesforce Sandbox API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON response
data = response.json()
# Print the retrieved records
for record in data['records']:
print(record)
else:
print(f"Failed to retrieve records: {response.status_code} - {response.text}")
Replace Your_Access_Token
with the access token obtained during the OAuth authentication setup, and your_instance
with your Salesforce instance URL. Also, replace XX.X
with the API version and Your_Object
with the object you wish to retrieve.
Running the Python Script and Verifying the Results
To execute the script, run the following command in your terminal or command prompt:
python get_salesforce_records.py
If successful, you should see the records printed in your terminal. Verify the retrieved data by comparing it with the records in your Salesforce Sandbox account.
Handling Errors and Common Issues with Salesforce Sandbox API
When making API calls, you may encounter errors. Here are some common error codes and their meanings:
- 401 Unauthorized: Check if your OAuth token is valid and has not expired.
- 403 Forbidden: Ensure your app has the necessary permissions to access the requested resource.
- 404 Not Found: Verify the endpoint URL and object name are correct.
For more detailed error handling, refer to the Salesforce API documentation.
Conclusion and Best Practices for Using Salesforce Sandbox API with Python
Integrating with the Salesforce Sandbox API using Python provides a powerful way to test and develop applications in a secure environment. By following the steps outlined in this guide, you can efficiently retrieve records and ensure your integrations are robust before deploying them to production.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your OAuth credentials, such as the Consumer Key and Consumer Secret, securely. Consider using environment variables or a secure vault to manage sensitive information.
- Handle Rate Limiting: Salesforce imposes rate limits on API calls. Be mindful of these limits to avoid disruptions. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that data fields retrieved from Salesforce are standardized and transformed as needed to fit your application's requirements.
- Monitor and Log API Calls: Keep track of API calls and responses for debugging and auditing purposes. Logging can help identify issues and improve the integration process.
Streamlining Integrations with Endgrate
While building integrations with Salesforce Sandbox API is essential, managing multiple integrations can be time-consuming. Endgrate offers a unified API solution that simplifies the integration process across various platforms, including Salesforce.
By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development. With a single API endpoint, you can build once for each use case and provide an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website.
Read More
- https://endgrate.com/provider/salesforce-sandbox
- https://help.salesforce.com/s/articleView?id=sf.create_test_instance.htm&type=5
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/intro_oauth_and_connected_apps.htm
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/quickstart_dev_org.htm
- https://developer.salesforce.com/docs/atlas.en-us.api_rest.meta/api_rest/resources_list.htm
Ready to get started?