How to Get Credit Notes with the Chargebee API in Javascript
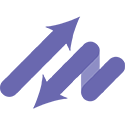
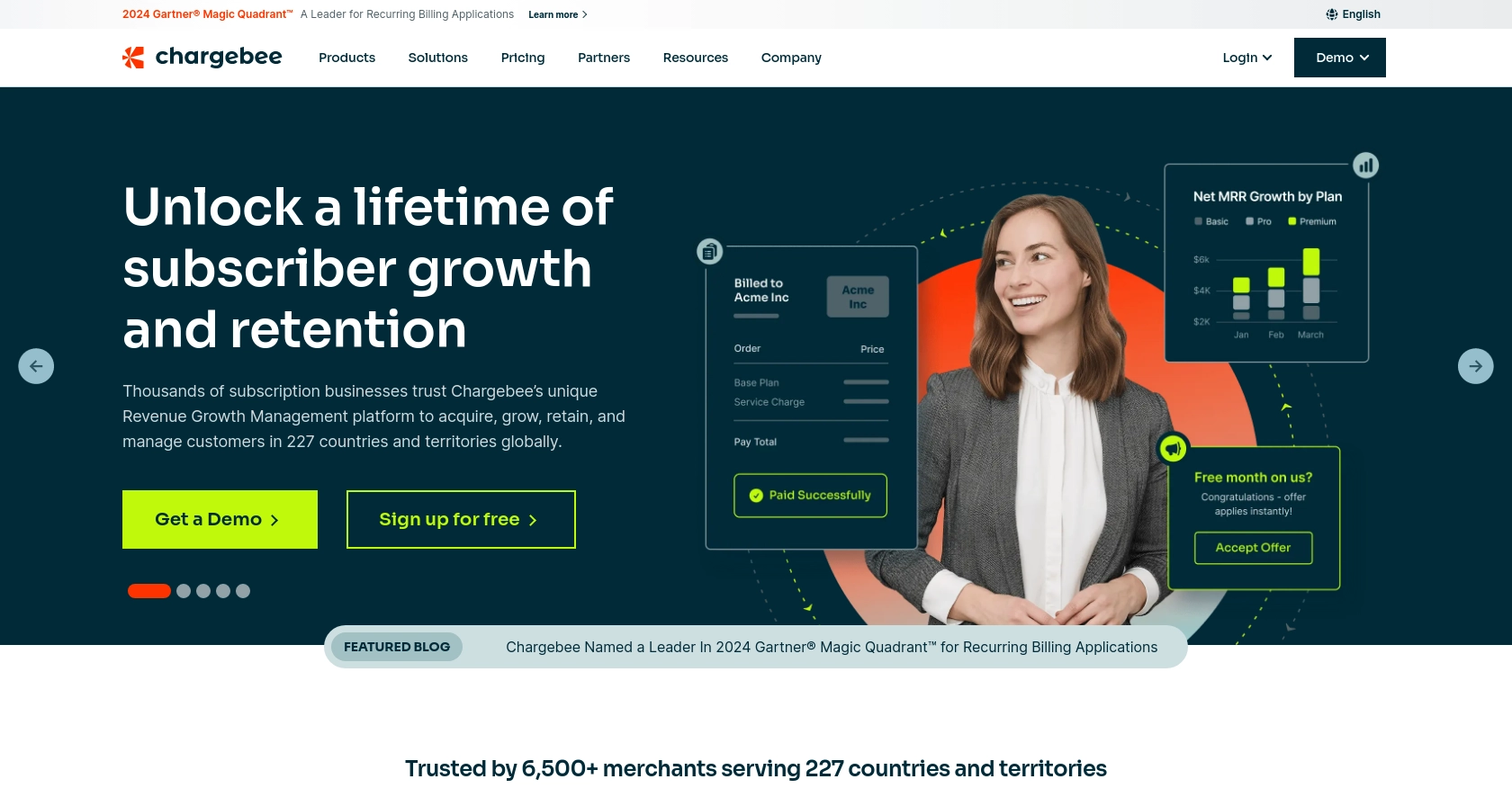
Introduction to Chargebee API for Credit Notes
Chargebee is a robust subscription management and billing platform that empowers businesses to streamline their revenue operations. With its comprehensive suite of tools, Chargebee simplifies billing, invoicing, and revenue recognition, making it a preferred choice for SaaS companies.
Integrating with Chargebee's API allows developers to automate and manage financial operations efficiently. For example, using the Chargebee API, developers can retrieve credit notes, which are essential for handling refunds and adjustments in billing. This integration can significantly enhance the financial workflows of a business by providing real-time access to credit note data.
In this article, we will explore how to interact with the Chargebee API using JavaScript to retrieve credit notes, providing a practical guide for developers looking to integrate this functionality into their applications.
Setting Up Your Chargebee Test/Sandbox Account
Before you can start retrieving credit notes using the Chargebee API, you'll need to set up a test or sandbox account. This allows you to safely experiment with the API without affecting live data.
Create a Chargebee Test Account
If you don't already have a Chargebee account, follow these steps to create a test account:
- Visit the Chargebee website and sign up for a free trial.
- Follow the on-screen instructions to complete the registration process.
- Once registered, log in to your Chargebee dashboard.
Generate API Keys for Chargebee
Chargebee uses HTTP Basic authentication for API calls, where your API key acts as the username and the password is left blank. Here's how to generate your API key:
- In the Chargebee dashboard, navigate to the Settings section.
- Click on API Keys under the Configure Chargebee menu.
- Click on Create a New API Key and provide a name for your key.
- Copy the generated API key and store it securely. You'll use this key to authenticate your API requests.
Note: Ensure you are using the API key for your test site, as it differs from the live site key.
Set Up OAuth for Chargebee API Access
Chargebee also supports OAuth-based authentication for more secure access. To set this up:
- In the Chargebee dashboard, go to Settings and select OAuth Apps.
- Click on Create a New OAuth App and fill in the required details, such as the app name and redirect URL.
- Once created, note the Client ID and Client Secret. These will be used to obtain access tokens for API calls.
Testing Your Chargebee API Setup
With your test account and API keys ready, you can now test your setup by making a simple API call to list credit notes. Use the following JavaScript code snippet to verify your setup:
const axios = require('axios');
const site = 'your-site';
const apiKey = 'your-api-key';
axios.get(`https://${site}.chargebee.com/api/v2/credit_notes`, {
auth: {
username: apiKey,
password: ''
}
})
.then(response => {
console.log('Credit Notes:', response.data);
})
.catch(error => {
console.error('Error fetching credit notes:', error);
});
Replace your-site
and your-api-key
with your actual Chargebee site name and API key. This code uses the axios
library to make an authenticated request to the Chargebee API.
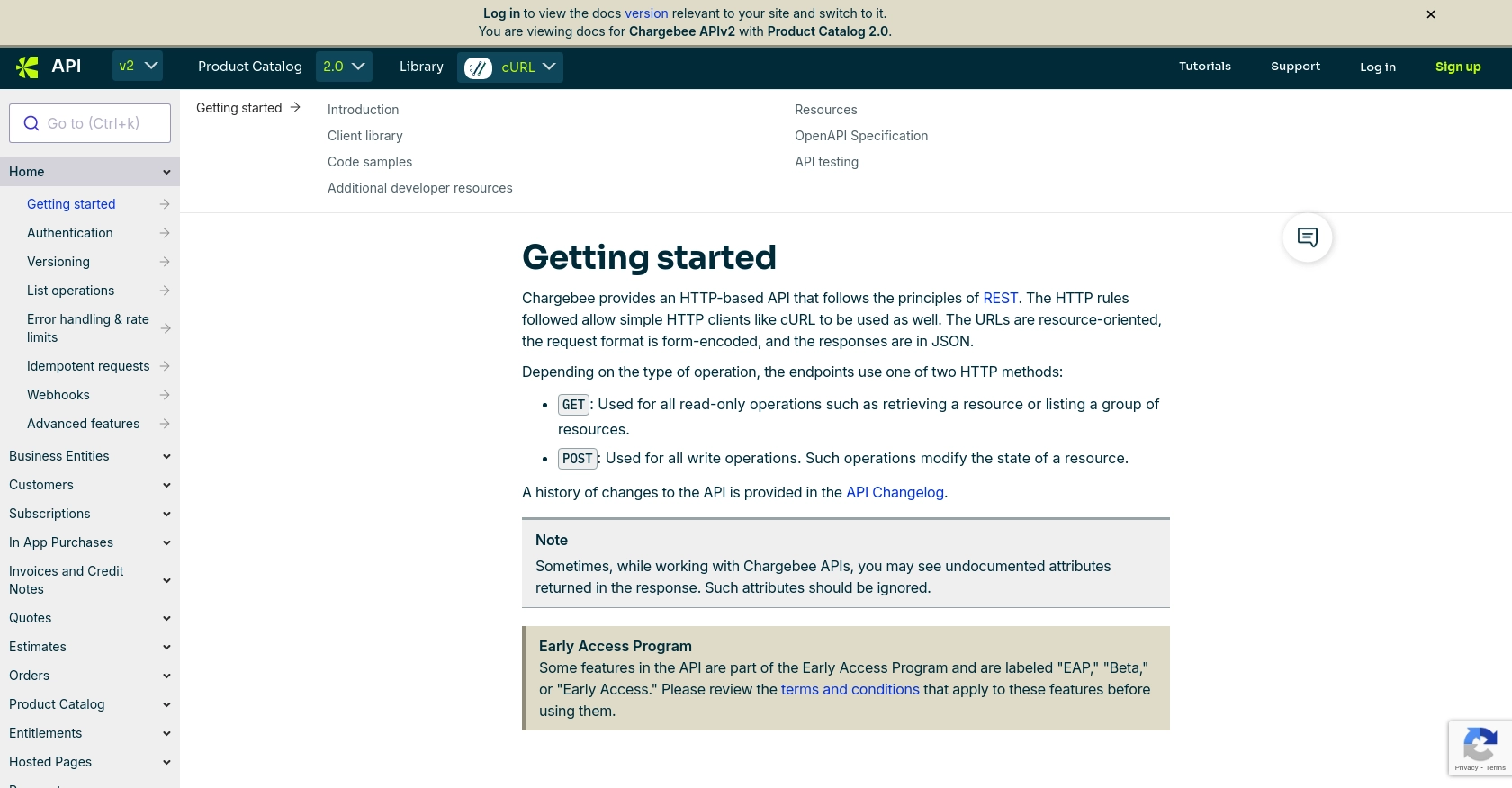
sbb-itb-96038d7
Making API Calls to Retrieve Credit Notes with Chargebee API in JavaScript
To effectively interact with the Chargebee API and retrieve credit notes using JavaScript, you need to ensure that your development environment is set up correctly. This section will guide you through the necessary steps, including setting up your JavaScript environment, installing dependencies, and writing the code to make API calls.
Setting Up Your JavaScript Environment for Chargebee API
Before making API calls, ensure you have Node.js installed on your machine. Node.js provides the runtime environment needed to execute JavaScript code outside a web browser.
- Download and install Node.js from the official website.
- Verify the installation by running
node -v
andnpm -v
in your terminal to check the versions of Node.js and npm (Node Package Manager).
Installing Required Dependencies for Chargebee API Integration
To make HTTP requests to the Chargebee API, you will use the axios
library. Install it using npm:
npm install axios
Writing JavaScript Code to Retrieve Credit Notes from Chargebee
With your environment set up and dependencies installed, you can now write the JavaScript code to retrieve credit notes from Chargebee. Below is a sample code snippet:
const axios = require('axios');
const site = 'your-site';
const apiKey = 'your-api-key';
axios.get(`https://${site}.chargebee.com/api/v2/credit_notes`, {
auth: {
username: apiKey,
password: ''
}
})
.then(response => {
console.log('Credit Notes:', response.data.list);
})
.catch(error => {
console.error('Error fetching credit notes:', error.response.data);
});
Replace your-site
and your-api-key
with your actual Chargebee site name and API key. This code uses the axios
library to make an authenticated GET request to the Chargebee API, retrieving a list of credit notes.
Verifying Successful API Requests in Chargebee
After running the code, verify the successful retrieval of credit notes by checking the console output. The response should include a list of credit notes from your Chargebee test account. If the request fails, the error message will be displayed in the console.
Handling Errors and Understanding Chargebee API Error Codes
When interacting with the Chargebee API, it's crucial to handle potential errors gracefully. Chargebee provides detailed error codes to help diagnose issues:
- 401 Unauthorized: Check your API key and ensure it's correct.
- 404 Not Found: Verify the API endpoint URL.
- 429 Too Many Requests: Implement rate limiting strategies as Chargebee allows ~750 API calls per 5 minutes for test sites.
For more detailed error handling, refer to the Chargebee API documentation.
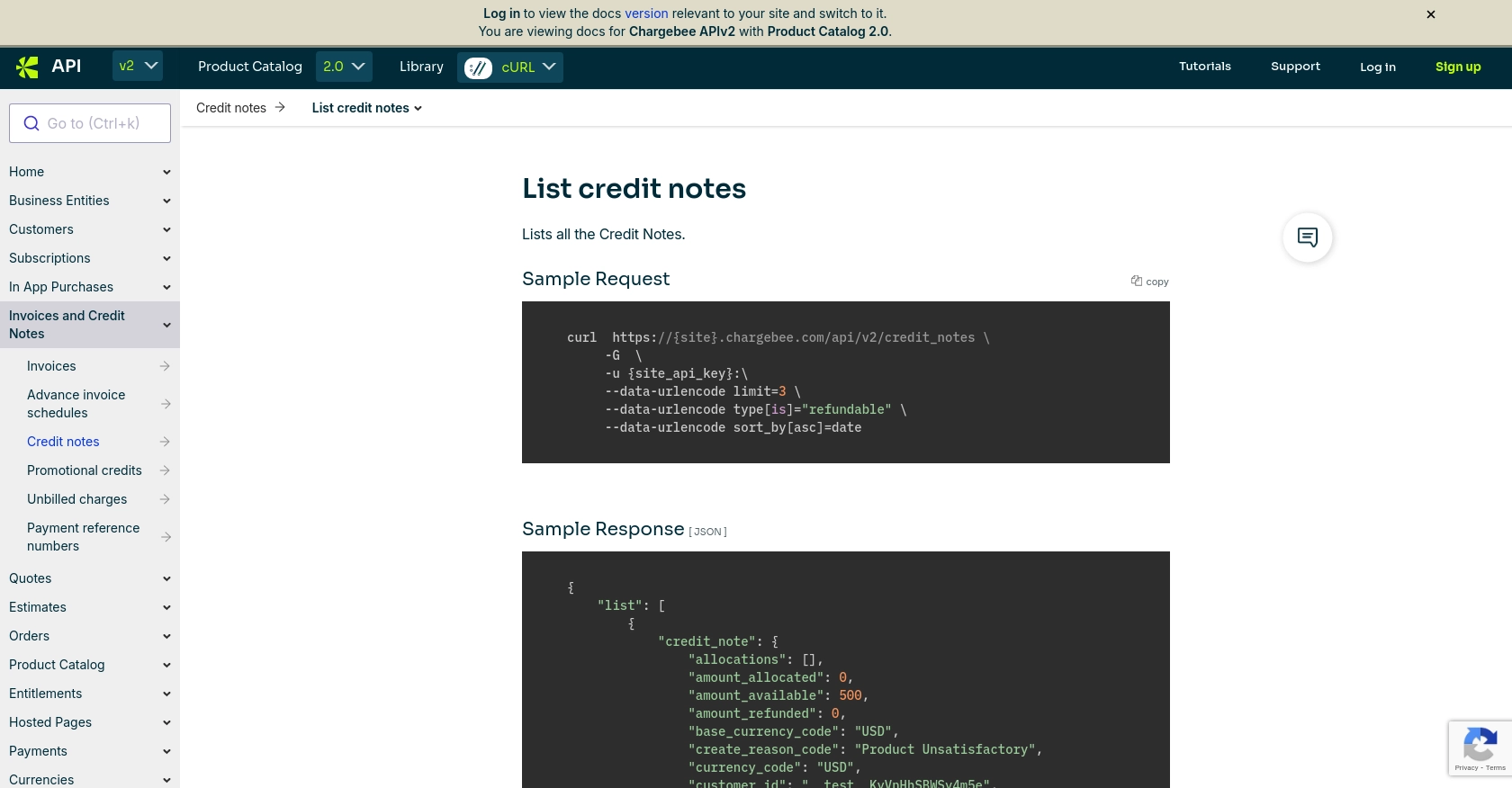
Conclusion and Best Practices for Using Chargebee API with JavaScript
Integrating Chargebee's API into your JavaScript applications can significantly enhance your ability to manage financial operations, such as retrieving credit notes. By following the steps outlined in this guide, you can efficiently set up your environment, authenticate requests, and handle API interactions.
Best Practices for Secure and Efficient Chargebee API Integration
- Secure API Keys: Always store your API keys securely and avoid hardcoding them in your source code. Consider using environment variables or secure vaults.
- Handle Rate Limits: Be mindful of Chargebee's rate limits, which allow ~750 API calls per 5 minutes for test sites. Implement strategies like exponential backoff to handle rate limiting gracefully.
- Data Standardization: Ensure that data retrieved from Chargebee is standardized and transformed as needed to fit your application's requirements.
- Error Handling: Implement robust error handling to manage potential issues, using Chargebee's detailed error codes to guide your responses.
Enhancing Integration Efficiency with Endgrate
While integrating Chargebee's API can be straightforward, managing multiple integrations can become complex. Endgrate offers a unified API endpoint that simplifies the integration process across various platforms, including Chargebee. By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can streamline your integration efforts by visiting Endgrate and discover a more intuitive integration experience for your team and customers.
Read More
Ready to get started?