Using the Mode API to Get Users (with Python examples)
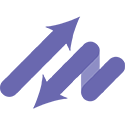
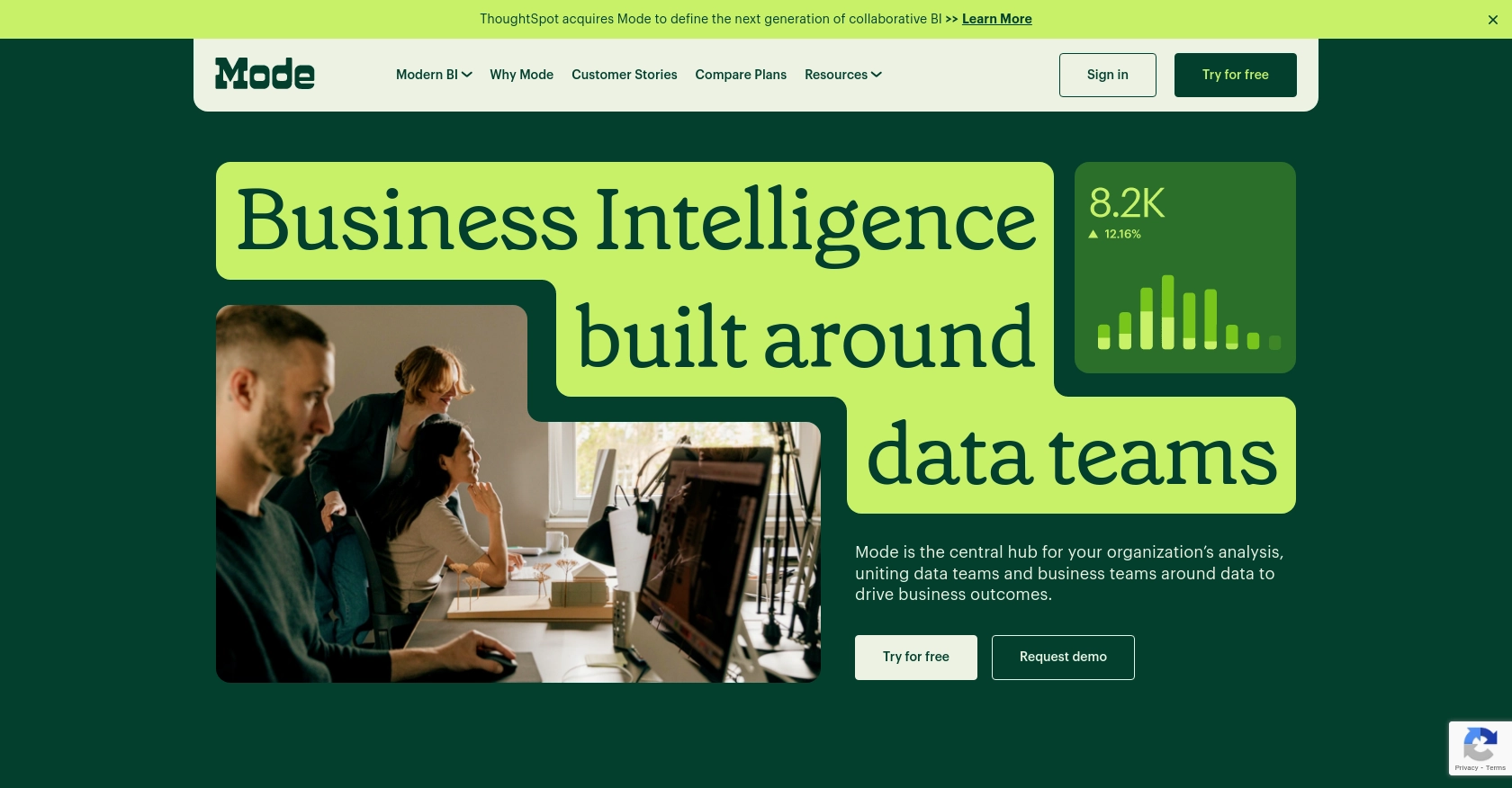
Introduction to Mode API for User Management
Mode is a collaborative analytics platform that empowers teams to make data-driven decisions through seamless collaboration. With its robust suite of analytics tools, Mode enables users to write queries, build reports, and manage data efficiently.
Integrating with the Mode API allows developers to programmatically access and manage user data within a Mode Workspace. This can be particularly useful for automating user management tasks, such as retrieving a list of users or managing workspace memberships.
For example, a developer might use the Mode API to automate the process of syncing user data between Mode and an internal system, ensuring that user information is always up-to-date across platforms.
Setting Up Your Mode Test Account for API Integration
Before diving into the Mode API, you need to set up a Mode account. This will allow you to access and interact with the API effectively. Follow the steps below to create your account and obtain the necessary credentials.
Create a Mode Account and Workspace
If you don't already have a Mode account, start by signing up on the Mode website. Once registered, you'll need to create a Workspace, which is essential for accessing Mode's API resources.
- Visit the Mode website and click on "Sign Up."
- Follow the prompts to create your account.
- After registration, create a new Workspace by navigating to the Workspace section in your dashboard.
Generate an API Access Token for Mode
Mode uses API tokens for authentication. To interact with the Mode API, you need to generate an API access token. Note that your Workspace must be on a paid Mode plan to access the API.
- Navigate to your Workspace Settings.
- Go to "Privacy & Security" and select "API."
- Click the gear icon and choose "Create new API token."
- Provide a display name for your token and save it securely.
The token consists of two parts:
- Token: The public component, often referred to as the username or access key.
- Secret: The private component, often referred to as the password or access secret. This is shown only once, so ensure you save it securely.
Testing Your Mode API Access
Once you have your API token, it's crucial to test your access to ensure everything is set up correctly. You can do this by sending a simple request to the Mode API.
import requests
from requests.auth import HTTPBasicAuth
# Define your Mode API credentials
username = 'your_api_token'
password = 'your_api_secret'
# Set the API endpoint
url = 'https://app.mode.com/api/account'
# Make a GET request to the API
response = requests.get(url, auth=HTTPBasicAuth(username, password))
# Print the response
print(response.json())
Replace your_api_token
and your_api_secret
with the actual values you obtained earlier. Running this code should return a JSON response with your account details, confirming that your API access is working.
For more information on authentication, visit the Mode API authentication documentation.
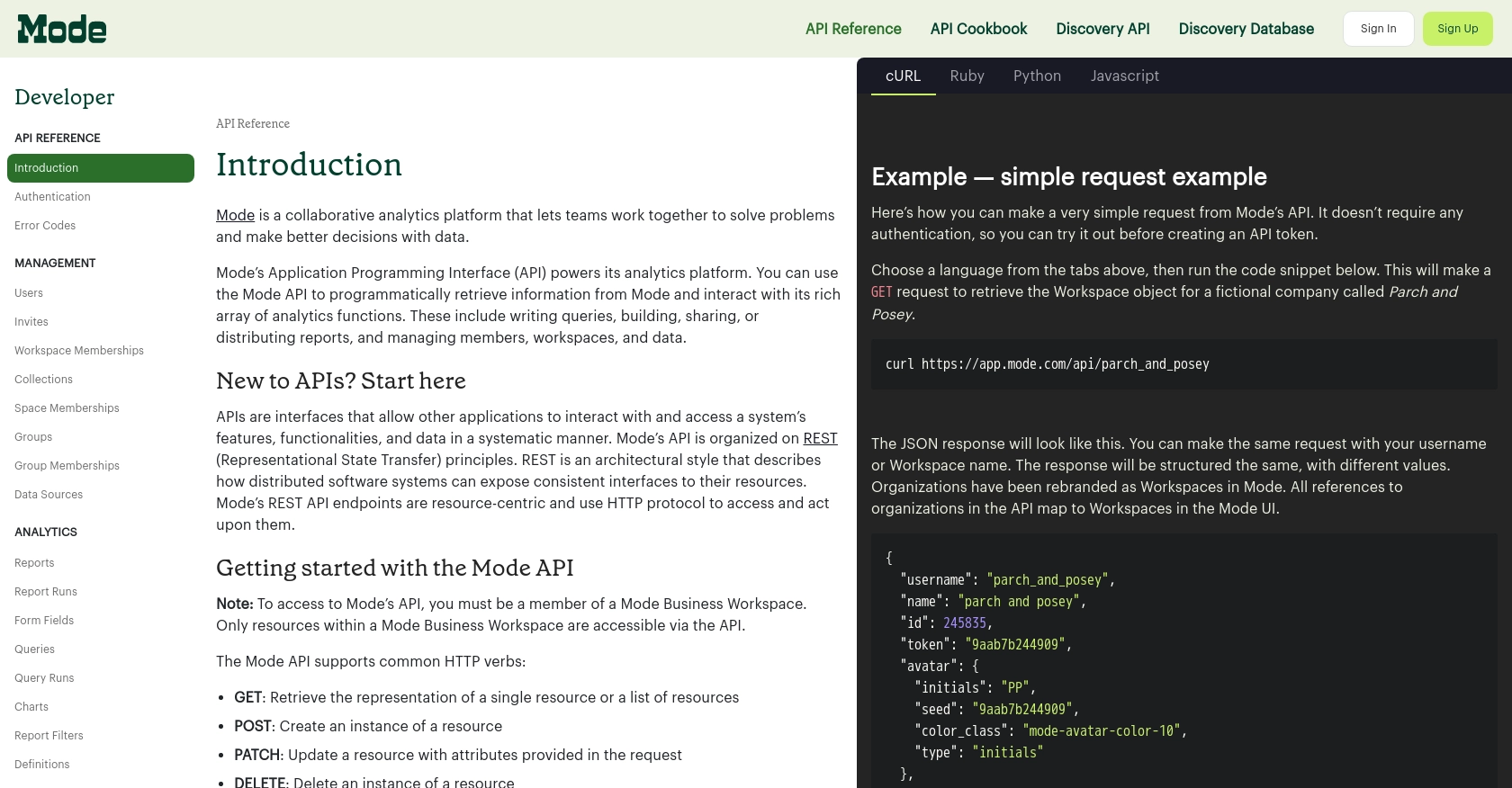
sbb-itb-96038d7
Making API Calls to Retrieve Users from Mode Using Python
Once you have set up your Mode account and obtained the necessary API credentials, you can start making API calls to retrieve user information from your Mode Workspace. This section will guide you through the process of making these calls using Python.
Setting Up Your Python Environment for Mode API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests. Install it using pip:
pip install requests
Python Code to Retrieve Users from Mode Workspace
To retrieve a list of users from your Mode Workspace, you'll need to make a GET request to the appropriate API endpoint. Below is a Python script to help you achieve this:
import requests
from requests.auth import HTTPBasicAuth
# Define your Mode API credentials
username = 'your_api_token'
password = 'your_api_secret'
# Set the API endpoint for listing memberships
workspace = 'your_workspace_name'
url = f'https://app.mode.com/api/{workspace}/memberships'
# Make a GET request to the API
response = requests.get(url, auth=HTTPBasicAuth(username, password))
# Check if the request was successful
if response.status_code == 200:
users = response.json()
for user in users:
print(f"User: {user['member_username']}, State: {user['state']}")
else:
print(f"Failed to retrieve users. Status code: {response.status_code}")
Replace your_api_token
, your_api_secret
, and your_workspace_name
with your actual API token, secret, and workspace name. Running this script will print out the usernames and their states from your Mode Workspace.
Verifying API Call Success in Mode
To verify that your API call was successful, check the response status code. A status code of 200 indicates success. You can also log in to your Mode account and navigate to the Workspace to confirm that the user data matches the API response.
Handling Errors and Common HTTP Response Codes
When interacting with the Mode API, you may encounter various HTTP response codes. Here are some common ones:
- 200 OK: The request was successful.
- 401 Unauthorized: Authentication failed. Check your API token and secret.
- 404 Not Found: The specified resource could not be found. Verify the workspace name.
For more detailed information on error codes, refer to the Mode API error codes documentation.
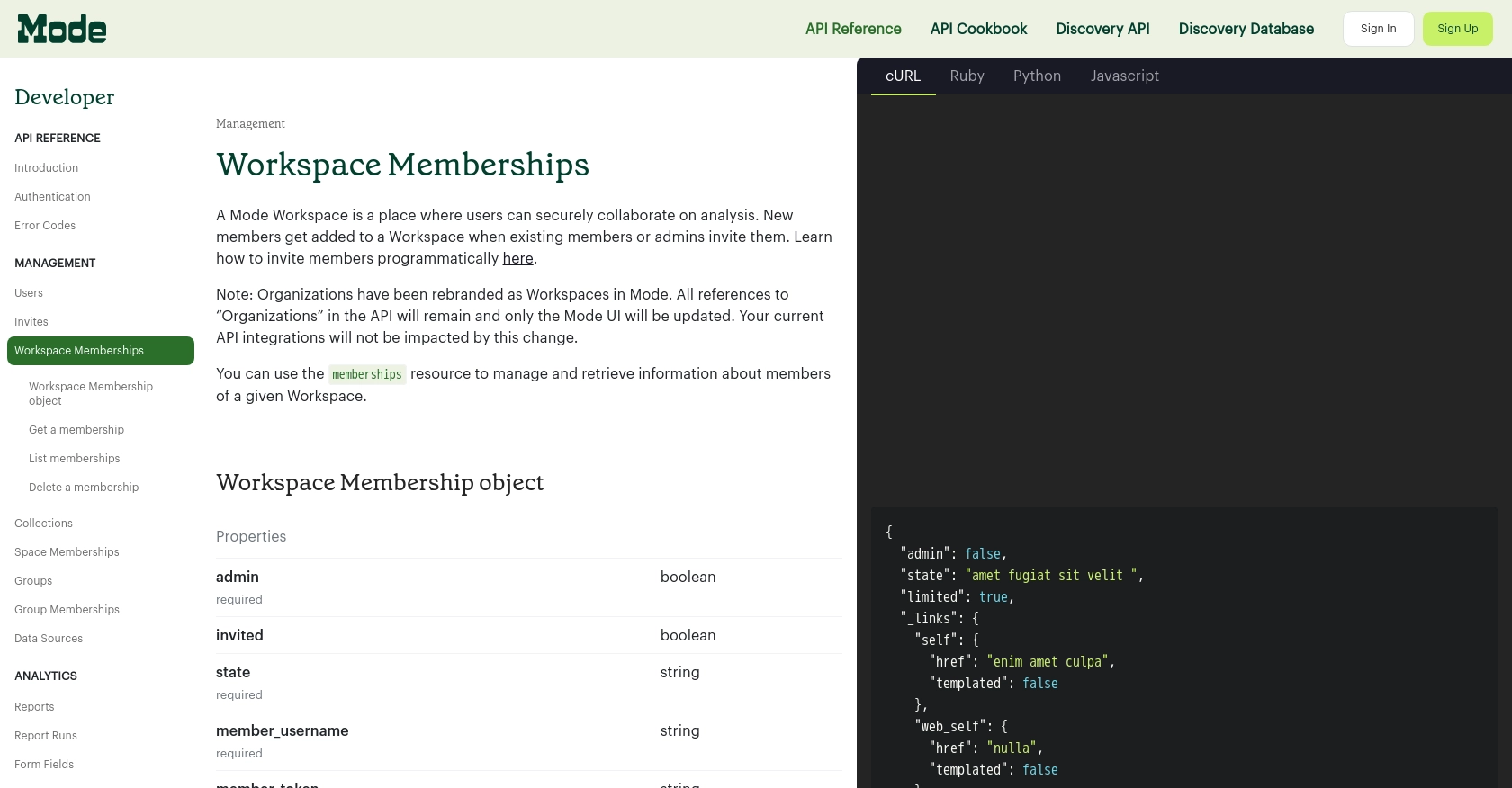
Conclusion and Best Practices for Using Mode API with Python
Integrating with the Mode API offers a powerful way to manage user data and automate workflows within your Mode Workspace. By following the steps outlined in this guide, you can efficiently retrieve user information using Python, ensuring that your data management processes are both streamlined and effective.
Best Practices for Secure and Efficient Mode API Integration
- Secure Storage of API Credentials: Always store your API token and secret securely. Avoid exposing them in public repositories or client-side code.
- Handling Rate Limits: Be mindful of any rate limits imposed by the Mode API. Implement retry logic and exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that data retrieved from the API is standardized and transformed as needed to fit your internal systems.
Enhance Your Integration Strategy with Endgrate
While integrating with Mode API can be straightforward, managing multiple integrations across different platforms can become complex. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Mode. This allows you to focus on your core product while outsourcing integration management.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Explore how Endgrate can streamline your integration efforts by visiting Endgrate.
Read More
Ready to get started?