Using the Teamleader API to Create Or Update Company in Javascript
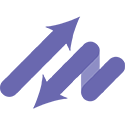
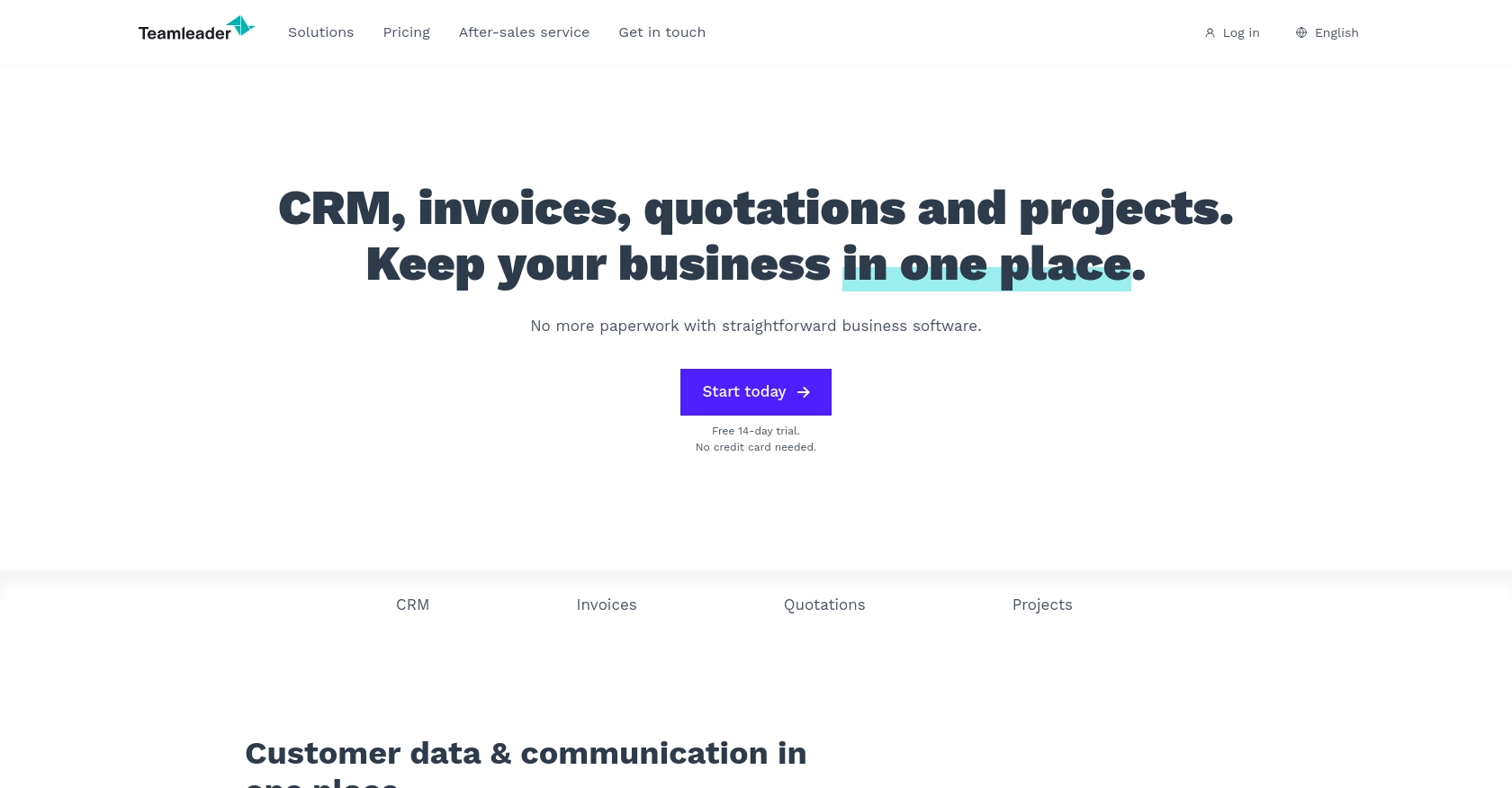
Introduction to Teamleader API Integration
Teamleader is a versatile business management tool that combines CRM, project management, and invoicing functionalities into a single platform. It is designed to help businesses streamline their operations, improve customer relationships, and enhance productivity.
Developers may want to integrate with Teamleader's API to automate and manage company data efficiently. For example, using the Teamleader API, a developer can create or update company information directly from their application, ensuring that the data remains consistent and up-to-date across platforms.
Setting Up Your Teamleader Test or Sandbox Account
Before you can start integrating with the Teamleader API, you need to set up a test or sandbox account. This allows you to safely experiment with API calls without affecting live data. Teamleader provides a sandbox environment that mimics the production environment, enabling developers to test their integrations thoroughly.
Creating a Teamleader Sandbox Account
To begin, you need to sign up for a Teamleader account. If you don't already have one, visit the Teamleader Developer Portal and follow the instructions to create a free trial account. This account will serve as your sandbox environment.
Setting Up OAuth Authentication for Teamleader API
The Teamleader API uses OAuth 2.0 for authentication, which is a secure method for authorizing API requests. Follow these steps to set up OAuth authentication:
- Log in to your Teamleader account and navigate to the Developer section.
- Create a new app by providing the necessary details such as the app name and description.
- Once the app is created, you will receive a Client ID and Client Secret. These are crucial for the OAuth process.
- Define the redirect URI, which is the endpoint where Teamleader will send the authorization code after the user grants permission.
- Set the required scopes for your application. Scopes define the level of access your app will have to the user's data.
Generating Access Tokens
With your Client ID and Client Secret, you can now generate access tokens. Here's a basic outline of the process:
- Direct the user to Teamleader's authorization URL, including your Client ID and requested scopes.
- After the user authorizes your app, Teamleader will redirect them to your specified redirect URI with an authorization code.
- Exchange the authorization code for an access token by making a POST request to Teamleader's token endpoint, including your Client ID, Client Secret, and the authorization code.
Once you have the access token, you can use it to authenticate your API requests. Remember to securely store the Client ID, Client Secret, and access tokens, as they are sensitive credentials.
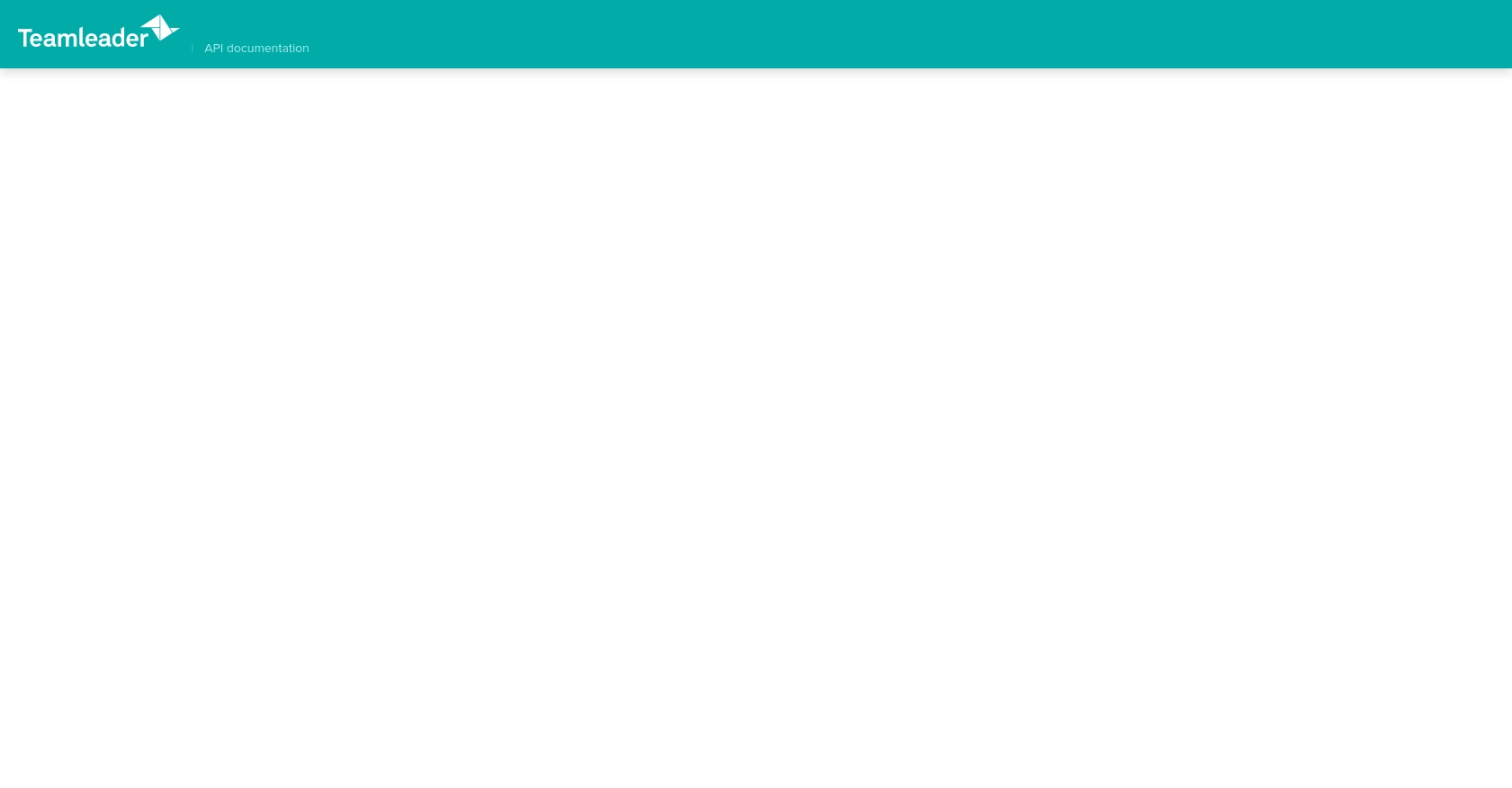
sbb-itb-96038d7
Making API Calls to Teamleader Using JavaScript
To interact with the Teamleader API and perform actions such as creating or updating company information, you need to make authenticated API calls using JavaScript. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling responses.
Setting Up Your JavaScript Environment
Before you start coding, ensure you have the following prerequisites:
- Node.js installed on your machine. You can download it from the official Node.js website.
- A code editor like Visual Studio Code or any other of your choice.
- Familiarity with JavaScript and basic understanding of HTTP requests.
Installing Necessary Dependencies
To make HTTP requests in JavaScript, you can use the popular axios
library. Install it using npm:
npm install axios
Creating or Updating a Company with Teamleader API
Now, let's write the JavaScript code to create or update a company in Teamleader. Here's a step-by-step guide:
const axios = require('axios');
// Define the API endpoint
const endpoint = 'https://api.teamleader.eu/companies.createOrUpdate';
// Set your access token
const accessToken = 'Your_Access_Token';
// Define the company data
const companyData = {
name: 'New Company',
business_type: 'customer',
emails: [{ type: 'primary', email: 'info@newcompany.com' }],
telephones: [{ type: 'phone', number: '+1234567890' }]
};
// Make the API call
axios.post(endpoint, companyData, {
headers: {
'Authorization': `Bearer ${accessToken}`,
'Content-Type': 'application/json'
}
})
.then(response => {
console.log('Company created or updated successfully:', response.data);
})
.catch(error => {
console.error('Error creating or updating company:', error.response.data);
});
Replace Your_Access_Token
with the access token you obtained during the OAuth authentication process. The code above sends a POST request to the Teamleader API endpoint to create or update a company. It includes the necessary headers and company data in JSON format.
Verifying the API Call Success
After running the code, you can verify the success of the API call by checking the response data. A successful response will include details of the created or updated company. Additionally, you can log into your Teamleader sandbox account to confirm the changes.
Handling Errors and Error Codes
It's crucial to handle potential errors when making API calls. The example code includes a catch
block to capture and log any errors. Common error codes you might encounter include:
- 400 Bad Request: The request was invalid. Check the request parameters and data format.
- 401 Unauthorized: Authentication failed. Ensure your access token is correct.
- 404 Not Found: The requested resource could not be found. Verify the endpoint URL.
- 500 Internal Server Error: An error occurred on the server. Try again later.
For more detailed error handling, refer to the Teamleader API Documentation.
Conclusion and Best Practices for Teamleader API Integration
Integrating with the Teamleader API using JavaScript allows developers to efficiently manage company data, ensuring consistency and accuracy across platforms. By following the steps outlined in this guide, you can create or update company information seamlessly within your application.
Best Practices for Secure and Efficient API Usage
- Secure Storage of Credentials: Always store your Client ID, Client Secret, and access tokens securely. Consider using environment variables or a secure vault to protect sensitive information.
- Handle Rate Limiting: Be mindful of API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that the data you send and receive is standardized to maintain consistency across different systems and applications.
Streamline Your Integrations with Endgrate
While integrating with individual APIs like Teamleader can be rewarding, it can also be time-consuming and complex, especially when managing multiple integrations. Endgrate offers a unified API solution that simplifies the integration process, allowing you to focus on your core product development.
With Endgrate, you can build once for each use case and leverage an intuitive integration experience for your customers. Save time and resources by outsourcing integrations and ensuring a seamless connection with platforms like Teamleader.
Visit Endgrate to learn more about how you can enhance your integration strategy and streamline your development workflow.
Read More
Ready to get started?