Using the FreshService API to Get Departments in Python
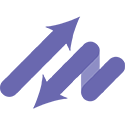
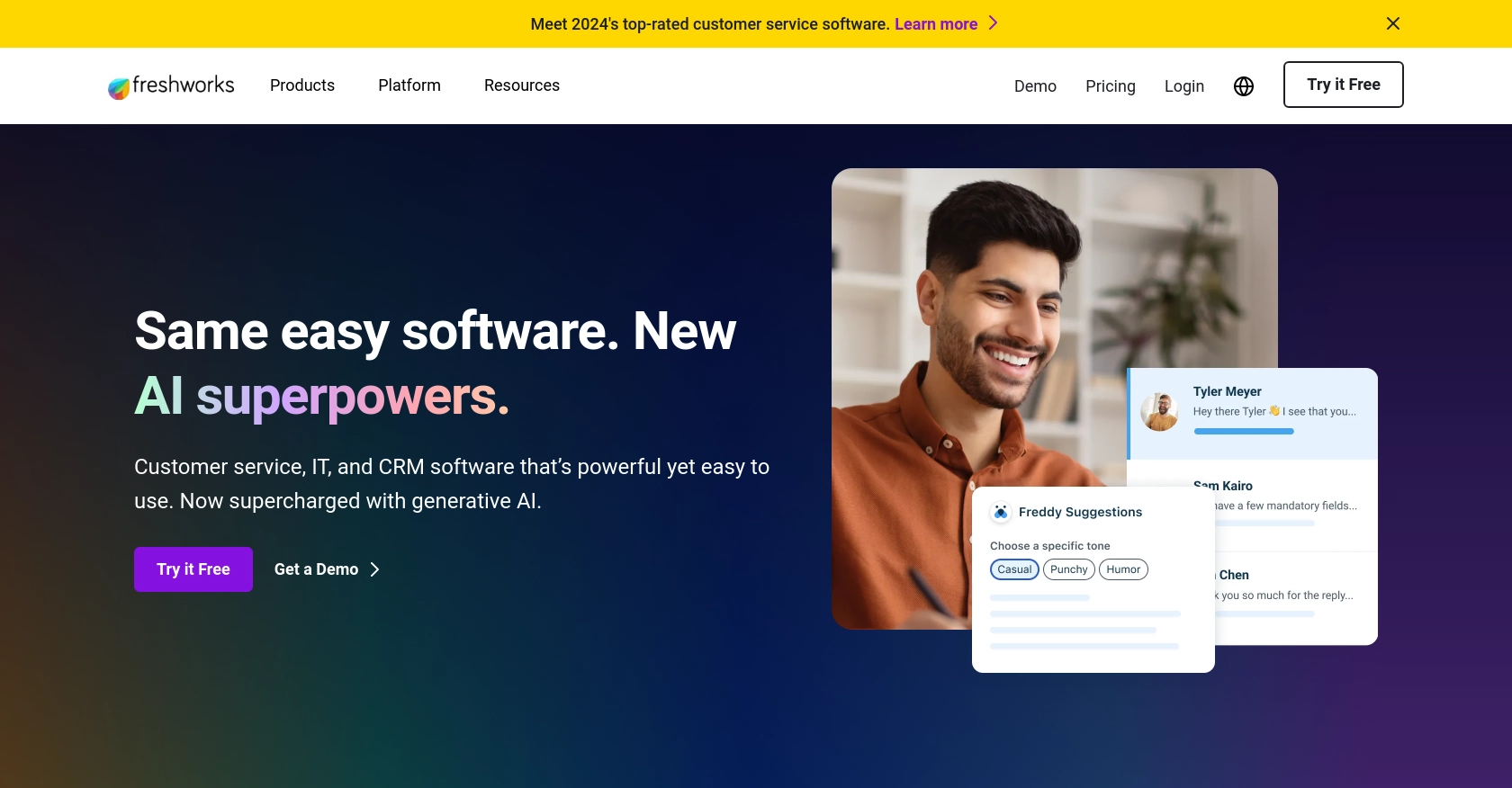
Introduction to FreshService API
FreshService is a cloud-based IT service management solution designed to simplify and enhance IT support processes for businesses. It offers a comprehensive suite of tools for incident management, asset management, change management, and more, making it a popular choice for IT teams looking to streamline their operations.
Integrating with the FreshService API allows developers to automate and manage various IT service tasks programmatically. For example, you might want to retrieve department information to generate reports or integrate with other systems for seamless data synchronization. This article will guide you through using Python to interact with the FreshService API to get department details efficiently.
Setting Up Your FreshService Test/Sandbox Account
Before you can start interacting with the FreshService API, you need to set up a test or sandbox account. This will allow you to safely experiment with API calls without affecting live data.
Creating a FreshService Account
If you don't already have a FreshService account, you can sign up for a free trial on the FreshService website. This trial will give you access to the necessary features to test API interactions.
- Visit the FreshService website and click on the "Free Trial" button.
- Fill in the required information, such as your name, email, and company details.
- Follow the instructions to complete the registration process.
Generating API Key for FreshService
FreshService uses API key-based authentication to authorize API requests. Follow these steps to generate your API key:
- Log in to your FreshService account.
- Navigate to your profile settings by clicking on your profile icon in the top right corner.
- Select "Profile Settings" from the dropdown menu.
- In the "Your API Key" section, you will find your API key. Copy this key and store it securely, as you will need it to authenticate your API requests.
With your FreshService account set up and your API key in hand, you're ready to start making API calls to retrieve department information using Python.
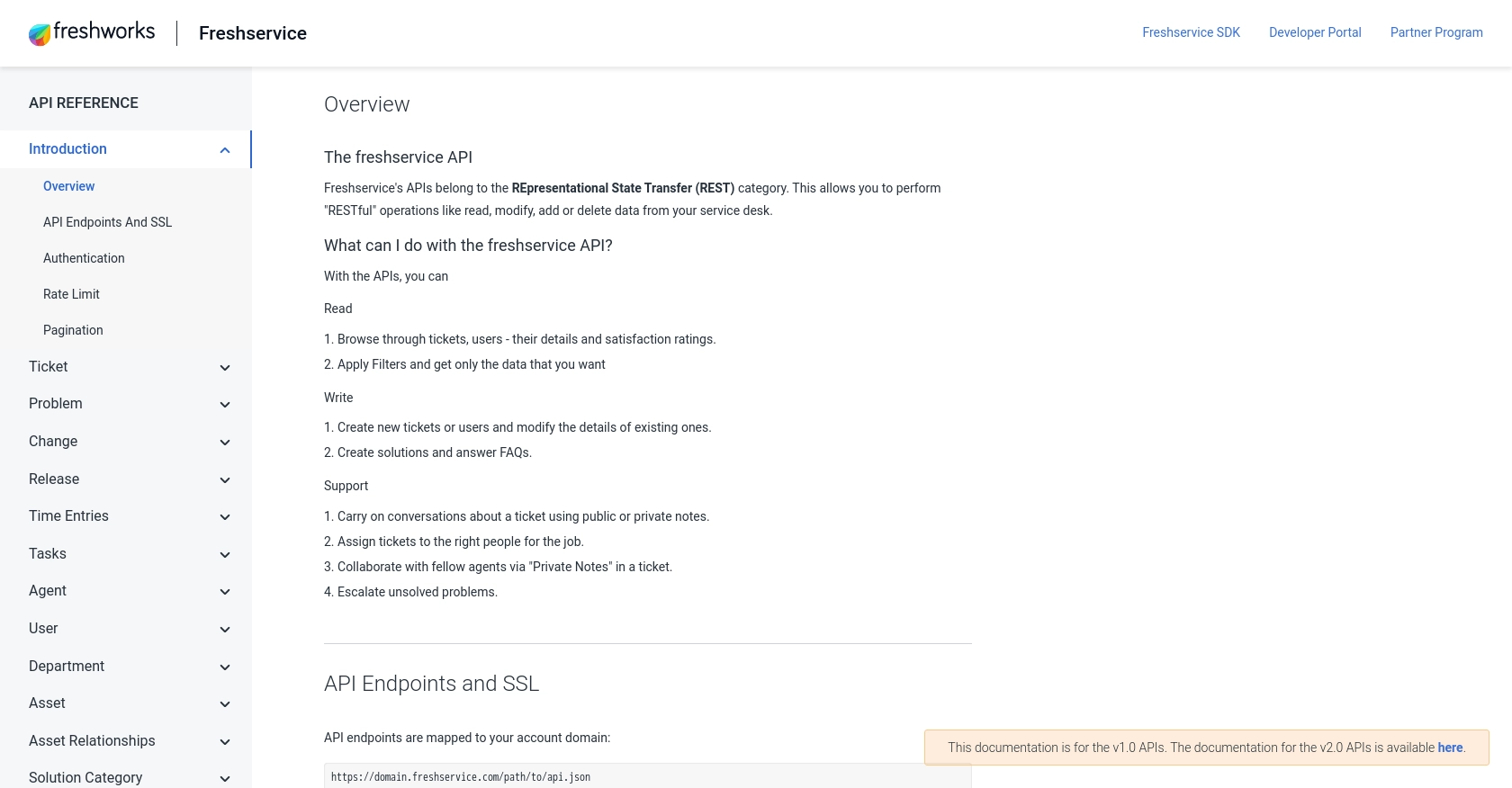
sbb-itb-96038d7
Making API Calls to Retrieve Department Information from FreshService Using Python
To interact with the FreshService API and retrieve department information, you'll need to use Python. This section will guide you through the necessary steps, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your Python Environment for FreshService API Integration
Before making API calls, ensure you have the following prerequisites installed on your machine:
- Python 3.11.1
- The Python package installer, pip
Once you have these installed, open your terminal or command prompt and install the requests
library, which is essential for making HTTP requests:
pip install requests
Writing Python Code to Retrieve Departments from FreshService
Create a new Python file named get_freshservice_departments.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://api.freshservice.com/v1/departments"
headers = {
"Content-Type": "application/json",
"Authorization": "Basic Your_API_Key"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON data from the response
departments = response.json()
for department in departments:
print(department)
else:
print(f"Failed to retrieve departments. Status code: {response.status_code}")
Replace Your_API_Key
with the API key you obtained from your FreshService account.
Running the Python Script and Verifying the Output
Run the script from your terminal or command line using the following command:
python get_freshservice_departments.py
If successful, you should see a list of departments printed in your terminal. This confirms that your API call to FreshService was successful and the data was retrieved correctly.
Handling Errors and Understanding FreshService API Response Codes
When making API calls, it's crucial to handle potential errors gracefully. The FreshService API may return various status codes indicating the result of your request:
- 200 OK: The request was successful, and the department data is returned.
- 401 Unauthorized: Authentication failed. Check your API key.
- 403 Forbidden: You do not have permission to access the resource.
- 404 Not Found: The requested resource does not exist.
- 500 Internal Server Error: An error occurred on the server. Try again later.
By checking the status code in your script, you can implement logic to handle these errors appropriately, ensuring a robust integration with the FreshService API.
Conclusion and Best Practices for Using FreshService API in Python
Integrating with the FreshService API using Python allows developers to efficiently manage IT service tasks, such as retrieving department information. By following the steps outlined in this article, you can set up your environment, authenticate using your API key, and handle API responses effectively.
Best Practices for Secure and Efficient FreshService API Integration
- Securely Store API Keys: Always store your API keys securely, such as in environment variables or a secure vault, to prevent unauthorized access.
- Handle Rate Limiting: Be mindful of FreshService's rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit errors gracefully.
- Standardize Data Fields: Ensure that data retrieved from FreshService is transformed and standardized to match your application's data structures for seamless integration.
- Monitor API Usage: Regularly monitor your API usage and logs to detect any anomalies or unauthorized access attempts.
Streamline Your Integrations with Endgrate
While integrating with FreshService API can be straightforward, managing multiple integrations can become complex and time-consuming. Endgrate offers a unified API solution that simplifies integration processes across various platforms, including FreshService.
By using Endgrate, you can save time and resources, allowing your team to focus on core product development. With a single API endpoint, you can build once for each use case and enjoy an intuitive integration experience for your customers.
Explore how Endgrate can enhance your integration strategy by visiting Endgrate's website and discover the benefits of a streamlined, efficient integration process.
Read More
Ready to get started?