How to Get Products (Professional/Enterprise Only) with the Hubspot API in Python
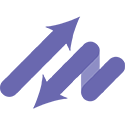
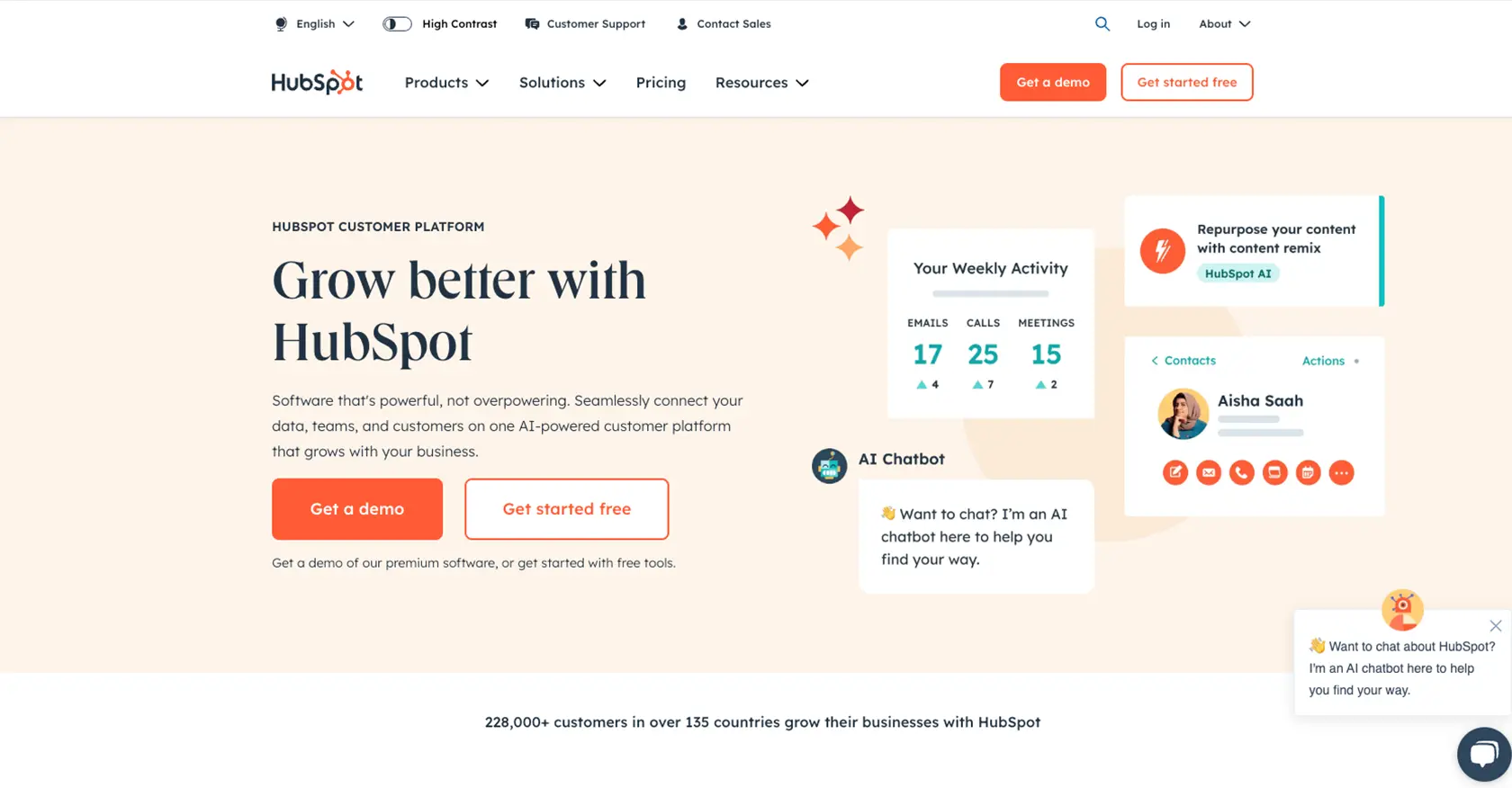
Introduction to HubSpot API for Product Management
HubSpot is a comprehensive CRM platform that offers a wide range of tools to help businesses manage their marketing, sales, and customer service efforts. Known for its user-friendly interface and robust features, HubSpot is a popular choice for companies looking to streamline their operations and improve customer engagement.
For developers working with B2B SaaS products, integrating with HubSpot's API can significantly enhance the ability to manage product data efficiently. By leveraging the HubSpot API, developers can access and manipulate product information, enabling seamless integration with other business systems.
One practical use case for integrating with the HubSpot API is retrieving product data for Professional and Enterprise accounts. This can be particularly useful for developers looking to synchronize product catalogs across different platforms, ensuring consistency and accuracy in product information.
Setting Up Your HubSpot Test/Sandbox Account for API Integration
Before you can start interacting with the HubSpot API to manage products, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data.
Creating a HubSpot Developer Account
If you don't already have a HubSpot developer account, you'll need to create one. Follow these steps to get started:
- Visit the HubSpot Developer Portal.
- Click on "Create a developer account" and fill out the necessary information.
- Once your account is set up, log in to access the developer dashboard.
Setting Up a HubSpot Sandbox Account
With your developer account ready, you can now create a sandbox account:
- Navigate to the "Test Accounts" section in your developer dashboard.
- Click on "Create a test account" to generate a new sandbox environment.
- Configure the sandbox to mimic your desired production settings.
Configuring OAuth for HubSpot API Access
HubSpot uses OAuth for API authentication. Follow these steps to set up OAuth:
- In your developer account, go to the "Apps" section and click "Create App."
- Provide a name and description for your app.
- Under "Auth," select OAuth and configure the redirect URL.
- Save your app to generate a client ID and client secret.
Ensure you have the necessary scopes for accessing product data. Refer to the HubSpot Scopes Documentation for more details.
Generating OAuth Tokens
To authenticate API requests, you'll need to generate OAuth tokens:
- Direct users to the authorization URL to obtain an authorization code.
- Exchange the authorization code for an access token using the token endpoint.
- Store the access token securely for future API calls.
For detailed steps on working with OAuth, visit the HubSpot Authentication Documentation.
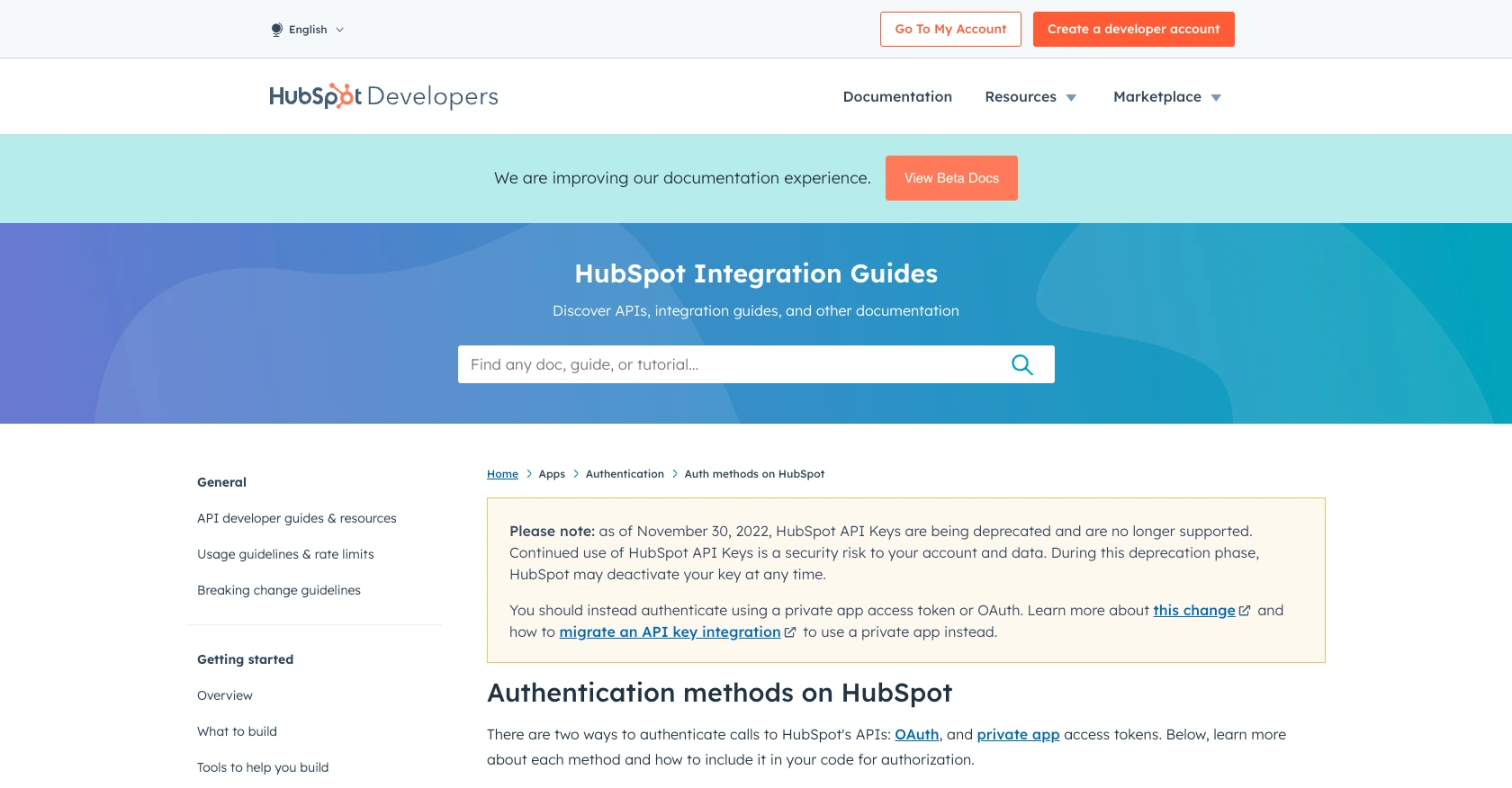
sbb-itb-96038d7
Making the API Call to Retrieve Products Using HubSpot API in Python
To interact with the HubSpot API for retrieving product data, you'll need to use Python. This section will guide you through setting up your environment, writing the necessary code, and handling the API response effectively.
Setting Up Your Python Environment for HubSpot API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python from the official Python website if you haven't already.
- Install the
requests
library using pip:
pip install requests
Writing the Python Code to Retrieve Products from HubSpot
Create a new Python file named get_hubspot_products.py
and add the following code:
import requests
# Set the API endpoint and headers
endpoint = "https://api.hubapi.com/crm/v3/objects/products"
headers = {"Authorization": "Bearer Your_Access_Token"}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
# Parse the JSON data from the response
data = response.json()
# Loop through the products and print their information
for product in data['results']:
print(f"Product ID: {product['id']}, Name: {product['properties']['name']}")
else:
print(f"Failed to retrieve products: {response.status_code} - {response.text}")
Replace Your_Access_Token
with the OAuth token you generated earlier.
Running the Code and Verifying the API Response
Execute the script from your terminal or command line:
python get_hubspot_products.py
If successful, the script will output a list of products with their IDs and names. If there are any errors, the script will print the error code and message.
Handling Errors and Understanding HubSpot API Error Codes
When making API calls, it's crucial to handle potential errors gracefully. The HubSpot API may return various error codes, such as:
- 401 Unauthorized: Indicates an issue with your OAuth token. Ensure it's valid and hasn't expired.
- 429 Too Many Requests: You've hit the rate limit. HubSpot allows 150 requests per 10 seconds for Professional and Enterprise accounts. Consider implementing a retry mechanism with exponential backoff.
- 500 Internal Server Error: A server-side issue. Retry the request after some time.
For more details on error handling, refer to the HubSpot API Usage Guidelines.
.webp)
Conclusion and Best Practices for HubSpot API Integration
Integrating with the HubSpot API to manage product data can significantly enhance your application's capabilities, especially for Professional and Enterprise accounts. By following the steps outlined in this guide, you can efficiently retrieve product information and ensure seamless synchronization across platforms.
Best Practices for Secure and Efficient API Usage
- Secure Storage of OAuth Tokens: Always store your OAuth tokens securely. Consider using environment variables or secure vaults to prevent unauthorized access.
- Implement Rate Limiting Strategies: HubSpot enforces a rate limit of 150 requests per 10 seconds for Professional and Enterprise accounts. Implement retry mechanisms with exponential backoff to handle rate limit errors gracefully.
- Data Standardization: Ensure that product data retrieved from HubSpot is standardized and transformed as needed to maintain consistency across your systems.
Enhance Your Integration Experience with Endgrate
While building integrations can be complex, tools like Endgrate simplify the process by providing a unified API endpoint for multiple platforms, including HubSpot. By leveraging Endgrate, you can save time and resources, allowing you to focus on your core product development.
Explore how Endgrate can streamline your integration efforts and provide an intuitive experience for your customers. Visit Endgrate to learn more and start optimizing your integration processes today.
Read More
- https://endgrate.com/provider/hubspot
- https://developers.hubspot.com/docs/api/overview
- https://developers.hubspot.com/docs/api/intro-to-auth
- https://developers.hubspot.com/docs/api/usage-details
- https://developers.hubspot.com/docs/api/scopes
- https://developers.hubspot.com/docs/api/crm/products
- https://developers.hubspot.com/docs/api/crm/properties
Ready to get started?