How to Get Contacts with the Teamwork CRM API in PHP
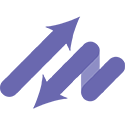
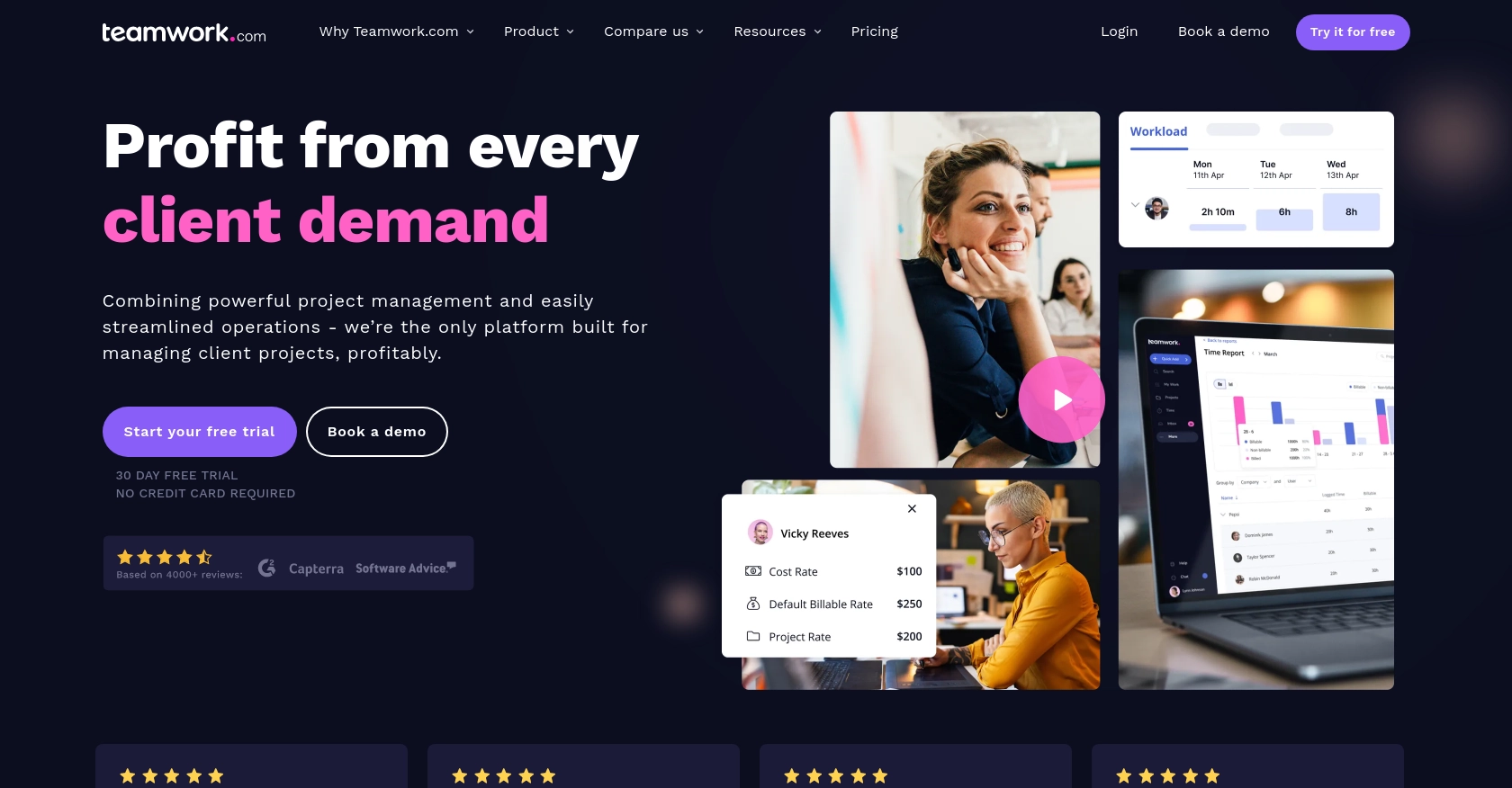
Introduction to Teamwork CRM
Teamwork CRM is a powerful customer relationship management tool designed to help businesses manage their sales processes and customer interactions more effectively. With features such as deal tracking, contact management, and sales forecasting, Teamwork CRM provides a comprehensive solution for sales teams looking to streamline their workflows and improve productivity.
Integrating with Teamwork CRM's API allows developers to automate various sales and customer management tasks. For example, you can use the API to retrieve contact information and synchronize it with other systems, ensuring that your sales team always has access to the most up-to-date customer data.
Setting Up Your Teamwork CRM Test Account
Before you can start integrating with the Teamwork CRM API, you'll need to set up a test account. This will allow you to safely experiment with API calls without affecting your live data.
Create a Teamwork CRM Account
If you don't already have a Teamwork CRM account, you can sign up for a free trial on the Teamwork website. This trial will give you access to all the features you need to test the API integration.
- Visit the Teamwork CRM website.
- Click on the "Start Free Trial" button.
- Follow the on-screen instructions to create your account.
Generate an API Key for Teamwork CRM
Once your account is set up, you'll need to generate an API key to authenticate your requests. Teamwork CRM uses Basic Authentication, which requires a base64-encoded string of your API key and password.
- Log in to your Teamwork CRM account.
- Navigate to the "Settings" section.
- Find the "API & Webhooks" tab and click on it.
- Generate a new API key and make sure to store it securely.
Configure OAuth 2.0 for Teamwork CRM
If you prefer using OAuth 2.0 for authentication, you'll need to register your application through the Teamwork Developer Portal.
- Go to the Teamwork Developer Portal.
- Register your application to obtain a client ID and secret.
- Implement the OAuth 2.0 App Login Flow using these credentials.
- Ensure you include the bearer token in the Authorization header of your API requests.
For more detailed information on authentication, refer to the Teamwork CRM Authentication Documentation.
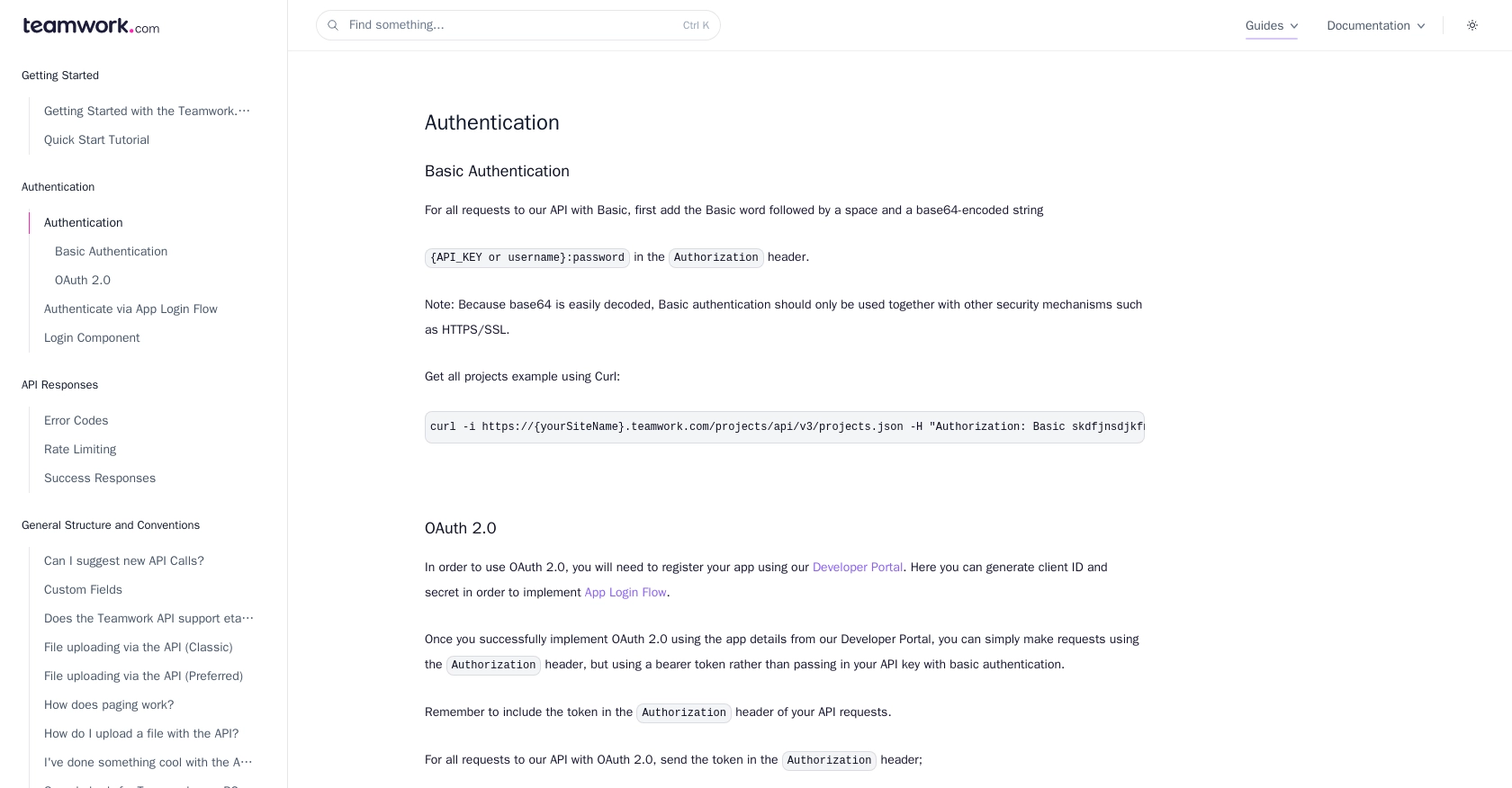
sbb-itb-96038d7
Making API Calls to Retrieve Contacts from Teamwork CRM Using PHP
To interact with the Teamwork CRM API and retrieve contact information, you'll need to write a PHP script that makes HTTP requests to the API endpoints. This section will guide you through the process of setting up your PHP environment, writing the necessary code, and handling potential errors.
Setting Up Your PHP Environment for Teamwork CRM API Integration
Before you begin, ensure that you have PHP installed on your machine. You can download it from the official PHP website. Additionally, you'll need to install the cURL extension, which is commonly used for making HTTP requests in PHP.
- Verify your PHP installation by running
php -v
in your terminal. - Ensure cURL is enabled by checking your
php.ini
file for the lineextension=curl
.
Writing the PHP Code to Retrieve Contacts from Teamwork CRM
Now that your environment is set up, you can write the PHP script to make an API call to Teamwork CRM and retrieve contact information. The following code demonstrates how to achieve this using Basic Authentication.
<?php
// Set the API endpoint URL
$endpoint = 'https://{yourSiteName}.teamwork.com/crm/v2/contacts.json';
// Your API key and password (base64-encoded)
$apiKey = 'your_api_key';
$password = '';
// Create a base64-encoded string for Basic Authentication
$authHeader = 'Authorization: Basic ' . base64_encode("$apiKey:$password");
// Initialize cURL session
$ch = curl_init($endpoint);
// Set cURL options
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, array($authHeader));
// Execute the cURL request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Request Error:' . curl_error($ch);
} else {
// Decode the JSON response
$data = json_decode($response, true);
// Display the contacts
foreach ($data['contacts'] as $contact) {
echo 'Name: ' . $contact['name'] . '<br>';
echo 'Email: ' . $contact['email'] . '<br><br>';
}
}
// Close the cURL session
curl_close($ch);
?>
Replace your_api_key
with the API key you generated earlier. Also, ensure you replace {yourSiteName}
with your actual Teamwork site name.
Verifying the API Request and Handling Errors
After running the script, you should see a list of contacts displayed. If the request is successful, the API will return a 200 OK status code. You can verify the contacts in your Teamwork CRM test account to ensure they match the retrieved data.
In case of errors, the API might return various status codes:
- 401 Unauthorized: Check your API key and ensure it's correctly set up.
- 404 Not Found: Verify the endpoint URL.
- 429 Rate Limit Exceeded: Teamwork CRM allows up to 150 requests per minute for most plans. If you exceed this, you'll receive a 429 error. Check the response headers for rate limit details.
For more information on error codes, refer to the Teamwork CRM Error Codes Documentation.
Conclusion and Best Practices for Integrating with Teamwork CRM API Using PHP
Integrating with the Teamwork CRM API using PHP provides a powerful way to automate and streamline your sales and customer management processes. By following the steps outlined in this guide, you can efficiently retrieve contact information and synchronize it with other systems, ensuring your sales team has access to the most current data.
Best Practices for Secure and Efficient API Integration with Teamwork CRM
- Securely Store API Credentials: Always store your API keys and OAuth tokens securely. Avoid hardcoding them in your scripts and consider using environment variables or secure vaults.
- Handle Rate Limiting: Be mindful of Teamwork CRM's rate limits, which allow up to 150 requests per minute for most plans. Implement retry logic and check the response headers for rate limit details to avoid disruptions.
- Implement Error Handling: Ensure robust error handling in your scripts to manage different API response codes effectively. Refer to the Teamwork CRM Error Codes Documentation for guidance.
- Data Transformation and Standardization: When integrating data from multiple sources, standardize data fields to maintain consistency across systems.
Streamline Your Integration Process with Endgrate
While integrating with APIs like Teamwork CRM can be rewarding, it can also be time-consuming and complex. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including Teamwork CRM. This allows you to build once for each use case instead of multiple times for different integrations, saving time and resources.
Explore how Endgrate can simplify your integration process and provide an intuitive experience for your customers by visiting Endgrate.
Read More
Ready to get started?