Using the BigCommerce API to Get Product Categories in PHP
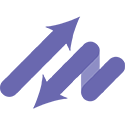
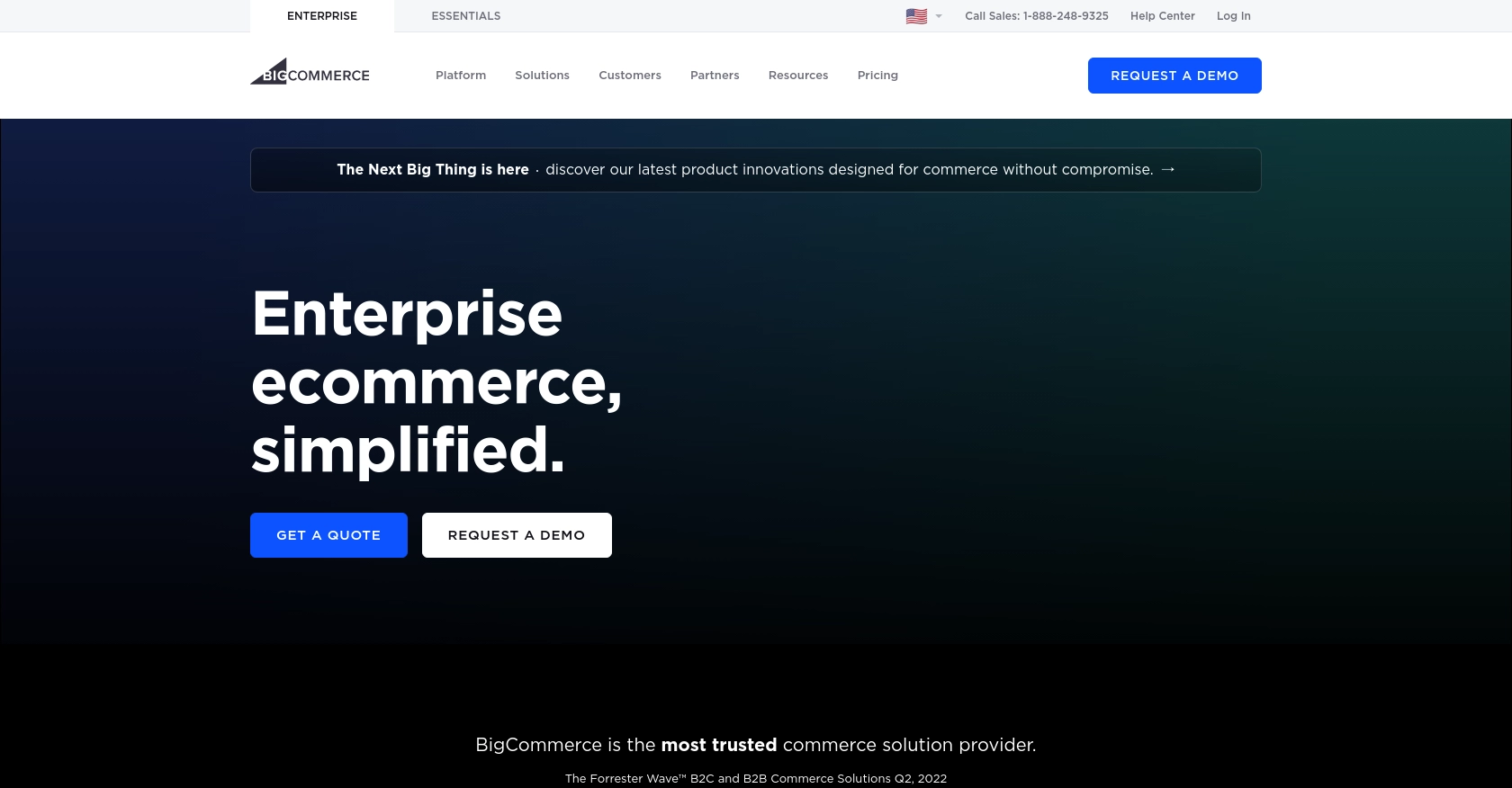
Introduction to BigCommerce API Integration
BigCommerce is a robust e-commerce platform that empowers businesses to build, innovate, and grow their online stores. With its comprehensive suite of tools, BigCommerce offers flexibility and scalability for businesses of all sizes, making it a popular choice for online retailers.
Integrating with the BigCommerce API allows developers to manage store data programmatically, enhancing the functionality and efficiency of e-commerce operations. For example, accessing product categories through the BigCommerce API can help developers organize and display products effectively, improving the shopping experience for customers.
This article will guide you through the process of using PHP to interact with the BigCommerce API, specifically focusing on retrieving product categories. By the end of this tutorial, you will be able to seamlessly integrate BigCommerce's product category data into your applications, streamlining your e-commerce management tasks.
Setting Up Your BigCommerce Test Account for API Integration
Before diving into the integration process, it's essential to set up a BigCommerce test account. This will allow you to safely experiment with API calls without affecting a live store. BigCommerce provides sandbox accounts for developers to test and develop their applications.
Creating a BigCommerce Sandbox Account
To begin, you need to create a BigCommerce sandbox account. Follow these steps to set up your account:
- Visit the BigCommerce Developer Portal.
- Sign up for a developer account if you don't already have one.
- Once logged in, navigate to the "Sandbox Stores" section.
- Create a new sandbox store by following the on-screen instructions.
After setting up your sandbox store, you will have access to a test environment where you can safely make API calls.
Generating API Credentials for BigCommerce
To interact with the BigCommerce API, you need to generate API credentials. These credentials will allow you to authenticate your requests. Follow these steps to create an API account:
- Log in to your BigCommerce sandbox store's admin panel.
- Navigate to Advanced Settings > API Accounts.
- Click on Create API Account.
- Select V2/V3 API Token as the type.
- Provide a name for your API account and set the necessary OAuth scopes for accessing product categories.
- Click Save to generate your API credentials, including the Client ID, Client Secret, and Access Token.
Ensure you store these credentials securely, as they will be used to authenticate your API requests.
Understanding BigCommerce API Authentication
The BigCommerce API uses the X-Auth-Token
header for authentication. You will need to include your access token in this header for each API request. For more details on authentication, refer to the BigCommerce Authentication Documentation.
With your sandbox account and API credentials ready, you can now proceed to make API calls to retrieve product categories using PHP.
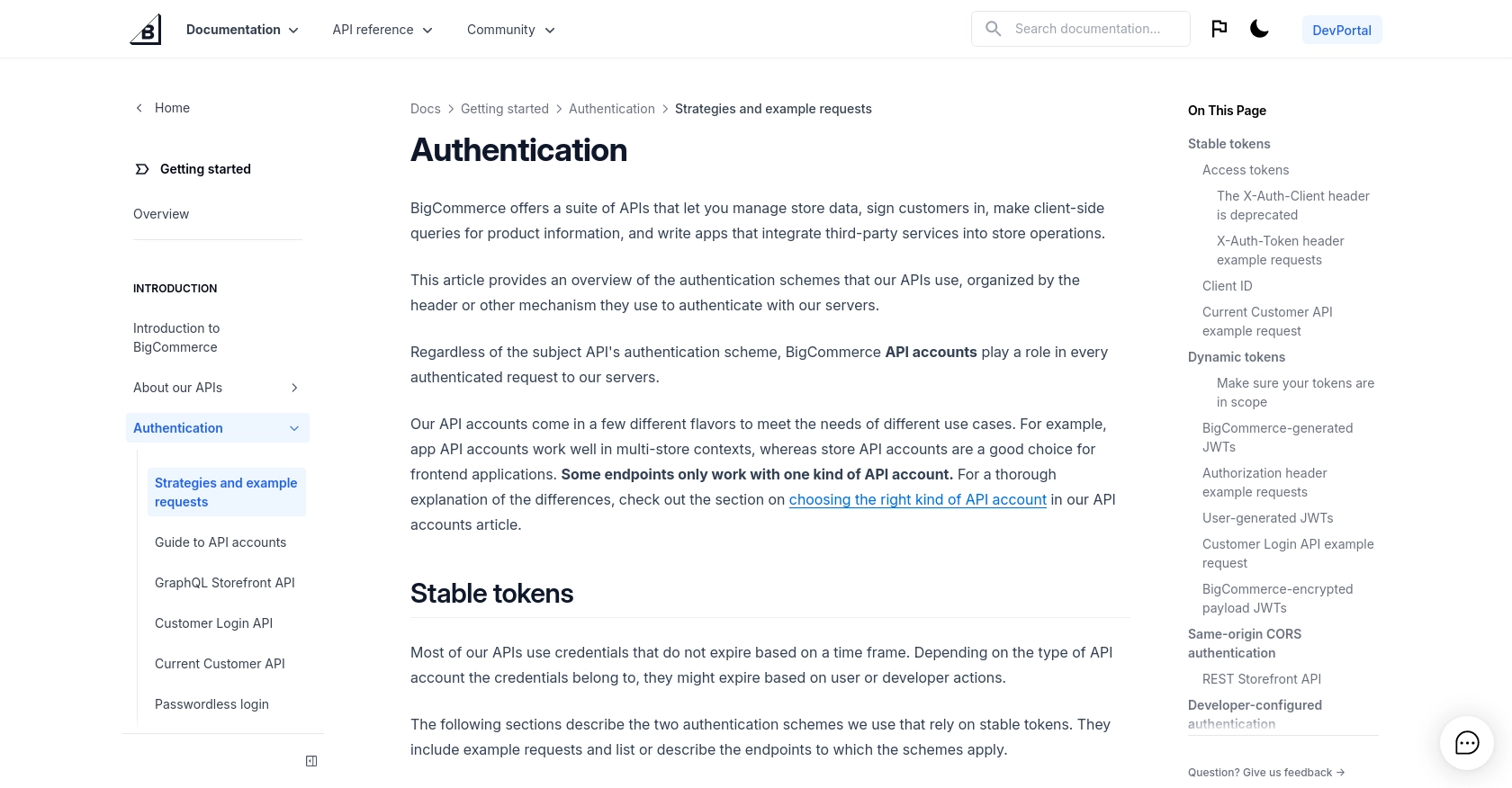
sbb-itb-96038d7
Making API Calls to Retrieve Product Categories from BigCommerce Using PHP
To interact with the BigCommerce API and retrieve product categories, you'll need to use PHP to send HTTP requests. This section will guide you through the process of setting up your PHP environment, making the API call, and handling the response.
Setting Up Your PHP Environment for BigCommerce API Integration
Before making API calls, ensure your PHP environment is properly configured. You will need:
- PHP 7.4 or later
- cURL extension enabled
To check your PHP version and enabled extensions, you can use the following command:
php -v
php -m | grep curl
Installing Required PHP Dependencies for BigCommerce API
To simplify HTTP requests, you can use the Guzzle HTTP client. Install it via Composer with the following command:
composer require guzzlehttp/guzzle
Example Code to Retrieve Product Categories from BigCommerce
With your environment set up, you can now write PHP code to make an API call to BigCommerce. Below is an example script to retrieve product categories:
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$client = new Client();
$storeHash = 'your_store_hash';
$accessToken = 'your_access_token';
$response = $client->request('GET', "https://api.bigcommerce.com/stores/{$storeHash}/v3/catalog/categories", [
'headers' => [
'X-Auth-Token' => $accessToken,
'Accept' => 'application/json',
]
]);
if ($response->getStatusCode() === 200) {
$categories = json_decode($response->getBody(), true);
foreach ($categories['data'] as $category) {
echo "Category ID: " . $category['id'] . " - Name: " . $category['name'] . "\n";
}
} else {
echo "Failed to retrieve categories. Status code: " . $response->getStatusCode();
}
Replace your_store_hash
and your_access_token
with your actual store hash and access token. This script uses Guzzle to send a GET request to the BigCommerce API, retrieves the product categories, and prints their IDs and names.
Verifying the API Call and Handling Errors
After running the script, verify the output to ensure the categories are retrieved correctly. If the request fails, check the status code and error message. Common error codes include:
- 401 Unauthorized: Check your access token.
- 404 Not Found: Verify the endpoint URL and store hash.
- 429 Too Many Requests: You've hit the rate limit of 40 requests per minute.
For more details on error codes, refer to the BigCommerce API documentation.
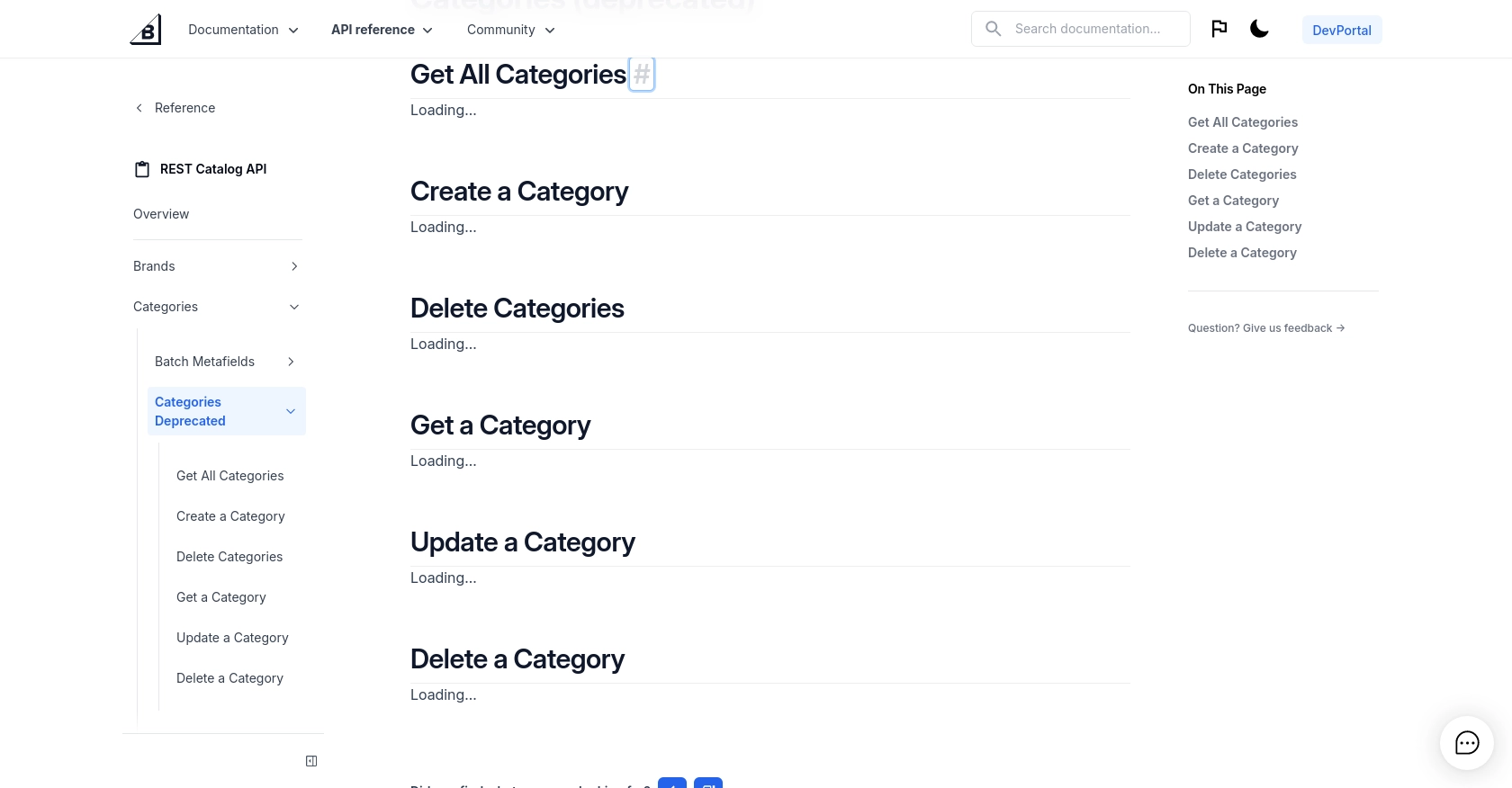
Conclusion and Best Practices for BigCommerce API Integration Using PHP
Integrating with the BigCommerce API using PHP allows developers to efficiently manage e-commerce data, such as product categories, enhancing the functionality of online stores. By following the steps outlined in this article, you can seamlessly retrieve and utilize product category data to improve your store's organization and customer experience.
Best Practices for Secure and Efficient BigCommerce API Usage
- Securely Store Credentials: Ensure that your API credentials, such as the access token, are stored securely and not hard-coded in your scripts. Consider using environment variables or secure vaults.
- Handle Rate Limits: Be mindful of BigCommerce's rate limit of 40 requests per minute. Implement logic to handle rate limiting gracefully, such as retry mechanisms or request throttling.
- Standardize Data: When integrating data from BigCommerce, consider transforming and standardizing it to fit your application's data model, ensuring consistency across platforms.
- Error Handling: Implement robust error handling to manage API response errors effectively. Log errors for debugging and provide user-friendly messages where applicable.
Streamlining Integrations with Endgrate
While integrating with APIs like BigCommerce can be powerful, it can also be time-consuming and complex. Endgrate offers a solution by providing a unified API endpoint that connects to multiple platforms, including BigCommerce. This allows developers to build integrations once and use them across various platforms, saving time and resources.
By leveraging Endgrate, you can focus on your core product while outsourcing the complexities of integration management. Explore how Endgrate can enhance your integration strategy by visiting Endgrate.
Read More
Ready to get started?