Using the Hubspot API to Get Custom Objects (with Python examples)
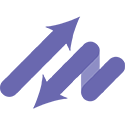
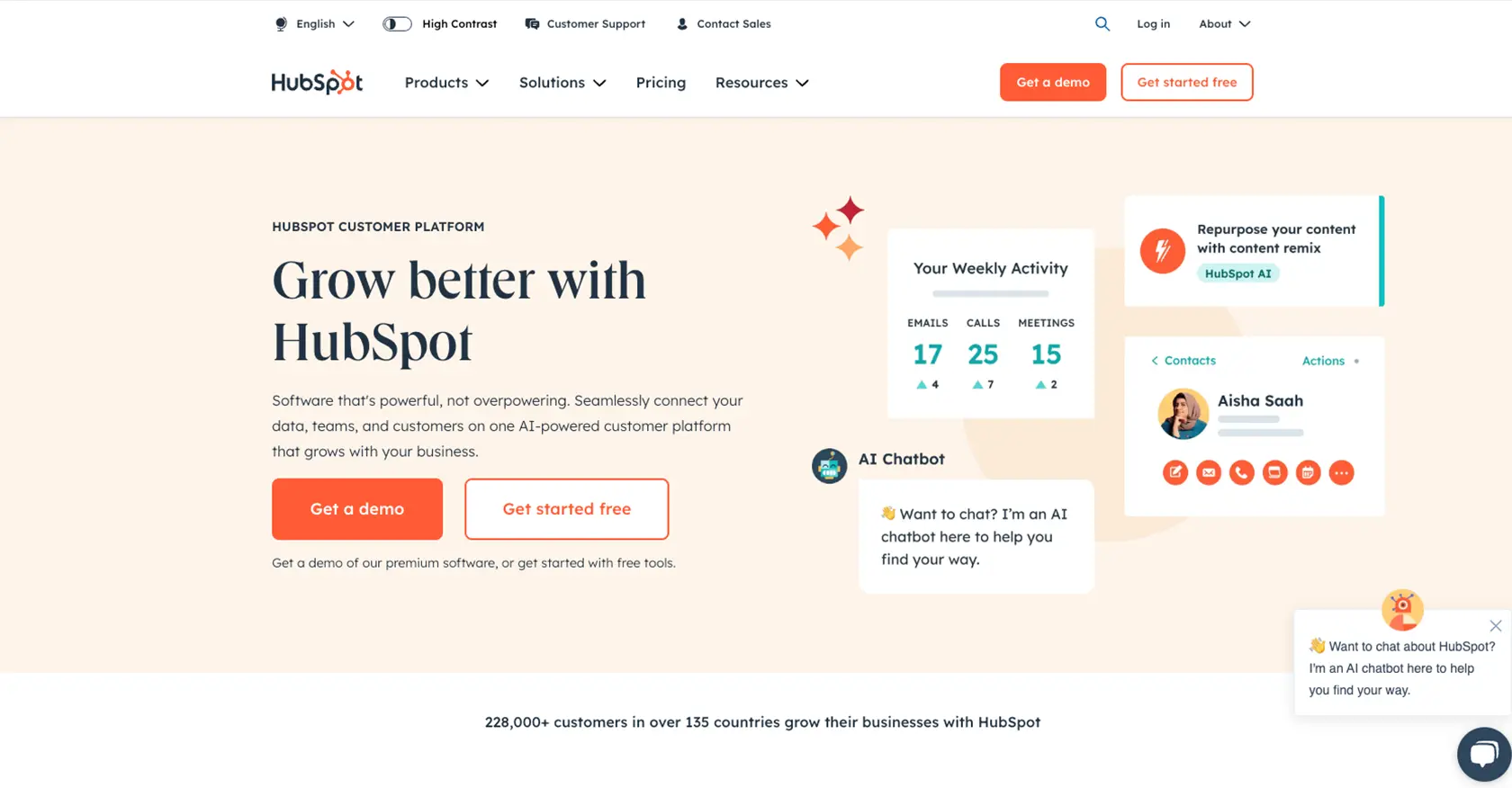
Introduction to HubSpot Custom Objects API
HubSpot is a versatile CRM platform that offers a wide range of tools to help businesses manage their marketing, sales, and customer service efforts. One of its powerful features is the ability to create and manage custom objects, allowing businesses to tailor their CRM data structure to fit specific needs.
Developers might want to integrate with HubSpot's Custom Objects API to enhance data management and create personalized solutions. For example, a developer could use the API to retrieve custom object data and integrate it into a bespoke reporting tool, providing deeper insights into customer interactions.
This article will guide you through using Python to interact with HubSpot's Custom Objects API, offering detailed steps to efficiently access and manipulate custom object data on the HubSpot platform.
Setting Up Your HubSpot Developer Account for Custom Objects API
Before you can start interacting with HubSpot's Custom Objects API, you'll need to set up a developer account and create a private app. This setup will allow you to authenticate your API requests securely using OAuth, ensuring that your application can access the necessary data.
Step 1: Create a HubSpot Developer Account
If you don't already have a HubSpot developer account, you'll need to create one. Follow these steps:
- Visit the HubSpot Developer Portal.
- Click on "Create a developer account" and fill in the required information.
- Once your account is created, log in to access the developer dashboard.
Step 2: Set Up a HubSpot Sandbox Account
To test your API interactions, it's recommended to use a sandbox account. Here's how to set it up:
- In your developer dashboard, navigate to the "Test Accounts" section.
- Click on "Create a test account" to generate a sandbox environment.
- This sandbox will mimic a real HubSpot account, allowing you to safely test your API calls.
Step 3: Create a Private App for OAuth Authentication
HubSpot uses OAuth for secure API authentication. Follow these steps to create a private app:
- In your HubSpot account, go to "Settings" and select "Integrations" from the sidebar.
- Click on "Private Apps" and then "Create a private app."
- Fill in the app details, including name and description.
- Navigate to the "Scopes" section and select the necessary scopes for accessing custom objects, such as
crm.objects.custom.read
andcrm.objects.custom.write
. - Click "Create app" to generate your OAuth credentials, including the client ID and client secret.
Step 4: Obtain OAuth Access Token
With your private app set up, you can now obtain an OAuth access token:
- Use the client ID and client secret to request an access token from HubSpot's OAuth server.
- Refer to the OAuth Quickstart Guide for detailed instructions on obtaining the token.
- Store the access token securely, as it will be used in the authorization header for your API requests.
With your developer account, sandbox environment, and OAuth setup complete, you're ready to start making API calls to interact with HubSpot's Custom Objects.
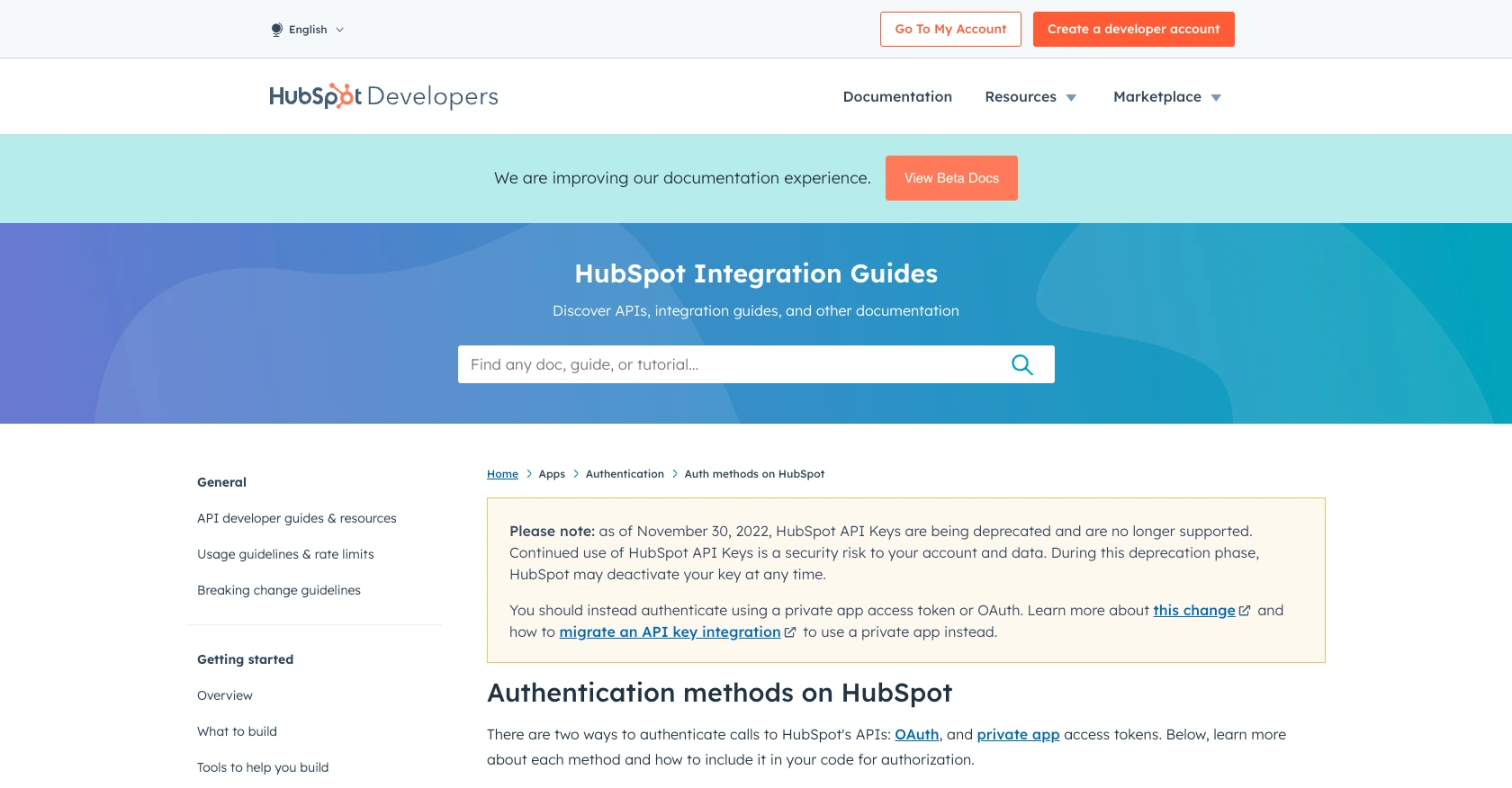
sbb-itb-96038d7
How to Make API Calls to HubSpot's Custom Objects Using Python
To interact with HubSpot's Custom Objects API using Python, you'll need to ensure your development environment is set up correctly. This section will guide you through the necessary steps to make API calls, including setting up your Python environment, writing the code, and handling responses.
Setting Up Your Python Environment for HubSpot API Integration
Before making API calls, ensure you have Python installed on your machine. This tutorial uses Python 3.11.1. Additionally, you'll need the requests
library to handle HTTP requests.
- Install Python 3.11.1 from the official Python website if you haven't already.
- Use pip to install the
requests
library by running the following command in your terminal:
pip install requests
Writing Python Code to Retrieve HubSpot Custom Objects
With your environment ready, you can now write a Python script to retrieve custom objects from HubSpot. Follow these steps:
import requests
# Define the API endpoint and headers
endpoint = "https://api.hubapi.com/crm/v3/objects/{objectType}"
headers = {
"Authorization": "Bearer Your_Access_Token",
"Content-Type": "application/json"
}
# Make a GET request to the API
response = requests.get(endpoint, headers=headers)
# Check if the request was successful
if response.status_code == 200:
data = response.json()
print("Custom Objects Retrieved Successfully:")
print(data)
else:
print(f"Failed to retrieve custom objects. Status Code: {response.status_code}")
print(response.json())
Replace Your_Access_Token
with the OAuth access token you obtained earlier. Also, replace {objectType}
with the specific custom object type you wish to retrieve.
Understanding and Handling API Responses
After running the script, you should see the custom objects data printed in your terminal if the request is successful. If the request fails, the script will print the status code and error message.
To verify the request's success, check your HubSpot sandbox account to ensure the data matches the retrieved information. This verification step is crucial for confirming that your API calls are functioning as expected.
Error Handling and Troubleshooting HubSpot API Calls
When working with APIs, it's essential to handle potential errors gracefully. Common error codes include:
- 401 Unauthorized: Ensure your access token is valid and has not expired.
- 404 Not Found: Verify the endpoint URL and object type are correct.
- 429 Too Many Requests: HubSpot's rate limit for OAuth apps is 100 requests every 10 seconds. Implement rate limiting in your code to avoid hitting this limit.
For more detailed error information, refer to the HubSpot API documentation.
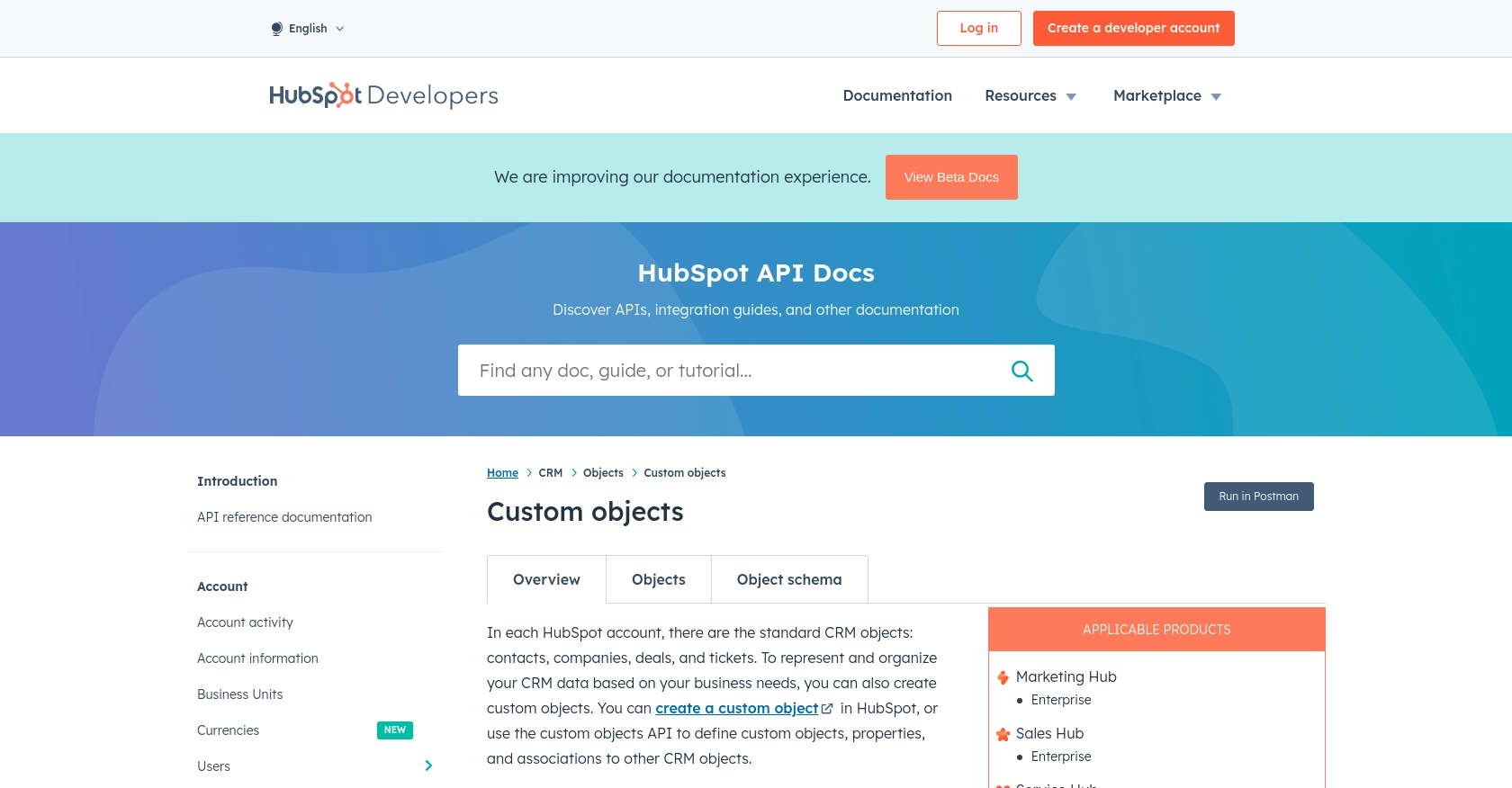
Conclusion and Best Practices for Using HubSpot Custom Objects API with Python
Integrating with HubSpot's Custom Objects API using Python can significantly enhance your ability to manage and customize CRM data. By following the steps outlined in this guide, you can efficiently retrieve and manipulate custom object data, enabling you to create tailored solutions that meet your business needs.
Best Practices for Secure and Efficient API Integration
- Securely Store Credentials: Always store your OAuth access tokens securely. Avoid hardcoding them in your scripts and consider using environment variables or secure vaults.
- Implement Rate Limiting: HubSpot's API rate limit for OAuth apps is 100 requests every 10 seconds. Ensure your application handles rate limiting to prevent hitting these limits and receiving 429 error responses.
- Data Standardization: When working with custom objects, ensure that data fields are standardized across your application to maintain consistency and improve data quality.
Next Steps with Endgrate for Seamless Integrations
While integrating with HubSpot's API directly can be powerful, it can also be time-consuming and complex, especially when managing multiple integrations. Consider using Endgrate to streamline your integration processes. With Endgrate, you can:
- Save time and resources by outsourcing integrations, allowing you to focus on your core product development.
- Build once for each use case instead of multiple times for different integrations, simplifying your development workflow.
- Provide an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website and discover the benefits of a unified API solution.
Read More
- https://endgrate.com/provider/hubspot
- https://developers.hubspot.com/docs/api/overview
- https://developers.hubspot.com/docs/api/intro-to-auth
- https://developers.hubspot.com/docs/api/usage-details
- https://developers.hubspot.com/docs/api/scopes
- https://developers.hubspot.com/docs/api/crm/crm-custom-objects
- https://developers.hubspot.com/docs/api/crm/properties
Ready to get started?