How to Get Users with the Cloze API in PHP
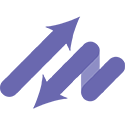
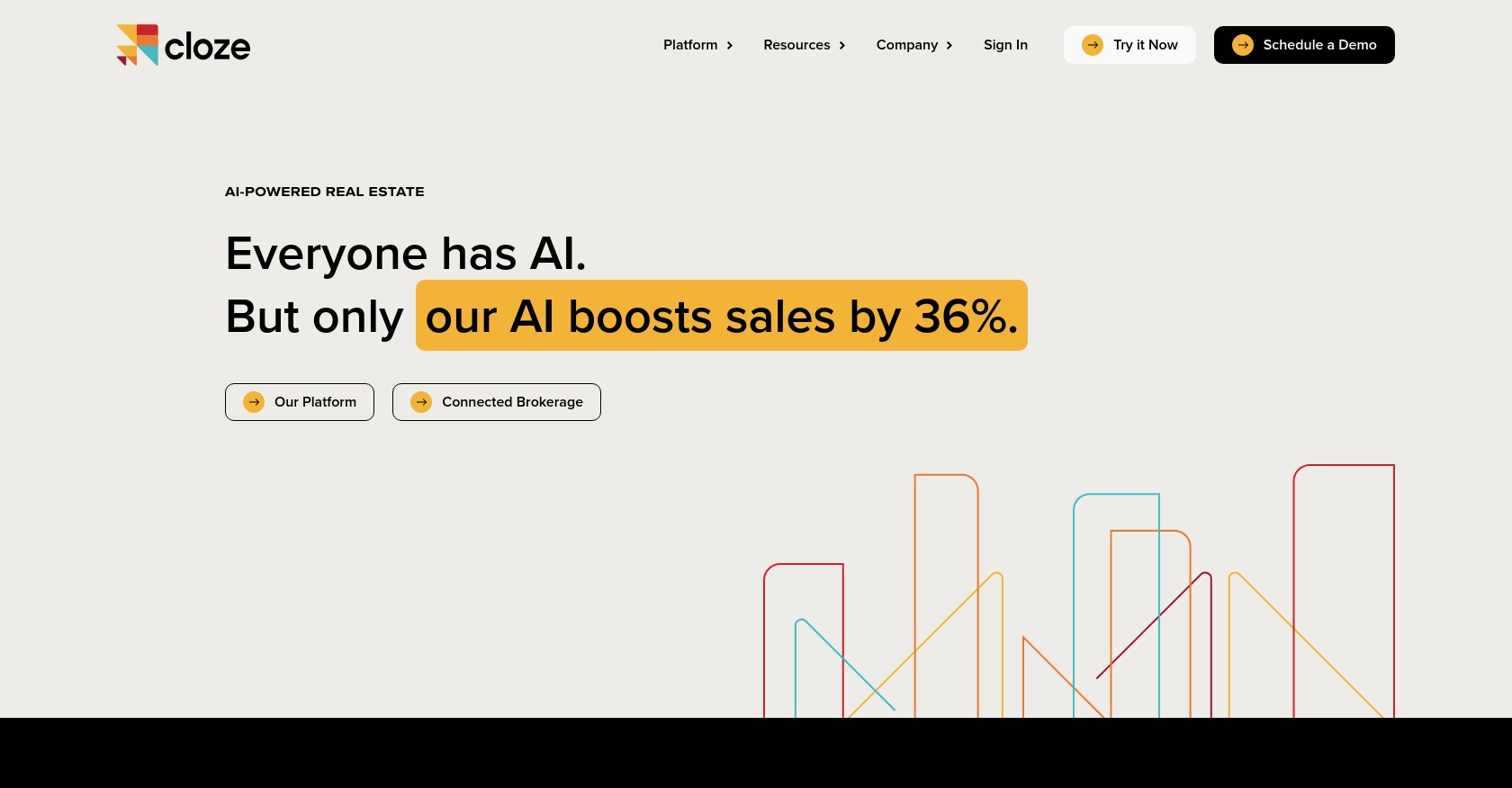
Introduction to Cloze API for User Management
Cloze is a powerful relationship management platform that helps businesses manage interactions with clients, partners, and team members. It offers a comprehensive suite of tools to streamline communication and enhance productivity by organizing emails, calls, meetings, and more.
For developers, integrating with the Cloze API can significantly enhance the ability to manage user data efficiently. By connecting to the Cloze API, you can automate tasks such as retrieving user information, updating profiles, and managing team members. This can be particularly useful for businesses looking to maintain up-to-date records and improve team collaboration.
In this article, we will explore how to use PHP to interact with the Cloze API to get user data. This integration can be beneficial for applications that require seamless access to user profiles and team information, enabling developers to build robust solutions that leverage Cloze's capabilities.
Setting Up Your Cloze API Test Account
Before you begin integrating with the Cloze API, it's essential to set up a test account. This will allow you to safely experiment with API calls without affecting live data. Cloze offers a straightforward process to get started with their API using an API key for authentication.
Create a Cloze Account
If you don't already have a Cloze account, you can sign up for a free trial on the Cloze website. This will give you access to the platform's features and allow you to generate an API key for development purposes.
Generate an API Key for Cloze API Access
Once you have your Cloze account set up, follow these steps to generate an API key:
- Log in to your Cloze account.
- Navigate to the settings section, usually found in the top navigation bar.
- Look for the API settings or developer options.
- Follow the instructions to create a new API key. You will need to provide your Cloze account email address as the user parameter and the generated API key as the api_key parameter.
- Save your API key securely, as you will need it to authenticate your API requests.
For more detailed instructions, you can refer to the Cloze API documentation: Cloze API Key Documentation.
Understanding Cloze API Authentication
The Cloze API supports both API key and OAuth2 authentication. For initial development and testing, using an API key is recommended. Ensure that you include the user and api_key parameters in your API requests to authenticate successfully.
If you plan to build a public integration intended for use by Cloze users outside your organization, you will need to implement OAuth2 authentication. For more information on OAuth2 setup, contact Cloze support.
With your test account and API key ready, you can now proceed to make API calls to retrieve user data using PHP.
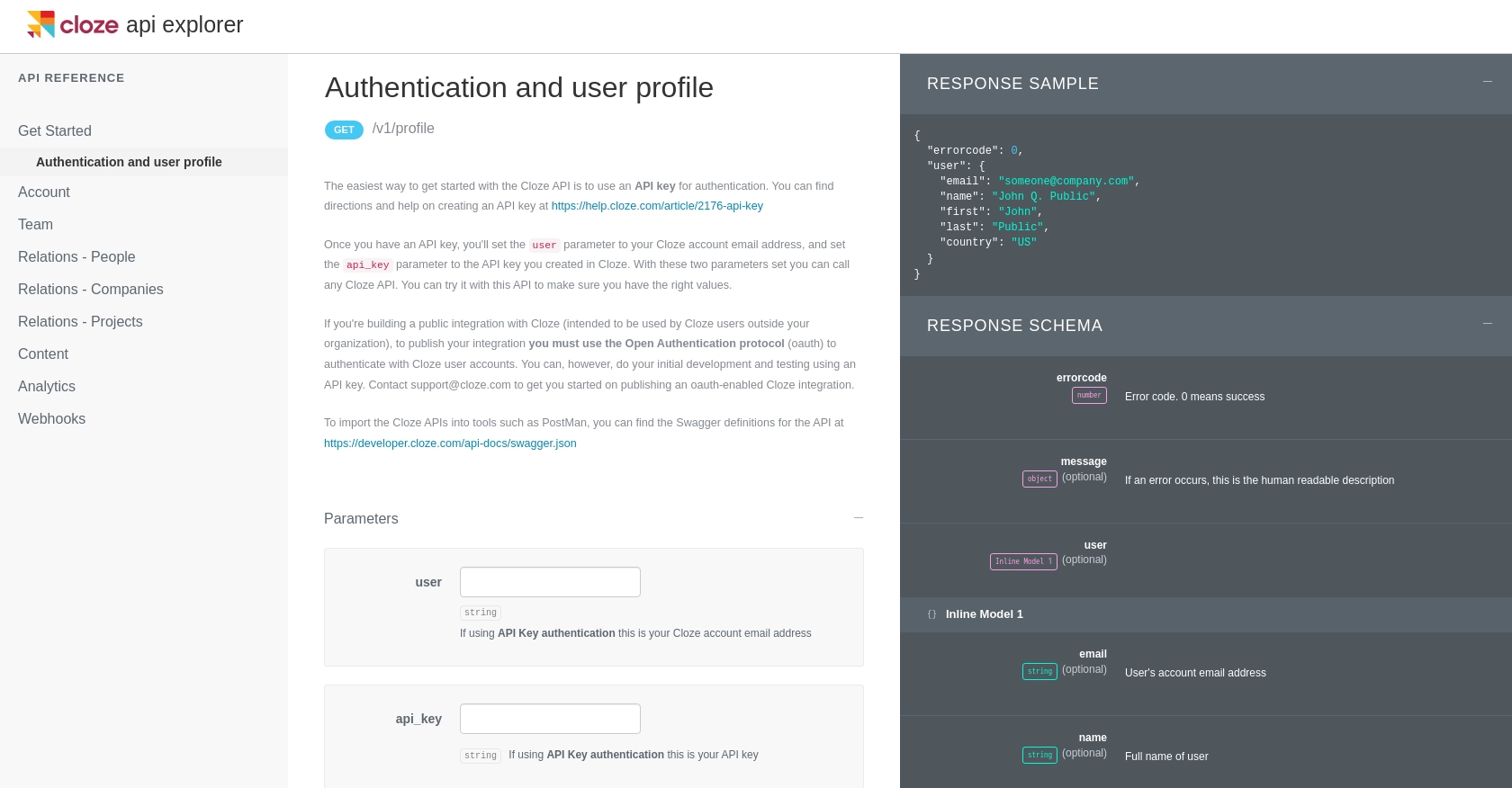
sbb-itb-96038d7
Making API Calls to Retrieve Users with Cloze API in PHP
To interact with the Cloze API using PHP, you'll need to set up your environment and write code to make HTTP requests. This section will guide you through the necessary steps to retrieve user data from Cloze.
Setting Up Your PHP Environment for Cloze API Integration
Before making API calls, ensure you have PHP installed on your machine. This tutorial assumes you are using PHP 7.4 or later. Additionally, you'll need the cURL
extension enabled, which is commonly used for making HTTP requests in PHP.
Installing Required PHP Dependencies
To make HTTP requests, we'll use the cURL
library. Ensure it's enabled in your php.ini
file. You can verify this by running the following command:
php -m | grep curl
If cURL
is not listed, enable it by uncommenting the line extension=curl
in your php.ini
file and restart your web server.
Writing PHP Code to Retrieve Users from Cloze API
Create a new PHP file named get_cloze_users.php
and add the following code:
<?php
// Cloze API endpoint for retrieving team members
$endpoint = "https://api.cloze.com/v1/team/members";
// Your Cloze account email and API key
$user = "your_email@domain.com";
$api_key = "your_api_key";
// Initialize cURL session
$ch = curl_init();
// Set cURL options
curl_setopt($ch, CURLOPT_URL, $endpoint);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Authorization: Bearer $api_key",
"Content-Type: application/json"
]);
// Execute cURL request
$response = curl_exec($ch);
// Check for errors
if (curl_errno($ch)) {
echo 'Error:' . curl_error($ch);
} else {
// Decode JSON response
$data = json_decode($response, true);
// Check for success
if ($data['errorcode'] === 0) {
// Loop through users and print their information
foreach ($data['list'] as $user) {
echo "Name: " . $user['name'] . " - Email: " . $user['email'] . "\n";
}
} else {
echo "Failed to retrieve users. Error code: " . $data['errorcode'];
}
}
// Close cURL session
curl_close($ch);
?>
Replace your_email@domain.com
and your_api_key
with your actual Cloze account email and API key.
Running the PHP Script to Fetch User Data
Run the script from the command line using the following command:
php get_cloze_users.php
You should see a list of users from your Cloze account displayed in the terminal.
Handling Errors and Verifying API Requests
Ensure that your API requests are successful by checking the errorcode
in the response. An error code of 0 indicates success. If you encounter errors, refer to the Cloze API documentation for more information on error codes and troubleshooting.
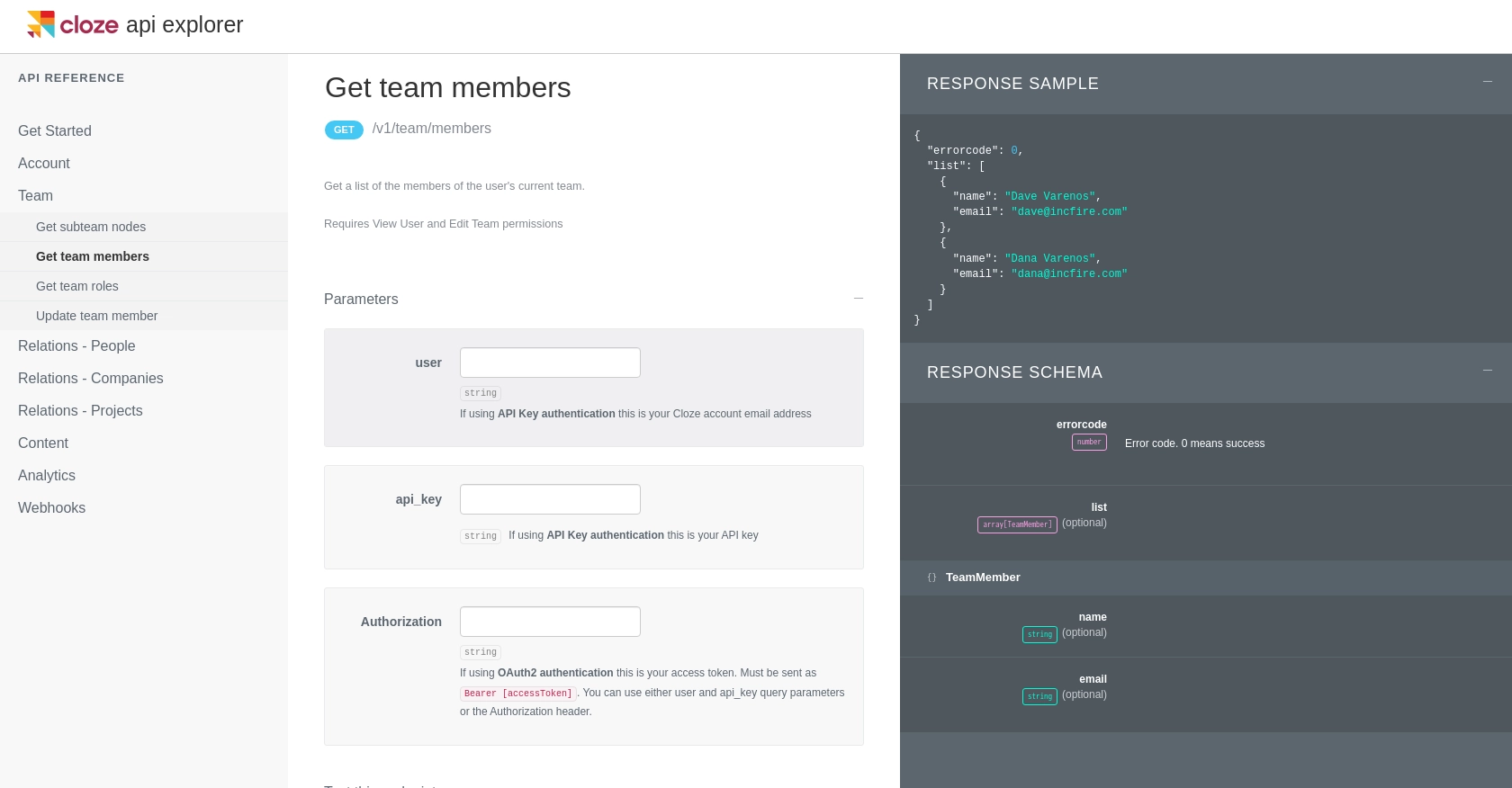
Conclusion and Best Practices for Using Cloze API with PHP
Integrating with the Cloze API using PHP allows developers to efficiently manage user data and enhance team collaboration. By following the steps outlined in this guide, you can seamlessly retrieve user information and leverage Cloze's capabilities to build robust applications.
Best Practices for Storing Cloze API Credentials Securely
- Store your API keys and user credentials in a secure location, such as environment variables or a secure vault, to prevent unauthorized access.
- Avoid hardcoding sensitive information directly in your codebase.
Handling Rate Limiting and API Request Optimization
To ensure smooth API interactions, be mindful of Cloze's rate limits. Implement strategies such as request batching and caching to minimize the number of API calls and optimize performance.
Data Transformation and Standardization
When integrating with the Cloze API, consider transforming and standardizing data fields to align with your application's requirements. This ensures consistency and improves data quality across your systems.
Explore More with Endgrate for Seamless Integrations
If you're looking to simplify your integration process and focus on your core product, consider using Endgrate. With Endgrate, you can save time and resources by outsourcing integrations, building once for each use case, and providing an intuitive integration experience for your customers. Learn more at Endgrate.
Read More
Ready to get started?