Using the Intercom API to Create Or Update Companies in Python
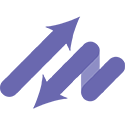
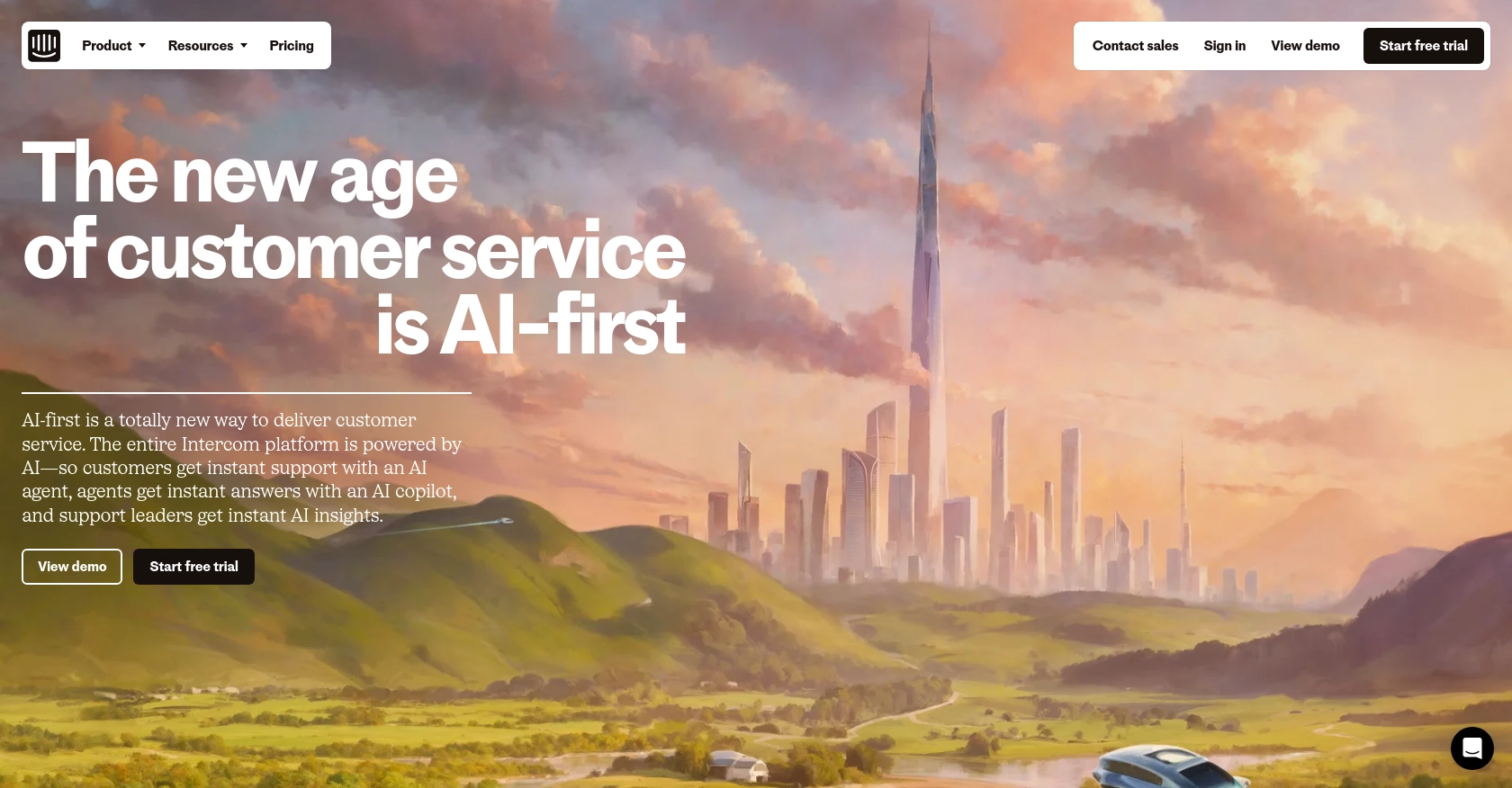
Introduction to Intercom API for Company Management
Intercom is a powerful customer communication platform that enables businesses to engage with their customers through personalized messaging and support. It offers a suite of tools for managing customer interactions, including chat, email, and in-app messaging, making it a popular choice for businesses looking to enhance their customer experience.
Developers may want to integrate with Intercom's API to manage company data efficiently. By using the Intercom API, developers can automate the process of creating or updating company records, ensuring that customer information is always up-to-date. For example, a developer might use the Intercom API to automatically update company details when changes occur in an external CRM system, streamlining data management and reducing manual entry.
Setting Up Your Intercom Developer Account for API Integration
Before you can start using the Intercom API to create or update companies, you need to set up a developer account. This involves creating a development workspace and configuring OAuth for authentication. Follow these steps to get started:
Create a Free Intercom Developer Workspace
- Visit the Intercom Developer Hub and sign up for a free developer account.
- Once registered, create a development workspace. This will give you access to the necessary tools and settings to build and test your integration.
Configure OAuth for Intercom API Access
Intercom uses OAuth for authentication, allowing your app to securely access user data. Here's how to set it up:
- In your Intercom Developer Hub, navigate to the Authentication section.
- Enable the Use OAuth option.
- Provide the necessary information, including redirect URLs. Ensure these URLs use HTTPS for secure communication.
- Select the required permissions for your app, such as reading and writing company data.
- Save your settings to generate a Client ID and Client Secret, which you'll use to authenticate API requests.
For more detailed instructions, refer to the Intercom OAuth setup guide.
Generate an Access Token
Once OAuth is configured, you'll need to obtain an access token to authenticate your API requests:
- Direct users to the Intercom authorization URL, including your Client ID and a unique state parameter for security.
- After users authorize your app, they'll be redirected to your specified URL with an authorization code.
- Exchange this code for an access token by making a POST request to the Intercom token endpoint.
import requests
url = "https://api.intercom.io/auth/eagle/token"
data = {
"code": "AUTHORIZATION_CODE",
"client_id": "YOUR_CLIENT_ID",
"client_secret": "YOUR_CLIENT_SECRET"
}
response = requests.post(url, data=data)
access_token = response.json().get("access_token")
print("Access Token:", access_token)
With the access token, you can now make authenticated requests to the Intercom API. For more information, visit the Intercom API documentation.
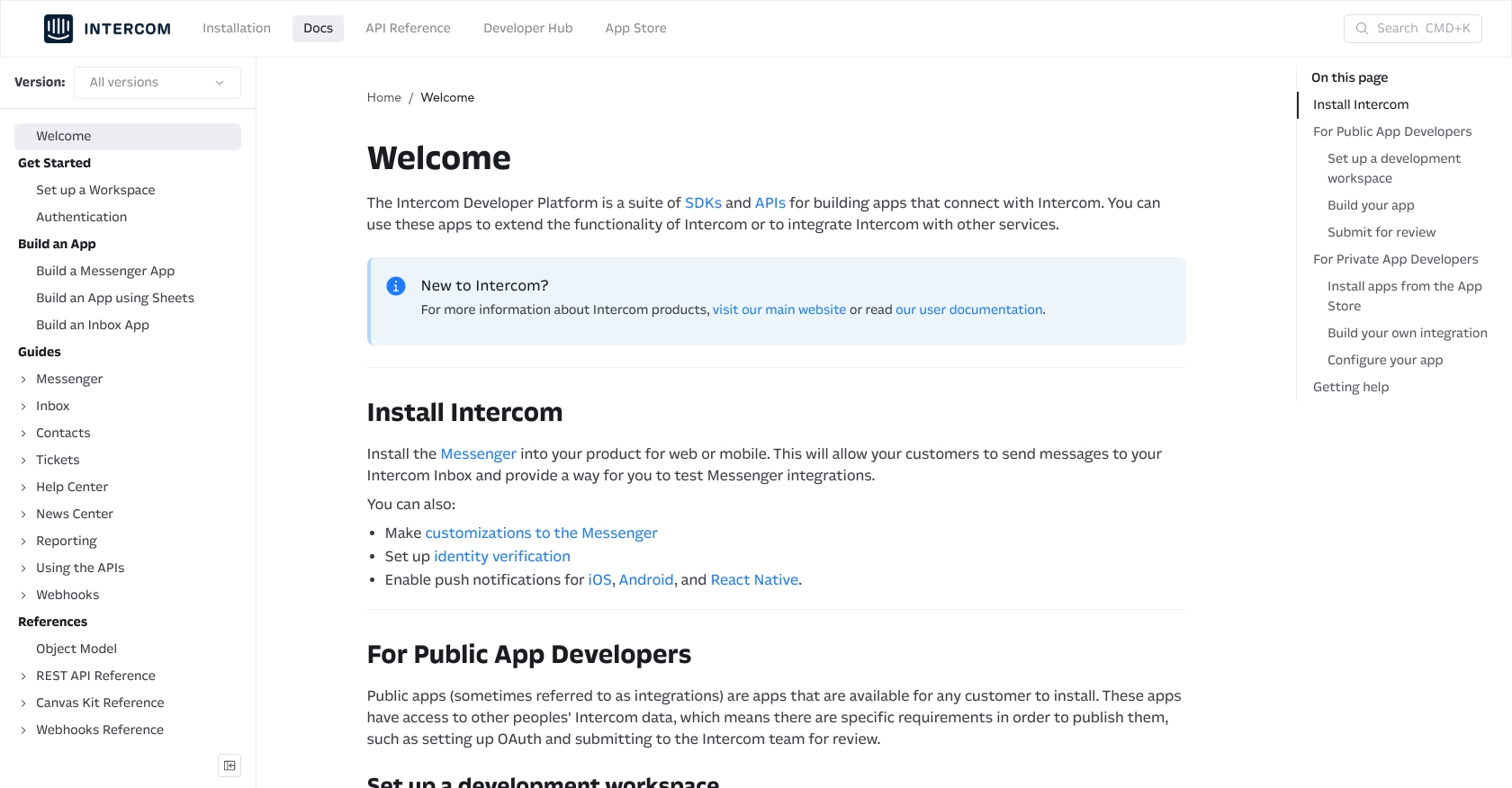
sbb-itb-96038d7
Making API Calls to Intercom for Company Management Using Python
To interact with the Intercom API for creating or updating companies, you'll need to use Python. This section will guide you through the process of setting up your environment, writing the necessary code, and handling responses effectively.
Setting Up Your Python Environment for Intercom API Integration
Before making API calls, ensure you have the following prerequisites installed:
- Python 3.11.1 or later
- The Python package installer,
pip
Install the requests
library to handle HTTP requests:
pip install requests
Creating or Updating Companies with the Intercom API
With your environment ready, you can now write a Python script to create or update companies in Intercom. Use the following code as a template:
import requests
# Define the API endpoint and headers
url = "https://api.intercom.io/companies"
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"Content-Type": "application/json",
"Intercom-Version": "2.11"
}
# Define the company data
company_data = {
"company_id": "unique_company_id",
"name": "Example Company",
"remote_created_at": 1663597223,
"custom_attributes": {
"industry": "Software",
"monthly_spend": 1000
}
}
# Make the POST request to create or update the company
response = requests.post(url, json=company_data, headers=headers)
# Check if the request was successful
if response.status_code == 200:
print("Company created or updated successfully:", response.json())
else:
print("Failed to create or update company:", response.status_code, response.json())
Replace YOUR_ACCESS_TOKEN
with the access token obtained during the OAuth setup. The company_id
should be unique for each company, as it cannot be updated once set.
Verifying API Call Success and Handling Errors
After running the script, verify the success of your API call by checking the response:
- If successful, the response will contain the company details, confirming the creation or update.
- If unsuccessful, the response will include error details. Common error codes include 400 (Bad Request) and 401 (Unauthorized). Refer to the Intercom API documentation for more information on error handling.
To ensure your integration runs smoothly, handle errors gracefully by logging them and implementing retry logic if necessary.
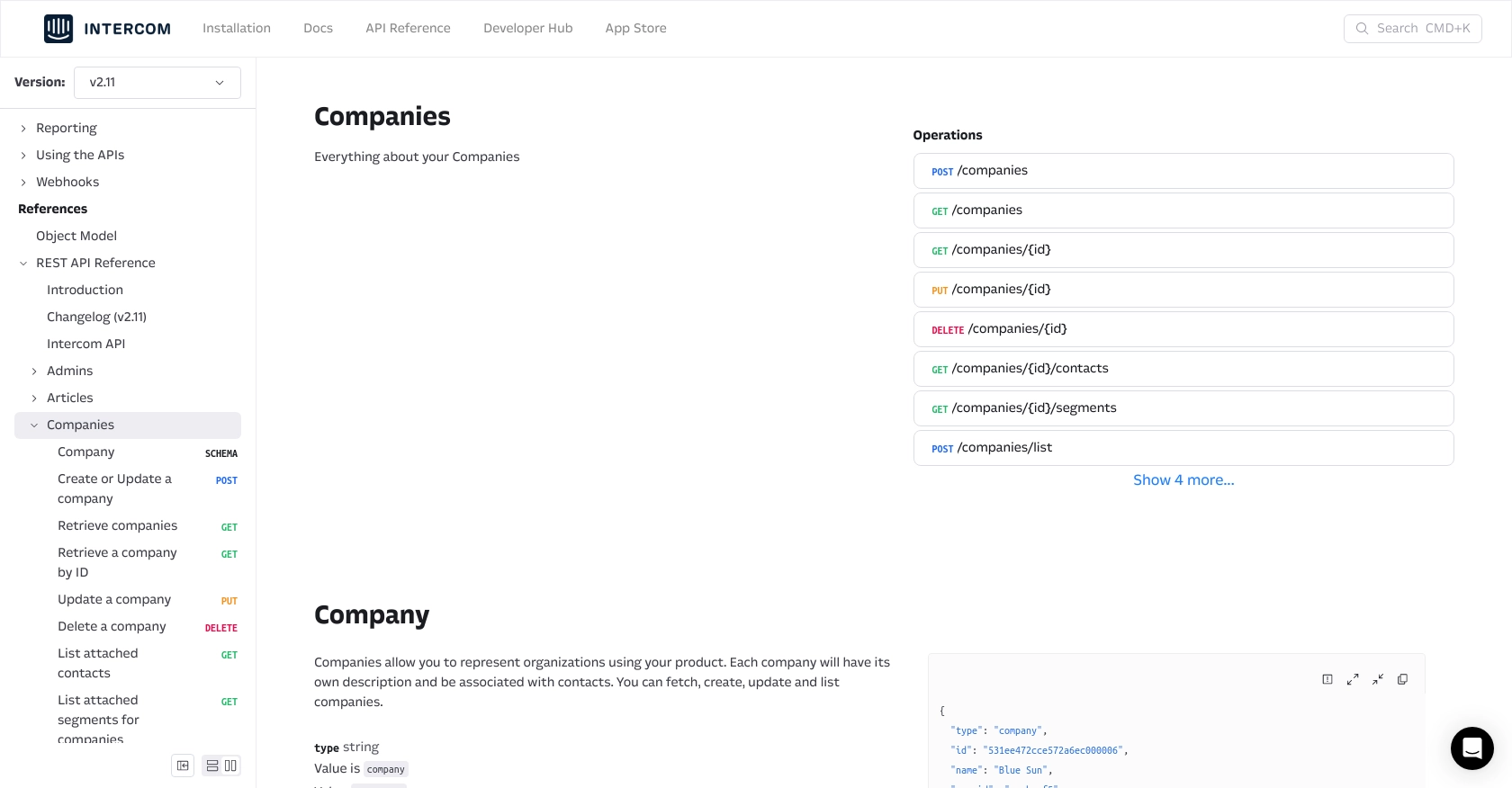
Best Practices for Intercom API Integration
Successfully integrating with the Intercom API requires adherence to best practices to ensure security, efficiency, and maintainability. Here are some recommendations:
- Securely Store Credentials: Always store your API credentials, such as the client ID, client secret, and access tokens, securely. Use environment variables or secure vaults to prevent unauthorized access.
- Handle Rate Limiting: Be aware of Intercom's rate limits to avoid exceeding them. Implement retry logic with exponential backoff to handle rate limit errors gracefully. For more details, refer to the Intercom API documentation.
- Standardize Data Fields: Ensure that data fields are consistent and standardized across your application to avoid discrepancies and data integrity issues.
- Implement Error Handling: Use robust error handling to manage API errors effectively. Log errors for debugging and consider implementing alerting mechanisms for critical failures.
Streamlining Intercom Integrations with Endgrate
Building and maintaining integrations can be time-consuming and complex. Endgrate simplifies this process by providing a unified API endpoint that connects to multiple platforms, including Intercom. By using Endgrate, you can:
- Save time and resources by outsourcing integration development, allowing you to focus on your core product.
- Build once for each use case instead of multiple times for different integrations, reducing redundancy and complexity.
- Offer an intuitive integration experience for your customers, enhancing user satisfaction and engagement.
Explore how Endgrate can transform your integration strategy by visiting Endgrate's website.
Read More
Ready to get started?