Using the Chargebee API to Get Customers (with Python examples)
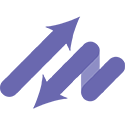
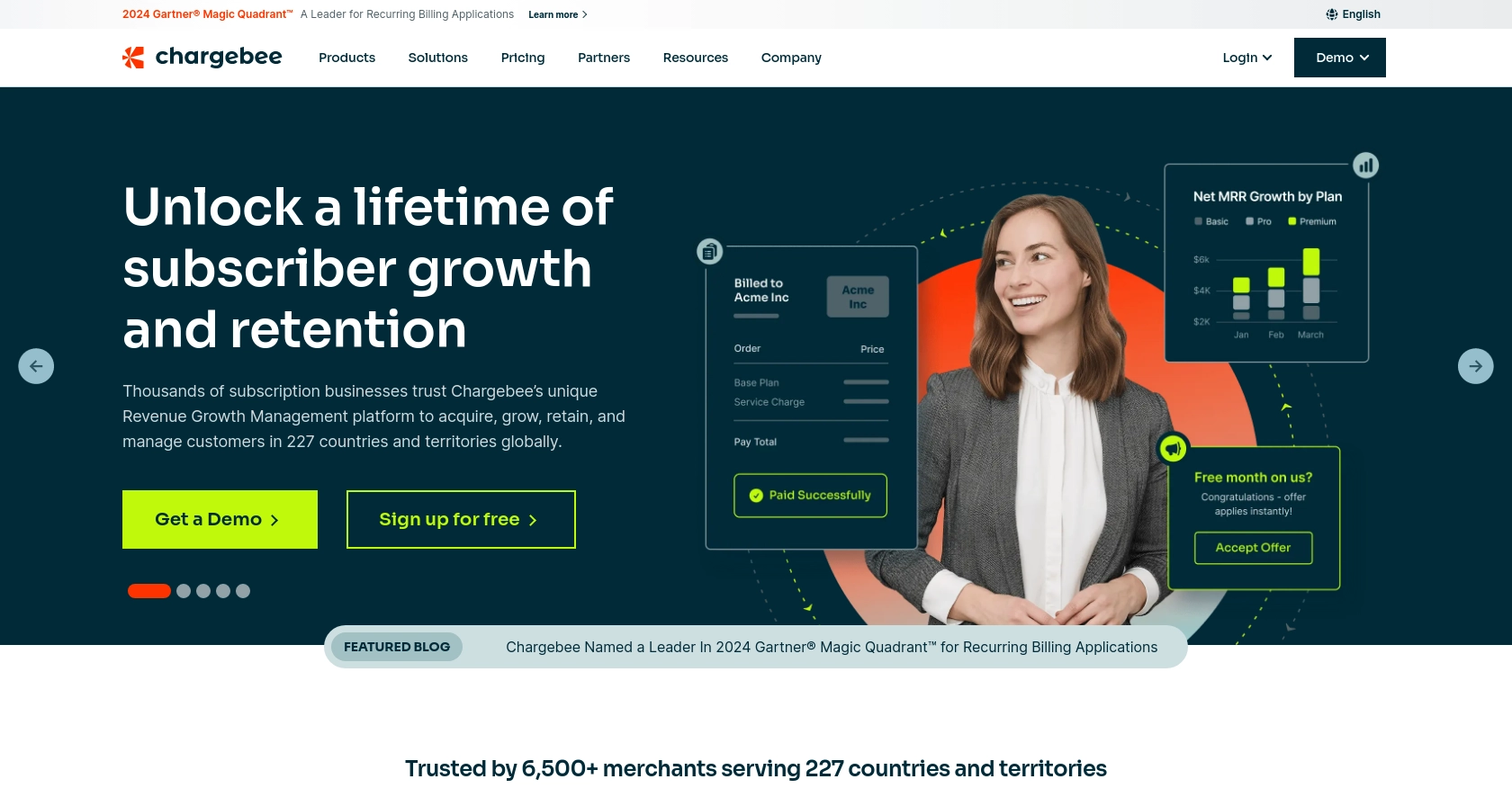
Introduction to Chargebee API Integration
Chargebee is a robust subscription management and billing platform designed to streamline the financial operations of SaaS businesses. It offers a comprehensive suite of tools for managing subscriptions, invoicing, and revenue recognition, making it an essential tool for businesses looking to automate their billing processes.
Integrating with Chargebee's API allows developers to efficiently manage customer data and automate billing workflows. For example, a developer might use the Chargebee API to retrieve customer information and synchronize it with their internal CRM system, ensuring that all customer data is up-to-date and accurate.
This article will guide you through using Python to interact with the Chargebee API, specifically focusing on retrieving customer data. By following this tutorial, you'll learn how to set up your environment, authenticate with Chargebee, and execute API calls to manage customer information effectively.
Setting Up Your Chargebee Test or Sandbox Account
Before you can start integrating with the Chargebee API, you'll need to set up a test or sandbox account. This environment allows you to safely experiment with API calls without affecting live data. Chargebee provides a dedicated test site for developers to simulate real-world scenarios and ensure their integration works as expected.
Creating a Chargebee Test Account
To get started, follow these steps to create your Chargebee test account:
- Visit the Chargebee signup page and register for a new account.
- During the signup process, select the option to create a test site. This will give you access to a sandbox environment where you can test your API integrations.
- Once your account is set up, log in to the Chargebee dashboard to access your test site.
Generating API Keys for Chargebee Authentication
Chargebee uses HTTP Basic authentication for API calls, where the username is your API key, and the password is left blank. Follow these steps to generate your API keys:
- Navigate to the Settings section in the Chargebee dashboard.
- Under API & Webhooks, find the API Keys section.
- Click on Create a Key to generate a new API key for your test site.
- Copy the generated API key and store it securely, as you will need it to authenticate your API requests.
Configuring Chargebee API Access
With your API key ready, you can now configure your application to interact with the Chargebee API. Ensure that your API requests are directed to the test site URL, which typically follows the format: https://{your-test-site}.chargebee.com/api/v2/
.
By setting up your Chargebee test account and generating the necessary API keys, you're now ready to begin integrating with the Chargebee API using Python. In the next section, we'll explore how to make API calls to retrieve customer data.
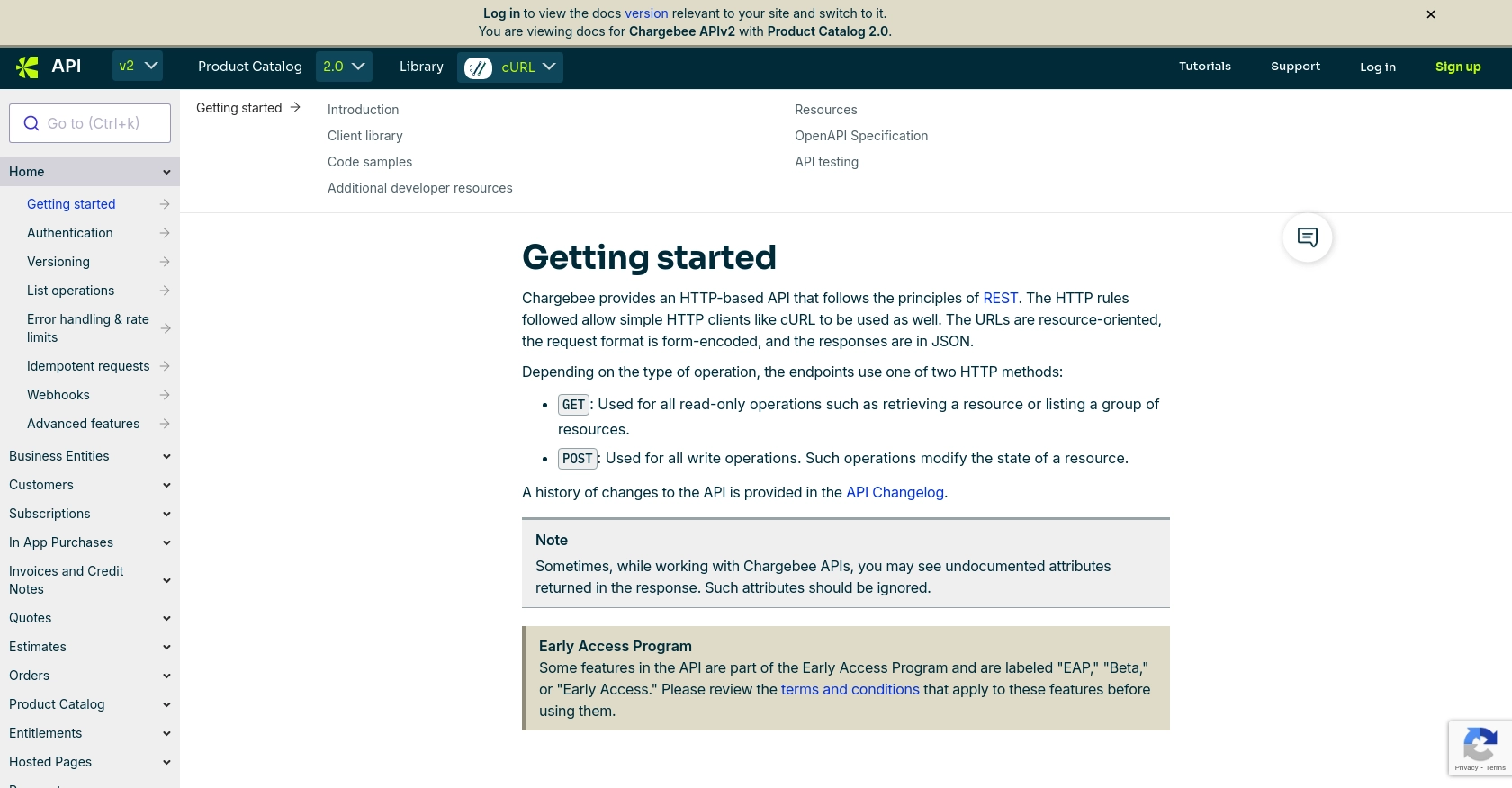
sbb-itb-96038d7
Making API Calls to Retrieve Customer Data from Chargebee Using Python
To interact with the Chargebee API and retrieve customer data, you'll need to set up your Python environment and execute the appropriate API calls. This section will guide you through the process, including setting up your environment, writing the code, and handling potential errors.
Setting Up Your Python Environment for Chargebee API Integration
Before making API calls, ensure you have the necessary tools and libraries installed. You'll need Python 3.x and the requests
library to handle HTTP requests.
- Ensure Python is installed on your machine. You can download it from the official Python website.
- Install the
requests
library by running the following command in your terminal:
pip install requests
Writing Python Code to Retrieve Customers from Chargebee
With your environment set up, you can now write the Python code to make API calls to Chargebee. Below is an example script to retrieve a list of customers:
import requests
# Set the Chargebee API endpoint and your test site URL
url = "https://{your-test-site}.chargebee.com/api/v2/customers"
# Set your API key for authentication
api_key = "your_api_key_here"
# Make a GET request to the Chargebee API
response = requests.get(url, auth=(api_key, ''))
# Check if the request was successful
if response.status_code == 200:
# Parse and print the JSON response
customers = response.json()
for customer in customers['list']:
print(f"Customer ID: {customer['customer']['id']}, Email: {customer['customer']['email']}")
else:
print(f"Failed to retrieve customers: {response.status_code} - {response.text}")
Replace {your-test-site}
with your actual test site name and your_api_key_here
with your Chargebee API key.
Handling API Response and Error Codes
After executing the API call, it's crucial to handle the response correctly. The Chargebee API returns data in JSON format, and you can access customer details from the response object.
If the request fails, the response will include an error code and message. Common error codes include:
- 401 Unauthorized: Check if your API key is correct.
- 404 Not Found: Verify the endpoint URL.
- 429 Too Many Requests: You've exceeded the rate limit. Wait before retrying.
For more details on error handling, refer to the Chargebee API documentation.
Verifying Successful API Requests in Chargebee
To confirm that your API requests are successful, you can log in to your Chargebee test site and verify the customer data. If the API call retrieves customer information, it should match the data displayed in the Chargebee dashboard.
By following these steps, you can effectively use Python to interact with the Chargebee API and manage customer data. In the next section, we'll explore best practices for secure and efficient API integration.
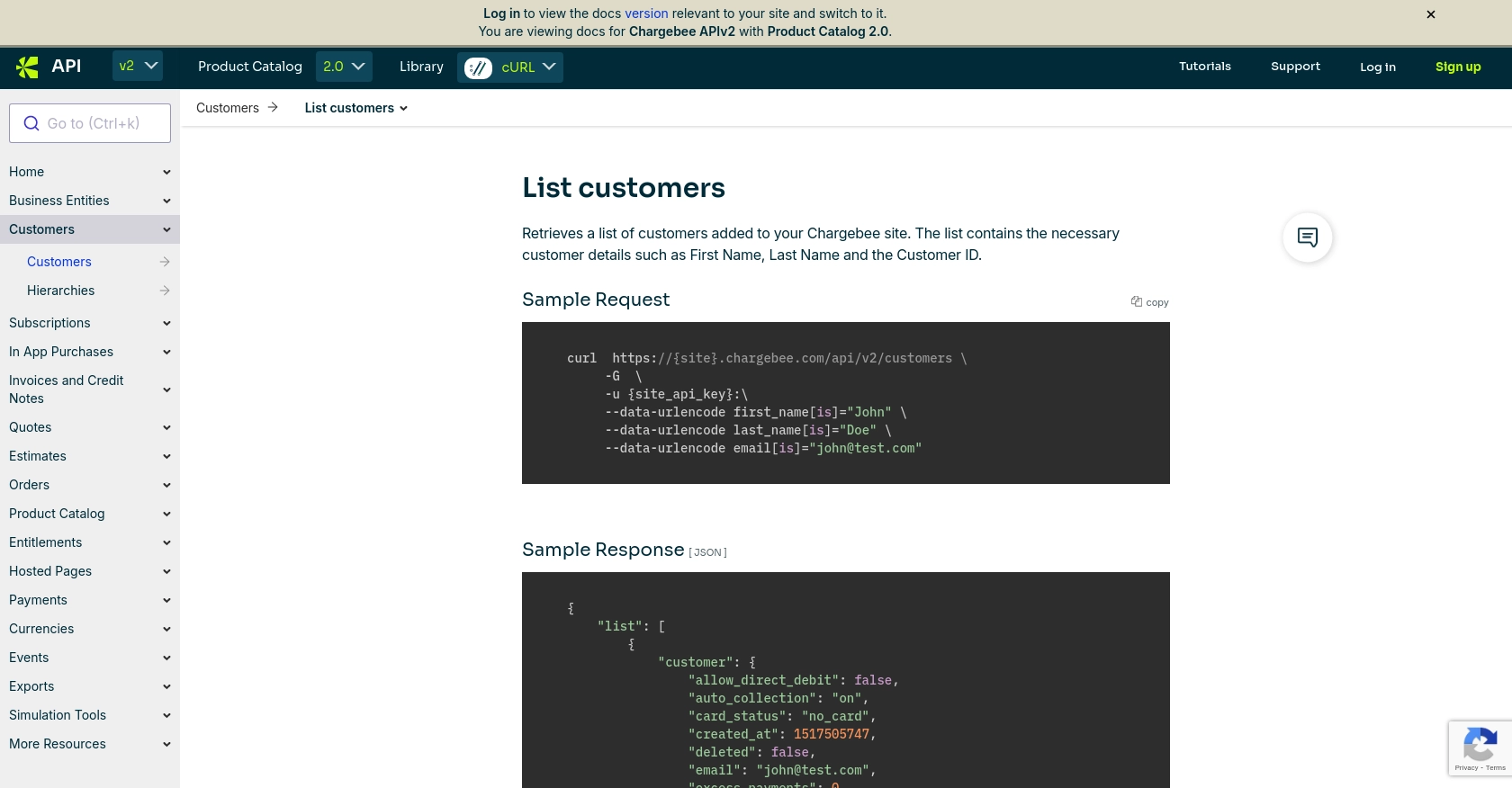
Best Practices for Secure and Efficient Chargebee API Integration
When integrating with the Chargebee API, it's essential to follow best practices to ensure security and efficiency. Here are some key recommendations:
Securely Storing Chargebee API Credentials
- Never hard-code API keys in your source code. Instead, use environment variables or secure vaults to store them.
- Regularly rotate your API keys and delete any that are no longer in use to minimize security risks.
Handling Chargebee API Rate Limits
Chargebee imposes rate limits to ensure fair usage of its API. For test sites, the limit is approximately 750 API calls every 5 minutes, while live sites have a limit of 150 API calls per minute. To handle rate limits effectively:
- Implement exponential backoff strategies to retry requests after receiving a 429 Too Many Requests error.
- Monitor your API usage and adjust your integration to stay within the allowed limits.
Transforming and Standardizing Customer Data from Chargebee
When retrieving customer data from Chargebee, ensure that the data is transformed and standardized to match your internal systems. This may involve:
- Mapping Chargebee customer attributes to your CRM fields.
- Normalizing data formats, such as dates and currencies, to maintain consistency across platforms.
Conclusion: Streamlining Integrations with Endgrate
Integrating with Chargebee's API can significantly enhance your SaaS product's billing and subscription management capabilities. However, building and maintaining multiple integrations can be time-consuming and complex.
Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Chargebee. By leveraging Endgrate, you can focus on your core product while outsourcing the intricacies of integration management. This approach not only saves time and resources but also ensures a seamless and intuitive experience for your customers.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?