How to Get Active Users Count with the Mode API in Javascript
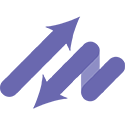
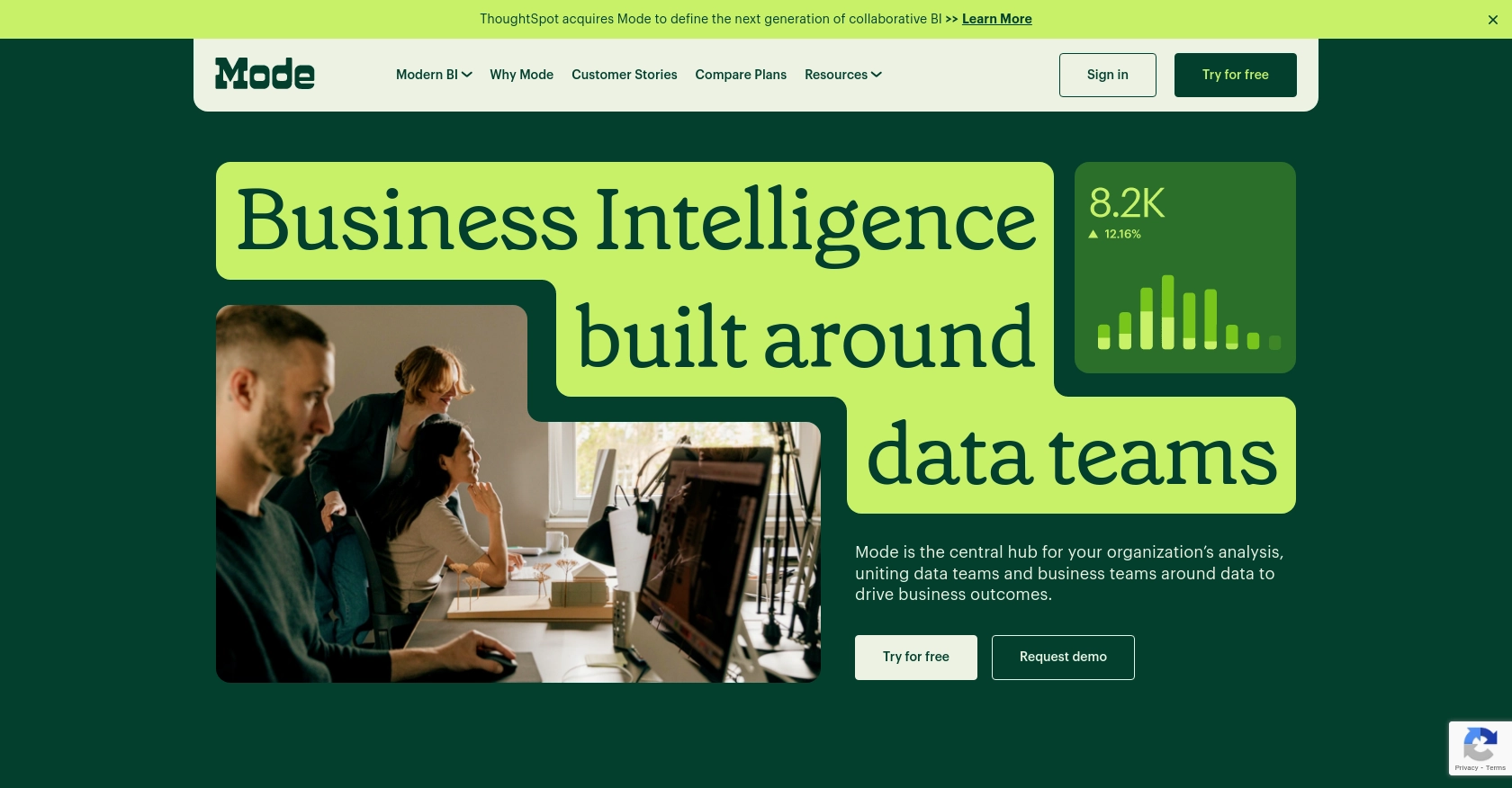
Introduction to Mode API for Active User Management
Mode is a collaborative analytics platform that empowers teams to make data-driven decisions through seamless integration and powerful analytics tools. By leveraging Mode's API, developers can programmatically access and manage various analytics functions, including querying data, generating reports, and managing workspace memberships.
Integrating with the Mode API allows developers to efficiently track and manage user activity within their workspaces. For example, you might want to retrieve the count of active users to monitor engagement levels or allocate resources effectively. This tutorial will guide you through using JavaScript to interact with the Mode API and obtain the active users count in your workspace.
Setting Up Your Mode API Test Account
Before diving into the Mode API to retrieve active user counts, you'll need to set up a Mode account and obtain the necessary API credentials. This setup will allow you to authenticate your requests and interact with the Mode API seamlessly.
Create a Mode Account and Workspace
If you don't already have a Mode account, follow these steps to create one:
- Visit the Mode website and sign up for an account.
- Once registered, create a new Workspace. This Workspace will serve as the environment where you'll manage your analytics and user data.
- Connect a database or use public data to start exploring Mode's capabilities.
Generate a Mode API Access Token
To interact with the Mode API, you'll need an API access token. Follow these steps to generate one:
- Navigate to your Workspace Settings.
- Go to Privacy & Security and select API.
- Click the gear icon and choose Create new API token.
- Provide a display name for your token and save it securely. Remember, the token will only be shown once.
Ensure you store your API token and secret securely, as they are crucial for authenticating your API requests.
Authenticate Your API Requests
Mode uses basic authentication for API requests. Here's how to set it up:
- Combine your API token and secret into a string formatted as
username:password
. - Encode this string using BASE64 encoding.
- Include the encoded string in the HTTP header for authorization in each API call:
Authorization: Basic "BASE-64 encoded string"
For more details on authentication, refer to the Mode API Authentication Documentation.
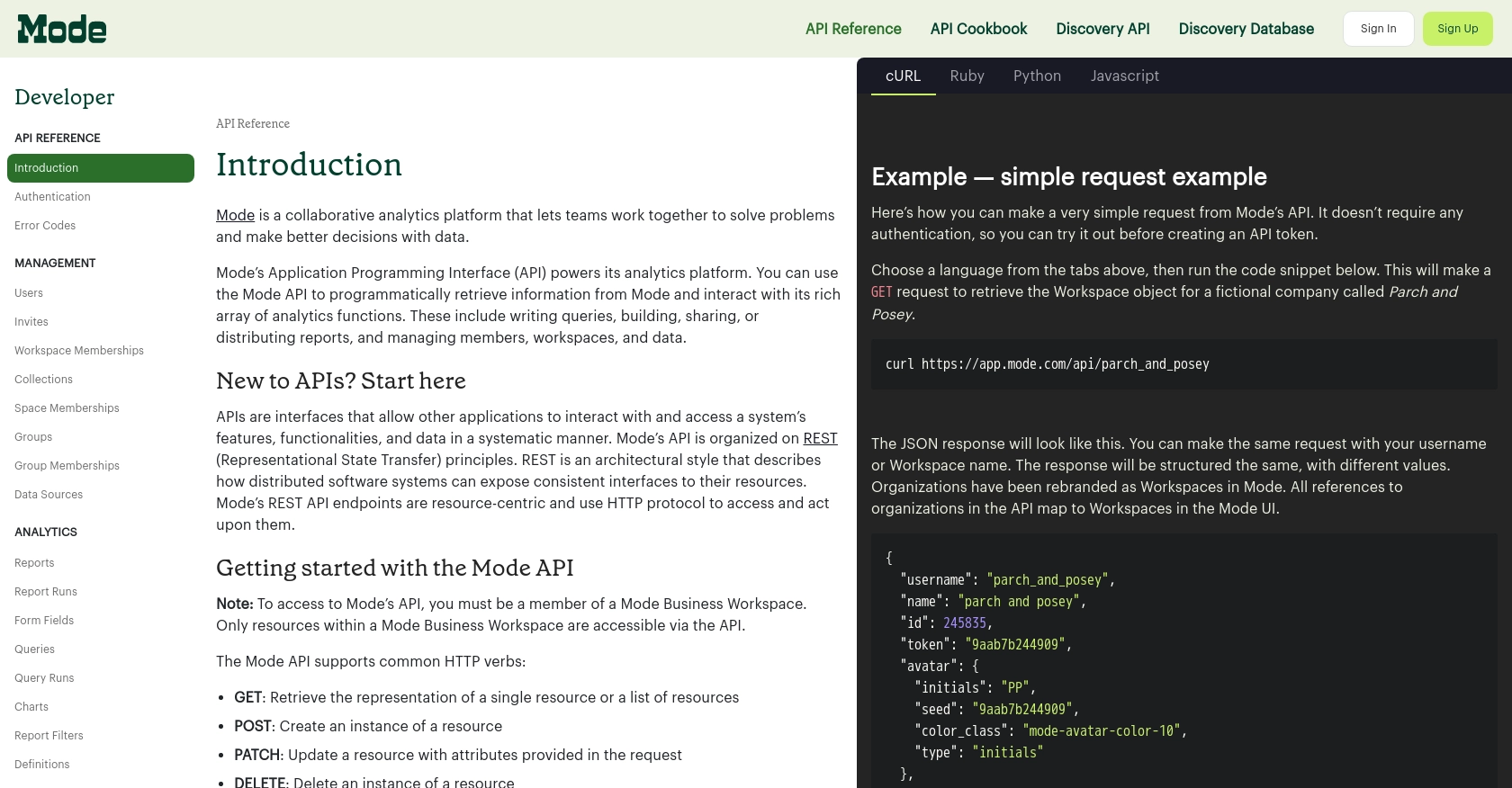
sbb-itb-96038d7
Executing API Calls to Retrieve Active Users Count with Mode API in JavaScript
To effectively interact with the Mode API using JavaScript, you need to ensure that your development environment is properly set up. This section will guide you through the necessary steps to make API calls and retrieve the active users count in your Mode Workspace.
Setting Up Your JavaScript Environment for Mode API
Before making API calls, ensure you have the following prerequisites:
- Node.js installed on your machine.
- A package manager like npm or yarn to manage dependencies.
Once you have these tools, you can install the request-promise
library, which simplifies making HTTP requests in JavaScript:
npm install request-promise
Making the API Call to Get Active Users Count
With your environment set up, you can now proceed to make the API call. The following code demonstrates how to retrieve the active users count from your Mode Workspace:
const request = require('request-promise');
const host = 'https://app.mode.com';
const username = 'your_api_token';
const password = 'your_api_secret';
const getActiveUsersCount = async () => {
try {
const response = await request({
method: 'GET',
url: `${host}/api/{workspace}/memberships`,
auth: { username, password },
json: true,
headers: {
'Content-Type': 'application/json',
'Accept': 'application/hal+json'
}
});
const activeUsers = response.filter(member => member.state === 'active');
console.log(`Active Users Count: ${activeUsers.length}`);
} catch (error) {
console.error('Error fetching active users:', error.message);
}
};
getActiveUsersCount();
Replace your_api_token
and your_api_secret
with your actual API credentials. The code above sends a GET request to the Mode API to list all memberships in your workspace and filters the response to count only active users.
Verifying the API Call Success
To ensure that your API call was successful, check the console output for the active users count. Additionally, you can verify the data by logging into your Mode Workspace and comparing the count with the active members listed there.
Handling Errors and Understanding Error Codes
When making API calls, it's crucial to handle potential errors gracefully. The Mode API uses standard HTTP response codes to indicate success or failure:
- 200 OK: The request was successful.
- 401 Unauthorized: Authentication failed. Check your API credentials.
- 404 Not Found: The requested resource could not be found.
For more detailed information on error codes, refer to the Mode API Error Codes Documentation.
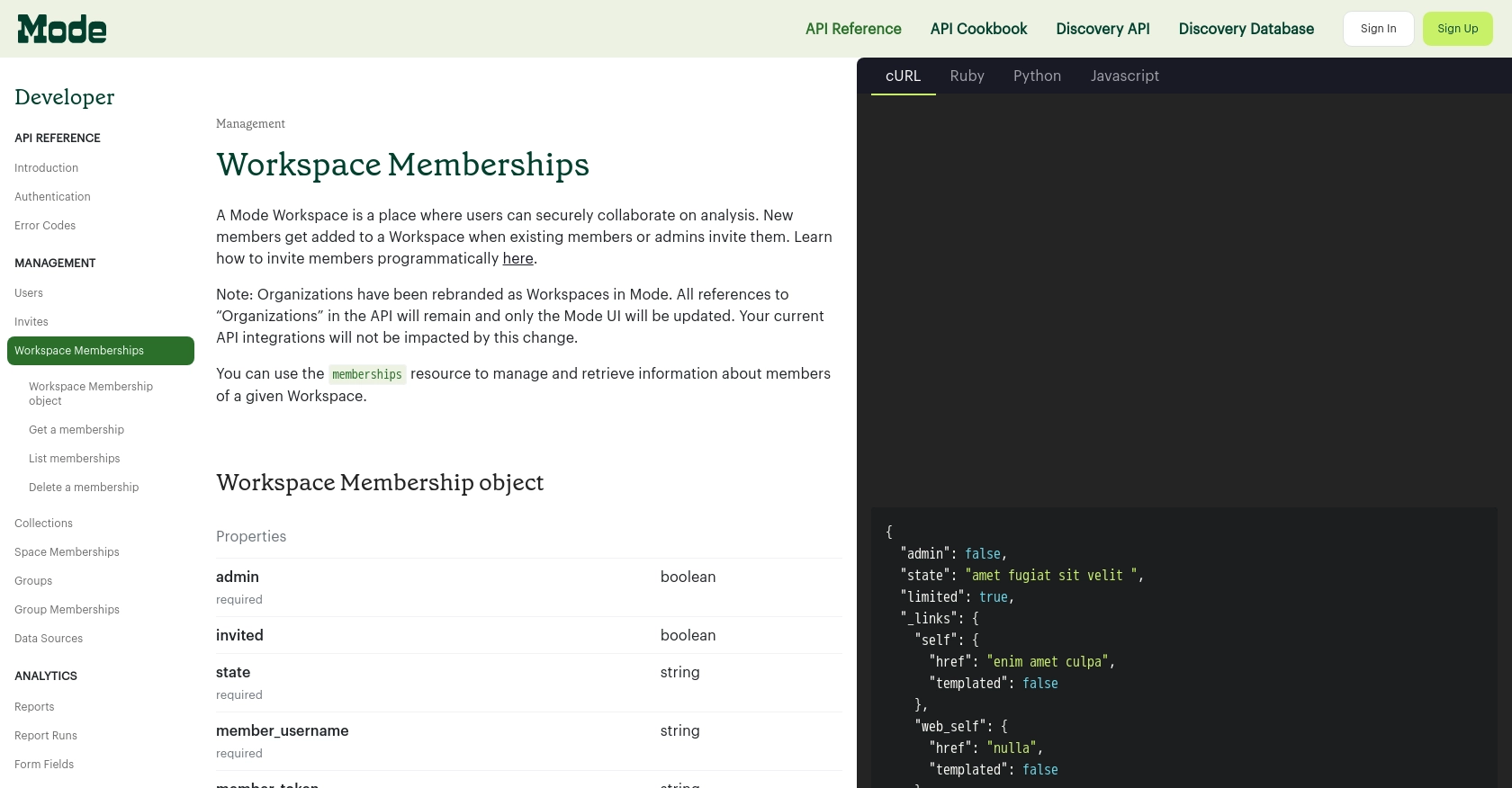
Best Practices for Using Mode API in JavaScript
When working with the Mode API to retrieve active user counts, it's essential to follow best practices to ensure secure and efficient integration. Here are some recommendations:
- Securely Store API Credentials: Always store your API token and secret securely. Avoid exposing them in client-side code or public repositories. Consider using environment variables or secure vaults for storage.
- Handle Rate Limiting: Be mindful of Mode's API rate limits to avoid throttling. Implement retry logic with exponential backoff to handle rate limit responses gracefully.
- Data Transformation and Standardization: Ensure that the data retrieved from the API is transformed and standardized according to your application's requirements. This will help maintain consistency across different integrations.
Streamline Your Integrations with Endgrate
Building and maintaining integrations can be time-consuming and complex, especially when dealing with multiple APIs. Endgrate simplifies this process by providing a unified API endpoint that connects to various platforms, including Mode.
By using Endgrate, you can:
- Save Time and Resources: Focus on your core product while Endgrate handles the intricacies of API integrations.
- Build Once, Deploy Anywhere: Create a single integration for each use case and deploy it across multiple platforms effortlessly.
- Enhance Customer Experience: Provide your customers with a seamless and intuitive integration experience.
Explore how Endgrate can transform your integration strategy by visiting Endgrate today.
Read More
Ready to get started?